Intro
Merge multiple Excel files with a single click using a Combine Excel Files Macro, streamlining data consolidation and automation with VBA scripting and worksheet integration.
Merging Excel files can be a tedious task, especially when dealing with multiple files. Fortunately, Excel provides a feature to combine files using macros. Macros are a series of instructions that can be executed with a single command, making it easier to automate repetitive tasks. In this article, we will explore the importance of combining Excel files, the benefits of using macros, and provide a step-by-step guide on how to create a macro to merge Excel files.
The ability to combine Excel files is crucial in various industries, such as finance, marketing, and sales. It helps to consolidate data, simplify analysis, and improve decision-making. For instance, a marketing team may need to combine sales data from different regions to analyze overall performance. By merging Excel files, they can easily compare data, identify trends, and create comprehensive reports.
Using macros to combine Excel files offers several benefits. It saves time and effort, reduces errors, and increases productivity. Macros can be customized to meet specific needs, and they can be reused multiple times, making it an efficient solution for repetitive tasks. Additionally, macros can be used to perform complex tasks, such as data validation, formatting, and calculations, which can be challenging to do manually.
Getting Started with Macros
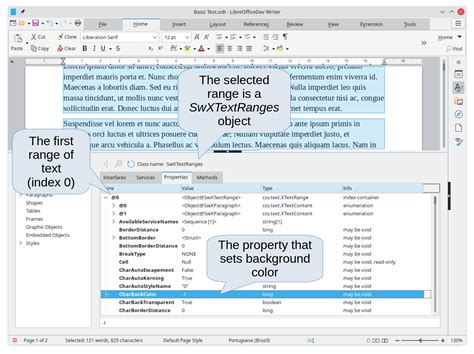
To create a macro to combine Excel files, you need to have a basic understanding of Excel and macros. Here are the steps to follow:
- Open the Visual Basic Editor: Press Alt + F11 or navigate to Developer > Visual Basic in the ribbon.
- Create a new module: In the Visual Basic Editor, click Insert > Module to create a new module.
- Write the macro code: In the module, write the code to combine Excel files. You can use the following code as an example:
Sub CombineExcelFiles()
Dim folderPath As String
Dim fileName As String
Dim wb As Workbook
Dim ws As Worksheet
' Set the folder path and file name
folderPath = "C:\Path\To\Folder\"
fileName = "CombinedFile.xlsx"
' Create a new workbook
Set wb = Workbooks.Add
' Loop through all files in the folder
fileName = Dir(folderPath & "*.xlsx")
While fileName <> ""
' Open the file
Workbooks.Open folderPath & fileName
' Loop through all worksheets in the file
For Each ws In ActiveWorkbook.Worksheets
' Copy the worksheet to the new workbook
ws.Copy After:=wb.Worksheets(wb.Worksheets.Count)
Next ws
' Close the file
ActiveWorkbook.Close False
' Get the next file
fileName = Dir
Wend
' Save the combined file
wb.SaveAs folderPath & fileName
' Close the combined file
wb.Close False
End Sub
- Save the macro: Click File > Save to save the macro.
How to Use the Macro
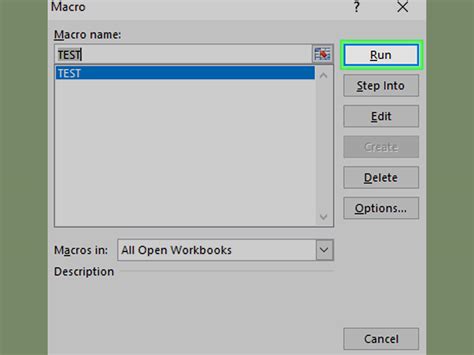
To use the macro, follow these steps:
- Open the Excel file that contains the macro.
- Click Developer > Macros in the ribbon.
- Select the macro from the list and click Run.
- The macro will prompt you to select the folder that contains the files you want to combine.
- Select the folder and click OK.
- The macro will combine the files and save the combined file in the same folder.
Benefits of Using Macros
Using macros to combine Excel files offers several benefits, including:- Time-saving: Macros can automate repetitive tasks, saving you time and effort.
- Error-reduction: Macros can reduce errors by performing tasks consistently and accurately.
- Increased productivity: Macros can help you complete tasks faster, allowing you to focus on more important tasks.
- Customization: Macros can be customized to meet specific needs, making it an efficient solution for unique tasks.
Common Errors and Troubleshooting
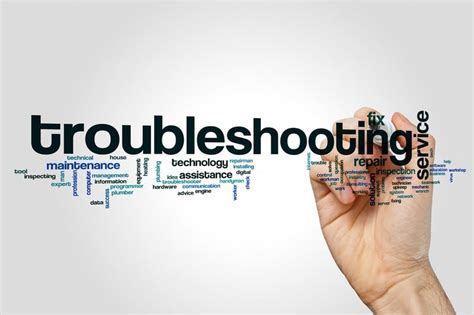
When using macros to combine Excel files, you may encounter errors or issues. Here are some common errors and troubleshooting tips:
- Error 1: The macro is not running.
- Solution: Check if the macro is enabled and if the file is saved as a macro-enabled file.
- Error 2: The macro is not combining files correctly.
- Solution: Check if the file path and name are correct, and if the files are in the correct format.
- Error 3: The macro is taking too long to run.
- Solution: Check if the files are large or if there are too many files to combine. You can also try optimizing the macro code to improve performance.
Best Practices for Using Macros
To get the most out of using macros to combine Excel files, follow these best practices:- Use meaningful variable names and comments to make the code easy to understand.
- Test the macro thoroughly to ensure it works correctly.
- Use error handling to catch and handle errors.
- Keep the macro code organized and concise.
Advanced Techniques for Combining Excel Files
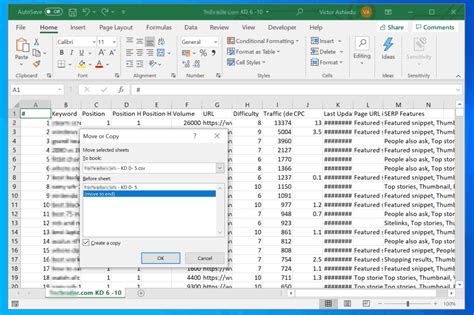
Once you have mastered the basics of using macros to combine Excel files, you can explore advanced techniques to improve performance and functionality. Here are some advanced techniques to consider:
- Using arrays to store and manipulate data.
- Using loops to iterate through files and worksheets.
- Using conditional statements to handle errors and exceptions.
- Using functions to perform calculations and data validation.
Real-World Applications of Combining Excel Files
Combining Excel files has numerous real-world applications, including:- Consolidating sales data from different regions.
- Merging customer data from different sources.
- Combining financial data from different departments.
- Analyzing data from different experiments or studies.
Gallery of Excel Files
Excel Files Image Gallery
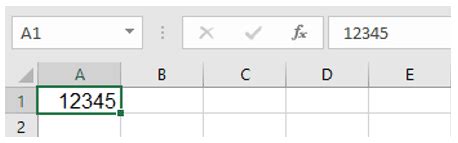
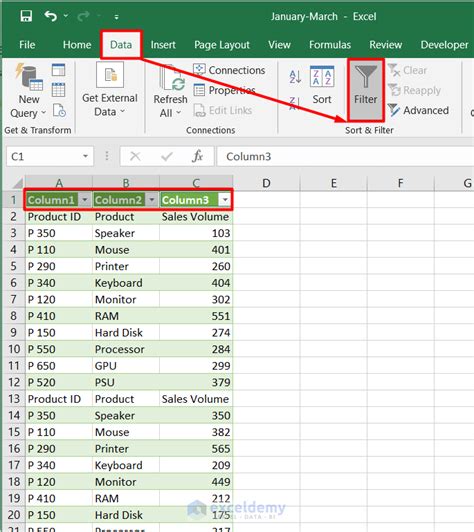
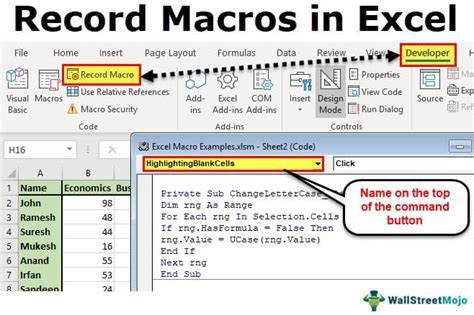

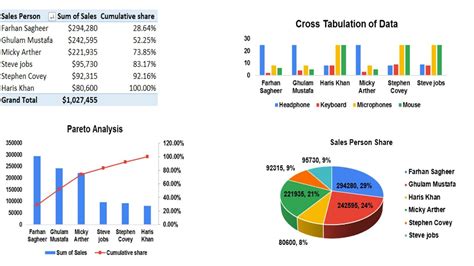
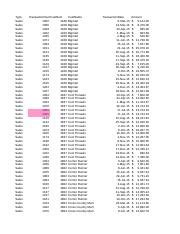
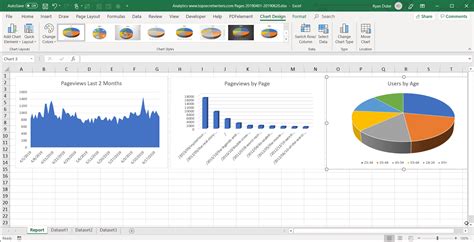
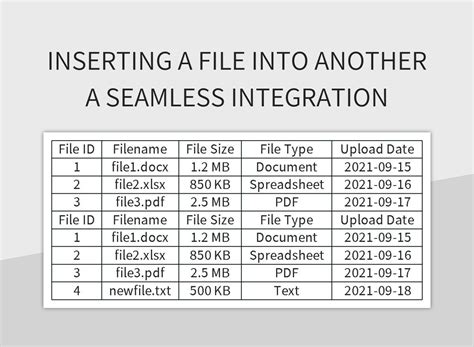
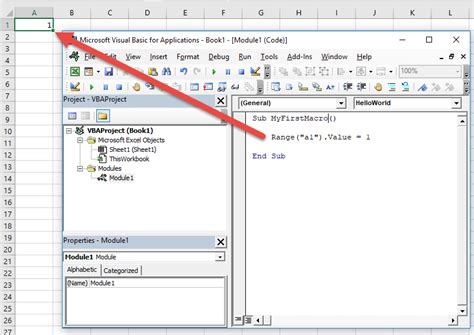
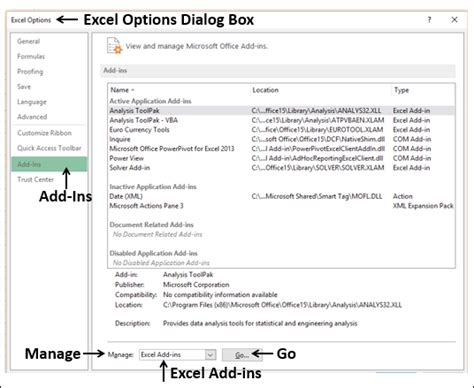
What is a macro in Excel?
+A macro is a series of instructions that can be executed with a single command, making it easier to automate repetitive tasks.
How do I create a macro in Excel?
+To create a macro, open the Visual Basic Editor, click Insert > Module, and write the macro code.
What are the benefits of using macros to combine Excel files?
+Using macros to combine Excel files offers several benefits, including time-saving, error-reduction, and increased productivity.
In conclusion, combining Excel files using macros is a powerful tool that can save time, reduce errors, and increase productivity. By following the steps outlined in this article, you can create a macro to combine Excel files and take your data analysis to the next level. Whether you are a beginner or an advanced user, macros can help you automate repetitive tasks and improve your workflow. So, start exploring the world of macros today and discover the endless possibilities of combining Excel files! We invite you to share your experiences, ask questions, or provide feedback on this article. Your input will help us improve and provide more valuable content in the future.