Intro
Creating a new sheet in Excel using VBA (Visual Basic for Applications) can be a straightforward process. This can be particularly useful for automating tasks, such as generating reports or creating templates. Below is a step-by-step guide on how to create a new sheet in Excel using VBA, along with examples and explanations to help you understand the process better.
Introduction to VBA in Excel
VBA is a powerful tool in Excel that allows you to automate tasks, create custom functions, and interact with the Excel interface. To access the VBA editor, you can press Alt + F11
or navigate to the Developer tab (if available) and click on Visual Basic.
Creating a New Sheet
To create a new sheet, you can use the Worksheets.Add
method. This method allows you to add a new worksheet to the active workbook.
Basic Example
The following code snippet demonstrates how to add a new sheet:
Sub CreateNewSheet()
Worksheets.Add
End Sub
This code will add a new sheet to your workbook. The new sheet will be added after the last existing sheet.
Specifying the Location of the New Sheet
You might want to specify where the new sheet should be added. For example, you can add it before the first sheet or after a specific sheet.
Sub CreateNewSheetBeforeFirst()
Worksheets.Add Before:=Worksheets(1)
End Sub
Sub CreateNewSheetAfterLast()
Worksheets.Add After:=Worksheets(Worksheets.Count)
End Sub
Naming the New Sheet
It's often useful to name the new sheet immediately after creating it. You can do this by referencing the ActiveSheet
property after adding the new sheet.
Sub CreateAndNameNewSheet()
Worksheets.Add
ActiveSheet.Name = "MyNewSheet"
End Sub
Copying an Existing Sheet
Sometimes, you might want to create a new sheet that is a copy of an existing one. This can be achieved by using the Worksheets.Add
method with the Type
argument set to xlWorksheet
and specifying the After
argument to position the new sheet.
Sub CopyExistingSheet()
Worksheets("Sheet1").Copy After:=Worksheets(Worksheets.Count)
End Sub
This will copy the sheet named "Sheet1" and place the copy after the last sheet in the workbook.
Practical Applications
Creating new sheets can be useful in a variety of scenarios, such as:
- Automating Reports: You can create a new sheet for each month or quarter to automatically generate reports.
- Templates: By creating a template sheet and then copying it, you can quickly set up new projects or tasks with a standardized format.
- Data Analysis: Adding new sheets for different data sets or analysis types can help keep your workbook organized and make it easier to compare data.
Tips and Best Practices
- Error Handling: Always consider adding error handling to your code to manage potential issues, such as attempting to name a sheet with a name that already exists.
- Workbook Protection: Be aware that if the workbook is protected, you may not be able to add new sheets without first unprotecting it.
- Performance: When working with large workbooks or performing operations that involve adding many sheets, consider the impact on workbook size and performance.
Gallery of Excel VBA Examples
Excel VBA Image Gallery
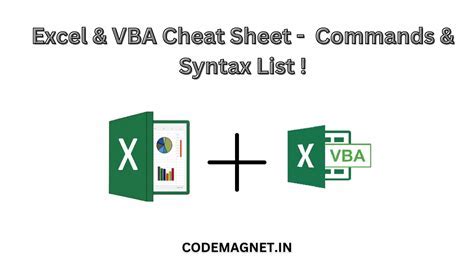
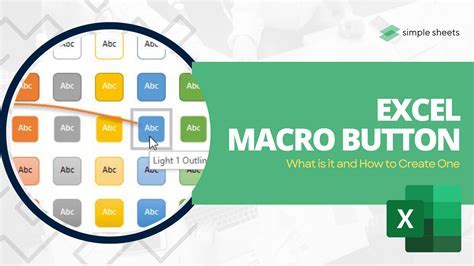
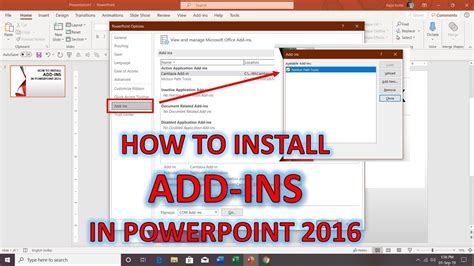
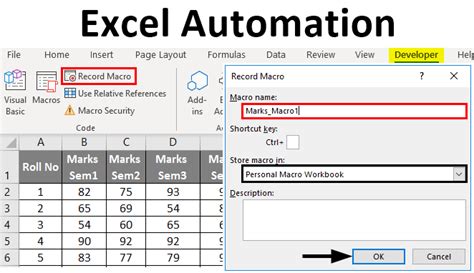
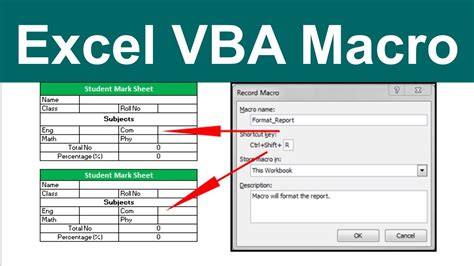
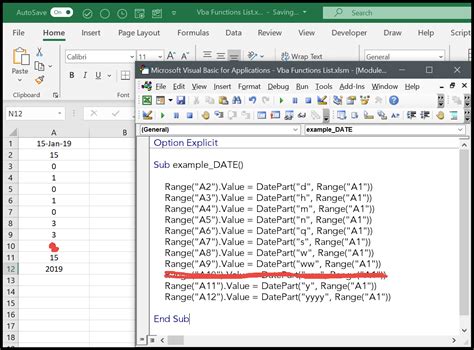
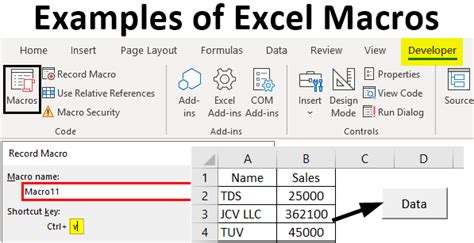

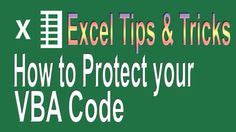
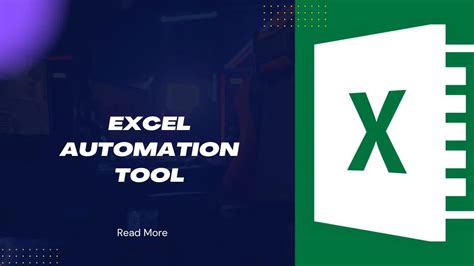
FAQs
How do I access the VBA editor in Excel?
+You can access the VBA editor by pressing Alt + F11 or by navigating to the Developer tab and clicking on Visual Basic.
Can I create a new sheet with a specific name using VBA?
+Yes, you can create a new sheet and name it immediately after creation using the ActiveSheet.Name property.
How do I copy an existing sheet to create a new one in VBA?
+You can copy an existing sheet by using the Worksheets("SheetName").Copy method and specifying the After argument to position the new sheet.
Final Thoughts
Creating new sheets in Excel using VBA is a versatile and powerful technique that can significantly enhance your productivity and workflow. Whether you're automating reports, creating templates, or organizing data, mastering the basics of VBA will open up new possibilities for managing and analyzing data in Excel. Remember to practice and explore more advanced features of VBA to fully leverage its capabilities. If you have any questions or need further assistance, don't hesitate to ask. Share your experiences or tips on using VBA for creating new sheets in the comments below.