Intro
Learn to rename Excel sheets using VBA, with step-by-step code examples and macros for dynamic worksheet naming, sheet management, and automation.
Renaming sheets in Excel VBA is a fundamental task that can greatly enhance the flexibility and usability of your spreadsheets. Whether you're automating tasks, creating interactive dashboards, or simply organizing your data, the ability to rename sheets dynamically can be incredibly powerful. In this article, we will delve into the world of Excel VBA, exploring how to rename sheets using various methods and scenarios.
The importance of organizing and managing worksheets in Excel cannot be overstated. As your workbook grows, either in complexity or size, being able to identify and access specific sheets quickly becomes crucial. This is where VBA comes into play, offering a programmable way to interact with Excel's interface and functionality. By mastering how to rename sheets in VBA, you can automate repetitive tasks, improve your workflow, and make your spreadsheets more intuitive for users.
Before we dive into the specifics of renaming sheets, it's worth noting that Excel VBA is a versatile tool that can be applied to a wide range of tasks, from simple automation to complex data analysis. Understanding the basics of VBA, including how to access the Visual Basic Editor, write macros, and interact with Excel objects, is essential for leveraging its full potential. For those new to VBA, taking the time to learn these fundamentals will pay dividends in the long run, especially when it comes to tasks like renaming sheets.
Introduction to Renaming Sheets in Excel VBA
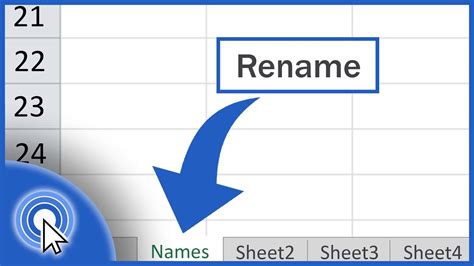
To rename a sheet in Excel VBA, you essentially need to access the sheet object and then modify its name property. This can be achieved through a straightforward line of code, as we will see in the examples below. However, the real power of VBA comes from its ability to perform actions dynamically based on conditions, loops, or user input, which can greatly simplify the process of managing multiple sheets.
Basic Syntax for Renaming Sheets
The basic syntax to rename a sheet is as follows: ```vba Worksheets("OldSheetName").Name = "NewSheetName" ``` This code snippet directly changes the name of a sheet from "OldSheetName" to "NewSheetName". It's a simple yet effective way to rename sheets, and it forms the basis for more complex renaming tasks.Methods for Renaming Sheets in Excel VBA
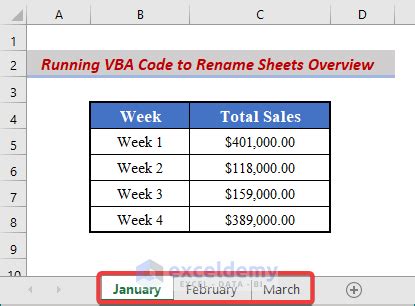
There are several methods to rename sheets in Excel VBA, each suited to different scenarios and requirements. Whether you're renaming a single sheet, multiple sheets, or using more dynamic methods based on cell values or user input, VBA provides the flexibility to adapt to your needs.
Renaming a Single Sheet
Renaming a single sheet is the most straightforward task. You can use the Worksheets
collection to access the sheet you want to rename and then change its Name
property.
Sub RenameSingleSheet()
Worksheets("Sheet1").Name = "MyNewSheet"
End Sub
Renaming Multiple Sheets
If you need to rename multiple sheets, you can do so by looping through the Worksheets
collection. This method is particularly useful when you have a pattern for renaming sheets, such as adding a prefix or suffix to the original names.
Sub RenameMultipleSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = "Prefix_" & ws.Name
Next ws
End Sub
Renaming Sheets Based on Cell Values
Sometimes, you might want to rename sheets based on values in specific cells. This can be useful for creating dynamic and interactive workbooks where the sheet names reflect the data they contain.
Sub RenameSheetsBasedOnCellValues()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Name = ws.Range("A1").Value
Next ws
End Sub
Best Practices for Renaming Sheets in Excel VBA
When renaming sheets in Excel VBA, it's essential to follow best practices to avoid errors and ensure your code runs smoothly. Here are some key considerations: - **Avoid Duplicate Names**: Excel does not allow sheets with the same name. Always check if a sheet with the intended name already exists before renaming. - **Use Valid Characters**: Sheet names cannot contain certain characters like "/", "\", "[", "]", ":", "*", "?", and ".". Ensure your renaming code accounts for these restrictions. - **Handle Errors**: Use error handling to manage situations where renaming might fail, such as when a sheet with the new name already exists.Advanced Techniques for Renaming Sheets
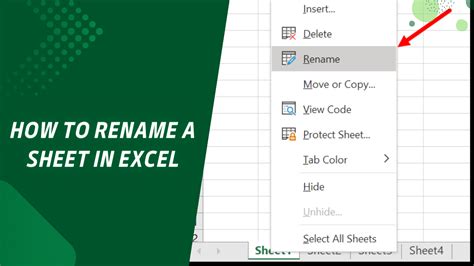
Beyond the basic methods, Excel VBA offers advanced techniques for renaming sheets, including the use of arrays, loops, and conditional statements. These techniques can significantly enhance the functionality of your renaming macros, allowing for more complex logic and dynamic interactions.
Using Arrays to Rename Sheets
You can store new sheet names in an array and then loop through the array to rename the sheets. This method is useful when you have a list of new names that you want to apply to your sheets in a specific order.
Sub RenameSheetsUsingArray()
Dim newNames() As Variant
newNames = Array("Sheet1New", "Sheet2New", "Sheet3New")
Dim i As Integer
For i = LBound(newNames) To UBound(newNames)
Worksheets(i + 1).Name = newNames(i)
Next i
End Sub
Renaming Sheets with Conditional Logic
Sometimes, you might want to rename sheets based on certain conditions, such as the value in a specific cell or the current date. VBA's conditional statements (If, Select Case) can be used to implement this logic.
Sub RenameSheetsWithConditions()
If Range("A1").Value = "Rename" Then
Worksheets("Sheet1").Name = "ConditionMet"
Else
Worksheets("Sheet1").Name = "ConditionNotMet"
End If
End Sub
Gallery of Renaming Sheets in Excel VBA
Renaming Sheets Image Gallery
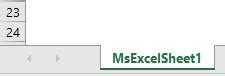

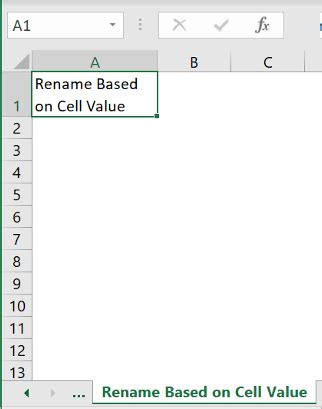
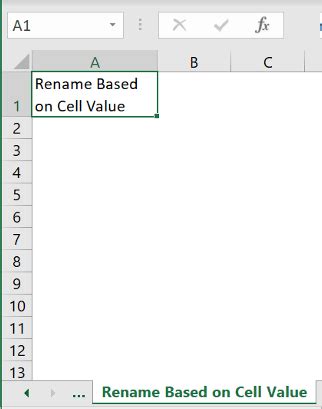
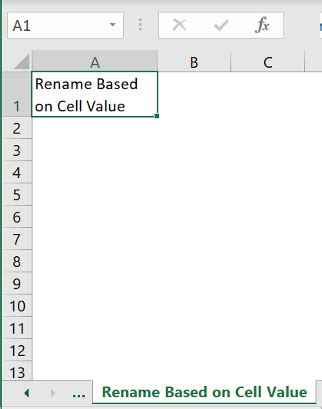
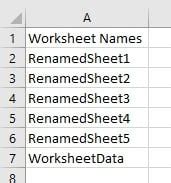
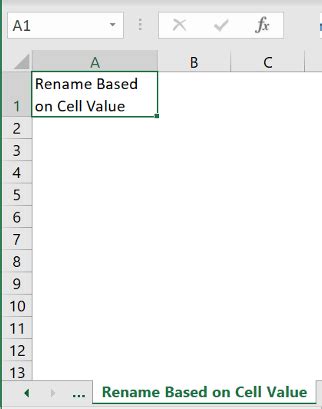
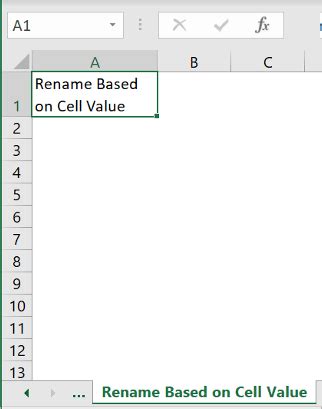
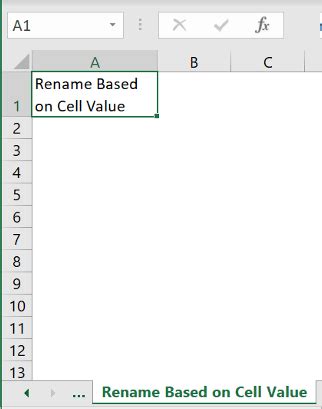
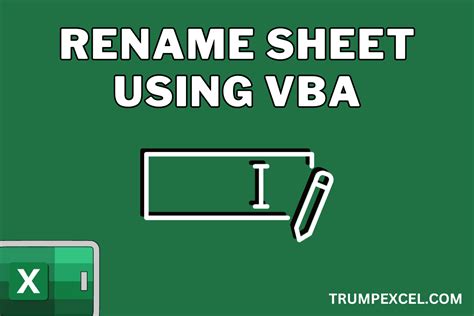
Frequently Asked Questions
How do I rename a sheet in Excel VBA?
+To rename a sheet in Excel VBA, use the syntax `Worksheets("OldSheetName").Name = "NewSheetName"`, replacing "OldSheetName" with the current name of your sheet and "NewSheetName" with the desired new name.
Can I rename multiple sheets at once using VBA?
+Yes, you can rename multiple sheets by looping through the `Worksheets` collection and changing the `Name` property of each sheet. This can be done using a For Each loop or a For loop, depending on your specific needs.
How do I avoid renaming a sheet to a name that already exists?
+To avoid renaming a sheet to a name that already exists, you can check if a sheet with the intended new name already exists before attempting to rename it. This can be done using a loop to check the names of all sheets in the workbook.
In conclusion, renaming sheets in Excel VBA is a powerful tool that can significantly enhance your ability to manage and automate tasks within your spreadsheets. By understanding the basics of VBA and how to apply them to renaming sheets, you can unlock new levels of productivity and efficiency. Whether you're a beginner looking to learn more about Excel VBA or an experienced user seeking to refine your skills, the ability to dynamically rename sheets will undoubtedly prove to be a valuable asset in your toolkit. So, take the next step and explore the world of Excel VBA further, discovering how you can leverage its capabilities to transform your workflow and take your spreadsheet management to the next level.