Intro
Fix Subscript Out Of Range Error 9 with expert solutions, troubleshooting steps, and code corrections to resolve runtime errors and array issues in programming.
The Subscript Out Of Range Error 9 is a common issue that occurs when a program attempts to access an array or a collection with an index that is outside the valid range. This error can be frustrating, but fortunately, there are several ways to fix it. In this article, we will delve into the causes of the Subscript Out Of Range Error 9 and provide step-by-step solutions to resolve it.
The Subscript Out Of Range Error 9 typically occurs in programming languages such as Visual Basic, VBA, and other scripting languages. It is essential to understand the root cause of the error to apply the correct fix. The error message usually indicates that the subscript is out of range, but it does not provide much information about the cause. Therefore, we need to investigate the code and the data to identify the issue.
Understanding the Subscript Out Of Range Error 9
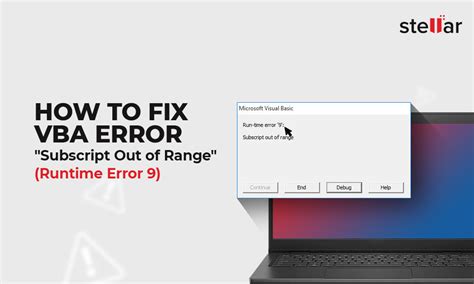
To fix the Subscript Out Of Range Error 9, we need to understand how arrays and collections work in programming. In most programming languages, arrays and collections are zero-based, meaning that the first element is at index 0. When we try to access an element with an index that is greater than or equal to the size of the array or collection, we get the Subscript Out Of Range Error 9.
Cause of the Subscript Out Of Range Error 9
The Subscript Out Of Range Error 9 can occur due to several reasons, including: * Attempting to access an array or collection with an index that is outside the valid range. * Using a variable or a value that is not a valid index. * Trying to access an element that does not exist in the array or collection. * Using a loop that iterates beyond the bounds of the array or collection.Fixing the Subscript Out Of Range Error 9
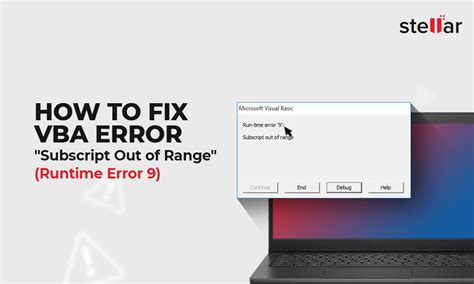
To fix the Subscript Out Of Range Error 9, we can follow these steps:
- Check the array or collection bounds: Verify that the index we are trying to access is within the valid range of the array or collection.
- Use a valid index: Ensure that the variable or value we are using as an index is valid and within the bounds of the array or collection.
- Initialize the array or collection: Make sure that the array or collection is initialized and has the correct size.
- Use a loop that iterates within the bounds: Use a loop that iterates within the bounds of the array or collection to avoid accessing elements that do not exist.
Example Code to Fix the Subscript Out Of Range Error 9
Here is an example code in VBA that demonstrates how to fix the Subscript Out Of Range Error 9: ```vb Dim myArray(10) As Integer Dim i As IntegerFor i = 0 To 9 myArray(i) = i * 2 Next i
' Attempting to access an element outside the bounds will raise an error ' myArray(10) = 20
' To fix the error, we can check the bounds of the array If i < UBound(myArray) Then myArray(i) = i * 2 End If
In this example, we define an array `myArray` with a size of 10 elements. We then use a loop to iterate within the bounds of the array and assign values to each element. Finally, we check the bounds of the array before attempting to access an element to avoid raising the Subscript Out Of Range Error 9.
Best Practices to Avoid the Subscript Out Of Range Error 9
To avoid the Subscript Out Of Range Error 9, we can follow these best practices:
* Always check the bounds of an array or collection before attempting to access an element.
* Use a valid index or variable when accessing an array or collection.
* Initialize arrays and collections with the correct size.
* Use loops that iterate within the bounds of an array or collection.
* Avoid using magic numbers or hardcoded values as indices.
Common Scenarios Where the Subscript Out Of Range Error 9 Occurs
The Subscript Out Of Range Error 9 can occur in various scenarios, including:
* When working with arrays or collections in VBA or other scripting languages.
* When using loops to iterate over an array or collection.
* When attempting to access an element that does not exist in an array or collection.
* When using a variable or value that is not a valid index.
Troubleshooting the Subscript Out Of Range Error 9
To troubleshoot the Subscript Out Of Range Error 9, we can follow these steps:
1. **Check the error message**: Read the error message carefully to understand the cause of the error.
2. **Review the code**: Examine the code to identify the line or statement that is causing the error.
3. **Use debugging tools**: Utilize debugging tools such as the debugger or print statements to inspect the values of variables and expressions.
4. **Test the code**: Test the code with different inputs or scenarios to reproduce the error.
Additional Tips for Fixing the Subscript Out Of Range Error 9
Here are some additional tips for fixing the Subscript Out Of Range Error 9:
* Use the `UBound` function to get the upper bound of an array.
* Use the `LBound` function to get the lower bound of an array.
* Use the `ReDim` statement to resize an array.
* Use the `Erase` statement to delete an array.
Subscript Out Of Range Error 9 Image Gallery
What is the Subscript Out Of Range Error 9?
+
The Subscript Out Of Range Error 9 is a common error that occurs when a program attempts to access an array or a collection with an index that is outside the valid range.
How do I fix the Subscript Out Of Range Error 9?
+
To fix the Subscript Out Of Range Error 9, check the bounds of the array or collection, use a valid index, initialize the array or collection, and use a loop that iterates within the bounds.
What are the common causes of the Subscript Out Of Range Error 9?
+
The common causes of the Subscript Out Of Range Error 9 include attempting to access an array or collection with an index that is outside the valid range, using a variable or value that is not a valid index, and trying to access an element that does not exist in the array or collection.
In summary, the Subscript Out Of Range Error 9 is a common error that can be fixed by checking the bounds of an array or collection, using a valid index, initializing the array or collection, and using a loop that iterates within the bounds. By following the best practices and tips outlined in this article, you can avoid the Subscript Out Of Range Error 9 and write more robust and efficient code. We hope this article has been helpful in resolving the Subscript Out Of Range Error 9. If you have any further questions or need additional assistance, please do not hesitate to comment or share this article with others.