Intro
Master Excel automation with VBA, learning to select sheets efficiently using code, including worksheet objects, sheet indexing, and activation methods.
Working with Excel sheets in VBA (Visual Basic for Applications) is a fundamental skill for automating tasks and creating interactive workbooks. Selecting a sheet in VBA is a straightforward process, but it's essential to understand the different methods and their applications.
When working with Excel VBA, you often need to switch between worksheets to perform various operations, such as copying data, formatting cells, or running calculations. Excel VBA provides several ways to select a worksheet, depending on your specific needs and the structure of your workbook.
To begin with, it's crucial to understand the basic syntax for referencing worksheets in VBA. You can reference a worksheet by its name or its index number in the workbook. For example, if you have a worksheet named "Sheet1," you can refer to it directly by its name. However, worksheets can also be referenced by their position in the workbook, where the leftmost sheet is index 1, the next one to the right is index 2, and so on.
Basic Syntax for Selecting a Sheet
The basic syntax to select a sheet in VBA is as follows:
Worksheets("SheetName").Select
Replace "SheetName"
with the actual name of the sheet you want to select. For instance:
Worksheets("Sheet1").Select
This line of code will select the worksheet named "Sheet1" in the active workbook.
Selecting a Sheet by Index
You can also select a sheet based on its position in the workbook:
Worksheets(1).Select
This will select the first sheet in the workbook.
Activating vs. Selecting
It's worth noting the difference between activating and selecting a worksheet. While Select
is used to select a range of cells, Activate
is used to make a worksheet the active sheet. Often, you'll see code that uses Activate
to switch between sheets:
Worksheets("Sheet1").Activate
This makes "Sheet1" the active sheet, allowing you to perform operations on it without necessarily selecting all cells.
Practical Example
Here's a simple example that demonstrates how to select a sheet, perform an action, and then move to another sheet:
Sub SelectAndPerformAction()
' Select Sheet1
Worksheets("Sheet1").Select
' Perform an action, for example, select cell A1
Range("A1").Select
' Now, let's activate another sheet and perform a different action
Worksheets("Sheet2").Activate
Range("B2").Value = "Hello, World!"
End Sub
This code snippet first selects "Sheet1" and then selects cell A1 on that sheet. Afterward, it activates "Sheet2" and writes "Hello, World!" into cell B2.
Best Practices
- Avoid Using Select: Whenever possible, try to avoid using
Select
orActivate
in your code. Instead, perform operations directly on the objects. For example, instead of selecting a range and then formatting it, you can format the range directly:Worksheets("Sheet1").Range("A1").Font.Bold = True
- Use Fully Qualified References: Always use fully qualified references to worksheets and ranges to avoid confusion and errors, especially in workbooks with multiple sheets:
ThisWorkbook.Worksheets("Sheet1").Range("A1").Value = "Example"
Embedding Images
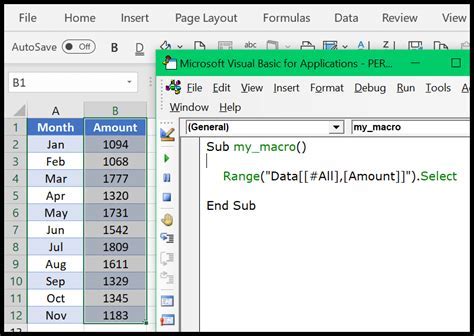
Gallery of VBA Examples
VBA Examples Image Gallery
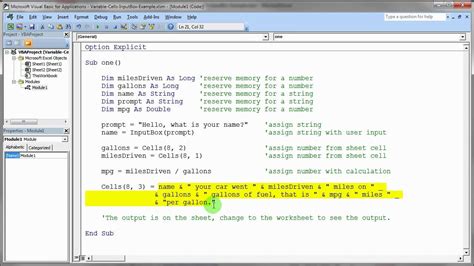
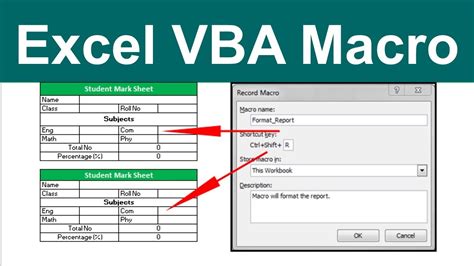
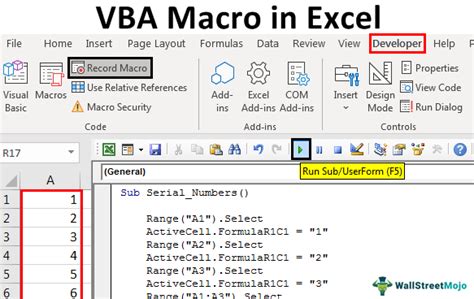
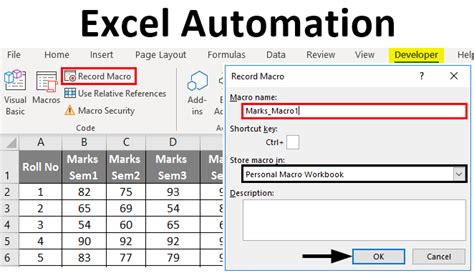
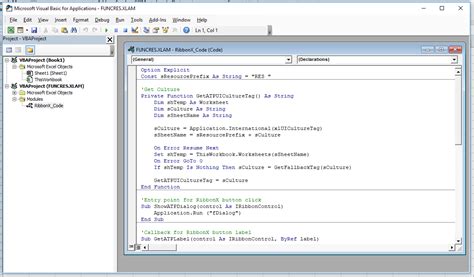
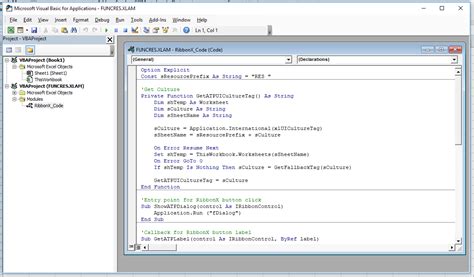
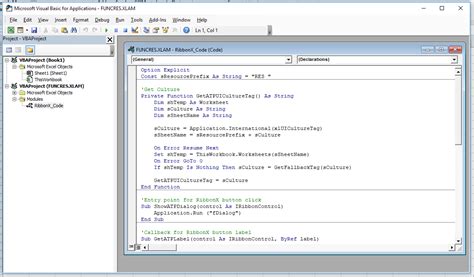
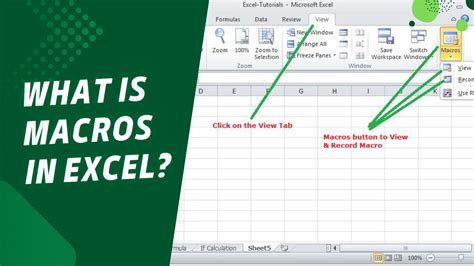
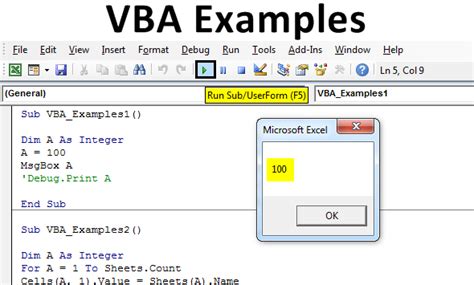
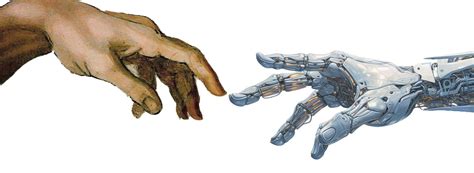
FAQs
What is VBA in Excel?
+VBA stands for Visual Basic for Applications. It's a programming language used for creating and automating tasks in Microsoft Excel and other Office applications.
How do I open the VBA Editor in Excel?
+You can open the VBA Editor by pressing Alt + F11 or by navigating to Developer > Visual Basic in the ribbon. If you don't see the Developer tab, you may need to activate it through Excel's settings.
What is the difference between selecting and activating a worksheet in VBA?
+Selecting a worksheet makes it the current worksheet for the operation, while activating a worksheet makes it the active sheet, allowing you to perform operations without selecting all cells. However, in practice, the terms are often used interchangeably.
Final Thoughts
Selecting sheets in VBA is a fundamental aspect of working with Excel programmatically. By mastering the basics of worksheet selection and manipulation, you can create powerful macros and automate complex tasks, significantly enhancing your productivity and workflow efficiency. Remember to follow best practices, such as avoiding the use of Select
whenever possible and using fully qualified references to ensure your code is robust and maintainable. With practice and experience, you'll become proficient in leveraging VBA to unlock the full potential of Excel and streamline your work processes.