Intro
Master Excel VBA sheet name management with 5 expert tips, including dynamic naming, worksheet loops, and error handling, to boost productivity and workflow efficiency in spreadsheet automation and macro development.
Working with Excel VBA can be a powerful way to automate and enhance your spreadsheet capabilities. One crucial aspect of VBA programming in Excel is understanding and manipulating sheet names. Whether you're creating new sheets, renaming existing ones, or referencing specific sheets in your code, knowing the tricks and tips can significantly improve your productivity and the efficiency of your VBA scripts. Here are five essential Excel VBA sheet name tips to help you navigate and manage your Excel sheets more effectively.
When it comes to Excel VBA, sheet names are not just identifiers; they are also integral to how you interact with your worksheets programmatically. A well-structured and consistently named set of sheets can make your VBA code more readable, maintainable, and less prone to errors. For instance, using descriptive names for your sheets, such as "JanuarySales" or "Budget2023", can make it easier to understand the purpose of each sheet at a glance, both for you and for anyone else who might need to work with your spreadsheet.
Understanding how to work with sheet names in VBA is also crucial for tasks like creating reports, generating summaries, or even automating the creation of new workbooks based on specific templates. For example, if you're managing a sales database and you want to generate a monthly sales report, being able to dynamically reference the correct sheet (e.g., "FebruarySales") in your VBA code can save you a lot of time and reduce the chance of human error.
Tip 1: Referencing Sheets by Name
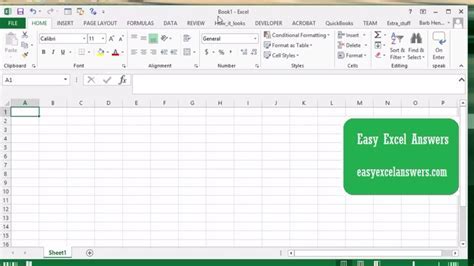
Referencing sheets by their names is one of the most straightforward ways to interact with them in VBA. You can use the Sheets
collection to access a sheet by its name. For example, Sheets("Sheet1").Range("A1").Value = "Hello World"
will write "Hello World" into cell A1 of the sheet named "Sheet1". This method is useful but be aware that it will throw an error if the sheet does not exist, so it's a good practice to check if the sheet exists before trying to access it.
Tip 2: Looping Through All Sheets
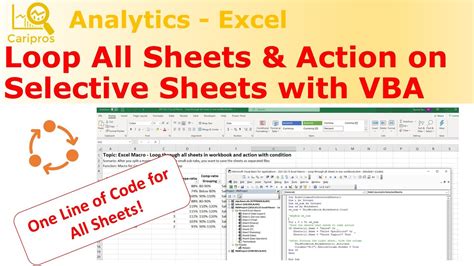
Sometimes, you might need to perform an action on every sheet in your workbook. VBA allows you to loop through all sheets using a For Each
loop. This can be particularly useful for tasks like formatting, data validation, or even just checking for specific conditions across all your sheets. Here's a simple example:
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Range("A1").Value = "Processed"
Next ws
This code will write "Processed" into cell A1 of every worksheet in your workbook.
Tip 3: Creating New Sheets

Creating new sheets programmatically can be very useful, especially when you're automating tasks or generating reports. You can add a new sheet using the Worksheets.Add
method. Here's how you can do it:
Dim newSheet As Worksheet
Set newSheet = ThisWorkbook.Worksheets.Add(After:=ThisWorkbook.Worksheets(ThisWorkbook.Worksheets.Count))
newSheet.Name = "MyNewSheet"
This code adds a new sheet at the end of your workbook and names it "MyNewSheet".
Tip 4: Renaming Sheets
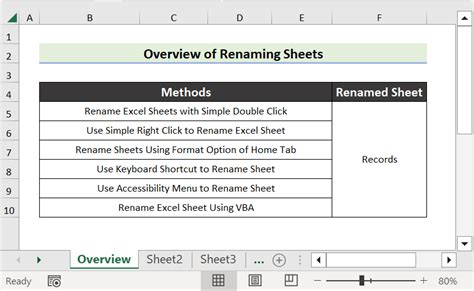
Renaming sheets can be necessary for organization or to reflect changes in your data. You can rename a sheet using the Name
property. For example:
ThisWorkbook.Worksheets("OldName").Name = "NewName"
This code renames the sheet "OldName" to "NewName". Remember, sheet names must be unique within a workbook, and they cannot exceed 31 characters.
Tip 5: Checking if a Sheet Exists
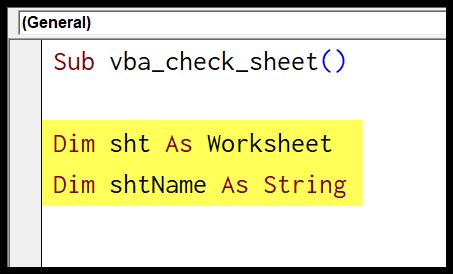
Before trying to access or manipulate a sheet, it's a good idea to check if it exists to avoid runtime errors. You can use a function like the following to check for the existence of a sheet:
Function SheetExists(sName As String) As Boolean
SheetExists = Evaluate("ISREF('" & sName & "'!A1)")
End Function
You can then use this function in your code like this:
If SheetExists("MySheet") Then
' The sheet exists, you can now work with it
Else
' The sheet does not exist, handle this situation
End If
This approach ensures that your code is more robust and less likely to fail due to missing sheets.
Gallery of Excel VBA Sheet Name Tips:
Excel VBA Sheet Name Tips Image Gallery
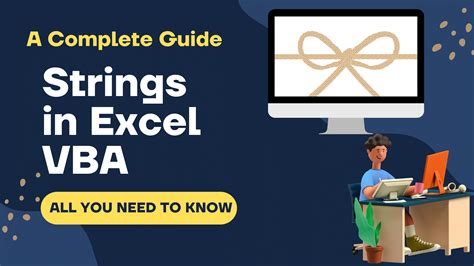
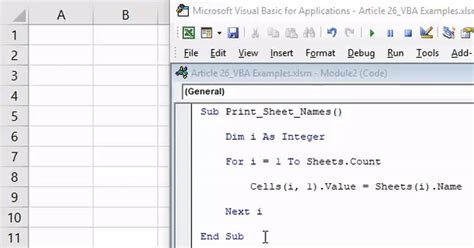
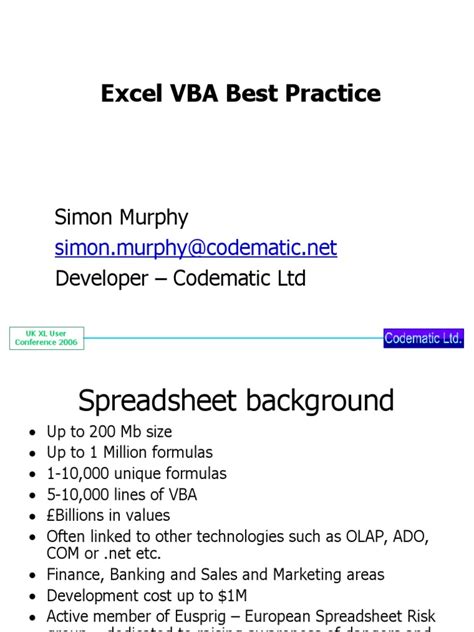
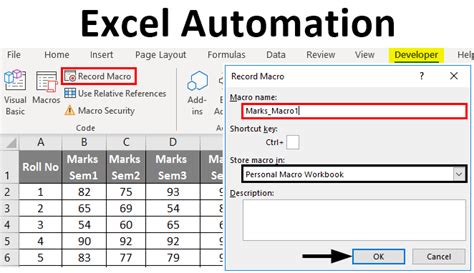
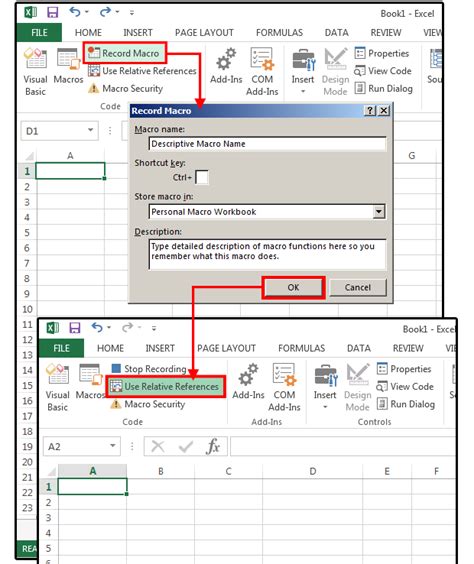
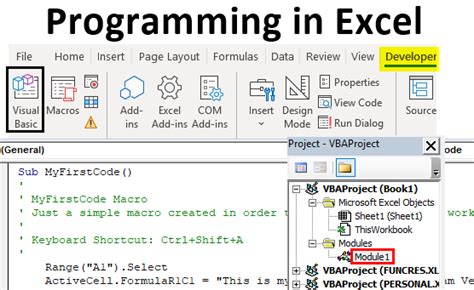
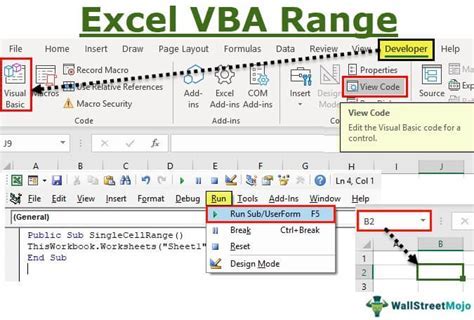
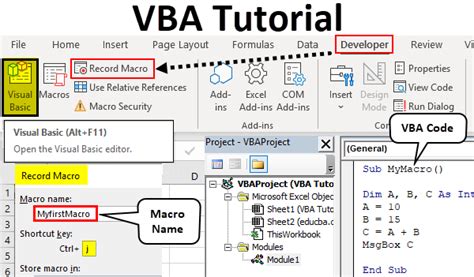
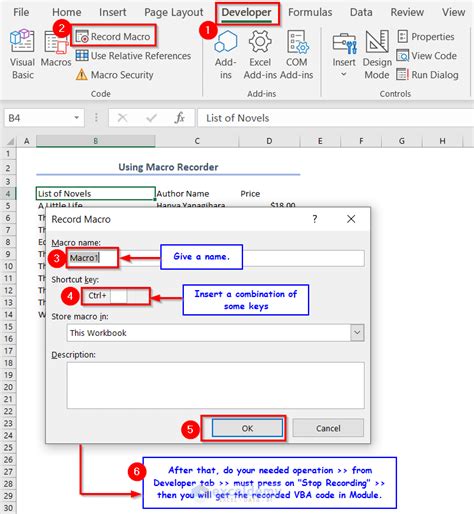
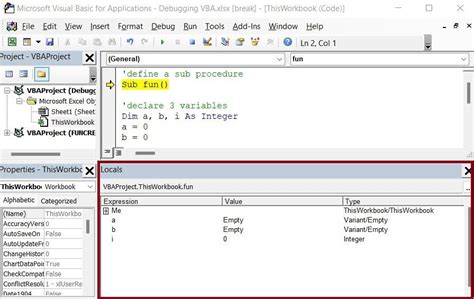
What is the maximum length for an Excel sheet name in VBA?
+The maximum length for an Excel sheet name is 31 characters.
How do I avoid errors when referencing sheets by name in VBA?
+To avoid errors, always check if the sheet exists before trying to access it. You can use a function that checks for the existence of a sheet by its name.
Can I use VBA to rename multiple sheets at once?
+Yes, you can rename multiple sheets using a loop in VBA. Simply iterate through the sheets you want to rename and change their `Name` property.
In conclusion, mastering the art of working with sheet names in Excel VBA can significantly enhance your productivity and the efficiency of your spreadsheet applications. By applying these tips and practices, you'll be better equipped to manage, automate, and analyze your data in Excel. Whether you're a beginner looking to learn the basics of VBA or an advanced user seeking to optimize your workflows, understanding how to effectively work with sheet names is a crucial skill. So, take the time to practice these methods, and soon you'll be creating powerful, dynamic Excel applications with ease. Don't hesitate to share your experiences, ask questions, or provide feedback on how you've applied these tips in your own projects. Your input is invaluable in helping others learn and grow in their Excel VBA journey.