Intro
Master Excel VBA by learning to split data with ease, using macros, arrays, and loops to divide datasets, automate tasks, and boost productivity.
The ability to split data in Excel VBA is a powerful tool for managing and analyzing large datasets. Excel VBA provides various methods to achieve this, including using loops, the Split
function, and the Text-to-Columns
feature. In this article, we will explore the different ways to split data in Excel VBA, along with examples and code snippets to help you get started.
When working with data in Excel, it's common to encounter situations where you need to split a single column of data into multiple columns. This could be due to various reasons, such as splitting a full name into first and last names, separating a combined date and time field, or dividing a list of keywords into individual columns. Whatever the reason, Excel VBA provides the necessary tools to accomplish this task efficiently.
To begin with, let's consider a simple example where we have a column of data containing full names, and we want to split each name into first and last names. We can achieve this using the Split
function in VBA, which splits a string into an array of substrings based on a specified delimiter.
Using the Split Function
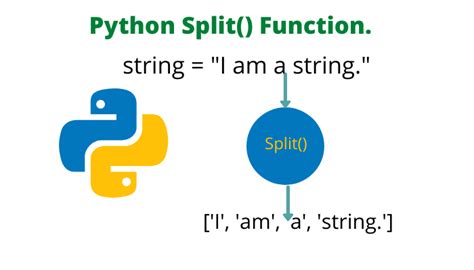
The Split
function is a versatile tool that can be used to split strings based on various delimiters, such as spaces, commas, or other characters. Here's an example code snippet that demonstrates how to use the Split
function to split full names into first and last names:
Sub SplitNames()
Dim cell As Range
Dim nameArray() As String
Dim firstName As String
Dim lastName As String
For Each cell In Range("A1:A10")
nameArray = Split(cell.Value, " ")
firstName = nameArray(0)
lastName = nameArray(1)
cell.Offset(0, 1).Value = firstName
cell.Offset(0, 2).Value = lastName
Next cell
End Sub
In this example, we loop through each cell in the range A1:A10, split the value of each cell using the Split
function, and then assign the first and last names to separate variables. Finally, we write the first and last names to adjacent columns.
Using the Text-to-Columns Feature
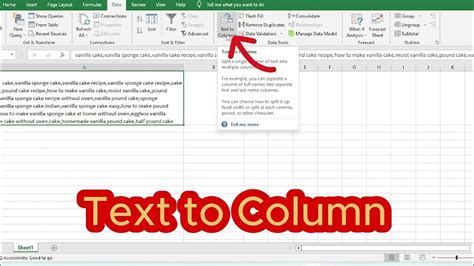
Another way to split data in Excel VBA is by using the Text-to-Columns
feature. This feature allows you to split a single column of data into multiple columns based on a specified delimiter. Here's an example code snippet that demonstrates how to use the Text-to-Columns
feature to split full names into first and last names:
Sub SplitNamesUsingTextToColumns()
Range("A1:A10").TextToColumns Destination:=Range("A1"), DataType:=xlDelimited, Space:=True
End Sub
In this example, we use the TextToColumns
method to split the data in the range A1:A10 into multiple columns based on spaces. The DataType
parameter is set to xlDelimited
, and the Space
parameter is set to True
to specify that we want to split on spaces.
Splitting Data Using Loops
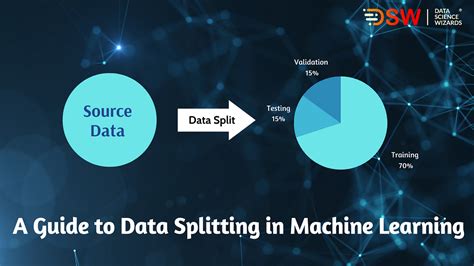
In addition to using the Split
function and the Text-to-Columns
feature, you can also use loops to split data in Excel VBA. This approach involves looping through each character in a string and checking for a specified delimiter. Here's an example code snippet that demonstrates how to use loops to split full names into first and last names:
Sub SplitNamesUsingLoops()
Dim cell As Range
Dim name As String
Dim firstName As String
Dim lastName As String
Dim spaceIndex As Integer
For Each cell In Range("A1:A10")
name = cell.Value
spaceIndex = InStr(name, " ")
If spaceIndex > 0 Then
firstName = Left(name, spaceIndex - 1)
lastName = Right(name, Len(name) - spaceIndex)
Else
firstName = name
lastName = ""
End If
cell.Offset(0, 1).Value = firstName
cell.Offset(0, 2).Value = lastName
Next cell
End Sub
In this example, we loop through each cell in the range A1:A10, find the index of the first space in the string, and then use the Left
and Right
functions to extract the first and last names.
Best Practices for Splitting Data in Excel VBA
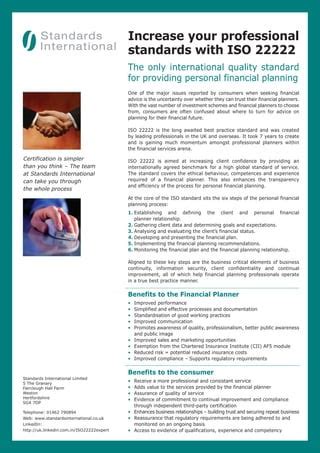
When splitting data in Excel VBA, there are several best practices to keep in mind. Here are a few tips to help you get the most out of your code:
- Always test your code on a small sample of data before running it on a large dataset.
- Use the
Split
function or theText-to-Columns
feature whenever possible, as these methods are generally faster and more efficient than using loops. - Make sure to handle errors and exceptions properly, such as when a delimiter is not found in a string.
- Use meaningful variable names and comments to make your code easy to understand and maintain.
Common Errors When Splitting Data in Excel VBA
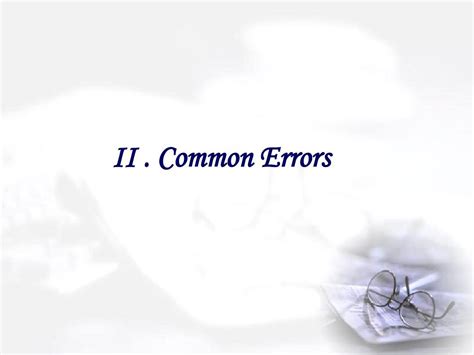
When splitting data in Excel VBA, there are several common errors to watch out for. Here are a few examples:
- Not handling errors and exceptions properly, such as when a delimiter is not found in a string.
- Using the wrong delimiter or not specifying a delimiter at all.
- Not testing your code on a small sample of data before running it on a large dataset.
- Using loops when the
Split
function or theText-to-Columns
feature would be more efficient.
Split Data in Excel VBA Image Gallery
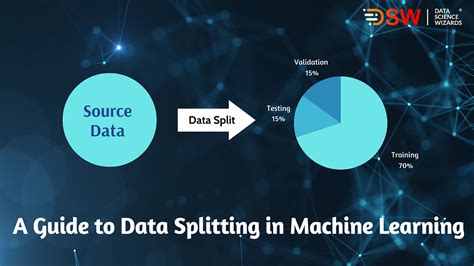
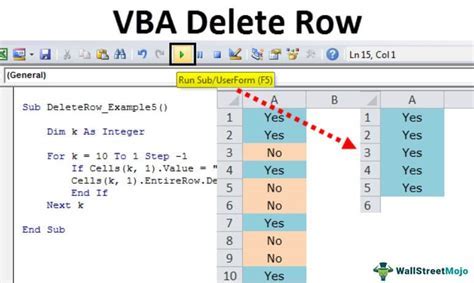
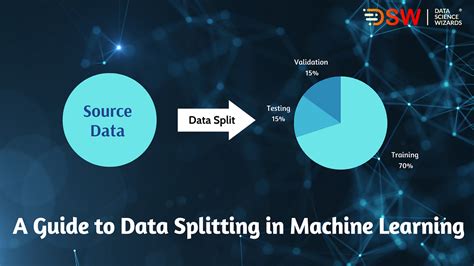
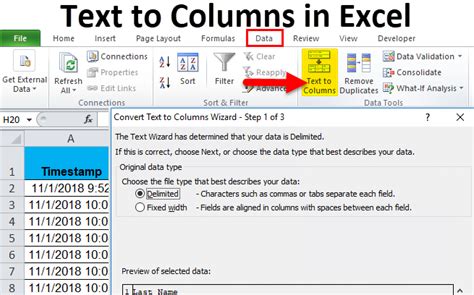
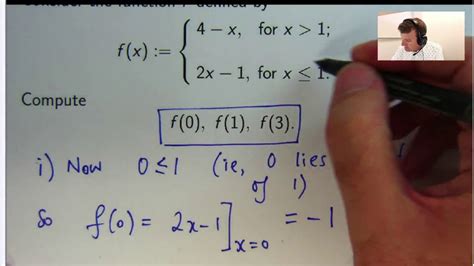
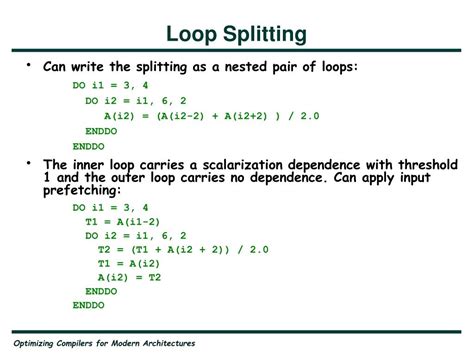
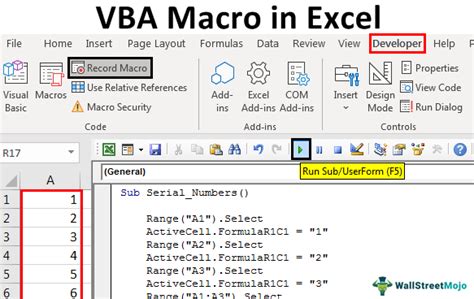
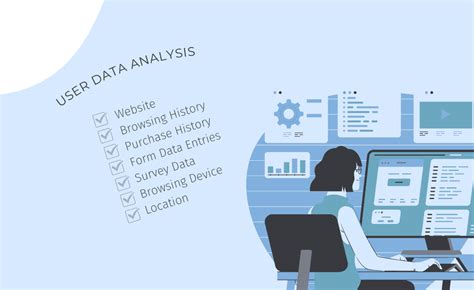
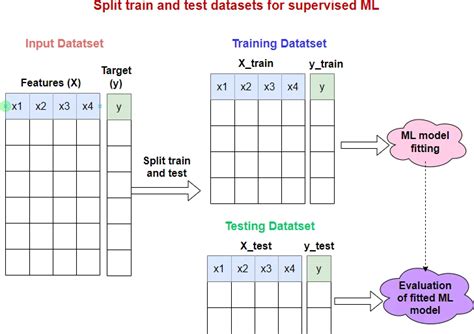

What is the best way to split data in Excel VBA?
+The best way to split data in Excel VBA depends on the specific requirements of your project. However, the `Split` function and the `Text-to-Columns` feature are generally the most efficient and effective methods.
How do I handle errors and exceptions when splitting data in Excel VBA?
+To handle errors and exceptions when splitting data in Excel VBA, you can use error-handling statements such as `On Error Resume Next` or `On Error GoTo 0`. You can also use conditional statements to check for specific errors and exceptions.
Can I use loops to split data in Excel VBA?
+Yes, you can use loops to split data in Excel VBA. However, loops can be slower and less efficient than the `Split` function or the `Text-to-Columns` feature, especially for large datasets.
In conclusion, splitting data in Excel VBA is a powerful tool for managing and analyzing large datasets. By using the Split
function, the Text-to-Columns
feature, or loops, you can efficiently split data into multiple columns and perform various data analysis tasks. Remember to always test your code on a small sample of data before running it on a large dataset, and to handle errors and exceptions properly to ensure that your code runs smoothly and efficiently. If you have any further questions or need help with a specific project, feel free to comment below or share this article with others who may find it useful.