Intro
Convert strings to integers in VBA with ease. Learn string to int VBA functions, integer parsing, and error handling techniques for seamless data type conversions and robust coding.
Converting strings to integers is a fundamental task in programming, including in Visual Basic for Applications (VBA). This process is essential when you need to perform numerical operations on data that is initially stored or input as text. VBA provides several methods to achieve this conversion, each with its own set of considerations and potential pitfalls.
The importance of converting strings to integers or other numerical data types lies in the ability to perform arithmetic operations, comparisons, and other actions that are not possible or meaningful with string data. For instance, if you have a string that represents a quantity or a value, converting it to an integer allows you to use it in calculations, sort it numerically, or compare it to other numerical values.
To understand the process and implications of converting strings to integers in VBA, it's crucial to delve into the methods available, their syntax, and examples of how they are used. Additionally, considering the potential errors that can occur during this conversion process is vital for writing robust and reliable VBA code.
Introduction to Converting Strings to Integers
VBA offers several functions to convert strings to integers, including CInt()
, CLng()
, Int()
, and Val()
. Each of these functions has its own specific use cases and limitations. Understanding these functions is key to effectively converting string data to numerical data in VBA.
Methods for Converting Strings to Integers
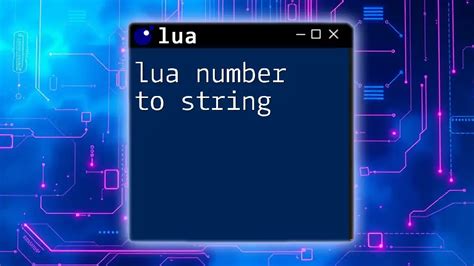
Using CInt()
The CInt()
function is one of the most commonly used methods for converting a string to an integer in VBA. It attempts to convert a string or any other data type into an integer. However, it will round the number to the nearest integer if the string represents a decimal number.
Dim myString As String
myString = "10"
Dim myInteger As Integer
myInteger = CInt(myString)
Using CLng()
The CLng()
function is similar to CInt()
but converts the string to a long integer. This is useful when you're dealing with larger numbers that exceed the range of the Integer
data type.
Dim myString As String
myString = "1000000"
Dim myLong As Long
myLong = CLng(myString)
Using Int()
The Int()
function also converts a value to an integer but does so by truncating the decimal part, rather than rounding. This can be useful in specific scenarios but is generally less commonly used for string conversions.
Dim myString As String
myString = "10.9"
Dim myInteger As Integer
myInteger = Int(CDbl(myString)) ' First convert to double to avoid type mismatch
Using Val()
The Val()
function is another method to convert a string to a number. However, it returns a Double
by default, so you may need to use it in conjunction with CInt()
or CLng()
to get an integer.
Dim myString As String
myString = "10.5"
Dim myInteger As Integer
myInteger = CInt(Val(myString))
Handling Errors During Conversion
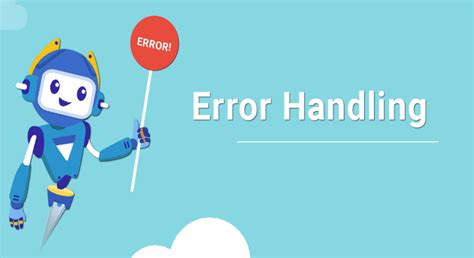
When converting strings to integers, one of the most common issues is encountering strings that cannot be converted to numbers, such as strings containing letters or special characters. VBA will throw a runtime error in such cases. To manage these situations, you can use error handling techniques like On Error
statements or validate the string before attempting the conversion.
On Error Resume Next
Dim myString As String
myString = "Not a number"
Dim myInteger As Integer
myInteger = CInt(myString)
If Err.Number <> 0 Then
MsgBox "Error converting string to integer: " & Err.Description
Err.Clear
End If
On Error GoTo 0
Best Practices for Conversion
- Validate Input: Before converting, ensure the string is in the correct format and can be converted to an integer.
- Use Appropriate Data Types: Choose between
Integer
andLong
based on the range of values you expect to handle. - Error Handling: Implement robust error handling to manage cases where the conversion fails.
Applications and Examples
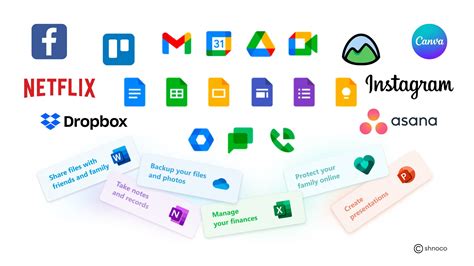
Converting strings to integers is crucial in various applications, such as data analysis, where imported data might be in string format, or in user interfaces, where user input needs to be processed numerically.
Data Analysis Example
When analyzing data imported from a text file or database, you might need to convert string representations of numbers to integers for calculations.
Dim dataArray() As Variant
' Assume dataArray is populated with string values
For i = LBound(dataArray) To UBound(dataArray)
dataArray(i) = CInt(dataArray(i))
Next i
User Input Example
In a user form, if a user enters a quantity, you might need to convert this input to an integer for further processing.
Dim userInput As String
userInput = TextBox1.Value
Dim quantity As Integer
quantity = CInt(userInput)
Conclusion and Future Directions
In conclusion, converting strings to integers in VBA is a fundamental skill that can be achieved through various methods, each suited to different needs and scenarios. By understanding these methods, developers can write more effective and robust code, handling potential errors and edge cases with grace. As VBA continues to evolve and be used in new contexts, mastering these conversion techniques will remain essential for anyone working with data in Excel, Access, or other Microsoft Office applications.
Gallery of String to Integer Conversion
String to Integer Conversion Image Gallery
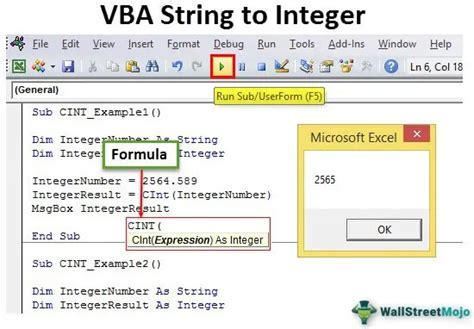
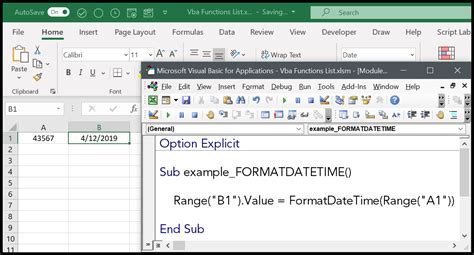
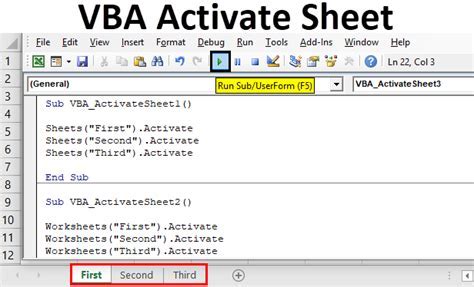
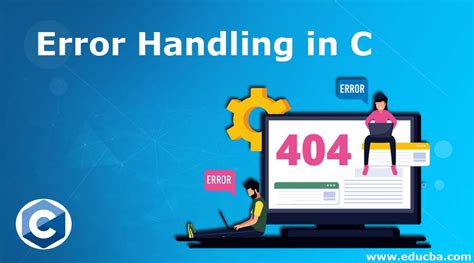
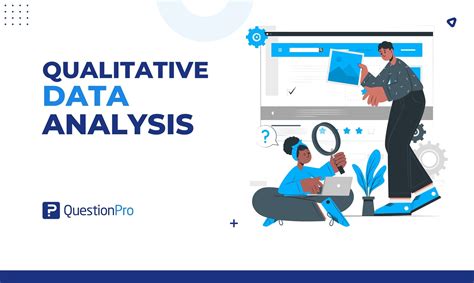

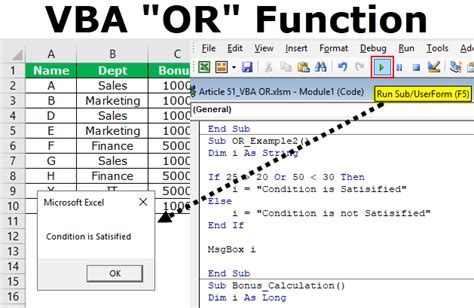
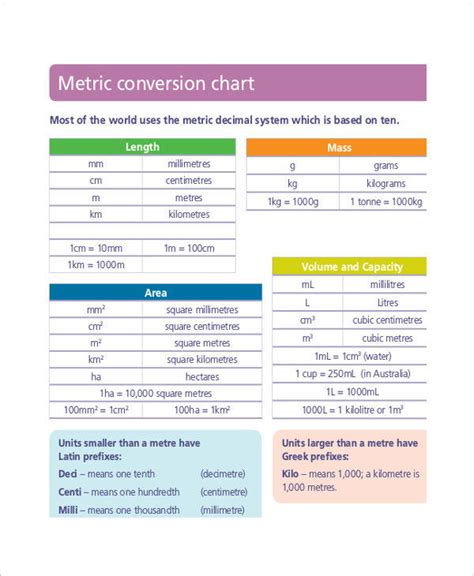
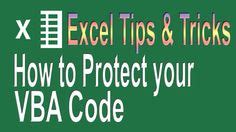
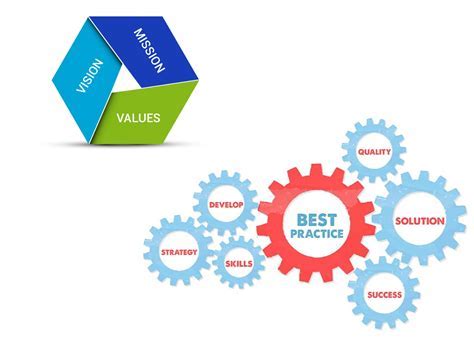
What is the purpose of converting strings to integers in VBA?
+
The purpose is to enable numerical operations and comparisons on data initially stored as text.
How do you handle errors during string to integer conversion in VBA?
+
Use error handling techniques like On Error statements or validate the string before conversion.
What is the difference between CInt() and CLng() in VBA?
+
CInt() converts to an integer, while CLng() converts to a long integer, which can handle larger numbers.
What is the purpose of converting strings to integers in VBA?
+The purpose is to enable numerical operations and comparisons on data initially stored as text.
How do you handle errors during string to integer conversion in VBA?
+Use error handling techniques like On Error statements or validate the string before conversion.
What is the difference between CInt() and CLng() in VBA?
+CInt() converts to an integer, while CLng() converts to a long integer, which can handle larger numbers.
We invite you to share your thoughts, experiences, or questions regarding string to integer conversion in VBA. Your input can help others understand this topic better and provide valuable insights into real-world applications and challenges. Feel free to comment below or share this article with others who might find it useful.