Intro
Pivot tables are a powerful tool in Excel for analyzing and summarizing large datasets. They allow users to rotate, aggregate, and filter data to gain insights and spot trends. However, when working with dynamic data or when the data source changes, pivot tables may need to be updated manually or through automation. VBA (Visual Basic for Applications) provides a way to automate tasks in Excel, including updating pivot tables. This article will delve into the importance of updating pivot tables, how to do it manually, and most importantly, how to automate the process using VBA.
The ability to update pivot tables efficiently is crucial for data analysis and reporting. Manually updating pivot tables can be time-consuming, especially when dealing with large datasets or multiple pivot tables. Moreover, manual updates are prone to human error, which can lead to inaccuracies in analysis and decision-making. Automating the update process with VBA not only saves time but also reduces the likelihood of errors, making data analysis more reliable and efficient.
Understanding Pivot Tables
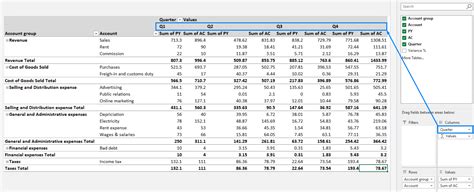
Before diving into how to update pivot tables with VBA, it's essential to understand the basics of pivot tables. Pivot tables are a feature in Excel that allows users to summarize, analyze, and visualize data. They enable the rotation of data fields to display different summaries of the source data. Pivot tables consist of fields, which are the columns from the source data, and areas, which include the row labels, column labels, values, and filters.
Manually Updating Pivot Tables
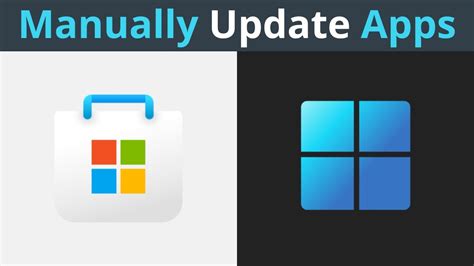
Manually updating a pivot table involves refreshing the data to reflect changes in the source data. This can be done by right-clicking on the pivot table and selecting "Refresh" or by using the "Refresh All" button in the "Data" tab of the ribbon. However, this method is not practical for automated reporting or when dealing with multiple pivot tables.
Steps to Manually Update a Pivot Table
- Select the pivot table. - Right-click on the pivot table. - Choose "Refresh" from the context menu. - Alternatively, go to the "Data" tab in the ribbon and click "Refresh All" to update all pivot tables in the workbook.Updating Pivot Tables with VBA
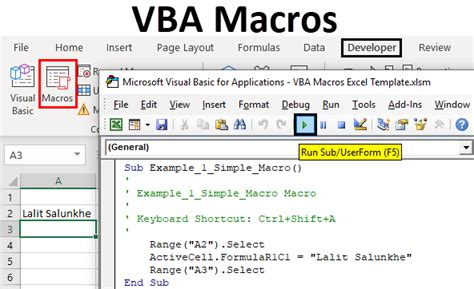
VBA provides a powerful way to automate tasks in Excel, including the update of pivot tables. By writing a VBA script, you can update pivot tables automatically whenever the source data changes or at scheduled intervals. This is particularly useful for reports that need to be updated regularly.
Basic VBA Code to Update a Pivot Table
```vba Sub UpdatePivotTable() Dim pt As PivotTable' Specify the pivot table to update
Set pt = ThisWorkbook.Worksheets("Sheet1").PivotTables("PivotTableName")
' Refresh the pivot table
pt.RefreshTable
MsgBox "Pivot table updated successfully."
End Sub
Advanced VBA Techniques for Pivot Tables
Beyond simple updates, VBA can be used to perform more complex operations on pivot tables, such as changing the data source, adding or removing fields, and applying filters. These advanced techniques allow for dynamic and automated reporting solutions.
Changing the Data Source of a Pivot Table with VBA
```vba
Sub ChangePivotTableDataSource()
Dim pt As PivotTable
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
Set pt = ws.PivotTables("PivotTableName")
' Specify the new data source range
Dim sourceRange As Range
Set sourceRange = ThisWorkbook.Worksheets("DataSheet").Range("A1:E100")
' Change the data source
pt.ChangePivotCache ThisWorkbook.PivotCaches.Create(SourceType:=xlDatabase, SourceData:=sourceRange)
MsgBox "Data source changed successfully."
End Sub
Best Practices for Using VBA with Pivot Tables
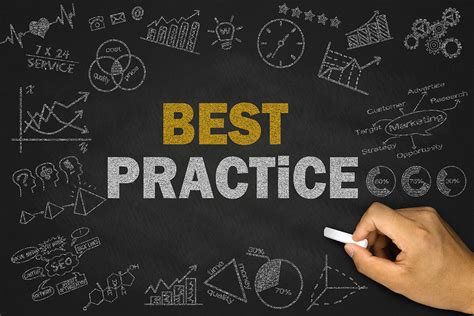
When using VBA to update or manipulate pivot tables, it's essential to follow best practices to ensure reliability, efficiency, and ease of maintenance. This includes commenting code, handling errors, and optimizing performance.
Commenting VBA Code
- Use comments to explain what each section of the code does. - This improves readability and makes the code easier to understand and maintain.Error Handling
- Implement error handling to manage and report errors gracefully. - This prevents the code from crashing unexpectedly and provides useful feedback instead.Optimizing Performance
- Optimize VBA code for performance by minimizing unnecessary operations and using efficient algorithms. - This is particularly important when working with large datasets or complex pivot tables.Pivot Table Image Gallery
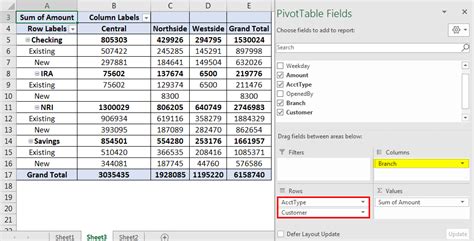
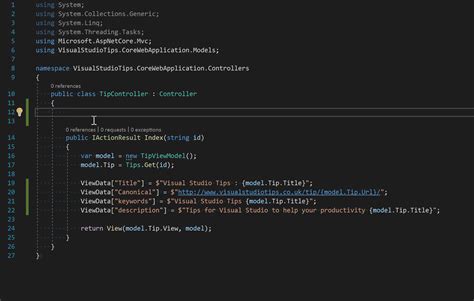

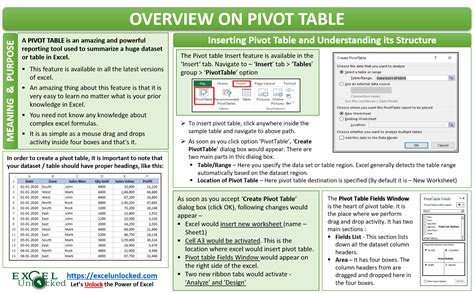
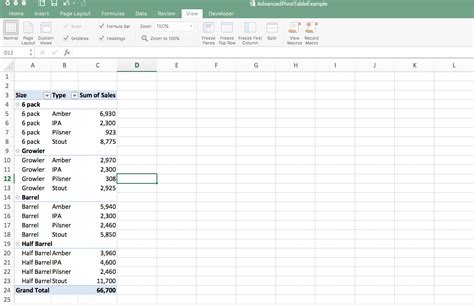
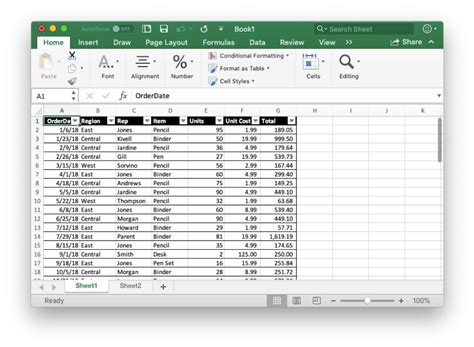

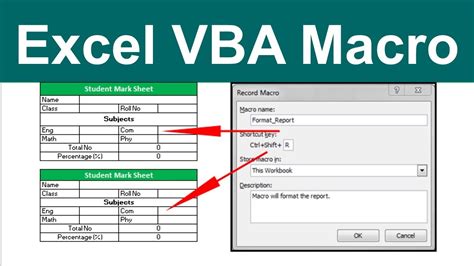
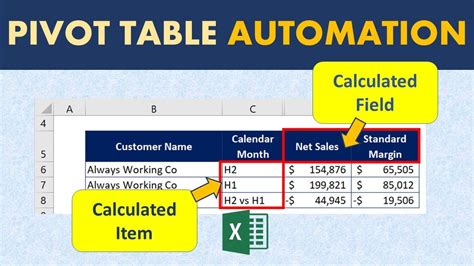
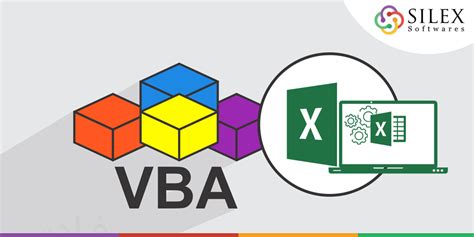
What is the purpose of using VBA with pivot tables?
+The purpose of using VBA with pivot tables is to automate tasks, such as updating, formatting, and analyzing pivot tables, which can save time and reduce errors.
How do I update a pivot table using VBA?
+To update a pivot table using VBA, you can use the `RefreshTable` method of the `PivotTable` object. This method refreshes the pivot table and updates it with the latest data from the source range.
Can I change the data source of a pivot table using VBA?
+Yes, you can change the data source of a pivot table using VBA. This can be done by using the `ChangePivotCache` method of the `PivotTable` object and specifying a new data source range.
In conclusion, updating pivot tables with VBA is a powerful way to automate tasks and improve efficiency in Excel. By understanding how to update pivot tables manually and how to automate this process with VBA, users can unlock the full potential of pivot tables for data analysis and reporting. Whether you're a beginner or an advanced user, mastering VBA techniques for pivot tables can significantly enhance your productivity and the accuracy of your data analysis. Feel free to share your experiences or ask questions about using VBA with pivot tables in the comments below.