Intro
When working with Visual Basic for Applications (VBA) in Microsoft Office, an "Ambiguous Name Detected" error can be frustrating and disrupt your workflow. This error typically occurs when VBA encounters a situation where it cannot uniquely identify a variable, procedure, or object due to duplicate names within the scope of your project. Here's how to understand and fix this issue.
The "Ambiguous Name Detected" error can arise from several sources, including but not limited to, naming conflicts between modules, user forms, classes, or even built-in Excel objects and your custom variables or procedures. For instance, if you have a module named Module1
and within that module, you declare a variable or subroutine also named Module1
, VBA will not know whether you're referring to the module itself or the variable/subroutine when you use the name Module1
.
To resolve the "Ambiguous Name Detected" error, follow these steps:
1. Identify the Source of Ambiguity
First, you need to identify where the ambiguity is coming from. This involves reviewing your code, module names, and any custom classes or forms you've created. Look for any duplicates in names.
2. Rename the Conflicting Element
Once you've identified the source of the conflict, rename one of the conflicting elements. For example, if you have a module named DataProcessor
and a subroutine also named DataProcessor
, consider renaming the subroutine to something like ProcessData
. The key is to ensure that each element in your VBA project has a unique name.
3. Use Fully Qualified References
In some cases, using fully qualified references can help VBA understand exactly what you're referring to. For instance, if you have a worksheet named Sheet1
and you want to refer to a range on that sheet, using Worksheets("Sheet1").Range("A1")
can help avoid confusion, especially if you have other elements named similarly.
4. Review External References
Sometimes, the ambiguity can arise from external references or libraries you've added to your project. Ensure that any external references are properly declared and that there are no conflicts with names used within those libraries and your own code.
5. Check for Typos
A simple typo can sometimes lead to unexpected errors, including the "Ambiguous Name Detected" error. Make sure to review your code for any typos or incorrect references.
6. Organize Your Code
Keeping your code organized can help prevent naming conflicts. Consider grouping related procedures and variables into modules or classes with descriptive names, making it easier to manage and understand your project's scope.
Example of Ambiguity and Resolution
Consider a scenario where you have a module named Utilities
and within that module, you've declared a subroutine also named Utilities
:
' Module named Utilities
Sub Utilities()
' Code here
End Sub
To fix the ambiguity, you could rename the subroutine to something more descriptive:
' Module named Utilities
Sub RunUtility()
' Code here
End Sub
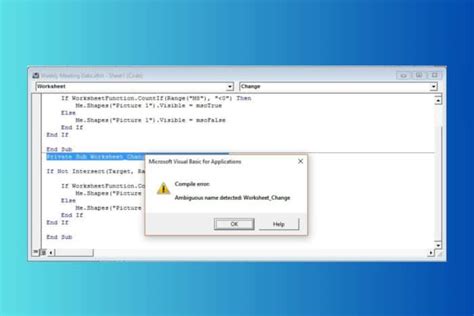
Steps to Prevent Future Ambiguities
- Plan Your Code Structure: Before you start coding, take a moment to plan out your project's structure, including module names, variable names, and subroutine names.
- Use Meaningful Names: Choose names that are descriptive and indicate the purpose or function of the variable, subroutine, or module.
- Avoid Generic Names: Names like
Module1
,Variable1
, etc., are too generic and can easily lead to conflicts. - Keep a Naming Convention: Establish a naming convention and stick to it throughout your project.
Common Scenarios for Ambiguity
- Duplicate Module Names: Having two or more modules with the same name.
- Variable and Subroutine Name Conflict: Using the same name for a variable and a subroutine.
- Class and Module Name Conflict: Naming a class the same as an existing module.
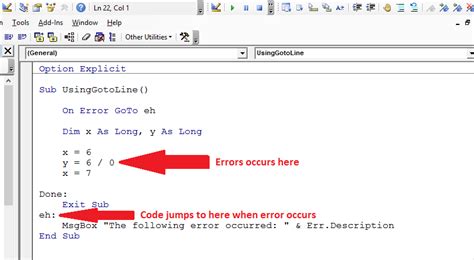
Best Practices for VBA Development
- Comment Your Code: Comments can help you and others understand what your code is supposed to do.
- Test Thoroughly: Always test your code in a controlled environment before deploying it.
- Use Version Control: If possible, use version control to track changes to your code.
Gallery of VBA Error Fixes
VBA Error Fixes Gallery
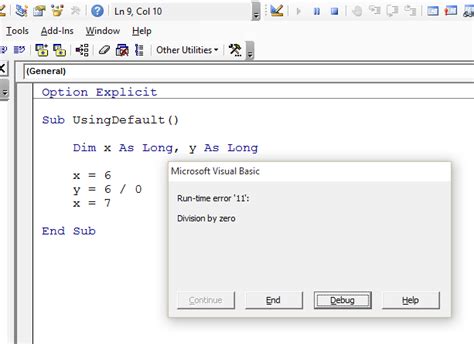
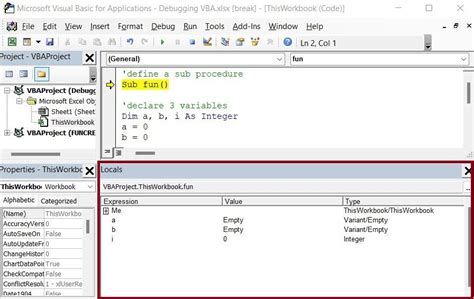
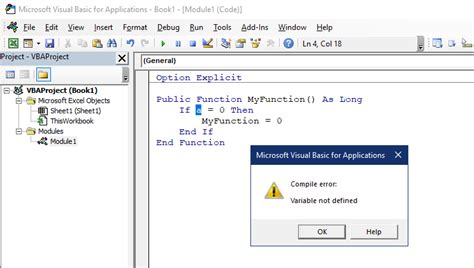
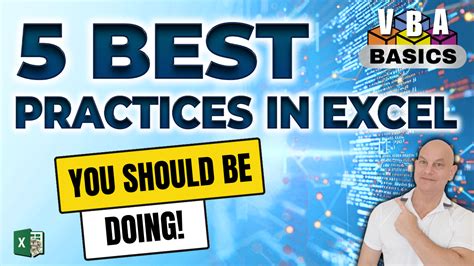
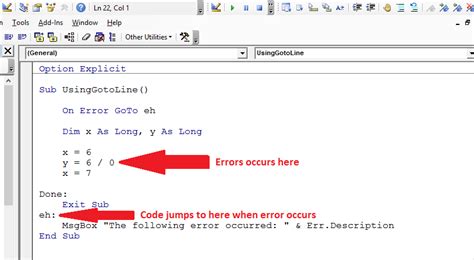
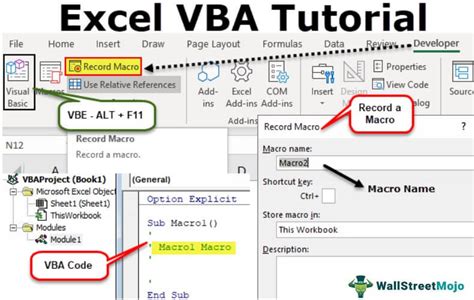
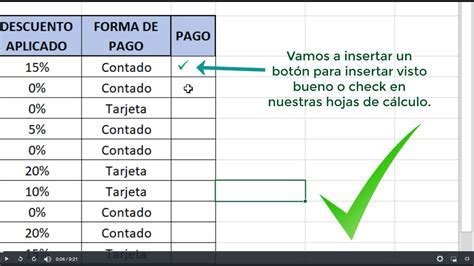
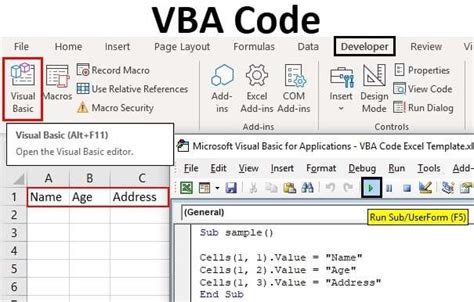
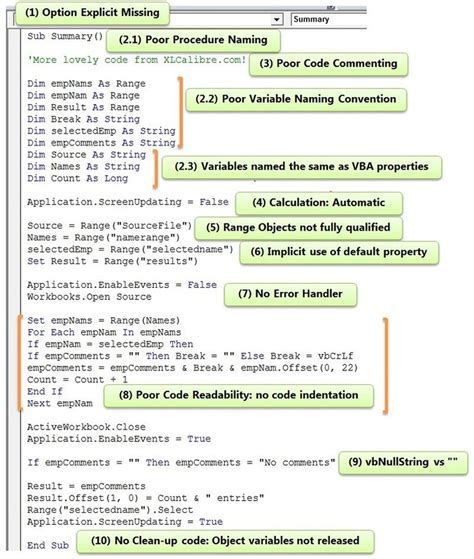
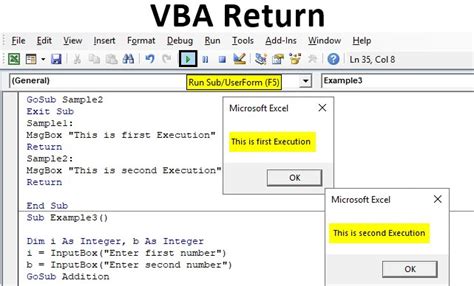
FAQs
What causes the "Ambiguous Name Detected" error in VBA?
+The error occurs due to naming conflicts within the VBA project, such as duplicate names for modules, variables, or subroutines.
How can I prevent naming conflicts in VBA?
+Plan your code structure, use meaningful and unique names for variables, subroutines, and modules, and avoid generic names.
What are some best practices for VBA development?
+Comment your code, test thoroughly, use version control, and follow a consistent naming convention.
If you've encountered the "Ambiguous Name Detected" error in your VBA projects, hopefully, this guide has provided you with the insights and steps needed to resolve the issue and improve your coding practices. Remember, clarity and uniqueness in naming conventions are key to avoiding such errors and making your code more maintainable and efficient.
Please feel free to share your experiences or ask further questions in the comments below. Your feedback is invaluable in helping others navigate the world of VBA programming and error resolution.