Intro
The importance of working with network paths in VBA (Visual Basic for Applications) cannot be overstated, especially when it comes to managing and automating tasks across different directories and locations. One of the fundamental commands used in such scenarios is the ChDir
statement, which changes the current directory to a specified path. When dealing with network paths, the process can become slightly more complex due to the way network locations are referenced and accessed. Understanding how to use ChDir
with network paths effectively is crucial for anyone looking to automate tasks or interact with files and folders across a network.
Working with network paths involves considering the unique addressing and security aspects of network environments. Network paths are often represented using the Universal Naming Convention (UNC) path, which provides a standard way of addressing resources on a network. However, VBA's ChDir
statement requires the path to be properly formatted to successfully change the directory to a network location. This involves understanding the syntax for specifying network paths, including the use of mapped drive letters, UNC paths, or other methods that might be specific to the operating system or the network configuration.
In the realm of VBA programming, mastering the ChDir
statement is essential for tasks such as file management, data transfer, and automation of repetitive tasks that involve navigating through different directories. The ability to change the current directory to a network path enables developers to access and manipulate files and folders located on network shares, which is a common requirement in many business environments. Moreover, understanding the nuances of working with network paths in VBA can help in troubleshooting issues related to file access and directory navigation, making it a valuable skill for anyone involved in VBA development.
Using ChDir with Network Paths in VBA
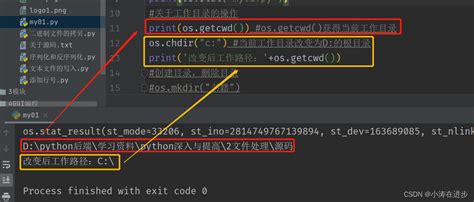
To use the ChDir
statement with a network path in VBA, you need to ensure that the path is correctly formatted. Here are some key considerations and steps to follow:
- Mapped Drive Letters: If the network path is mapped to a drive letter on your system, you can use the drive letter in the
ChDir
statement. For example, if the network share is mapped to the drive letter "Z:", you can useChDir "Z:\\"
to change the directory to the root of the network share. - UNC Paths: For UNC paths, which start with "\\", you need to ensure that the path is properly escaped in the VBA string. An example of using a UNC path with
ChDir
would beChDir "\\\\\\\\\\\\\\\\"
followed by the rest of the path. However, working directly with UNC paths in VBA can be tricky due to the need for double escaping backslashes.
Example Code for Using ChDir with Network Paths
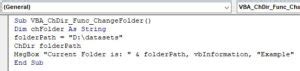
Sub ChangeDirectoryToNetworkPath()
' Using a mapped drive letter
ChDir "Z:\MyNetworkFolder"
' Alternatively, using a UNC path (ensure proper escaping of backslashes)
' ChDir "\\\\\\\\\\\\\\\" & "\\\\\" & "MyNetworkFolder"
' It's often easier and less error-prone to use the mapped drive letter
' or to construct the path using string variables to minimize the need for escaping.
End Sub
Benefits and Considerations of Using ChDir with Network Paths
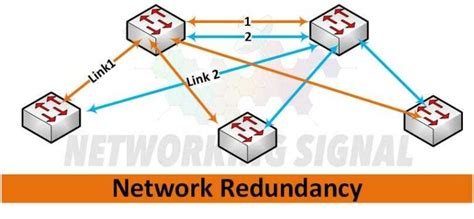
The benefits of using ChDir
with network paths include the ability to automate file management tasks across the network, access shared resources, and simplify directory navigation in VBA scripts. However, there are also considerations to keep in mind, such as:
- Security Permissions: Ensure that the VBA script has the necessary permissions to access the network path.
- Network Connectivity: The script's ability to change the directory to a network path depends on the network connection being available.
- Path Format: Correctly formatting the network path is crucial for the
ChDir
statement to work as expected.
Best Practices for Working with Network Paths in VBA
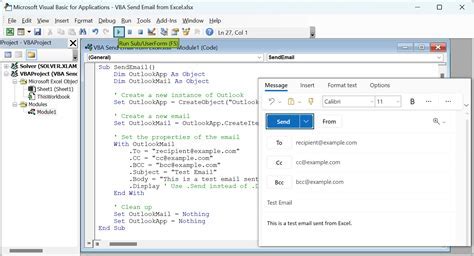
- Use Mapped Drive Letters: When possible, use mapped drive letters for simplicity and readability.
- Test UNC Paths Thoroughly: If using UNC paths, test them extensively to ensure they work as expected in different scenarios.
- Handle Errors: Implement error handling to manage situations where the network path is inaccessible.
Gallery of VBA ChDir Examples
VBA ChDir Gallery
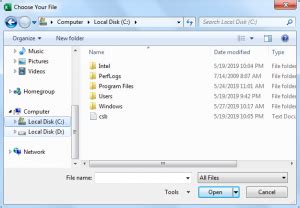
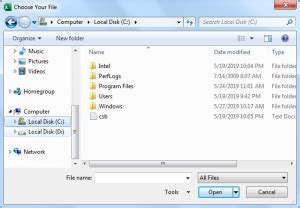
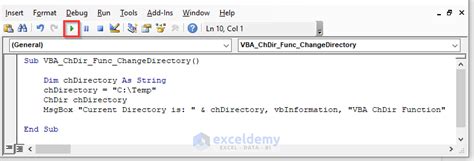
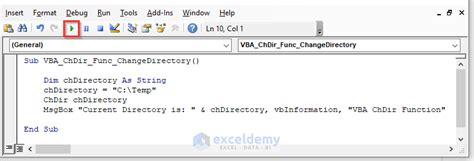
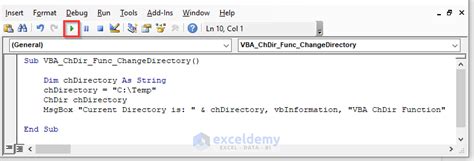
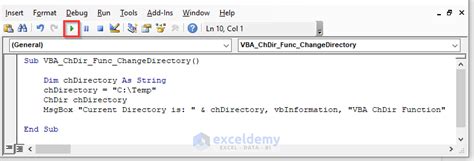
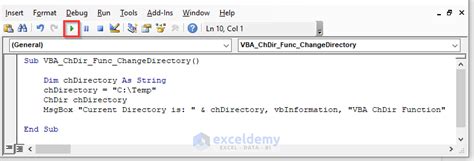
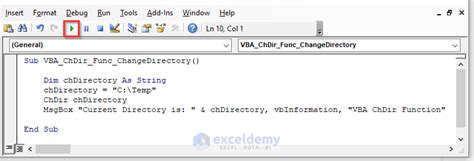
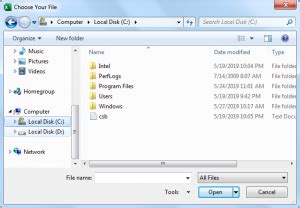
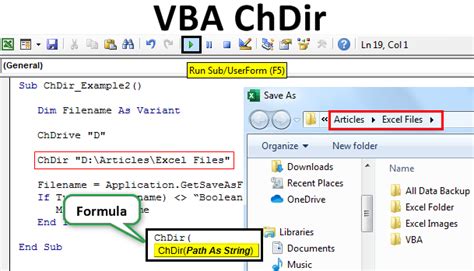
What is the ChDir statement used for in VBA?
+The ChDir statement is used to change the current directory to a specified path, allowing for navigation and access to files and folders in that directory.
How do I use a UNC path with the ChDir statement in VBA?
+To use a UNC path, ensure it is properly formatted and escaped in the VBA string, or consider using a mapped drive letter for simplicity.
What are some best practices for working with network paths in VBA?
+Best practices include using mapped drive letters when possible, thoroughly testing UNC paths, and implementing error handling for network connectivity issues.
In conclusion, mastering the use of the ChDir
statement with network paths in VBA is essential for efficient file management and automation across network environments. By understanding the correct formatting and usage of network paths, developers can leverage the full potential of VBA in managing and automating tasks that involve network resources. We invite you to share your experiences, ask questions, or provide insights into working with network paths in VBA, contributing to a community that values knowledge sharing and collaboration. Whether you're a seasoned developer or just starting out with VBA, exploring the capabilities and best practices of working with network paths can significantly enhance your productivity and the effectiveness of your VBA applications.