Intro
The Immediate window in Visual Basic for Applications (VBA) is a powerful tool for debugging and testing code. It allows you to execute commands and view results in real-time, which can be incredibly useful for troubleshooting and understanding how your code works. However, as you work with the Immediate window, it can become cluttered with previous commands and their outputs, making it harder to focus on your current task. Clearing the Immediate window can help you start fresh and improve your productivity. Here's how you can do it:
To clear the Immediate window manually, you can simply click inside the window and press Ctrl + A
to select all the text, and then press Delete
to remove it. This method is straightforward but can become tedious if you need to clear the window frequently.
A more efficient way to clear the Immediate window is by using a VBA command. You can type the following command into the Immediate window and press Enter
to execute it:
Debug.Clear
or
Application.Debug.Clear
However, these commands are not available in all VBA environments.
Another approach is to create a simple subroutine that you can run to clear the Immediate window. Here's how you can do it:
- Open the Visual Basic Editor (VBE) in your application (e.g., Excel, Word, etc.).
- In the VBE, go to
Insert
>Module
to insert a new module. - Paste the following code into the module:
Sub ClearImmediateWindow()
Dim i As Integer
For i = 1 To 200
Debug.Print vbCrLf
Next i
End Sub
- Save the module by clicking
File
>Save
(or pressCtrl + S
).
To clear the Immediate window using this subroutine, follow these steps:
- Open the Immediate window if it's not already open (
View
>Immediate Window
orCtrl + G
). - Type the following command into the Immediate window and press
Enter
:
ClearImmediateWindow
This subroutine works by printing a large number of blank lines to the Immediate window, effectively pushing any previous output out of view. While it doesn't truly "clear" the window in the sense of removing all text, it achieves a similar effect by making previous commands and outputs no longer visible.
Remember, the Debug.Clear
method is the most straightforward way to clear the Immediate window if it's available in your VBA environment. However, creating a custom subroutine like ClearImmediateWindow
provides a useful workaround when Debug.Clear
is not an option.
Benefits of Using the Immediate Window
- Debugging: The Immediate window is invaluable for debugging your VBA code. You can use it to check variable values, test expressions, and step through your code line by line.
- Testing: Before incorporating a piece of code into your larger project, you can test it in the Immediate window to ensure it works as expected.
- Learning: The Immediate window is a great place to experiment with VBA commands and learn how different functions and methods work.
Tips for Efficient Use of the Immediate Window
- Keep it Organized: Regularly clear the Immediate window to keep it organized and focused on your current task.
- Use it for Quick Tests: Instead of writing a full subroutine for a simple test, use the Immediate window to quickly execute commands and view results.
- Combine with Breakpoints: For more complex debugging, combine the use of the Immediate window with breakpoints in your code to step through and analyze your program's execution.
By mastering the use of the Immediate window and learning how to clear it efficiently, you can significantly enhance your productivity and effectiveness when working with VBA. Whether you're debugging, testing, or learning, the Immediate window is a powerful tool that can help you achieve your goals.

Advanced VBA Techniques
For those looking to delve deeper into VBA and explore more advanced techniques, understanding how to manipulate and utilize the Immediate window is just the beginning. Advanced topics might include creating custom user interfaces, working with external data sources, and optimizing code performance.

VBA Best Practices
Adopting best practices in VBA coding can significantly improve the quality, readability, and maintainability of your code. This includes using meaningful variable names, commenting your code, and structuring your projects in a logical and organized manner.
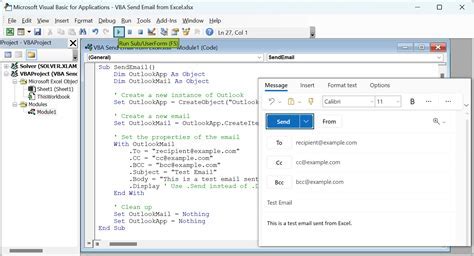
VBA Error Handling
Error handling is a critical aspect of VBA programming, allowing your applications to gracefully manage and recover from unexpected errors. By implementing robust error handling mechanisms, you can enhance the user experience and reduce the risk of data corruption or loss.
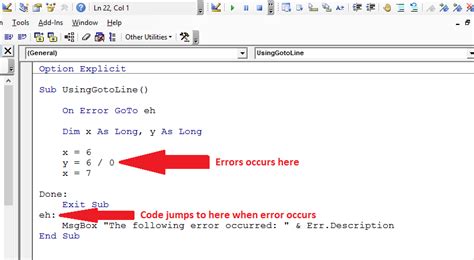
VBA Security
Ensuring the security of your VBA projects is essential, especially when distributing macros to other users. Understanding how to digitally sign your code, manage macro settings, and avoid common security pitfalls can help protect your work and the integrity of the systems it interacts with.
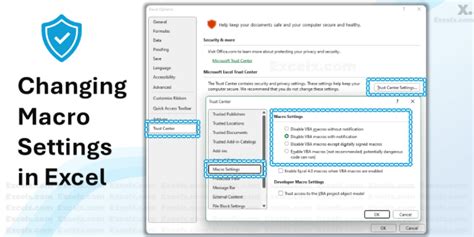
VBA and External Data
VBA's ability to interact with external data sources, such as databases, text files, and web services, opens up a wide range of possibilities for automating tasks and integrating different systems. Learning how to work with external data in VBA can significantly expand the capabilities of your applications.
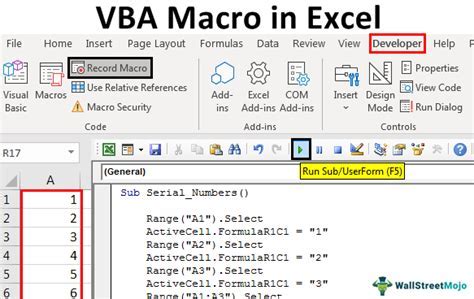
VBA Community and Resources
The VBA community is vast and active, with numerous online forums, tutorials, and blogs dedicated to sharing knowledge and best practices. Engaging with this community and leveraging available resources can be a great way to learn new skills, solve problems, and stay updated on the latest developments in VBA.

Gallery of VBA Topics
VBA Topics Image Gallery
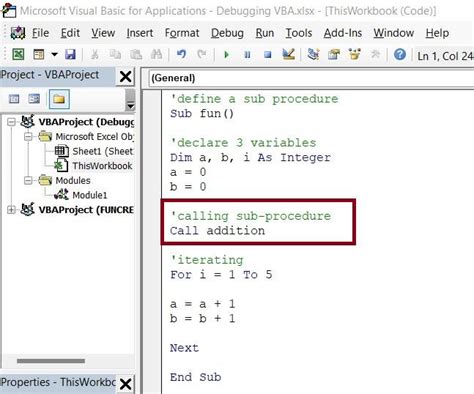
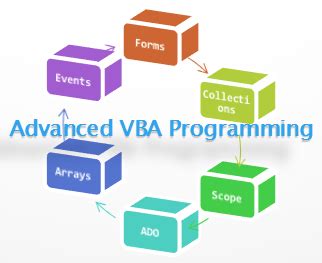
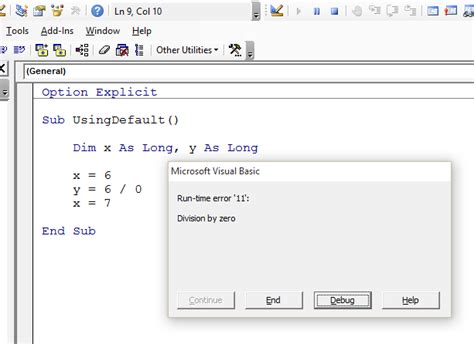
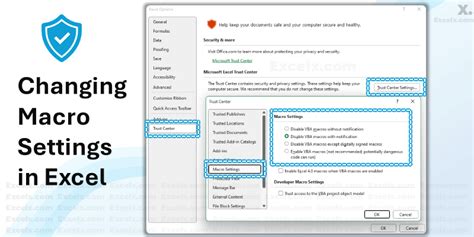
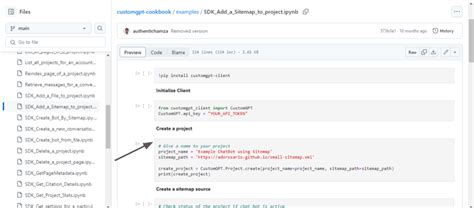
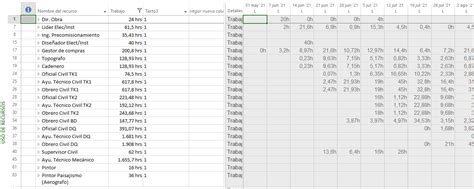
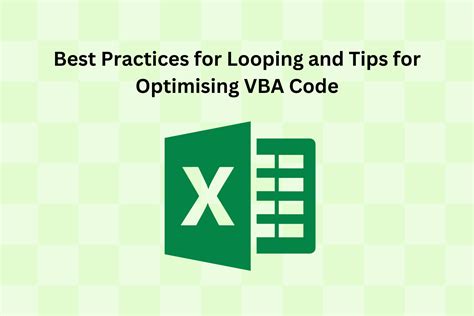
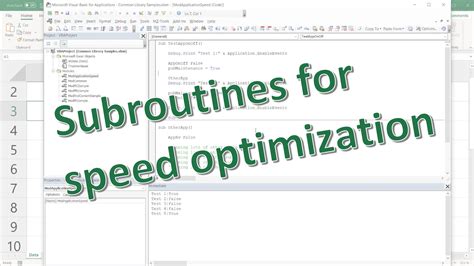
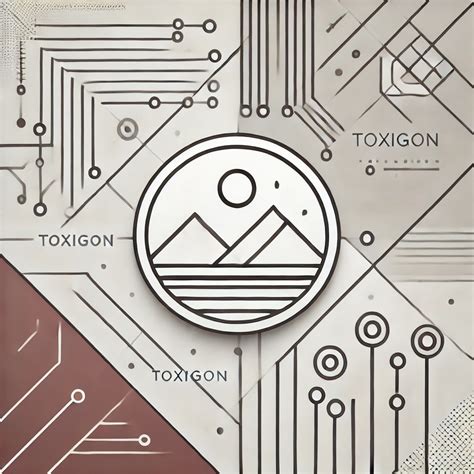
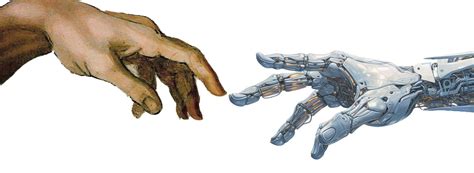
What is the purpose of the Immediate window in VBA?
+The Immediate window is used for debugging and testing VBA code, allowing users to execute commands and view results in real-time.
How do I clear the Immediate window in VBA?
+You can clear the Immediate window by typing Debug.Clear
and pressing Enter, or by creating and running a subroutine like ClearImmediateWindow
.
What are some best practices for using the Immediate window?
+Best practices include regularly clearing the window, using it for quick tests, and combining its use with breakpoints for more complex debugging.
To further enhance your understanding and skills in VBA, consider exploring the resources and community available online. With practice and dedication, you can become proficient in using the Immediate window and other VBA tools to create powerful and efficient applications. Whether you're automating tasks, analyzing data, or building custom interfaces, VBA offers a wide range of possibilities for those willing to learn and adapt. So, keep exploring, stay curious, and remember to share your knowledge with others to contribute to the vibrant VBA community.