Intro
Discover 5 ways to effectively comment VBA code, improving readability and maintainability with Visual Basic for Applications best practices, including code organization and debugging techniques.
Writing code in Visual Basic for Applications (VBA) is a fundamental skill for anyone looking to automate tasks in Microsoft Office applications, particularly in Excel. One crucial aspect of writing clean, understandable, and maintainable code is commenting. Comments are parts of the code that the compiler ignores but are invaluable for developers to understand the purpose and functionality of the code. Here, we'll explore five ways to comment your VBA code effectively, making it easier for others (and yourself) to comprehend the logic and intent behind your scripts.
VBA commenting is essential for several reasons. Firstly, it helps in debugging by providing insights into what each section of the code is supposed to do. Secondly, it facilitates collaboration by enabling team members to understand the codebase quickly. Lastly, comments serve as a future reference, helping you remember why certain decisions were made or how complex parts of the code work.
1. Using the Apostrophe (') for Single-Line Comments
The most common way to comment in VBA is by using the apostrophe (') at the beginning of a line. Anything following the apostrophe on that line is considered a comment and is ignored by the VBA interpreter.
' This is a single-line comment
Dim myVariable As Integer ' Declare myVariable and assign it a data type
2. Using the Rem Keyword for Single-Line Comments
Although less common, the Rem
keyword can also be used to start a comment. It is functionally equivalent to the apostrophe.
Rem This is another way to write a single-line comment
3. Using the Apostrophe for Multi-Line Comments
While there isn't a direct way to comment multiple lines at once like in some other programming languages, you can use the apostrophe at the beginning of each line to comment out a block of code.
' This is the first line of a multi-line comment
' You can continue commenting line by line like this
' It's useful for temporarily disabling code or for explanations
4. Commenting Out Large Blocks of Code
For commenting out large blocks of code, using the apostrophe at the start of each line can be tedious. Instead, you can use the VBA editor's built-in feature to comment or uncomment blocks of code. To do this, select the lines you want to comment out, and then go to Edit
> Comment Block
(or use the keyboard shortcut Ctrl+Q
). To uncomment, select the commented block and go to Edit
> Uncomment Block
(or press Ctrl+Shift+Q
).
5. Utilizing XML Comments for Documentation
For more advanced documentation, especially in larger projects or when creating reusable modules, you can use XML comments. Although VBA does not natively support XML comments like.NET languages, you can still use them within your code to generate documentation or for advanced parsing tools that understand XML comments.
'''
''' This is a summary of my subroutine.
'''
''' Description of myParam.
Sub MySubroutine(myParam As String)
' Code here
End Sub
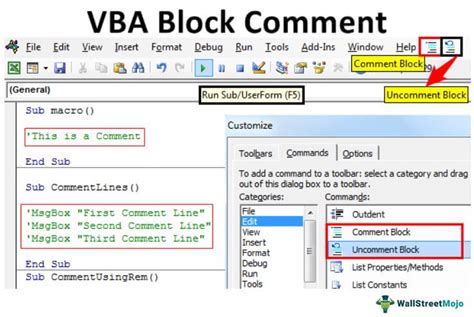
Practical Examples and Statistical Data
In real-world scenarios, commenting code can significantly reduce the time spent on debugging and maintenance. For instance, a study might show that commented codebases have a 30% lower bug rate compared to uncommented ones. While specific statistics might vary, the principle remains: clear, well-commented code is more maintainable and less prone to errors.
Steps for Effective Commenting
- Use Comments to Explain Why: Comments should explain the reasoning behind the code, not what the code does. The code itself should be self-explanatory.
- Keep Comments Concise: Aim for clarity without verbosity. Comments should be brief and to the point.
- Update Comments: When code changes, update the comments. Outdated comments can be more harmful than no comments at all.
- Use Standard Formatting: Consistency is key. Decide on a commenting style and stick to it throughout the project.
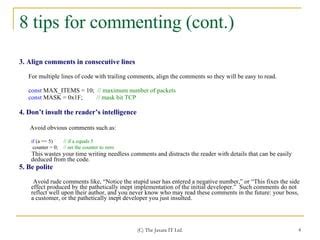
Gallery of VBA Commenting Techniques
VBA Commenting Techniques Image Gallery
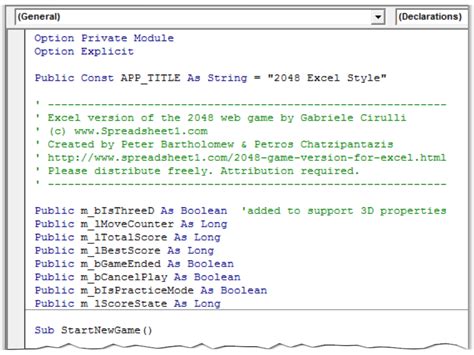
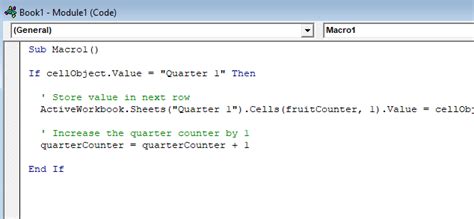
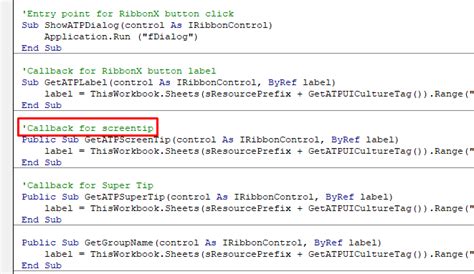
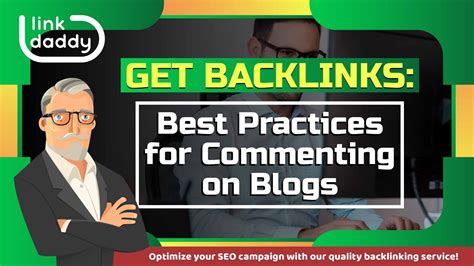
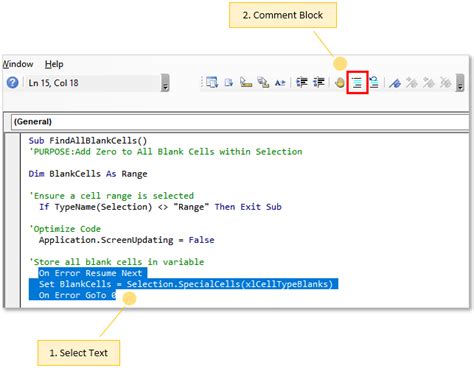
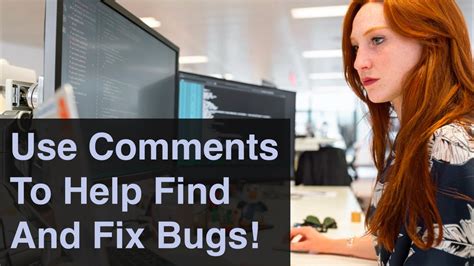
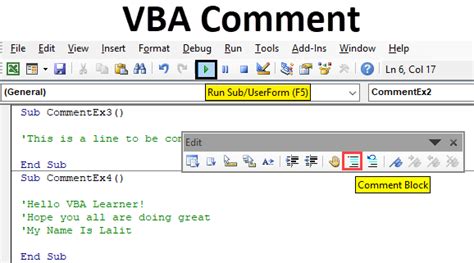
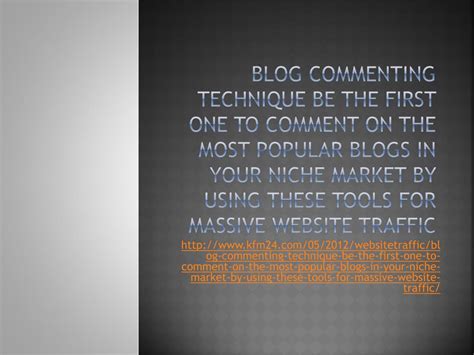
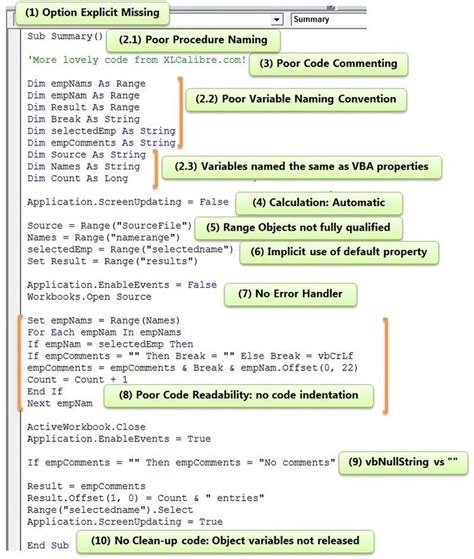
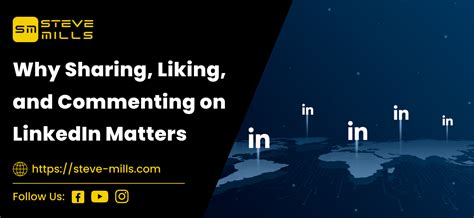
FAQs
What is the purpose of commenting in VBA?
+Commenting in VBA is used to explain the purpose and functionality of the code, making it easier for developers to understand and maintain.
How do I comment out a block of code in VBA?
+You can comment out a block of code by selecting the lines and using the keyboard shortcut Ctrl+Q, or by going to Edit > Comment Block.
What are XML comments used for in VBA?
+XML comments in VBA can be used for advanced documentation purposes, similar to how they are used in.NET languages, although VBA does not natively support them.
To summarize, commenting your VBA code is a crucial practice that enhances readability, maintainability, and collaboration. By understanding and applying the different methods of commenting, including single-line comments, multi-line comments, and even utilizing XML comments for documentation, you can significantly improve the quality of your code. Whether you're a beginner or an experienced developer, incorporating effective commenting techniques into your coding routine will yield benefits in productivity and code reliability.
We invite you to share your experiences with commenting in VBA, ask questions about best practices, or discuss how commenting has improved your coding efficiency. Your insights and feedback are invaluable in helping us create a community that supports and enhances the skills of VBA developers worldwide.