Intro
Learn how to Vba delete a file easily using Excel macros, file system objects, and VBA coding techniques, simplifying file management and automation tasks with related keywords like Excel VBA, file deletion, and macro programming.
Deleting a file using VBA (Visual Basic for Applications) can be a straightforward process, especially when you need to automate tasks or manage files within your Excel, Word, or other Office applications. VBA provides a powerful toolset for interacting with the operating system, including file management. Here's how you can easily delete a file using VBA, along with explanations and examples to guide you through the process.
The importance of knowing how to delete files via VBA lies in its ability to automate repetitive tasks. For instance, if you have a daily task that involves cleaning up temporary files or archiving old documents, VBA can help you achieve this efficiently. Moreover, understanding how to interact with the file system through VBA opens up a wide range of possibilities for customizing and automating your workflow.
Understanding the Basics of VBA File Deletion

To delete a file using VBA, you will typically use the Kill
statement. This statement requires you to specify the path and name of the file you wish to delete. It's crucial to exercise caution when using the Kill
statement, as it permanently deletes files without prompting for confirmation.
Basic Syntax of the Kill Statement
The basic syntax of the `Kill` statement is as follows: ```vba Kill pathname ``` Here, `pathname` is the path and name of the file you want to delete. For example: ```vba Kill "C:\Users\Username\Desktop\Example.txt" ``` This command would delete the file named `Example.txt` located on the desktop of the user `Username`.Practical Examples of Deleting Files with VBA
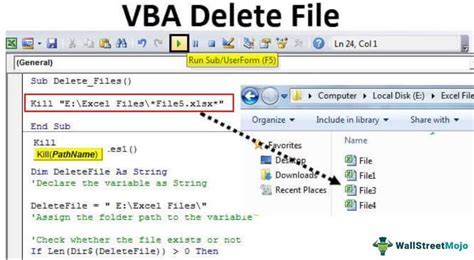
Example 1: Deleting a Specific File
If you want to delete a specific file, you can use the following code:
Sub DeleteSpecificFile()
Dim filePath As String
filePath = "C:\Users\Username\Desktop\Example.txt"
Kill filePath
End Sub
This subroutine defines the path to the file you want to delete and then uses the Kill
statement to delete it.
Example 2: Deleting Multiple Files
To delete multiple files, you can list each file path in the Kill
statement separated by commas:
Sub DeleteMultipleFiles()
Kill "C:\Users\Username\Desktop\Example1.txt", "C:\Users\Username\Desktop\Example2.txt"
End Sub
Alternatively, you can use a loop to delete files based on certain criteria, such as all files in a specific folder with a particular extension.
Deleting Files with a Loop
```vba Sub DeleteFilesWithLoop() Dim filePath As String Dim fileName As String' Specify the directory path
filePath = "C:\Users\Username\Desktop\"
' Specify the file extension
fileName = "*.txt"
' Use the Dir function to loop through files
fileName = Dir(filePath & "*.txt")
Do While fileName <> ""
Kill filePath & fileName
fileName = Dir
Loop
End Sub
This example deletes all `.txt` files in the specified directory.
Handling Errors and Exceptions
When working with file deletion in VBA, it's essential to handle potential errors, such as attempting to delete a file that does not exist or lacks the necessary permissions. You can use error handling mechanisms like `On Error Resume Next` or `On Error GoTo` to manage these scenarios.
```vba
Sub DeleteFileWithErrorHandling()
On Error Resume Next
Kill "C:\Users\Username\Desktop\Example.txt"
If Err.Number <> 0 Then
MsgBox "Error deleting file: " & Err.Description
End If
On Error GoTo 0
End Sub
This code checks if an error occurred during the file deletion attempt and displays a message box with the error description if necessary.
Best Practices for File Deletion in VBA
- Always specify the full path to the file you want to delete.
- Use error handling to catch and manage potential errors.
- Be cautious when using wildcards (
*
) to delete files, as this can lead to unintended file deletions. - Test your code in a safe environment before applying it to actual files.
Advanced File Management Techniques
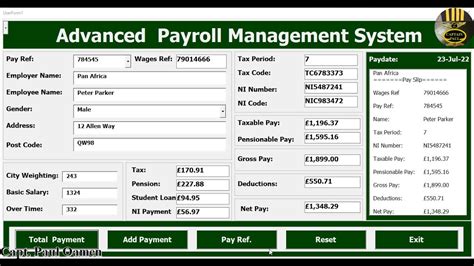
Beyond simple file deletion, VBA offers a range of advanced file management techniques. These include:
- File Existence Check: Before attempting to delete a file, you can check if it exists using the
Dir
function. - Folder Creation and Deletion: You can create new folders using the
MkDir
statement and delete them using theRmDir
statement. - File Attributes: You can modify file attributes, such as read-only or hidden, using the
SetAttr
statement.
Sub CheckFileExistence()
If Dir("C:\Users\Username\Desktop\Example.txt") <> "" Then
MsgBox "The file exists."
Else
MsgBox "The file does not exist."
End If
End Sub
Gallery of VBA File Management
VBA File Management Image Gallery
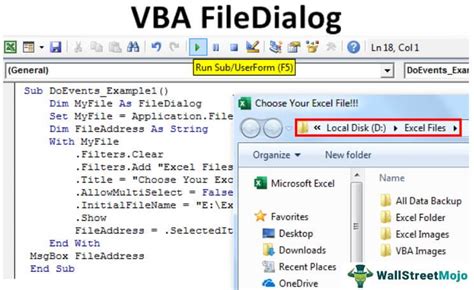
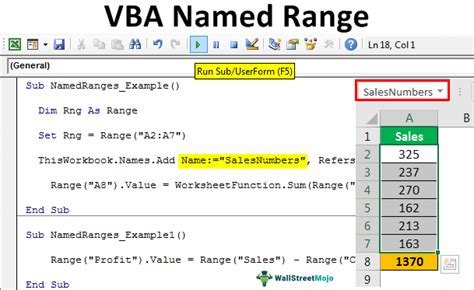
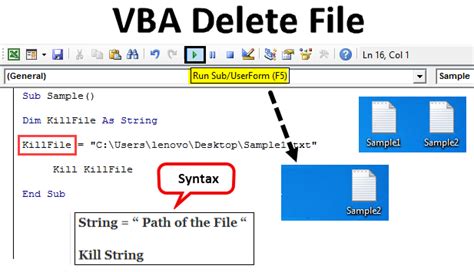
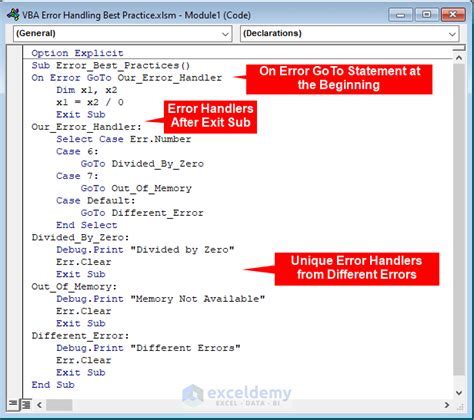

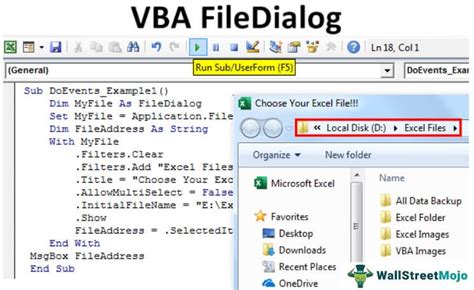
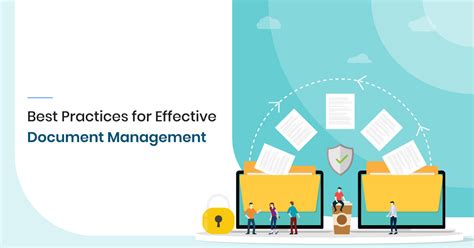
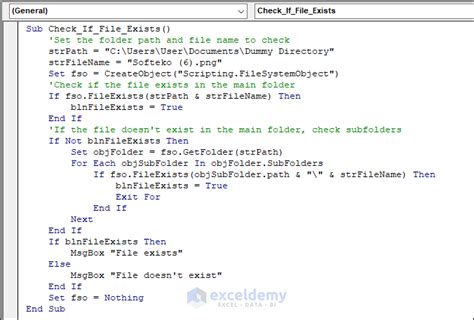
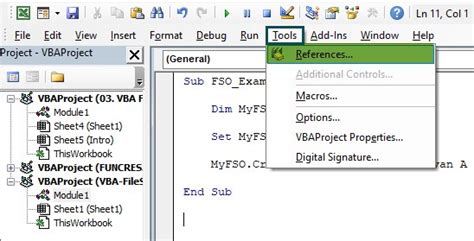
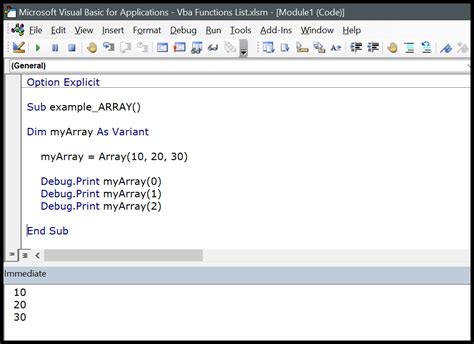
Frequently Asked Questions
What is the purpose of the Kill statement in VBA?
+The Kill statement is used to delete files in VBA. It requires the specification of the file path and name.
How can I check if a file exists before attempting to delete it?
+You can use the Dir function to check if a file exists. If Dir returns a string (the file name), the file exists; otherwise, it returns an empty string.
What are some best practices for deleting files with VBA?
+Always specify the full path to the file, use error handling, be cautious with wildcards, and test your code in a safe environment.
In conclusion, deleting files with VBA is a straightforward process that can be customized and automated to fit your needs. By understanding the basics of the Kill
statement, implementing error handling, and following best practices, you can efficiently manage files within your VBA applications. Whether you're automating tasks, cleaning up temporary files, or organizing your file system, VBA provides a powerful toolset to help you achieve your goals. We invite you to explore more about VBA file management, share your experiences, and ask questions to further enhance your understanding of this versatile programming language.