Intro
When working with Excel, one of the most common tasks is counting rows, whether it's to understand the scope of your data, to prepare it for analysis, or to ensure that your data is complete and accurate. Excel offers several ways to count rows, and one of the most powerful and flexible methods is by using Visual Basic for Applications (VBA). VBA allows you to automate tasks, including counting rows, with precision and speed.
Excel VBA provides a robust platform for automating repetitive tasks, and counting rows is one of the simplest yet most useful applications of VBA. Whether you're dealing with a small dataset or a large, complex spreadsheet, VBA can help you efficiently count rows based on various criteria, such as specific values, formatting, or conditions.
For those new to VBA, the concept might seem daunting, but it's actually quite accessible. VBA is essentially a programming language that allows you to interact with Excel (and other Microsoft Office applications) in a more direct way. By writing short scripts, or macros, you can perform a wide range of tasks, from simple actions like formatting cells to complex operations like data analysis and reporting.
To start counting rows with VBA, you first need to open the Visual Basic Editor. This can be done by pressing Alt + F11
while in Excel, or by navigating to the Developer tab (if available) and clicking on Visual Basic. If you don't see the Developer tab, you can add it by going to File > Options > Customize Ribbon and checking the Developer checkbox.
Once in the Visual Basic Editor, you can insert a new module by right-clicking on any of the objects for your workbook in the Project Explorer window, then choosing Insert > Module. This action creates a new module where you can write your VBA code.
Basic Row Counting with VBA
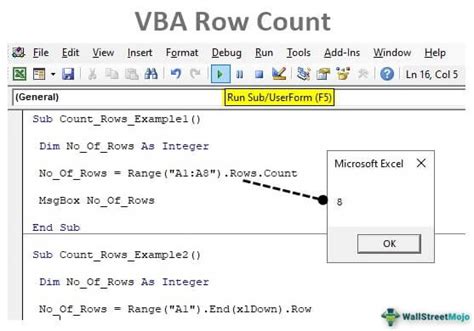
One of the simplest ways to count rows in VBA is by using the Rows.Count
property. However, this counts all rows in a worksheet, which might not be what you need if your data doesn't occupy the entire sheet. A more practical approach is to use the Range
object to specify the area of interest. For example, to count the number of rows in column A that contain data, you can use the following code:
Sub CountRowsInColumnA()
Dim lastRow As Long
lastRow = Cells(Rows.Count, "A").End(xlUp).Row
MsgBox "There are " & lastRow & " rows with data in column A."
End Sub
This script defines a subroutine named CountRowsInColumnA
, calculates the last row with data in column A by going to the bottom of the sheet and moving up until it finds a cell with data, and then displays a message box with the count.
Counting Rows Based on Conditions
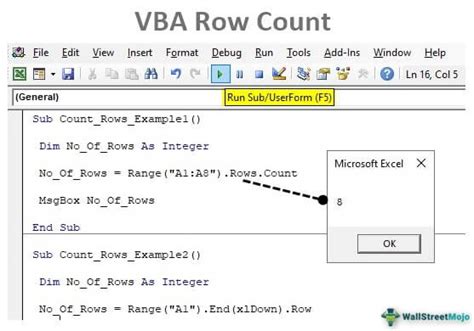
Often, you'll need to count rows based on specific conditions, such as the value in a certain column. VBA makes this easy by allowing you to loop through cells and check their values. For instance, to count how many rows have the word "Example" in column B, you could use:
Sub CountRowsWithExampleInColumnB()
Dim count As Long
Dim i As Long
count = 0
For i = 1 To Cells(Rows.Count, "B").End(xlUp).Row
If Cells(i, "B").Value = "Example" Then
count = count + 1
End If
Next i
MsgBox "There are " & count & " rows with 'Example' in column B."
End Sub
This code loops through each cell in column B, checks if the value is "Example", and increments a counter if it is. Finally, it displays the total count.
Using VBA to Count Rows with Multiple Conditions
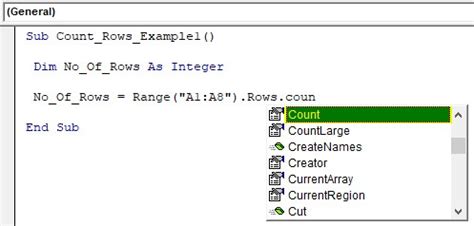
In many cases, you might need to count rows based on multiple conditions. This could involve checking values in different columns or applying more complex logic. VBA supports this through the use of logical operators (And
, Or
, Not
) in your If
statements. For example, to count rows where column A is "Yes" and column B is greater than 10, you could use:
Sub CountRowsWithMultipleConditions()
Dim count As Long
Dim i As Long
count = 0
For i = 1 To Cells(Rows.Count, "B").End(xlUp).Row
If Cells(i, "A").Value = "Yes" And Cells(i, "B").Value > 10 Then
count = count + 1
End If
Next i
MsgBox "There are " & count & " rows meeting the conditions."
End Sub
This code demonstrates how to apply multiple conditions to count rows, making it a powerful tool for data analysis.
Benefits of Using VBA for Row Counting
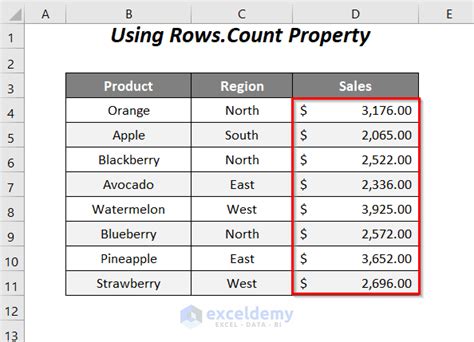
Using VBA for row counting offers several benefits, including flexibility, precision, and speed. Unlike manual methods or formulas, VBA scripts can be easily modified to accommodate changing requirements or different datasets. This flexibility, combined with the ability to perform complex logic and conditionals, makes VBA an indispensable tool for anyone working extensively with Excel.
Furthermore, VBA scripts can be run with a single click, making repetitive tasks much faster than manual methods. This not only saves time but also reduces the chance of human error, ensuring that your counts are accurate and reliable.
Steps to Implement VBA Row Counting
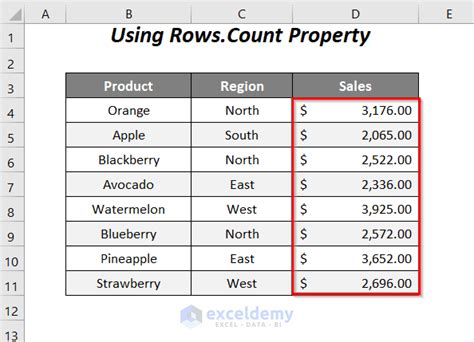
Implementing VBA for row counting involves several steps:
- Open the Visual Basic Editor: Press
Alt + F11
or navigate to the Developer tab and click on Visual Basic. - Insert a New Module: Right-click on any object for your workbook in the Project Explorer, then choose Insert > Module.
- Write Your VBA Code: In the module window, write the VBA script to count rows based on your needs.
- Run the Macro: Press
F5
while in the Visual Basic Editor with your script window active, or close the editor and run the macro from the Developer tab in Excel. - Test and Refine: Verify that your script works as expected and refine it as necessary to meet your specific requirements.
Practical Examples and Statistical Data
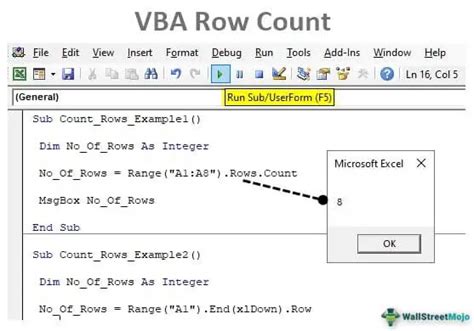
In practice, VBA row counting can be applied to a wide range of scenarios, from simple data summaries to complex data analysis tasks. For instance, in a sales dataset, you might use VBA to count the number of sales by region, product category, or time period, providing valuable insights into sales trends and patterns.
Statistical data can also be analyzed using VBA row counting. For example, you could count the number of data points that fall within a certain range or meet specific statistical criteria, aiding in the identification of outliers, trends, or correlations within the data.
VBA Excel Row Counting Image Gallery
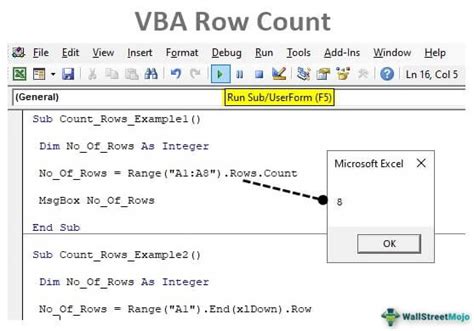
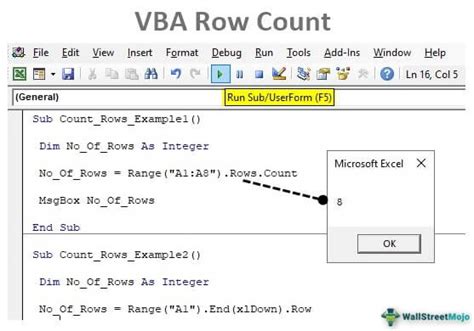
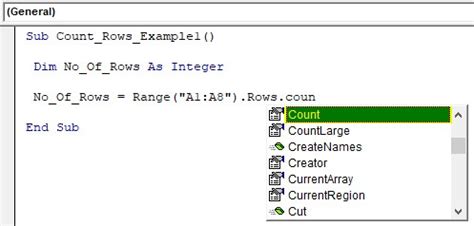
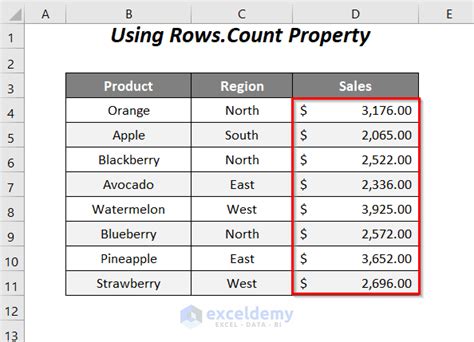
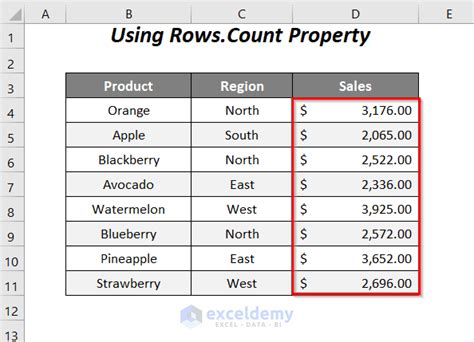
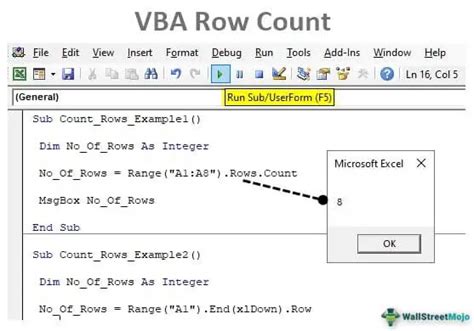
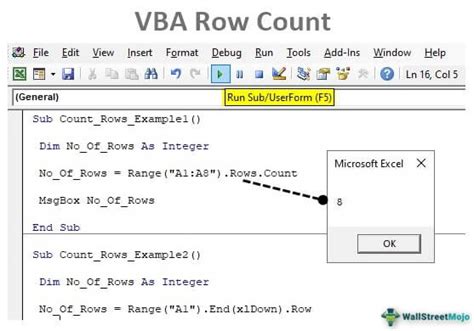
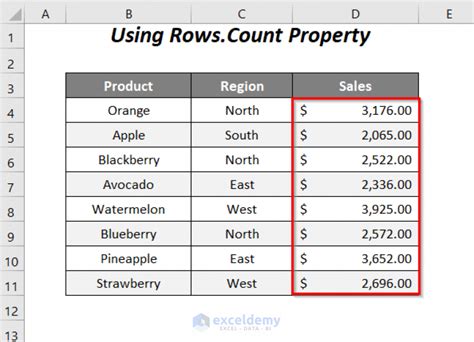
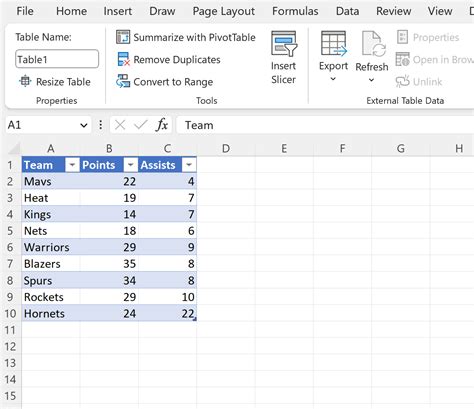
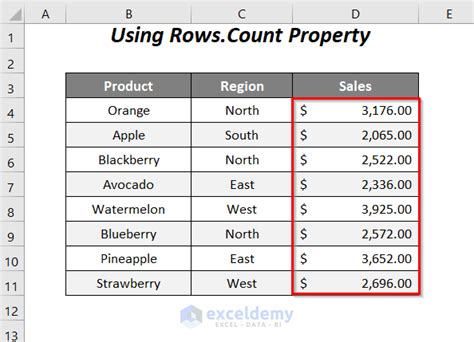
What is VBA in Excel?
+VBA stands for Visual Basic for Applications. It is a programming language developed by Microsoft that is used for creating and automating tasks in Microsoft Office applications like Excel.
How do I open the Visual Basic Editor in Excel?
+You can open the Visual Basic Editor by pressing `Alt + F11` while in Excel, or by navigating to the Developer tab and clicking on Visual Basic.
What are the benefits of using VBA for row counting in Excel?
+The benefits include flexibility, precision, and speed. VBA allows for complex logic and conditionals, making it ideal for tasks that require more than simple counting, and it reduces the chance of human error.
Can I use VBA to count rows based on multiple conditions?
+Yes, VBA supports the use of logical operators (`And`, `Or`, `Not`) in `If` statements, allowing you to count rows based on multiple conditions.
How do I run a VBA macro in Excel?
+You can run a VBA macro by pressing `F5` while in the Visual Basic Editor with your script window active, or by closing the editor and running the macro from the Developer tab in Excel.
In summary, using VBA to count rows in Excel offers a powerful and flexible way to manage and analyze your data. Whether you're looking to perform simple counts or complex data analysis, VBA provides the tools you need. By following the steps and examples outlined above, you can leverage VBA to streamline your workflow, reduce errors, and gain deeper insights into your data. If you have any further questions or would like to explore more advanced VBA techniques, feel free to comment below or share this article with others who might find it useful.