Intro
Discover 5 ways to VBA select column efficiently, using range, cells, and worksheet objects, with tips on looping, error handling, and optimization for Excel automation and macros.
The importance of selecting columns in Excel VBA cannot be overstated, as it forms the foundation of many tasks, from data manipulation to report generation. Selecting columns allows developers to target specific data ranges, perform operations on them, and automate tasks efficiently. This article delves into the various methods of selecting columns using Excel VBA, exploring their applications and providing practical examples to guide readers through the process.
Excel VBA is a powerful tool for automating tasks in Excel, and understanding how to select and manipulate data is crucial for anyone looking to leverage its capabilities. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to create complex applications, mastering column selection is a fundamental skill. Throughout this article, we'll explore different scenarios and methods for selecting columns, ensuring that readers have a comprehensive understanding of the topic.
The need to select columns arises in numerous scenarios, such as formatting specific data, performing calculations on certain ranges, or even copying data from one worksheet to another. Excel VBA provides several ways to achieve this, catering to different requirements and preferences. By the end of this article, readers will be equipped with the knowledge to select columns efficiently, paving the way for more complex and sophisticated VBA applications.
Understanding VBA Column Selection
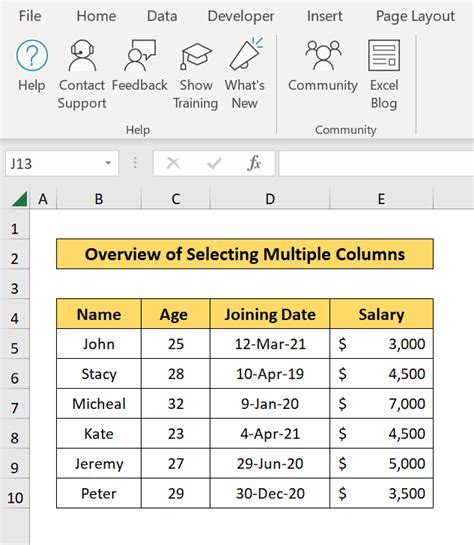
To begin with, it's essential to understand the basics of VBA and how Excel interacts with it. VBA, or Visual Basic for Applications, is a programming language developed by Microsoft, allowing users to create and automate tasks in Microsoft Office applications. In Excel, VBA can be used to perform a wide range of tasks, from simple automation to complex data analysis and manipulation. Selecting columns is a fundamental aspect of this, as it enables developers to specify exactly which data they want to work with.
Method 1: Using the `Range` Object
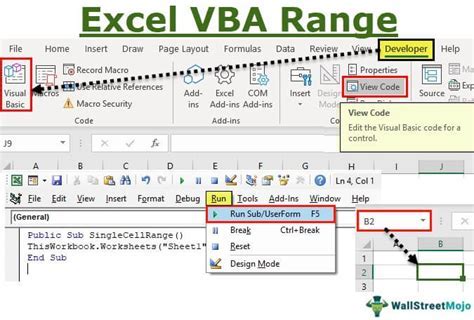
One of the most straightforward ways to select a column in VBA is by using the Range
object. This method involves specifying the exact range of cells you wish to select. For example, if you want to select the entire first column (Column A), you can use the following code:
Sub SelectColumnA()
Range("A:A").Select
End Sub
This code defines a subroutine named SelectColumnA
that, when executed, selects the entire first column.
Benefits of Using the `Range` Object
- Precision: Allows for precise selection of columns based on their letter designation (e.g., "A" for the first column).
- Flexibility: Can be used to select multiple columns by specifying a range (e.g., "A:C" for the first three columns).
- Ease of Use: The syntax is straightforward, making it easy for beginners to understand and implement.
Method 2: Using the `Columns` Object
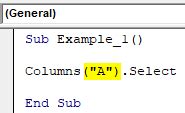
Another method for selecting columns involves the Columns
object. This object allows you to select columns based on their numerical position. For instance, to select the second column (Column B), you can use:
Sub SelectColumnB()
Columns(2).Select
End Sub
This code selects the second column, demonstrating how the Columns
object can be used for column selection based on the column's numerical index.
Benefits of Using the `Columns` Object
- Numerical Reference: Useful when the column position is known but the letter designation is not.
- Dynamic Selection: Can be used within loops to select columns dynamically based on changing conditions.
Method 3: Using the `Cells` Object
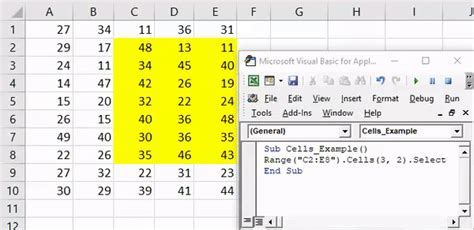
The Cells
object provides another versatile method for selecting columns by specifying the row and column numbers of the range you wish to select. For example, to select the entire third column, you can use:
Sub SelectColumnC()
Range(Cells(1, 3), Cells(Rows.Count, 3)).Select
End Sub
This code selects the third column by referencing the cells in the first and last rows of the third column.
Benefits of Using the `Cells` Object
- Row and Column Specification: Allows for the selection of ranges based on both row and column numbers.
- Dynamic Range Selection: Particularly useful for selecting dynamic ranges that may change based on data input or other factors.
Method 4: Using the `EntireColumn` Property
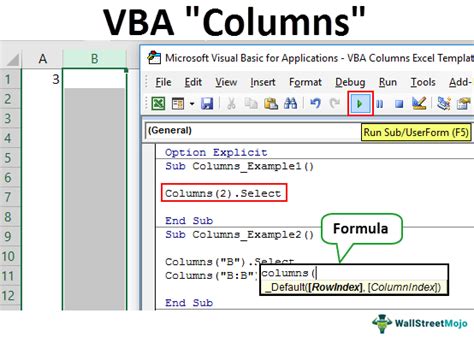
The EntireColumn
property is a straightforward method to select an entire column based on a specific cell. For example, to select the entire column of the active cell, you can use:
Sub SelectEntireColumn()
ActiveCell.EntireColumn.Select
End Sub
This method is convenient when you need to perform operations on the entire column containing the currently selected cell.
Benefits of Using the `EntireColumn` Property
- Convenience: Easy to use when the active cell is within the column you wish to select.
- Contextual Selection: Automatically selects the entire column of the active cell, reducing the need for manual range specification.
Method 5: Using Loops for Dynamic Column Selection
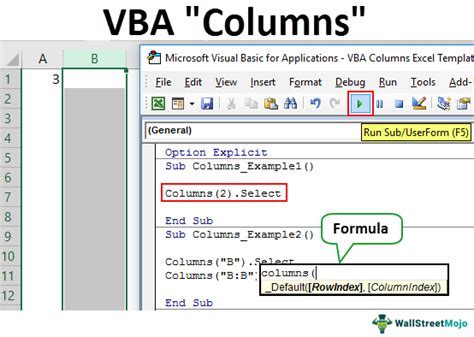
For scenarios where you need to select columns dynamically based on certain conditions, using loops in conjunction with the methods described above can be highly effective. For example, to select all columns that contain a specific value in the first row, you could use a loop like this:
Sub SelectColumnsBasedOnValue()
For Each cell In Range("1:1") 'Assuming the value to search is in the first row
If cell.Value = "SpecificValue" Then
cell.EntireColumn.Select
End If
Next cell
End Sub
This code loops through each cell in the first row, selecting the entire column if the cell contains the specified value.
Benefits of Using Loops
- Dynamic Selection: Enables the selection of columns based on dynamic conditions or criteria.
- Flexibility: Can be combined with various selection methods to achieve complex selection logic.
VBA Column Selection Image Gallery
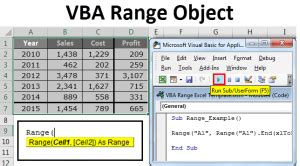
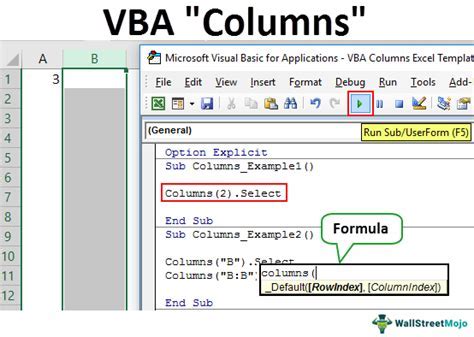
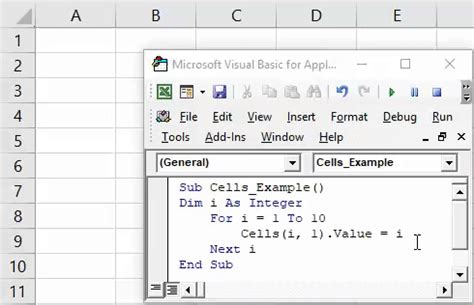

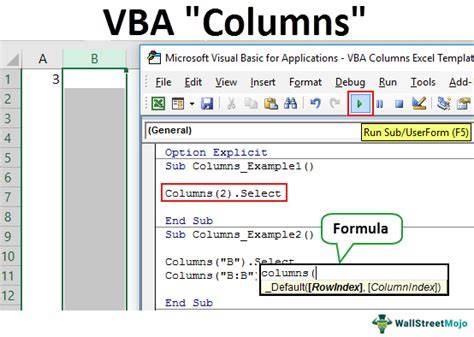
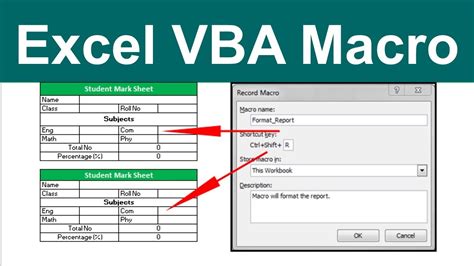
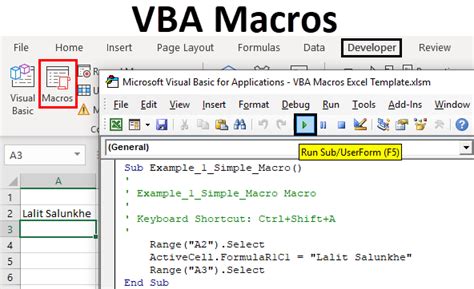
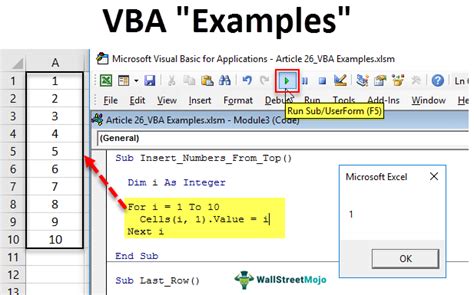
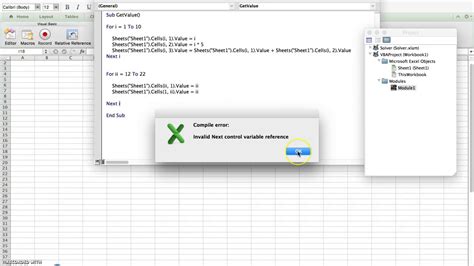
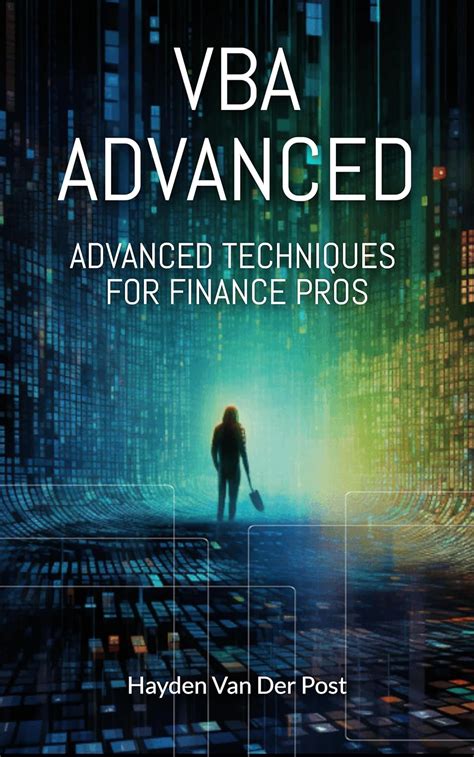
What is the most efficient way to select columns in Excel VBA?
+The most efficient way often depends on the specific task and data structure. However, using the `Range` or `Columns` object can be very efficient for direct selections.
Can I select multiple columns at once using VBA?
+Yes, you can select multiple columns by specifying a range (e.g., `Range("A:C").Select`) or by using the `Union` method to combine multiple `Range` objects.
How do I dynamically select columns based on certain conditions in VBA?
+You can use loops (For Each, For Next) to iterate through cells or columns and apply conditions to select the desired columns dynamically.
In conclusion, selecting columns in Excel VBA is a fundamental skill that can greatly enhance your ability to automate tasks and manipulate data. By understanding and applying the various methods outlined in this article, you can efficiently select columns based on your specific needs, whether it's for simple formatting, complex data analysis, or anything in between. We invite you to share your experiences, ask questions, or provide feedback on using VBA for column selection, and don't forget to share this article with others who might benefit from mastering this essential VBA skill.