Intro
Unlock efficient data management with VBA Text To Columns, utilizing macros, data parsing, and spreadsheet automation to split and organize text data effectively.
The ability to convert text to columns in Excel is a powerful feature that can save time and effort when working with large datasets. While Excel provides a built-in "Text to Columns" feature, using VBA (Visual Basic for Applications) can offer more flexibility and automation. In this article, we will delve into the world of VBA text to columns, exploring its benefits, how it works, and providing step-by-step guides on implementing this functionality.
When dealing with data in Excel, it's common to encounter text strings that contain multiple values separated by a specific delimiter, such as commas, tabs, or spaces. The "Text to Columns" feature allows users to split these strings into separate columns, making data analysis and manipulation easier. However, for more complex data processing tasks or when dealing with large datasets, using VBA can provide a more efficient and customized solution.
VBA Text to Columns: Benefits and Working Mechanism
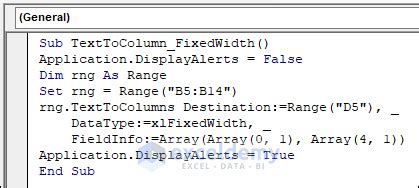
The primary benefit of using VBA for text to columns is automation. By writing a script, users can apply the text to columns functionality to multiple worksheets, workbooks, or even across an entire Excel application with a single command. This level of automation is particularly useful for tasks that need to be performed regularly or on large datasets where manual intervention would be time-consuming and prone to errors.
Moreover, VBA allows for more complex logic to be applied during the text to columns process. For example, users can specify conditions under which the text should be split, handle multiple delimiters, or even perform additional data manipulation steps immediately after splitting the text.
Basic Steps for Implementing VBA Text to Columns
To implement VBA text to columns, follow these basic steps: 1. **Open the Visual Basic Editor**: Press `Alt + F11` or navigate to Developer > Visual Basic in the ribbon to open the VBA editor. 2. **Insert a Module**: In the VBA editor, right-click on any of the objects for your workbook listed in the "Project" window. Choose `Insert` > `Module` to insert a new module. 3. **Write the VBA Code**: In the module window, you can write your VBA script. The code will typically involve looping through cells in a specified range, using the `Split` function to divide the text based on a delimiter, and then outputting the results to adjacent columns.Example VBA Code for Text to Columns
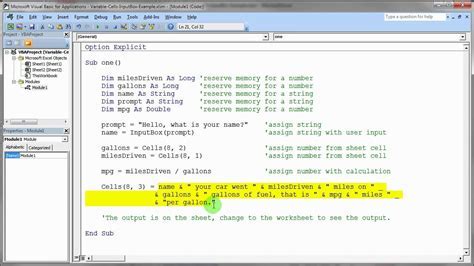
Here's a simple example of VBA code that splits text in column A based on a comma delimiter and outputs the results to columns B, C, etc.:
Sub TextToColumnsVBA()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim rng As Range
Set rng = ws.Range("A1:A10") ' Define the range to process
Dim cell As Range
For Each cell In rng
Dim text As String
text = cell.Value
Dim splitText() As String
splitText = Split(text, ",")
Dim i As Integer
For i = 0 To UBound(splitText)
ws.Cells(cell.Row, cell.Column + i + 1).Value = splitText(i)
Next i
Next cell
End Sub
This code snippet demonstrates how to loop through each cell in a specified range, split the text based on a comma, and then output each part of the split text to the next column.
Advanced VBA Text to Columns Techniques
For more complex scenarios, VBA offers advanced techniques such as handling multiple delimiters, skipping certain columns, or applying conditional logic to determine how the text should be split.- Handling Multiple Delimiters: Instead of using a single delimiter, you can use an array of delimiters and loop through each one to split the text.
- Conditional Splitting: You can apply conditions to decide whether or not to split the text in a particular cell. This could be based on the cell's value, its position, or other criteria.
- Error Handling: Implementing error handling is crucial when working with VBA. This ensures that your script can gracefully handle unexpected situations, such as encountering a cell with no text or dealing with an unexpected delimiter.
Practical Applications of VBA Text to Columns

The practical applications of VBA text to columns are vast and varied. Some common scenarios include:
- Data Import and Cleanup: Often, data imported from external sources requires cleaning up, including splitting text into separate columns.
- Automating Repetitive Tasks: For tasks that need to be performed regularly, such as weekly or monthly reports, automating the text to columns process can save significant time and reduce the likelihood of human error.
- Complex Data Analysis: In cases where data analysis requires splitting text based on complex rules or multiple conditions, VBA provides the flexibility to implement these rules efficiently.
Best Practices for Using VBA Text to Columns
When using VBA for text to columns, keep the following best practices in mind: - **Test Your Code**: Always test your VBA code on a small sample of data before applying it to a larger dataset. - **Use Meaningful Variable Names**: Choose variable names that clearly indicate their purpose to make your code easier to understand and maintain. - **Comment Your Code**: Adding comments to your code can help explain what each section of the code is intended to do, making it easier for others (or yourself in the future) to understand.VBA Text to Columns Image Gallery
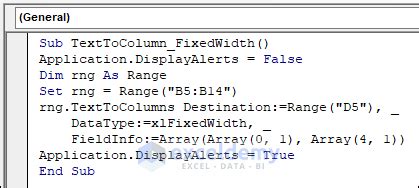
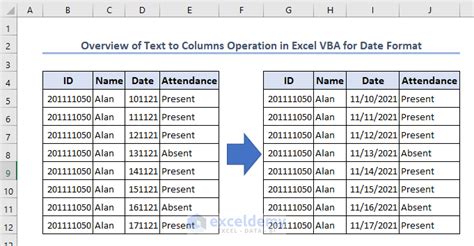
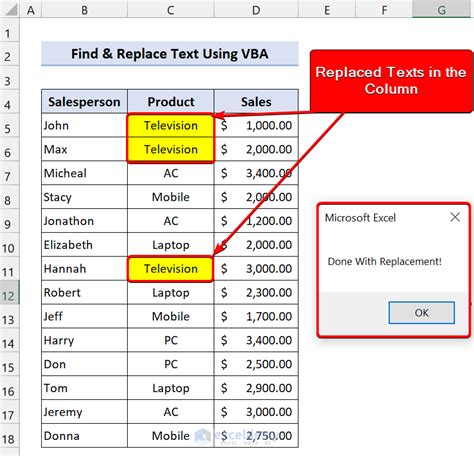
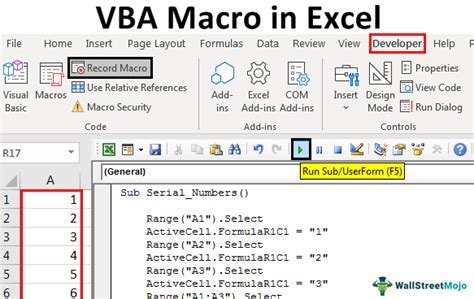
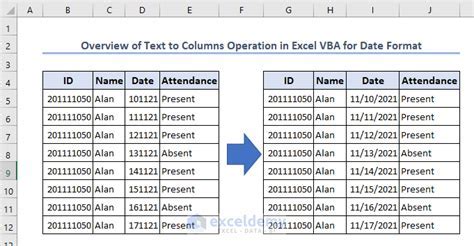
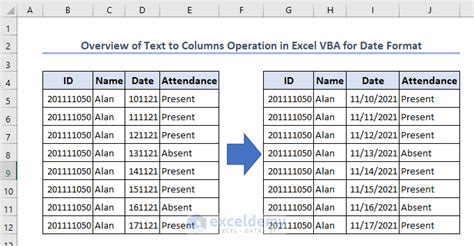
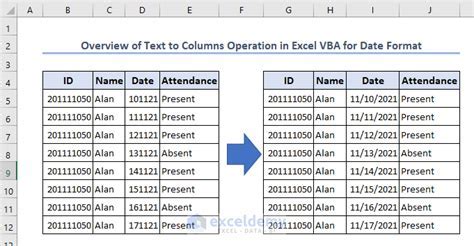
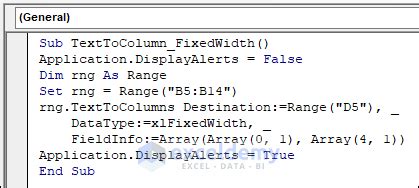
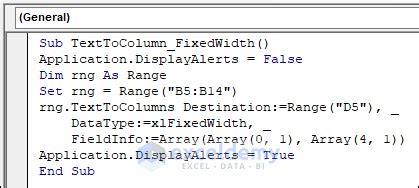
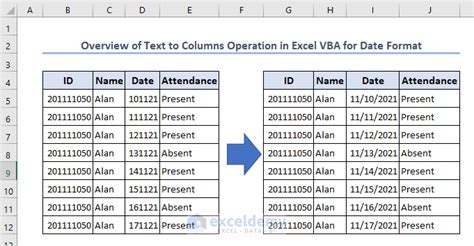
What is VBA text to columns used for?
+VBA text to columns is used to split text strings into separate columns based on a specified delimiter, allowing for more efficient data analysis and manipulation in Excel.
How do I implement VBA text to columns?
+To implement VBA text to columns, you need to open the Visual Basic Editor, insert a module, and write the VBA code that specifies the range to process, the delimiter to use, and how to output the split text.
What are the benefits of using VBA for text to columns?
+The benefits include automation, flexibility in handling complex logic, and the ability to apply the functionality across multiple worksheets or workbooks with ease.
In conclusion, leveraging VBA for text to columns in Excel offers a powerful way to automate and customize the process of splitting text into separate columns. By understanding the benefits, working mechanism, and practical applications of VBA text to columns, users can enhance their data analysis and manipulation capabilities. Whether you're dealing with simple or complex data processing tasks, VBA provides the tools to streamline your workflow and improve productivity. We invite you to share your experiences or ask questions about using VBA for text to columns, and don't forget to share this article with others who might benefit from learning about this valuable Excel feature.