Intro
Master Array Of Arrays Vba with expert guidance on multidimensional arrays, nested loops, and dynamic indexing, optimizing your VBA code for efficient data processing and manipulation.
In Visual Basic for Applications (VBA), an array of arrays is a data structure that allows you to store and manipulate multiple arrays within a single variable. This can be particularly useful when working with complex data sets or when you need to perform operations on multiple arrays simultaneously.
To create an array of arrays in VBA, you typically declare a variable as an array of a specific type, such as Variant, which can hold arrays. Here's a basic example of how to declare and use an array of arrays:
Sub ArrayOfArraysExample()
' Declare an array of arrays
Dim arrayOfArrays() As Variant
ReDim arrayOfArrays(1 To 3) ' Adjust size as needed
' Initialize each element of the array as an array
Dim i As Integer
For i = LBound(arrayOfArrays) To UBound(arrayOfArrays)
ReDim arrayOfArrays(i) As Variant
Next i
' However, the above approach is incorrect for creating an array of arrays.
' Correct approach:
Dim arr1() As Variant, arr2() As Variant, arr3() As Variant
' Initialize each array
ReDim arr1(1 To 3)
ReDim arr2(1 To 3)
ReDim arr3(1 To 3)
' Populate the arrays
arr1(1) = "Element 1-1"
arr1(2) = "Element 1-2"
arr1(3) = "Element 1-3"
arr2(1) = "Element 2-1"
arr2(2) = "Element 2-2"
arr2(3) = "Element 2-3"
arr3(1) = "Element 3-1"
arr3(2) = "Element 3-2"
arr3(3) = "Element 3-3"
' Now, correctly creating an array of arrays
Dim correctArrayOfArrays(1 To 3) As Variant
correctArrayOfArrays(1) = arr1
correctArrayOfArrays(2) = arr2
correctArrayOfArrays(3) = arr3
' Accessing elements
For i = LBound(correctArrayOfArrays) To UBound(correctArrayOfArrays)
For j = LBound(correctArrayOfArrays(i)) To UBound(correctArrayOfArrays(i))
Debug.Print correctArrayOfArrays(i)(j)
Next j
Next i
End Sub
This example illustrates the basic concept of creating and manipulating an array of arrays in VBA. However, the initial attempt to declare an array of arrays directly is corrected to reflect the proper method of creating separate arrays and then assigning them to an array of Variant type.
Benefits of Using Arrays of Arrays
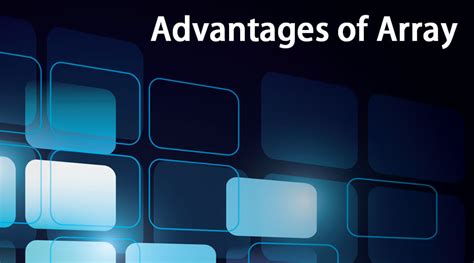
Using arrays of arrays in VBA offers several benefits, including:
- Efficient Data Storage: Arrays of arrays allow for the organization of complex data in a structured and accessible manner.
- Improved Code Readability: By encapsulating related arrays within a single variable, your code can become more readable and easier to understand.
- Simplified Data Manipulation: Operations can be performed on the entire collection of arrays or on individual arrays within the collection, streamlining data processing.
Common Applications
Arrays of arrays find applications in various scenarios, such as: - **Data Analysis**: When working with multiple datasets that need to be analyzed or compared. - **Simulation Models**: In simulations where multiple scenarios or variables need to be tested. - **Game Development**: For managing game states, levels, or character attributes.Working with Arrays of Arrays
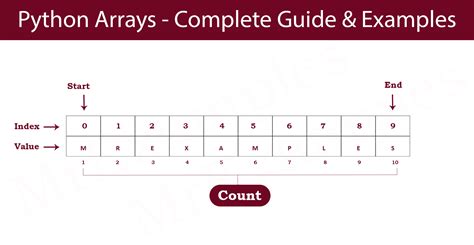
To effectively work with arrays of arrays, consider the following steps:
- Declare the Array: Declare a variable as an array of Variant type to hold the arrays.
- Initialize Sub-arrays: Create and initialize each sub-array that will be part of the array of arrays.
- Assign Sub-arrays: Assign each sub-array to an element of the main array.
- Access Elements: Use nested loops to access and manipulate elements within the sub-arrays.
Best Practices
- **Use Meaningful Variable Names**: Choose names that reflect the purpose of the array and its contents. - **Document Your Code**: Comments can help explain complex operations on arrays of arrays. - **Test Thoroughly**: Ensure that your code correctly handles all possible scenarios and edge cases.Advanced Techniques
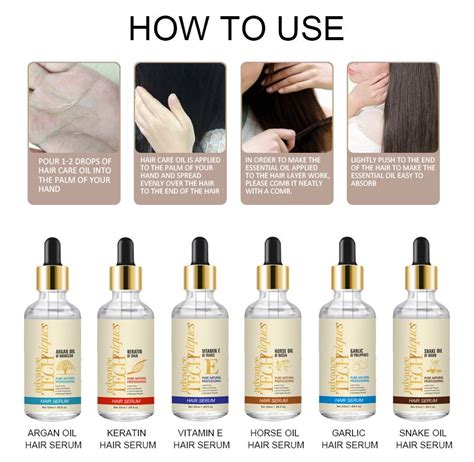
For more complex data structures or operations, consider:
- Jagged Arrays: Arrays of arrays where each sub-array can be of a different size.
- Dynamic Resizing: Using ReDim Preserve to dynamically adjust the size of arrays as needed.
- Object-Oriented Programming: Encapsulating arrays of arrays within custom objects for more organized code.
Performance Considerations
- **Memory Usage**: Large arrays of arrays can consume significant memory. - **Loop Optimization**: Optimize loops that iterate over arrays of arrays for better performance. - **Alternative Data Structures**: Consider using Collections or Dictionaries for certain applications.Array of Arrays Image Gallery
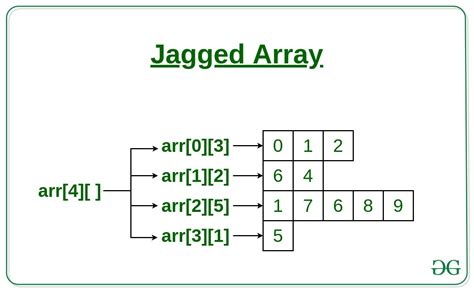
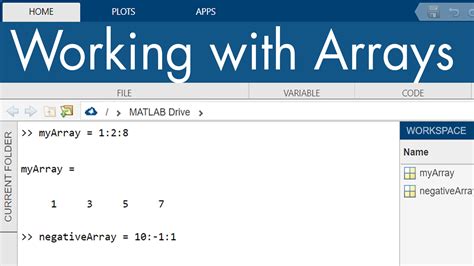
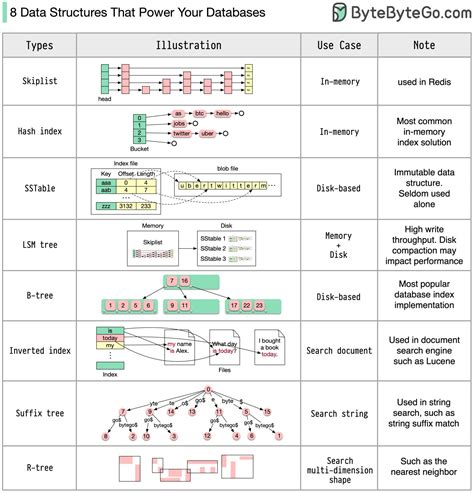
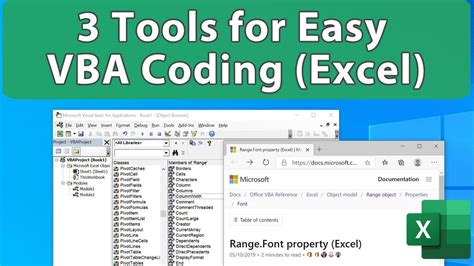
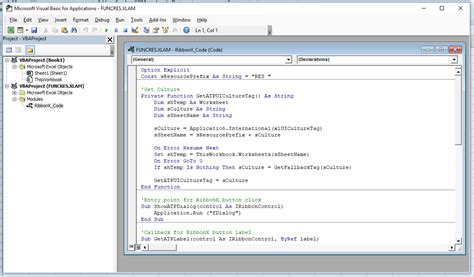
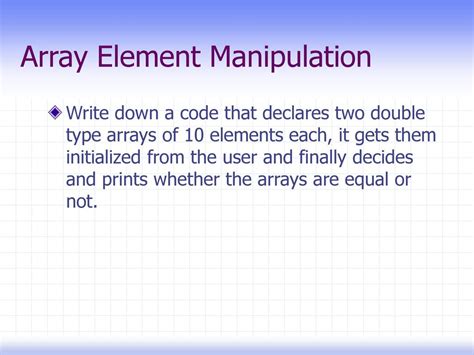
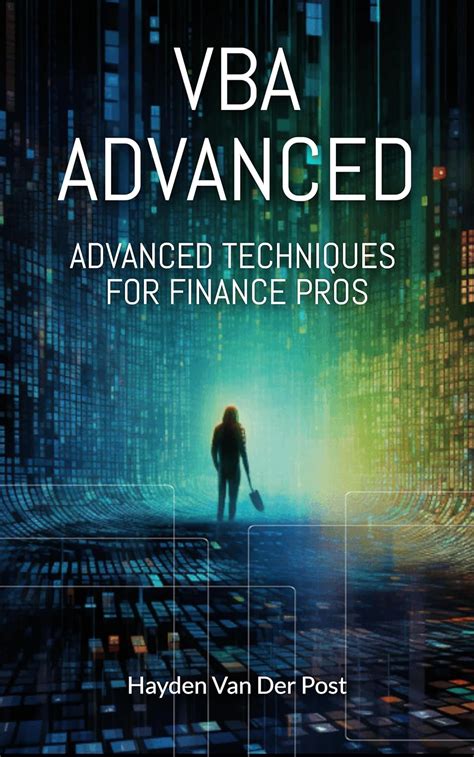
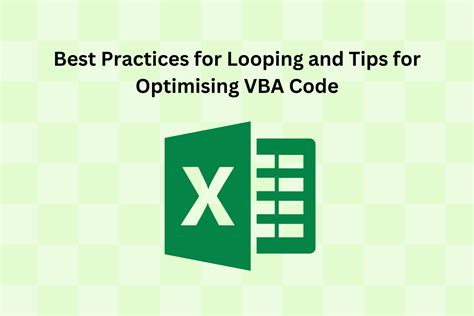
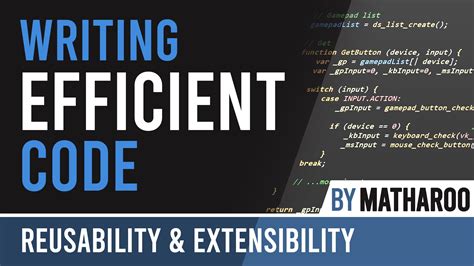
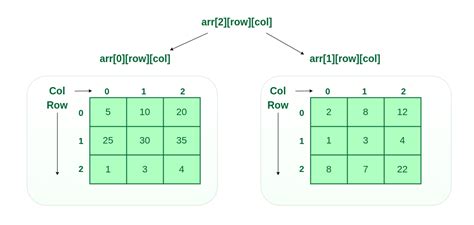
What is an array of arrays in VBA?
+An array of arrays in VBA is a data structure that allows you to store and manipulate multiple arrays within a single variable.
How do you declare an array of arrays in VBA?
+You declare a variable as an array of Variant type and then assign arrays to its elements.
What are the benefits of using arrays of arrays?
+Benefits include efficient data storage, improved code readability, and simplified data manipulation.
To further explore the capabilities of arrays of arrays in VBA, consider experimenting with different scenarios and applications. By mastering this data structure, you can significantly enhance your programming skills and tackle more complex projects with confidence. Whether you're working on data analysis, game development, or any other field that involves complex data manipulation, arrays of arrays can be a powerful tool in your arsenal.