Intro
Learn to copy files efficiently using VBA with our expert guide, covering file transfer, scripting, and automation techniques, including error handling and file management best practices.
The ability to copy files using VBA (Visual Basic for Applications) is a powerful tool for automating tasks in Microsoft Office applications, such as Excel, Word, and Access. This functionality can be particularly useful for tasks like data management, report generation, and file organization. In this article, we'll delve into the details of how to copy a file using VBA, exploring the benefits, the working mechanisms, and providing step-by-step guides and examples.
Copying files is an essential operation in many workflows, especially when dealing with large datasets, document management, or automated reporting. VBA, with its extensive library of commands and functions, makes it relatively straightforward to perform such tasks. The primary benefits of using VBA for file operations include increased efficiency, reduced manual labor, and the ability to integrate file copying with other automated tasks.
Introduction to VBA File Copying
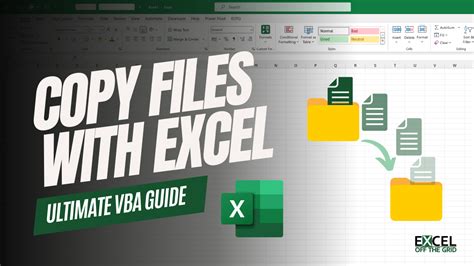
To copy a file using VBA, you'll typically use the FileSystemObject
from the Microsoft Scripting Runtime library. This object provides methods for file and folder manipulation, including copying. The process involves setting up the FileSystemObject
, specifying the source and destination files, and then executing the copy command.
Setting Up the Environment
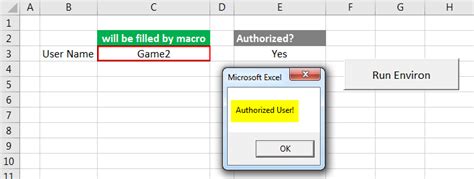
Before you can start copying files, you need to ensure that the Microsoft Scripting Runtime library is referenced in your VBA project. This can be done by following these steps:
- Open the Visual Basic Editor in your Microsoft Office application.
- In the Visual Basic Editor, click
Tools
>References
. - In the References dialog box, check if "Microsoft Scripting Runtime" is listed. If not, check the box next to it to add the reference.
- Click
OK
to close the dialog box.
Copying a File with VBA
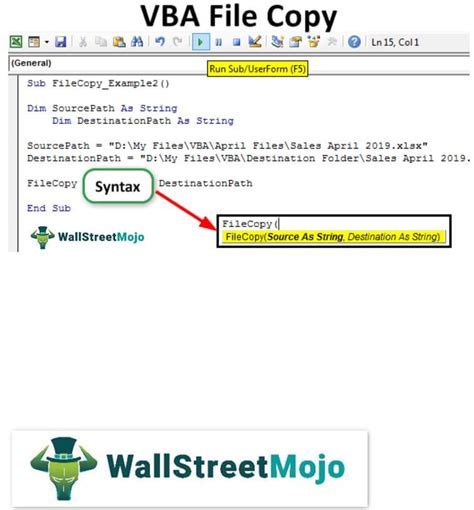
Here's a basic example of how to copy a file using VBA:
Sub CopyFile()
Dim fso As FileSystemObject
Dim sourceFile As String
Dim destinationFile As String
' Initialize the FileSystemObject
Set fso = New FileSystemObject
' Specify the source and destination files
sourceFile = "C:\Source\example.txt"
destinationFile = "D:\Destination\example.txt"
' Copy the file
fso.CopyFile sourceFile, destinationFile
' Clean up
Set fso = Nothing
End Sub
This example demonstrates the core steps involved in copying a file: setting up the FileSystemObject
, defining the source and destination paths, and executing the CopyFile
method.
Handling Errors and Exceptions
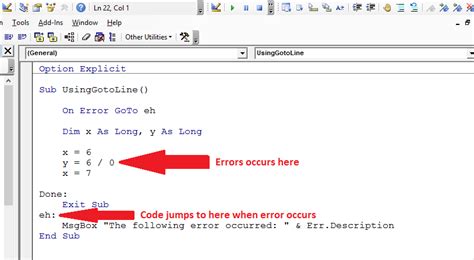
When working with file operations, it's crucial to handle potential errors, such as permissions issues, file not found errors, or insufficient disk space. VBA provides an On Error
statement that can be used to catch and handle exceptions. For example:
Sub CopyFileWithErrorHandling()
Dim fso As FileSystemObject
Dim sourceFile As String
Dim destinationFile As String
On Error GoTo ErrorHandler
' Initialize the FileSystemObject
Set fso = New FileSystemObject
' Specify the source and destination files
sourceFile = "C:\Source\example.txt"
destinationFile = "D:\Destination\example.txt"
' Copy the file
fso.CopyFile sourceFile, destinationFile
' Clean up
Set fso = Nothing
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
This modified version of the subroutine includes basic error handling, displaying a message box with the error description if an exception occurs.
Best Practices for File Copying with VBA
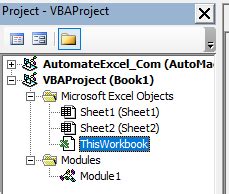
When using VBA for file copying, consider the following best practices:
- Validate File Paths: Before attempting to copy a file, ensure that both the source and destination paths are valid and exist.
- Handle Permissions: Be aware of file and folder permissions, especially when working in a network environment or with system files.
- Test Thoroughly: Always test your VBA scripts in a safe environment before running them on critical data.
- Use Relative Paths: When possible, use relative paths to make your scripts more portable and less dependent on specific drive configurations.
Advanced File Copying Techniques
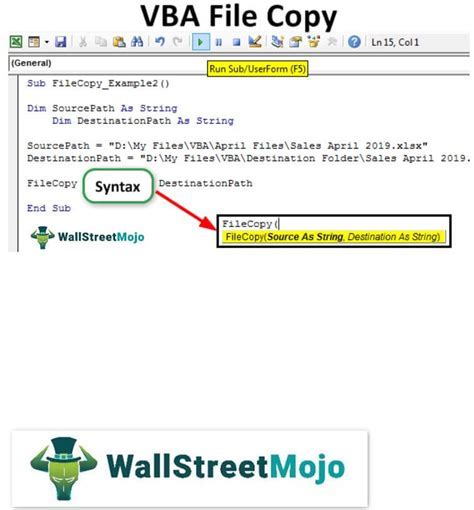
Beyond simple file copying, VBA can be used for more complex file management tasks, such as:
- Copying Multiple Files: Using loops to copy multiple files based on specific criteria.
- File Backup: Creating automated backup routines to ensure data safety.
- Folder Synchronization: Keeping two folders synchronized by copying files from one to another based on certain conditions.
Gallery of VBA File Copying Examples
VBA File Copying Image Gallery
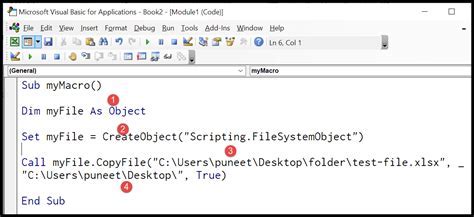

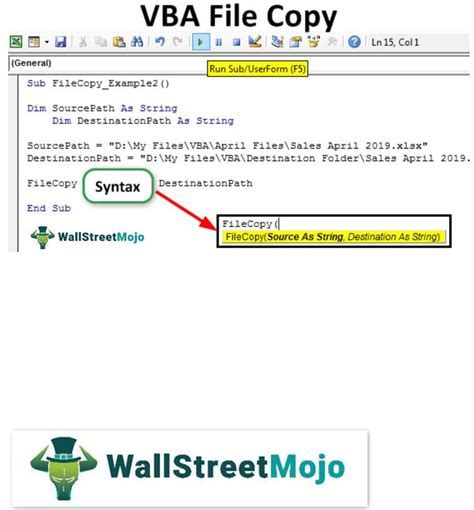
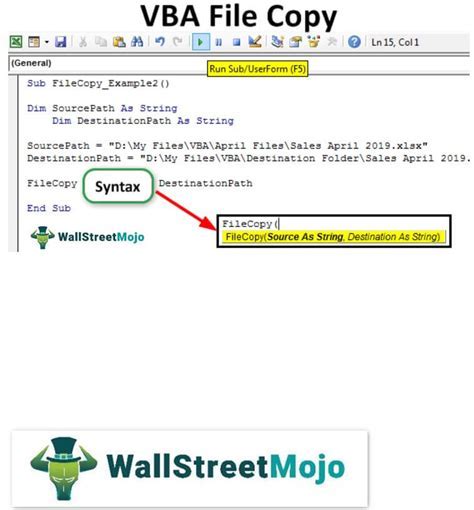
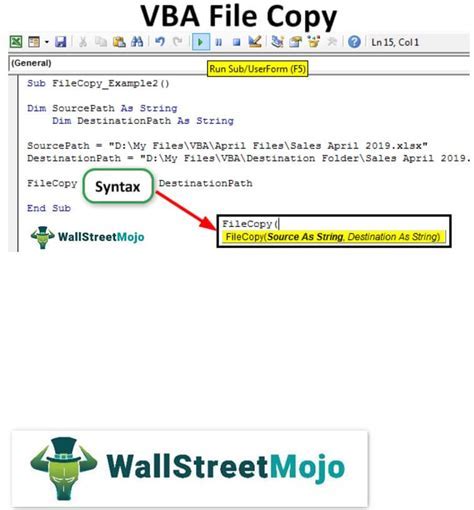
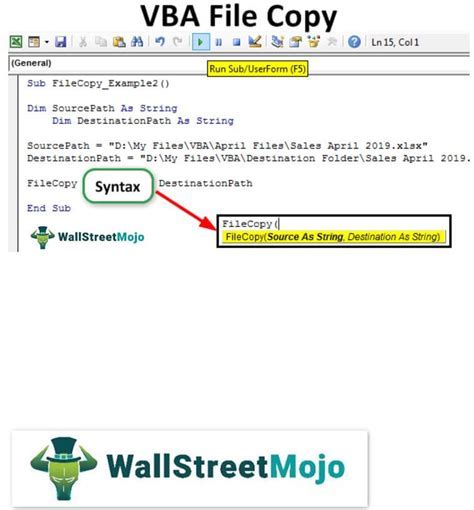
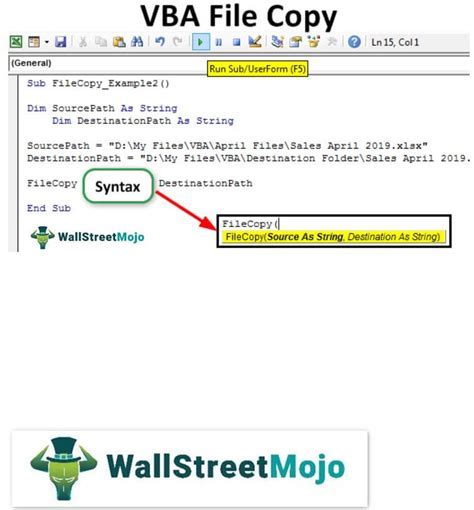
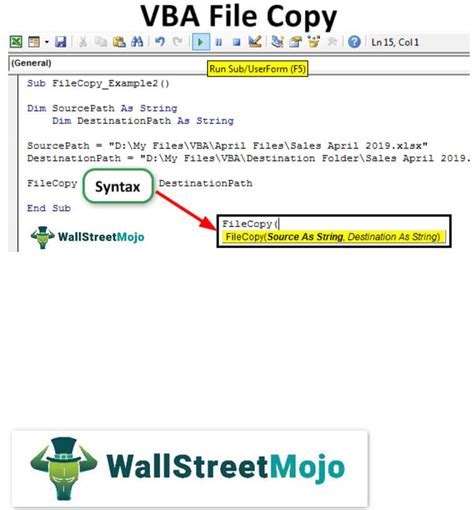
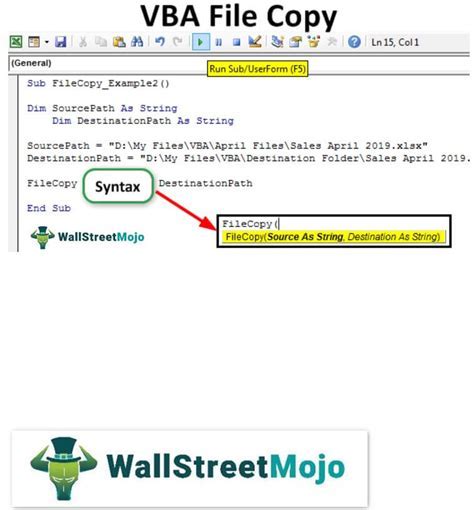
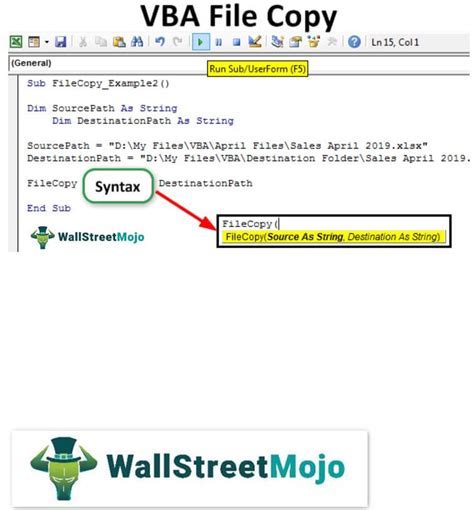
What is the purpose of using VBA for file copying?
+The purpose of using VBA for file copying is to automate file management tasks, increasing efficiency and reducing manual labor. It's particularly useful for repetitive tasks or when integrating file copying with other automated processes.
How do I handle errors when copying files with VBA?
+To handle errors, use the `On Error` statement to catch exceptions and provide appropriate error handling, such as displaying an error message or logging the error for later review.
Can I copy multiple files at once using VBA?
+In conclusion, copying files with VBA is a versatile and powerful technique for automating file management tasks within Microsoft Office applications. By understanding the basics of file copying, handling errors, and exploring advanced techniques, you can streamline your workflow, reduce manual effort, and increase productivity. Whether you're working with Excel, Word, or another Office application, mastering VBA file copying can significantly enhance your ability to manage and manipulate files efficiently. We invite you to share your experiences, ask questions, or explore more topics related to VBA and file management by commenting below or sharing this article with your network.