Intro
Converting strings to integers is a common task in programming, including in Visual Basic for Applications (VBA). This process is essential when you need to perform numerical operations on data that is initially stored as text. VBA provides several functions to achieve this conversion, each with its own set of characteristics and uses. Understanding these functions and their applications is crucial for effective data manipulation in VBA.
The importance of converting strings to integers cannot be overstated. In many scenarios, data is imported from external sources or user inputs in the form of strings. Without converting these strings into numerical values, you cannot perform calculations or comparisons that require integer data types. Moreover, ensuring that your data is in the correct format is fundamental for avoiding errors in your VBA applications.
To entice readers to continue reading, let's consider a practical example. Suppose you are managing a small business and need to calculate the total cost of items based on their quantities and prices, which are initially stored as strings in an Excel spreadsheet. Without converting these strings to integers or doubles, you wouldn't be able to perform the necessary calculations. This scenario highlights the necessity of understanding how to convert strings to integers in VBA effectively.
Introduction to VBA Conversion Functions
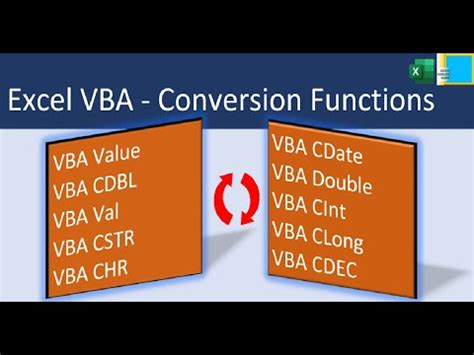
VBA offers several functions for converting strings to integers, including CInt()
, CLng()
, Val()
, and Int()
. Each of these functions serves a slightly different purpose and has its own limitations and potential pitfalls.
CInt() Function
The `CInt()` function is one of the most commonly used functions for converting strings to integers in VBA. It converts a string to a short integer, which can range from -32,768 to 32,767. If the string cannot be converted to an integer (for example, if it contains non-numerical characters), `CInt()` will raise a runtime error.CLng() Function
The `CLng()` function converts a string to a long integer, which has a wider range than the short integer converted by `CInt()`. Long integers can range from -2,147,483,648 to 2,147,483,647. Like `CInt()`, `CLng()` will also raise an error if the conversion fails.Val() Function
The `Val()` function is more versatile than `CInt()` or `CLng()` because it can convert a string to a numerical value, which can then be assigned to any numeric data type (including integers, doubles, etc.). `Val()` stops reading the string as soon as it encounters a non-numerical character. If the string starts with a non-numerical character, `Val()` returns 0.Int() Function
The `Int()` function returns the integer part of a number. While it can be used to convert a string to an integer by first converting the string to a number, it's less commonly used for direct string-to-integer conversions compared to `CInt()` or `CLng()`.Using Conversion Functions in VBA
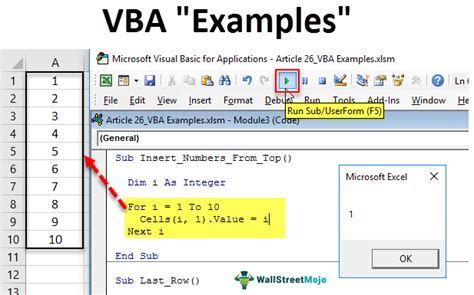
To effectively use these conversion functions, you should understand their syntax and how they handle different types of input. Here are some key points to consider:
- Error Handling: Always consider the potential for errors when converting strings to integers. Use
On Error
statements or check the string's content before attempting a conversion to handle situations where the conversion might fail. - Data Validation: Validate the data before conversion to ensure it can be successfully converted to an integer. This might involve checking for non-numerical characters or ensuring the string represents a number within the range of the target integer type.
- Choosing the Right Function: Select the conversion function based on the specific requirements of your application. For example, use
CLng()
for larger numbers andCInt()
for smaller integers.
Practical Examples
Here are some practical examples of how to use these functions in VBA:
Sub ConvertStringToInt()
Dim myString As String
myString = "123"
' Using CInt()
Dim myInt As Integer
myInt = CInt(myString)
MsgBox myInt
' Using CLng()
Dim myLong As Long
myLong = CLng(myString)
MsgBox myLong
' Using Val()
Dim myVal As Double
myVal = Val(myString)
MsgBox myVal
End Sub
Best Practices for String to Integer Conversion
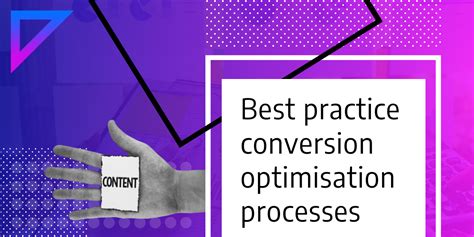
When converting strings to integers in VBA, it's essential to follow best practices to ensure your code is robust, reliable, and easy to maintain. Here are some guidelines:
- Validate User Input: Always validate user input to prevent errors. Check if the input string contains only numerical characters before attempting a conversion.
- Use Error Handling: Implement error handling mechanisms to gracefully handle situations where the conversion fails.
- Choose the Correct Data Type: Select the appropriate integer data type (
Integer
,Long
, etc.) based on the range of values you expect to work with. - Comment Your Code: Clearly comment your code to explain why a particular conversion function is used and how errors are handled.
Common Pitfalls and Troubleshooting
Converting strings to integers can sometimes lead to unexpected issues, especially if the input data is not as expected. Common pitfalls include:
- Non-Numerical Characters: The presence of non-numerical characters in the string can cause conversion errors.
- Out-of-Range Values: Attempting to convert a string that represents a number outside the range of the target integer type will result in an error.
- Null or Empty Strings: Trying to convert null or empty strings can lead to errors or unexpected results.
To troubleshoot these issues, carefully examine the input data, validate user input, and implement robust error handling mechanisms.
Gallery of VBA Conversion Examples
VBA Conversion Examples Gallery
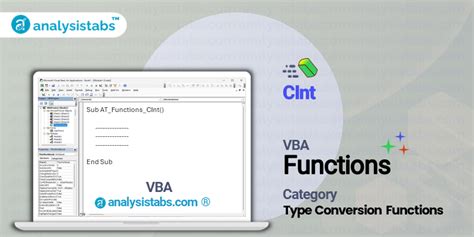
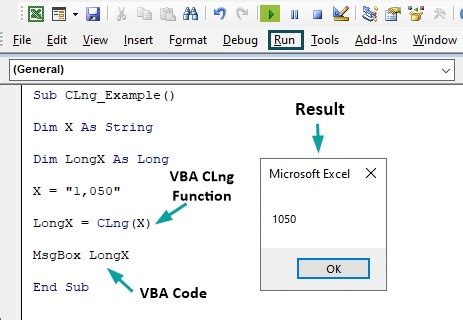
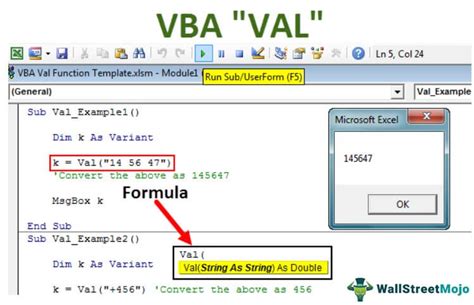
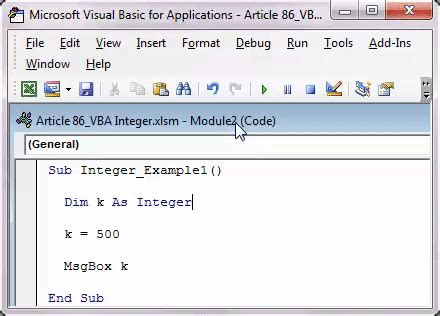
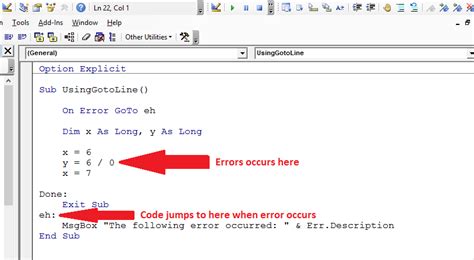
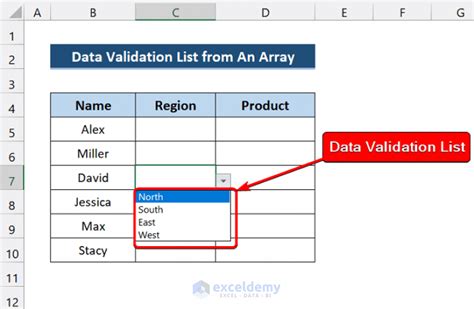
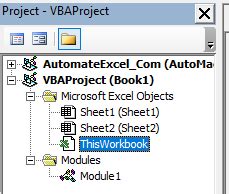
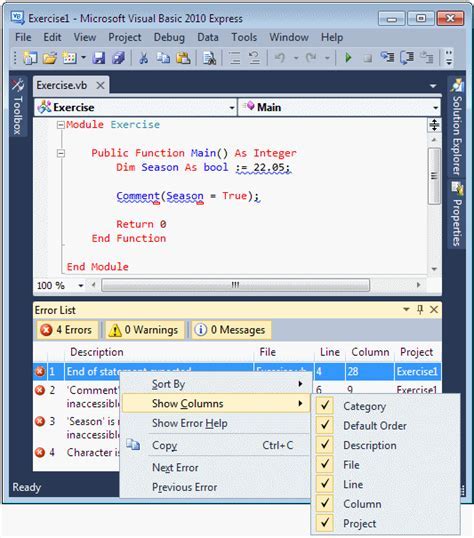
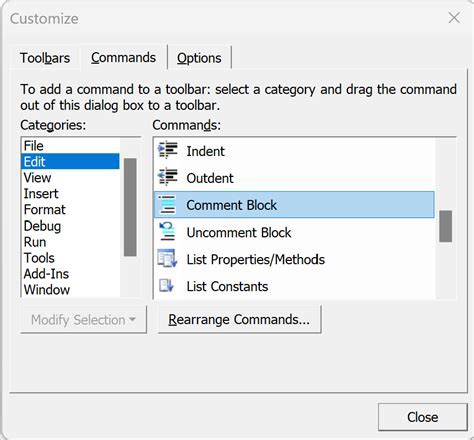
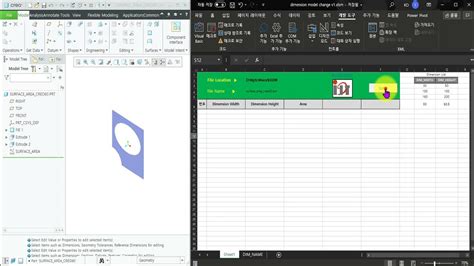
Frequently Asked Questions
What is the difference between CInt() and CLng() in VBA?
+CInt() converts a string to a short integer, while CLng() converts a string to a long integer. The main difference lies in the range of values they can handle, with CLng() supporting larger numbers.
How do I handle errors when converting strings to integers in VBA?
+You can handle errors by using On Error statements or by checking the input string for non-numerical characters before attempting the conversion. It's also a good practice to validate user input to prevent errors.
What is the purpose of the Val() function in VBA?
+The Val() function converts a string to a numerical value. It stops reading the string as soon as it encounters a non-numerical character, making it useful for converting strings that may contain a mix of numerical and non-numerical characters.
In conclusion, converting strings to integers is a fundamental aspect of programming in VBA, and understanding the various functions available, such as CInt()
, CLng()
, Val()
, and Int()
, is crucial for effective data manipulation. By following best practices, including data validation and error handling, you can ensure that your VBA applications are robust and reliable. Whether you're working with user input, imported data, or internal calculations, mastering string-to-integer conversions will significantly enhance your ability to create powerful and efficient VBA solutions. We invite you to share your experiences, ask questions, or provide feedback on this topic, and we look forward to continuing the conversation on VBA programming and its applications.