Intro
Learn 5 ways to create folders using VBA, including automation, scripting, and Excel integration, to streamline file management and organization with Visual Basic for Applications.
Creating folders using VBA (Visual Basic for Applications) is a useful skill for automating tasks in Microsoft Office applications, particularly in Excel. Folders are essential for organizing files, and being able to create them programmatically can save time and effort. Here are five ways to create a folder using VBA, each with its unique approach and application.
The ability to create folders programmatically is essential for managing and organizing files efficiently. Whether you're working on a project that involves generating reports, processing data, or automating workflows, being able to dynamically create folders can streamline your processes. VBA provides several methods to achieve this, catering to different scenarios and requirements.
Firstly, understanding the basics of VBA is crucial. VBA is a programming language developed by Microsoft, and it's used for creating and automating tasks in Microsoft Office applications. It allows users to interact with the application's interface, manipulate data, and even interact with the operating system to perform tasks such as creating folders.
To start creating folders with VBA, you'll typically need to open the Visual Basic Editor in your Microsoft Office application. In Excel, for example, you can do this by pressing Alt + F11
or by navigating to the Developer tab and clicking on Visual Basic. Once in the Visual Basic Editor, you can insert a new module to write your VBA code.
Using the MkDir Function
The MkDir
function is one of the simplest ways to create a folder using VBA. This function creates a new directory (folder) with the specified path.
Sub CreateFolderUsingMkDir()
Dim folderPath As String
folderPath = "C:\Users\YourUsername\Documents\NewFolder"
MkDir folderPath
End Sub
Using the FileSystemObject
The FileSystemObject
is a more versatile approach, offering more control over file system operations, including creating folders.
Sub CreateFolderUsingFSO()
Dim fso As Object
Dim folderPath As String
folderPath = "C:\Users\YourUsername\Documents\NewFolder"
Set fso = CreateObject("Scripting.FileSystemObject")
If Not fso.FolderExists(folderPath) Then
fso.CreateFolder folderPath
End If
Set fso = Nothing
End Sub
Using the Shell Object
The Shell
object provides another method to interact with the file system, including creating folders.
Sub CreateFolderUsingShell()
Dim shellObj As Object
Dim folderPath As String
folderPath = "C:\Users\YourUsername\Documents\NewFolder"
Set shellObj = CreateObject("WScript.Shell")
shellObj.Run "cmd /c mkdir """ & folderPath & """", 0, True
Set shellObj = Nothing
End Sub
Using the Dir Function with MkDir
This method checks if a folder exists using the Dir
function and creates it if it doesn't.
Sub CreateFolderUsingDir()
Dim folderPath As String
folderPath = "C:\Users\YourUsername\Documents\NewFolder"
If Dir(folderPath, vbDirectory) = "" Then MkDir folderPath
End Sub
Handling Errors
When creating folders, it's essential to handle potential errors, such as insufficient permissions or the folder already existing.
Sub CreateFolderWithErrorHandling()
Dim fso As Object
Dim folderPath As String
folderPath = "C:\Users\YourUsername\Documents\NewFolder"
On Error Resume Next
Set fso = CreateObject("Scripting.FileSystemObject")
fso.CreateFolder folderPath
If Err.Number <> 0 Then
MsgBox "Error creating folder: " & Err.Description
Else
MsgBox "Folder created successfully."
End If
Set fso = Nothing
On Error GoTo 0
End Sub
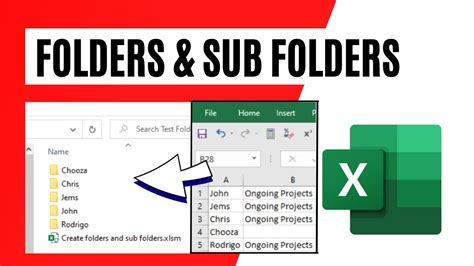
Practical Applications
- Automating Report Generation: Creating folders dynamically can be useful when generating reports. For instance, you can create a new folder for each month or quarter to organize your reports better.
- Data Processing: When processing data, you might need to create folders for different types of data or for different stages of the processing pipeline.
- Workflow Automation: In workflow automation, creating folders can be part of setting up a new project or task, helping to keep files organized from the start.
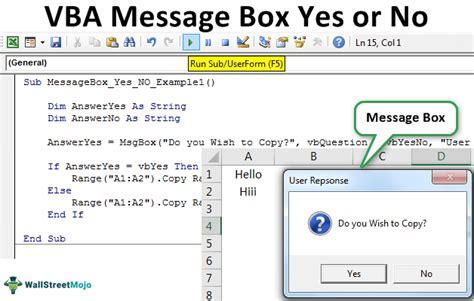
Best Practices
- Error Handling: Always include error handling in your code to gracefully manage unexpected situations.
- Path Validation: Validate the folder path before attempting to create a folder to avoid errors.
- Permissions: Be aware of the permissions required to create folders in different locations.
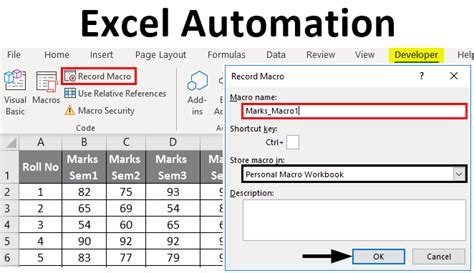
Gallery of VBA Folder Creation
VBA Folder Creation Gallery
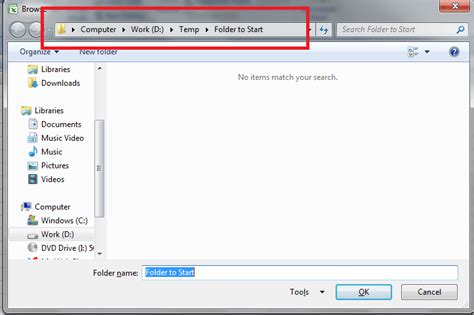
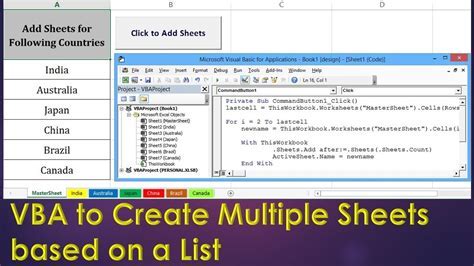
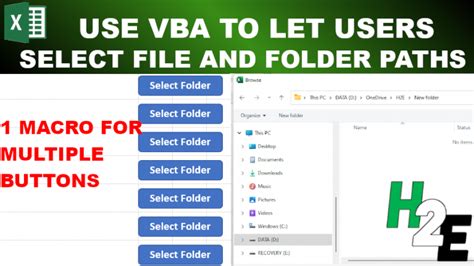
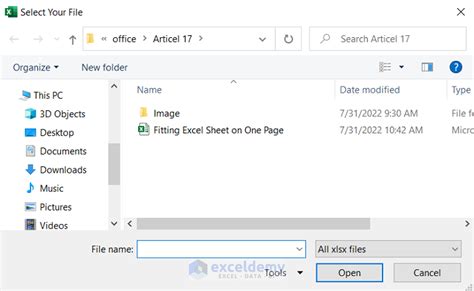
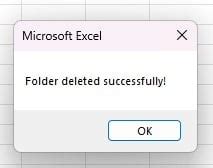
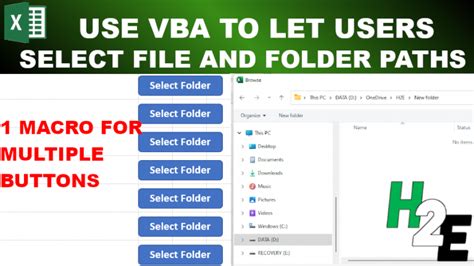
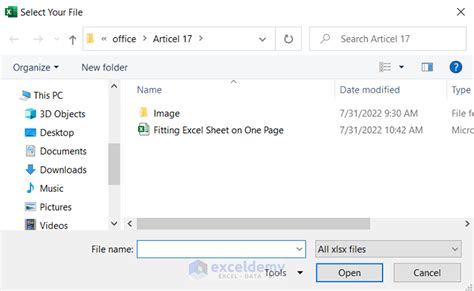
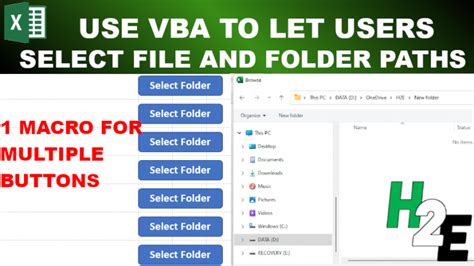
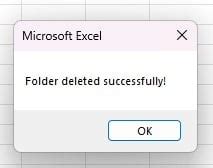

FAQs
What is VBA used for?
+VBA is used for creating and automating tasks in Microsoft Office applications.
How do I open the Visual Basic Editor in Excel?
+You can open the Visual Basic Editor by pressing `Alt + F11` or by navigating to the Developer tab and clicking on Visual Basic.
What is the `MkDir` function used for?
+The `MkDir` function is used to create a new directory (folder).
Creating folders with VBA is a powerful tool for automating tasks and organizing files. By mastering the different methods and best practices outlined above, you can enhance your productivity and workflow efficiency. Whether you're working with Excel, Word, or another Office application, the ability to dynamically create folders can be a game-changer for managing your digital workspace.
If you have any questions or need further clarification on any of the points discussed, please don't hesitate to ask. Share your experiences or tips on using VBA for folder creation in the comments below. Your insights could help others looking to leverage VBA for their file management needs.