Intro
In today's digital age, managing and manipulating date and time data is a crucial aspect of various applications, including databases, software development, and data analysis. One common requirement is to merge date and time into a single entity, which can be useful for storing, processing, and displaying temporal information. This article will delve into the importance of merging date and time, exploring five ways to achieve this, along with practical examples and code snippets.
Merging date and time is essential in many scenarios, such as scheduling appointments, logging events, or tracking time-series data. By combining date and time, you can create a unified timestamp that accurately represents a specific moment in time. This, in turn, enables efficient data processing, sorting, and filtering. Moreover, a merged date and time format can enhance user experience by providing a clear and concise representation of temporal information.
The need to merge date and time arises in various domains, including business, healthcare, finance, and transportation. For instance, in e-commerce, merging date and time is necessary for tracking order placements, shipping, and delivery. In healthcare, accurate timestamping is critical for medical records, appointments, and medication administration. As data-driven decision-making continues to grow, the ability to effectively merge and manipulate date and time data becomes increasingly important.
Understanding Date and Time Formats
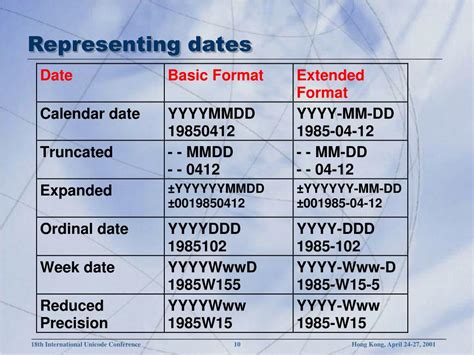
Before exploring the ways to merge date and time, it's essential to understand the various formats and standards used to represent temporal information. The most common date formats include YYYY-MM-DD, MM/DD/YYYY, and DD/MM/YYYY, while time formats include HH:MM:SS, HH:MM, and 12-hour clocks with AM/PM designations. Additionally, time zones and daylight saving time (DST) rules must be considered to ensure accurate and consistent timestamping.
Merging Date and Time using String Concatenation
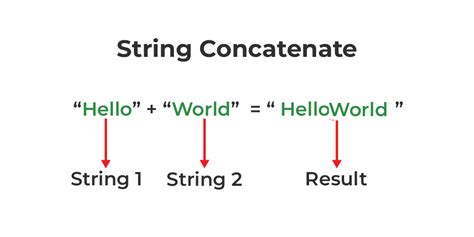
One simple way to merge date and time is by using string concatenation. This method involves combining the date and time strings using a separator, such as a space or a hyphen. For example, you can concatenate the date "2022-07-25" and time "14:30:00" to create the merged timestamp "2022-07-25 14:30:00". This approach is straightforward but may lead to inconsistencies if the date and time formats are not standardized.
Example Code: String Concatenation in Python
```python date = "2022-07-25" time = "14:30:00" merged_timestamp = date + " " + time print(merged_timestamp) # Output: 2022-07-25 14:30:00 ```Merging Date and Time using DateTime Objects
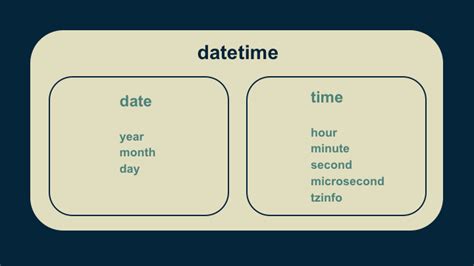
Another approach to merging date and time is by using DateTime objects, which provide a structured way to represent and manipulate temporal information. In programming languages like Python, Java, and C#, you can create DateTime objects from date and time strings and then combine them using methods or operators. For instance, you can use the datetime
module in Python to create a DateTime object from a date string and a time string, and then merge them using the combine
method.
Example Code: DateTime Objects in Python
```python from datetime import datetime, date, time date_obj = date(2022, 7, 25) time_obj = time(14, 30, 0) merged_timestamp = datetime.combine(date_obj, time_obj) print(merged_timestamp) # Output: 2022-07-25 14:30:00 ```Merging Date and Time using Time Zone-Aware Libraries

When working with date and time data, it's crucial to consider time zones and DST rules to ensure accurate and consistent timestamping. Time zone-aware libraries like pytz in Python or Joda-Time in Java provide a robust way to merge date and time while accounting for time zone differences. These libraries offer features like time zone conversion, DST handling, and timestamp normalization, making it easier to work with temporal data across different regions.
Example Code: Time Zone-Aware Library in Python
```python from datetime import datetime import pytz date = "2022-07-25" time = "14:30:00" timezone = pytz.timezone("America/New_York") merged_timestamp = timezone.localize(datetime.strptime(date + " " + time, "%Y-%m-%d %H:%M:%S")) print(merged_timestamp) # Output: 2022-07-25 14:30:00-04:00 ```Merging Date and Time using SQL
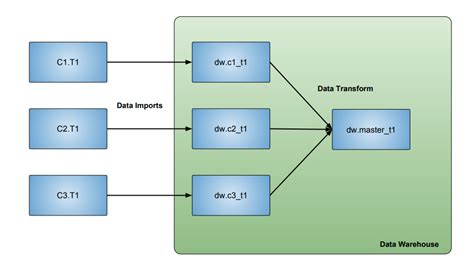
In database management systems like MySQL, PostgreSQL, or SQL Server, you can merge date and time using SQL functions and operators. For example, you can use the CONCAT
function to concatenate date and time columns, or the TIMESTAMP
function to create a timestamp from separate date and time columns. Additionally, SQL provides various date and time functions like DATE_FORMAT
, TIME_FORMAT
, and TIMESTAMPDIFF
to manipulate and format temporal data.
Example Code: Merging Date and Time in SQL
```sql SELECT CONCAT(date_column, " ", time_column) AS merged_timestamp FROM your_table; ```Merging Date and Time using Excel Formulas
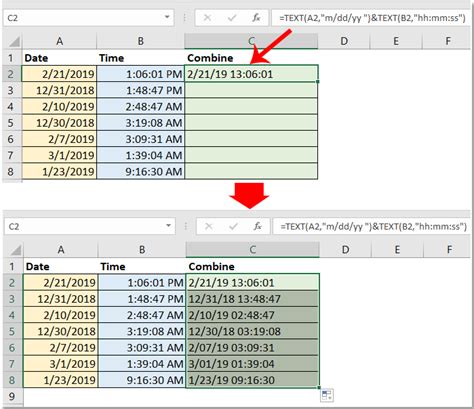
In Microsoft Excel, you can merge date and time using formulas like CONCATENATE
, TEXT
, and TIMEVALUE
. For instance, you can use the CONCATENATE
function to combine date and time cells, or the TEXT
function to format the merged timestamp as a string. Additionally, Excel provides various date and time functions like DATE
, TIME
, and NOW
to manipulate and format temporal data.
Example Code: Merging Date and Time in Excel
```excel =CONCATENATE(A1, " ", B1) ```DateTime Merge Image Gallery
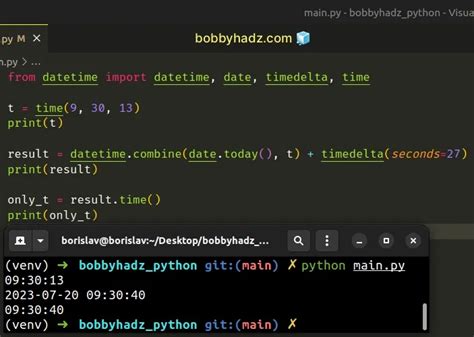

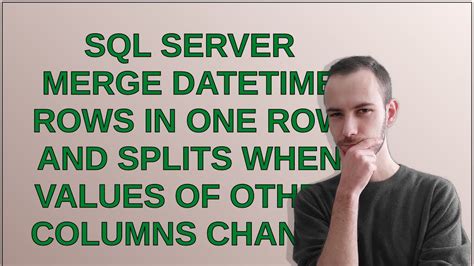
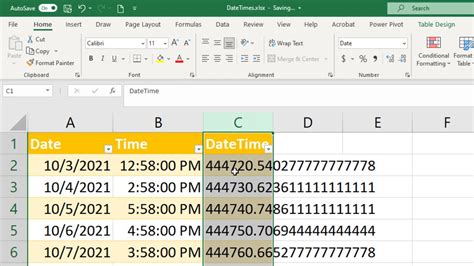
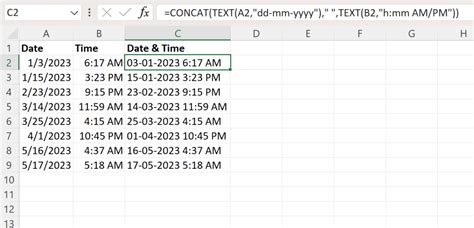
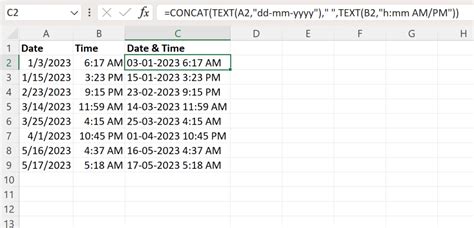
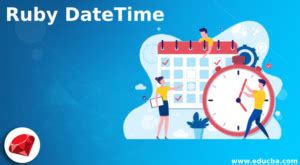

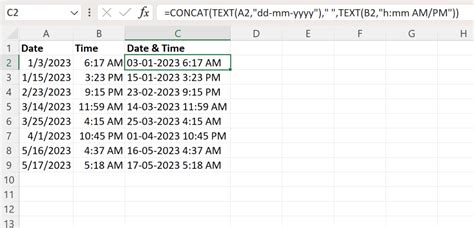
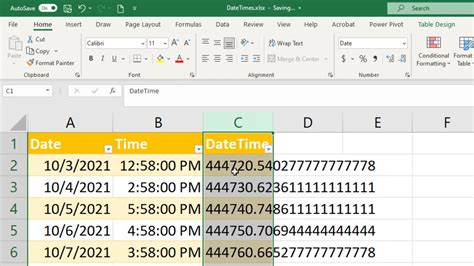
What is the importance of merging date and time?
+Merging date and time is crucial for accurate and consistent timestamping, which is essential in various domains like business, healthcare, finance, and transportation.
What are the common date and time formats?
+The most common date formats include YYYY-MM-DD, MM/DD/YYYY, and DD/MM/YYYY, while time formats include HH:MM:SS, HH:MM, and 12-hour clocks with AM/PM designations.
How can I merge date and time in Python?
+You can merge date and time in Python using string concatenation, DateTime objects, or time zone-aware libraries like pytz.
What is the difference between DateTime objects and string concatenation?
+DateTime objects provide a structured way to represent and manipulate temporal information, while string concatenation is a simpler approach that may lead to inconsistencies if the date and time formats are not standardized.
Can I merge date and time in SQL?
+Yes, you can merge date and time in SQL using functions like CONCAT, TIMESTAMP, and DATE_FORMAT.
In conclusion, merging date and time is a vital aspect of working with temporal data, and there are various ways to achieve this, including string concatenation, DateTime objects, time zone-aware libraries, SQL, and Excel formulas. By understanding the different approaches and formats, you can effectively merge date and time to create accurate and consistent timestamps, which is essential in many domains. We hope this article has provided you with valuable insights and practical examples to help you work with date and time data. If you have any further questions or would like to share your experiences, please don't hesitate to comment below. Additionally, feel free to share this article with others who may benefit from this information.