Intro
Optimize Excel VBA userforms on 64-bit systems with minimize and maximize functionality, using efficient coding techniques and API calls to enhance user experience and interface control.
The importance of userforms in Excel VBA cannot be overstated, as they provide a powerful means to interact with users and create custom interfaces for complex tasks. However, managing the size and state of these userforms, especially in 64-bit versions of Excel, can pose challenges due to differences in handling window states and sizes compared to 32-bit versions. Understanding how to minimize and maximize userforms in a 64-bit environment is crucial for developing applications that are both functional and user-friendly.
Excel VBA offers a range of tools and methods for controlling userforms, including properties and methods that allow developers to manipulate the size and state of these forms. However, the transition to 64-bit Excel introduces considerations related to pointer sizes and compatibility, which can affect how userforms are managed. Despite these challenges, with the right approaches and techniques, developers can create userforms that behave consistently and predictably across different Excel versions.
The ability to minimize and maximize userforms is not just a matter of aesthetics; it's also about usability. Users often need to switch between different parts of the Excel interface or even other applications, and being able to minimize a userform to the taskbar or maximize it to fill the screen can significantly enhance the user experience. Moreover, in applications where multiple userforms are used, managing their state becomes even more critical to prevent clutter and ensure that the most relevant information is always accessible.
Introduction to Userform Management in Excel VBA
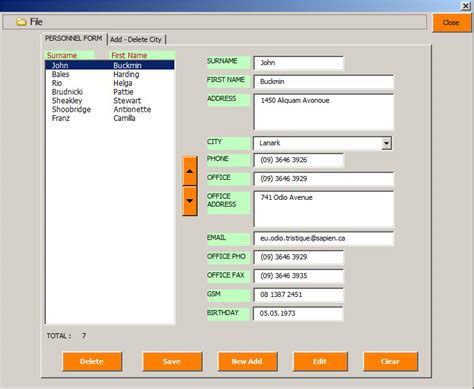
Managing userforms in Excel VBA involves understanding and utilizing various properties and methods. The Show
method is used to display a userform, while properties like Width
and Height
control its size. However, when it comes to minimizing and maximizing userforms, developers must delve into the Windows API, as Excel VBA does not provide built-in methods for these actions. This requires declaring API functions and using them to manipulate the userform's window state.
Utilizing Windows API for Userform Minimization and Maximization
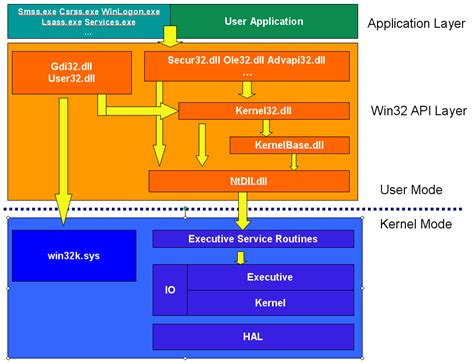
To minimize or maximize a userform, developers can use the ShowWindow
function from the Windows API. This function takes two parameters: the handle of the window to be shown and a parameter specifying how the window should be shown. The window handle can be obtained using the hwnd
property of the userform, and the show parameter can be set to minimize or maximize the window. This approach provides a flexible way to control the state of userforms, allowing for more sophisticated user interface designs.
Implementing Minimization and Maximization in 64-Bit Excel
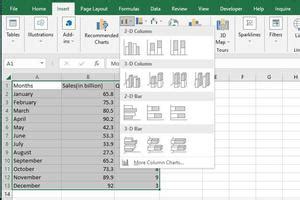
In 64-bit Excel, the primary consideration when working with the Windows API is ensuring that the declarations of API functions are compatible with 64-bit pointers. This typically involves using LongPtr
instead of Long
for parameters that represent pointers or handles. By making these adjustments, developers can ensure that their code works seamlessly in both 32-bit and 64-bit versions of Excel, providing a consistent user experience across different environments.
Example Code for Minimizing and Maximizing Userforms
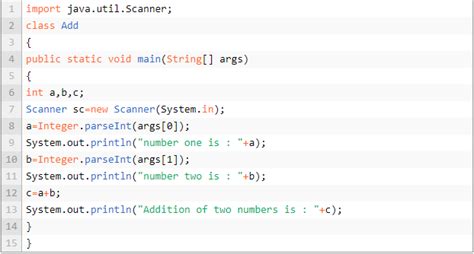
#If VBA7 Then
Private Declare PtrSafe Function ShowWindow Lib "user32" Alias "ShowWindow" (ByVal hwnd As LongPtr, ByVal nCmdShow As Long) As Long
#Else
Private Declare Function ShowWindow Lib "user32" Alias "ShowWindow" (ByVal hwnd As Long, ByVal nCmdShow As Long) As Long
#End If
Sub MinimizeUserform()
ShowWindow UserForm1.hwnd, SW_MINIMIZE
End Sub
Sub MaximizeUserform()
ShowWindow UserForm1.hwnd, SW_MAXIMIZE
End Sub
Best Practices for Userform Design and Management
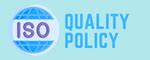
When designing and managing userforms, several best practices can enhance the user experience and ensure that the application behaves as expected. This includes keeping userforms simple and focused on a specific task, using clear and concise labeling, and providing intuitive navigation. Additionally, considering the impact of different screen resolutions and Excel versions on userform layout and behavior can help in creating applications that are robust and adaptable.
Common Challenges and Solutions
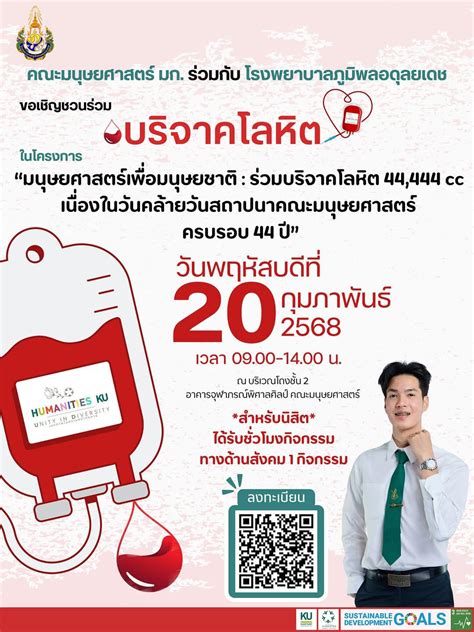
Developers often encounter challenges when working with userforms, such as managing their size and position, handling events, and ensuring compatibility across different Excel versions. Solutions to these challenges include using the Move
method to position userforms, leveraging event handlers to respond to user interactions, and thoroughly testing applications in various environments to identify and address compatibility issues.
Conclusion and Future Directions
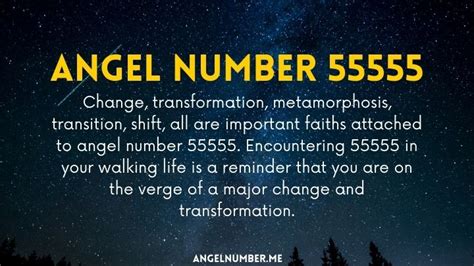
In conclusion, managing userforms in Excel VBA, particularly in 64-bit environments, requires a deep understanding of both Excel VBA and the Windows API. By mastering the techniques for minimizing and maximizing userforms, developers can create more engaging, flexible, and user-friendly applications. As Excel and VBA continue to evolve, staying abreast of new features and best practices will be crucial for creating applications that meet the changing needs of users.
Userform Management Gallery
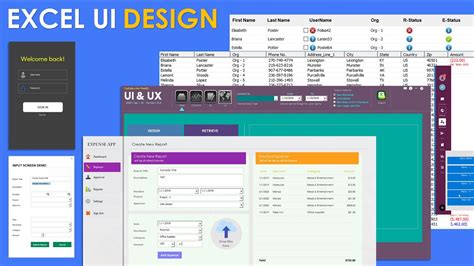
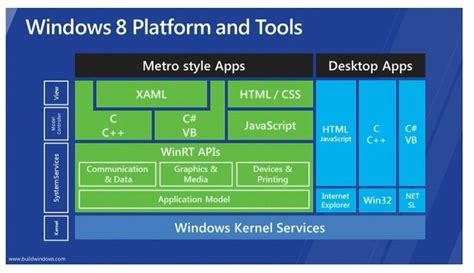
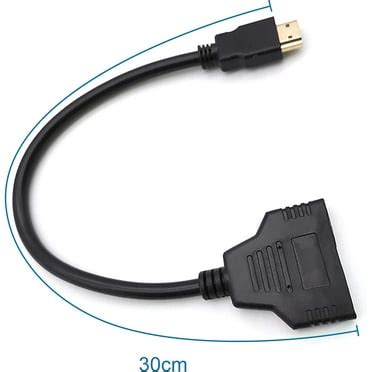
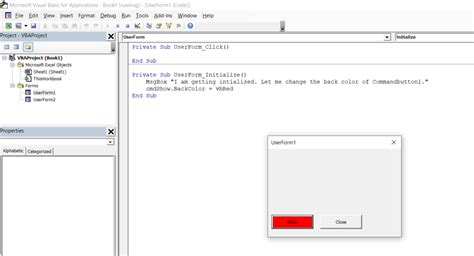
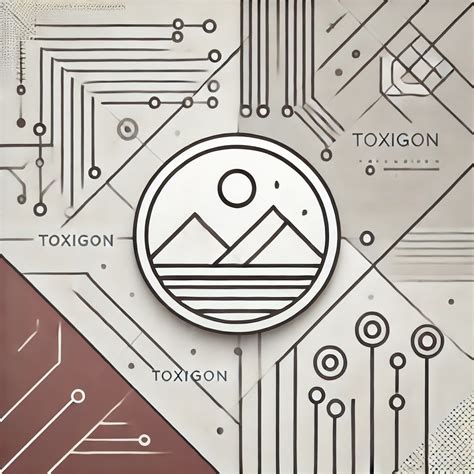
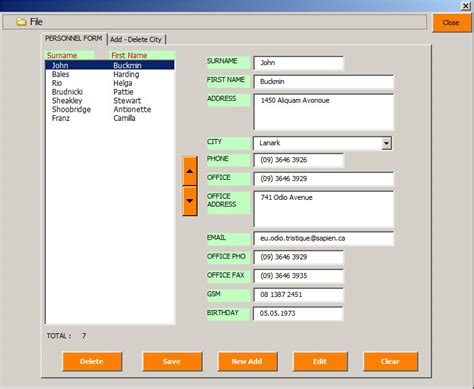
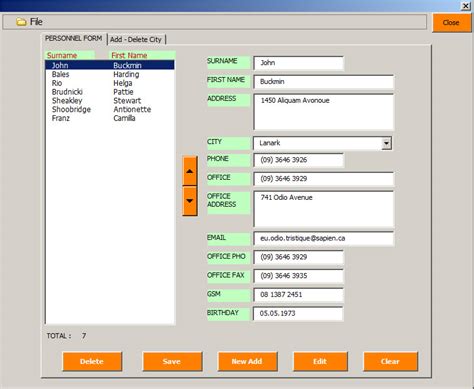
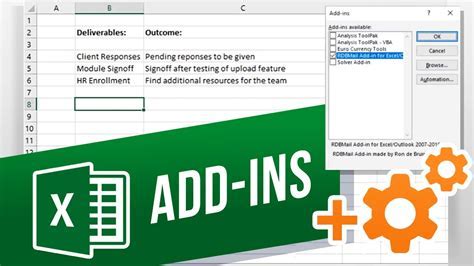
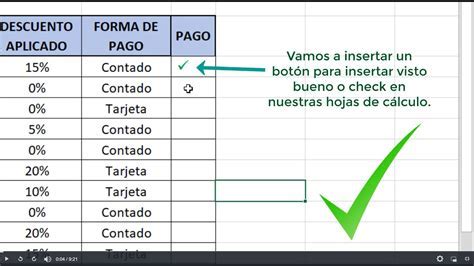

What is the purpose of minimizing and maximizing userforms in Excel VBA?
+The purpose is to enhance usability by allowing users to switch between different parts of the Excel interface or other applications efficiently.
How do you minimize a userform in Excel VBA using the Windows API?
+You can minimize a userform by using the `ShowWindow` API function with the `SW_MINIMIZE` parameter.
What considerations should be taken into account when working with userforms in 64-bit Excel?
+Ensure that API declarations are compatible with 64-bit pointers by using `LongPtr` instead of `Long` for handle parameters.
If you've found this article informative and helpful in your journey to master userform management in Excel VBA, especially in 64-bit environments, we invite you to share your thoughts, ask questions, or explore more topics related to Excel VBA and userform design. Your engagement and feedback are invaluable in creating a community that supports learning and growth in the field of Excel automation and development.