Intro
Excel VBA is a powerful tool that allows users to automate tasks and create custom solutions for their spreadsheets. One of the most common tasks that users need to perform is saving their workbooks. In this article, we will explore the different ways to save a workbook using Excel VBA.
Saving a workbook is an essential part of working with Excel. It ensures that all changes made to the workbook are preserved and can be retrieved later. Excel VBA provides several methods to save a workbook, including the Save method, the SaveAs method, and the SaveCopyAs method. Each of these methods has its own unique characteristics and uses.
To start with, let's look at the Save method. The Save method is used to save a workbook to its current file name and location. This method is useful when you want to save changes made to a workbook without changing its file name or location. The Save method can be used in the following way:
ActiveWorkbook.Save
This code will save the active workbook to its current file name and location.
SaveAs Method
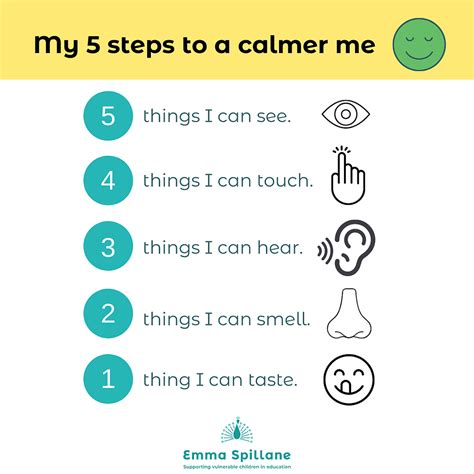
The SaveAs method is used to save a workbook to a new file name and location. This method is useful when you want to save a workbook with a different file name or location. The SaveAs method can be used in the following way:
ActiveWorkbook.SaveAs "C:\Users\Username\Desktop\NewWorkbook.xlsx"
This code will save the active workbook to a new file named "NewWorkbook.xlsx" located on the desktop.
SaveCopyAs Method
The SaveCopyAs method is used to save a copy of a workbook to a new file name and location. This method is useful when you want to save a copy of a workbook without changing the original workbook. The SaveCopyAs method can be used in the following way: ```vb ActiveWorkbook.SaveCopyAs "C:\Users\Username\Desktop\CopyOfWorkbook.xlsx" ``` This code will save a copy of the active workbook to a new file named "CopyOfWorkbook.xlsx" located on the desktop.File Formats
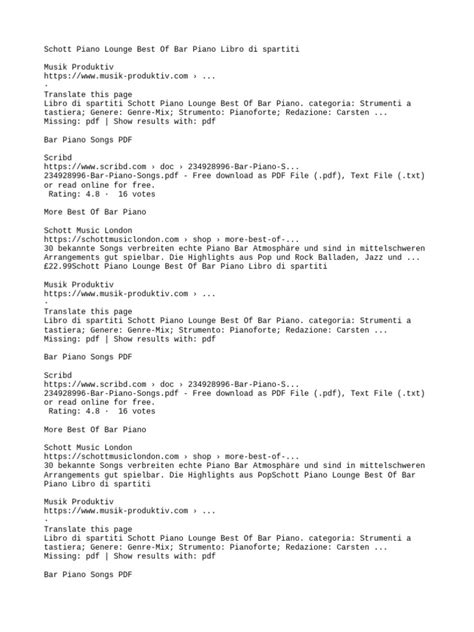
When saving a workbook, you can choose from a variety of file formats. The most common file formats are:
*.xlsx: This is the default file format for Excel 2007 and later versions. *.xls: This is the default file format for Excel 2003 and earlier versions. *.xlsm: This file format is used for macro-enabled workbooks. *.xlsb: This file format is used for binary workbooks.
You can specify the file format when using the SaveAs method by including the file extension in the file name. For example:
ActiveWorkbook.SaveAs "C:\Users\Username\Desktop\NewWorkbook.xlsx"
This code will save the active workbook to a new file named "NewWorkbook.xlsx" in the.xlsx file format.
File Path and Name
When saving a workbook, you need to specify the file path and name. The file path is the location where you want to save the workbook, and the file name is the name you want to give to the workbook. You can use the following code to get the current file path and name: ```vb Dim filePath As String Dim fileName As StringfilePath = ActiveWorkbook.Path fileName = ActiveWorkbook.Name
This code will get the current file path and name of the active workbook.
Workbook Properties
When saving a workbook, you can also specify workbook properties such as the author, title, and subject. You can use the following code to set workbook properties:
```vb
ActiveWorkbook.Author = "John Doe"
ActiveWorkbook.Title = "New Workbook"
ActiveWorkbook.Subject = "This is a new workbook"
This code will set the author, title, and subject of the active workbook.
Save Workbook with Password
You can also save a workbook with a password to protect it from unauthorized access. You can use the following code to save a workbook with a password: ```vb ActiveWorkbook.SaveAs "C:\Users\Username\Desktop\NewWorkbook.xlsx", Password:="password" ``` This code will save the active workbook to a new file named "NewWorkbook.xlsx" with a password.Best Practices
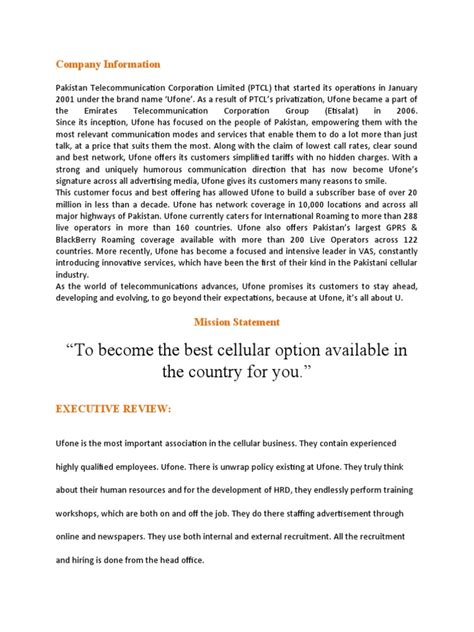
When saving a workbook using Excel VBA, it's essential to follow best practices to ensure that your code is efficient and effective. Here are some best practices to keep in mind:
- Always specify the file path and name when saving a workbook.
- Use the SaveAs method to save a workbook to a new file name and location.
- Use the SaveCopyAs method to save a copy of a workbook without changing the original workbook.
- Specify the file format when saving a workbook.
- Set workbook properties such as the author, title, and subject.
- Use a password to protect a workbook from unauthorized access.
Common Errors
When saving a workbook using Excel VBA, you may encounter common errors such as:- File not found error: This error occurs when the file path or name is incorrect.
- Permission denied error: This error occurs when you don't have permission to save the workbook to the specified location.
- File already exists error: This error occurs when a file with the same name already exists in the specified location.
You can use error handling to handle these errors and ensure that your code runs smoothly.
Gallery of Excel VBA Save Workbook
Excel VBA Save Workbook Image Gallery
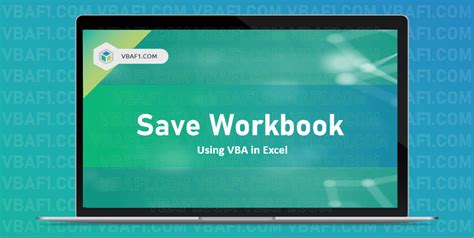
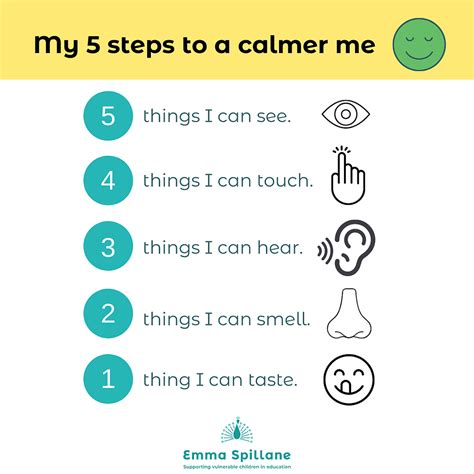
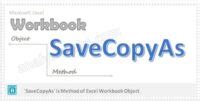
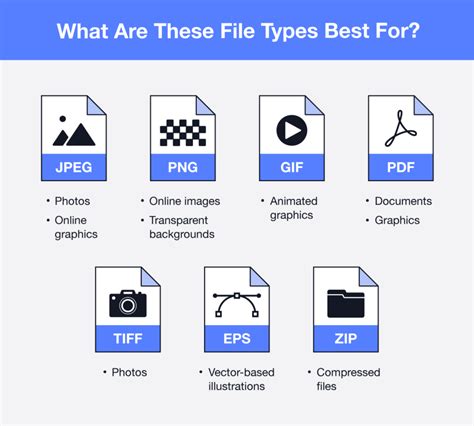
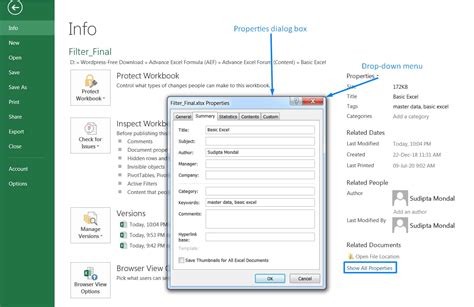
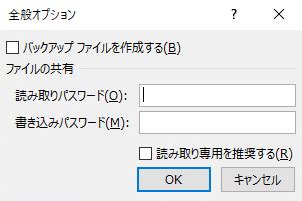
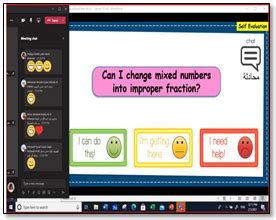
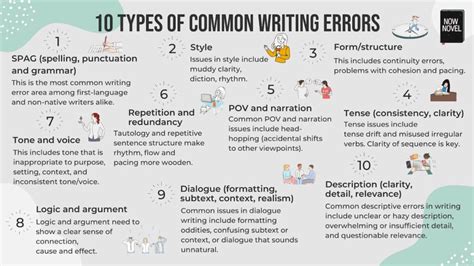
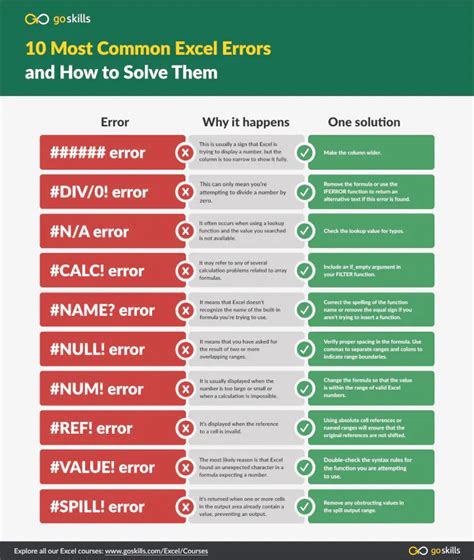
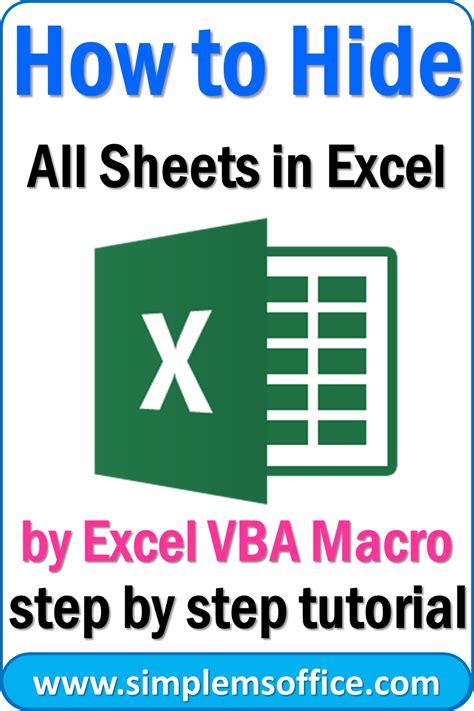
What is the difference between the Save and SaveAs methods?
+The Save method saves a workbook to its current file name and location, while the SaveAs method saves a workbook to a new file name and location.
How do I specify the file format when saving a workbook?
+You can specify the file format by including the file extension in the file name. For example, "NewWorkbook.xlsx" will save the workbook in the.xlsx file format.
How do I save a workbook with a password?
+You can save a workbook with a password by using the Password argument in the SaveAs method. For example, `ActiveWorkbook.SaveAs "C:\Users\Username\Desktop\NewWorkbook.xlsx", Password:="password"`
In conclusion, saving a workbook using Excel VBA is a straightforward process that can be accomplished using the Save, SaveAs, and SaveCopyAs methods. By following best practices and using error handling, you can ensure that your code runs smoothly and efficiently. If you have any questions or need further assistance, please don't hesitate to comment below. Share this article with your friends and colleagues who may benefit from learning about Excel VBA and saving workbooks. Take action today and start automating your Excel tasks with Excel VBA!