Intro
When working with Excel VBA, understanding how to manipulate and interact with worksheets is fundamental. One of the most basic yet crucial aspects of this interaction is being able to work with worksheet names. Whether you're creating new worksheets, renaming existing ones, or simply referencing them in your code, knowing how to handle worksheet names in VBA can significantly enhance your ability to automate and customize Excel tasks.
Excel VBA offers a robust set of tools and methods for managing worksheet names, from changing names to looping through all worksheets in a workbook. This article will delve into the specifics of working with worksheet names in Excel VBA, covering how to rename worksheets, reference worksheets by their names, and more. By the end of this guide, you'll have a solid understanding of how to leverage worksheet names in your VBA scripts to streamline your workflow and make your Excel applications more dynamic.
Importance of Worksheet Names in VBA
Worksheet names are crucial in VBA because they provide a unique identifier for each worksheet in a workbook. Unlike the worksheet index (which can change if worksheets are added, deleted, or rearranged), the worksheet name remains constant unless explicitly changed. This stability makes worksheet names a reliable way to reference specific worksheets in your VBA code, ensuring that your scripts operate on the intended data even if the workbook's structure changes.
Renaming Worksheets
Renaming worksheets is a common task when organizing or updating workbooks. In VBA, you can rename a worksheet using the Name
property of the Worksheet
object. Here's a basic example:
Sub RenameWorksheet()
ThisWorkbook.Worksheets("OldName").Name = "NewName"
End Sub
This code renames a worksheet currently named "OldName" to "NewName". Remember to replace "OldName" and "NewName" with the actual names you wish to use.
Referencing Worksheets by Name
To perform operations on a specific worksheet, you first need to reference it. You can do this by using the Worksheets
collection and specifying the worksheet's name:
Sub ReferenceWorksheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Now you can perform operations on ws
ws.Range("A1").Value = "Hello, World!"
End Sub
This example sets a variable ws
to reference the worksheet named "Sheet1" and then writes "Hello, World!" to cell A1 of that worksheet.
Looping Through All Worksheets
Sometimes, you might want to perform an action on every worksheet in a workbook. You can achieve this by looping through the Worksheets
collection:
Sub LoopThroughWorksheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Perform action on each worksheet
ws.Range("A1").Value = "Processed"
Next ws
End Sub
This code loops through each worksheet in the active workbook and writes "Processed" to cell A1 of every worksheet.
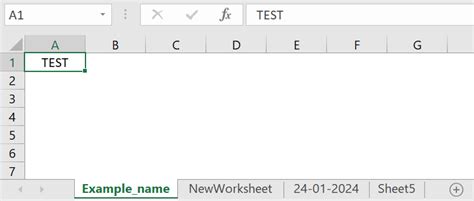
Creating New Worksheets
You can also create new worksheets using VBA. The Worksheets.Add
method allows you to add a new worksheet to the workbook:
Sub CreateNewWorksheet()
Dim newWS As Worksheet
Set newWS = ThisWorkbook.Worksheets.Add(After:=ThisWorkbook.Worksheets(ThisWorkbook.Worksheets.Count))
newWS.Name = "MyNewSheet"
End Sub
This code adds a new worksheet to the end of the workbook and names it "MyNewSheet".
Best Practices
- Use Meaningful Names: Choose worksheet names that are descriptive and easy to understand. This makes your code more readable and maintainable.
- Avoid Reserved Words: Ensure that your worksheet names do not conflict with VBA keywords or reserved words.
- Handle Errors: When working with worksheet names, consider implementing error handling to deal with situations where a worksheet might not exist or cannot be renamed.
Advanced Worksheet Name Management
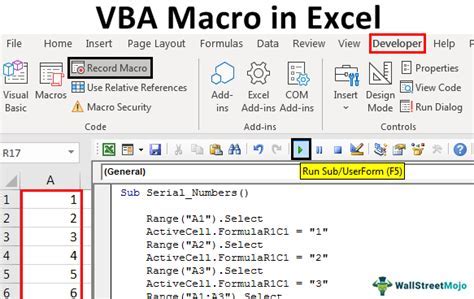
For more complex applications, you might need to manage worksheet names dynamically, such as generating names based on certain rules or input. VBA provides a versatile set of string manipulation functions that can be used to construct worksheet names programmatically.
Using Input for Worksheet Names
You can use the InputBox
function to prompt the user for a worksheet name:
Sub GetUserInputForWorksheetName()
Dim userInput As String
userInput = InputBox("Please enter a name for the new worksheet")
If userInput <> "" Then
ThisWorkbook.Worksheets.Add.Name = userInput
End If
End Sub
This code asks the user for a name and then adds a new worksheet with that name, provided the user entered something.
Gallery of Excel VBA Worksheet Name Examples
Excel VBA Worksheet Name Gallery
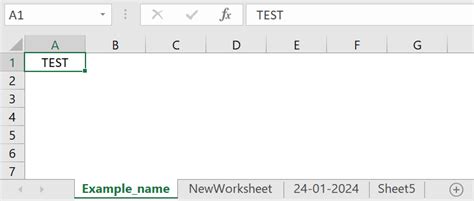
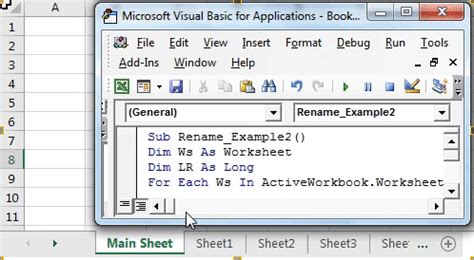

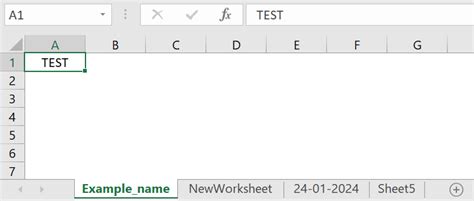
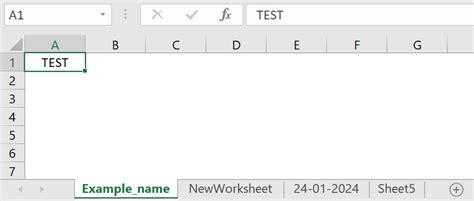
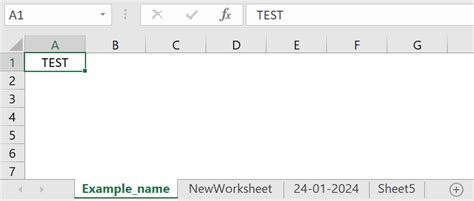
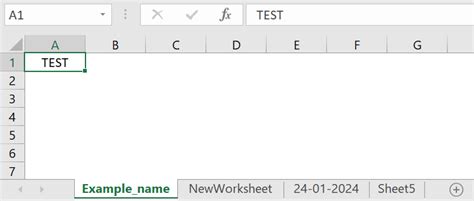
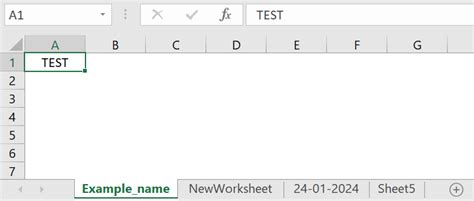
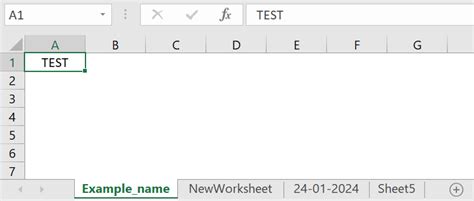
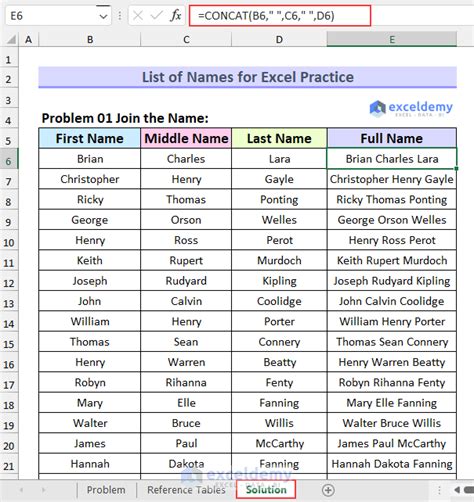
Frequently Asked Questions
How do I rename a worksheet in Excel VBA?
+You can rename a worksheet by using the `Name` property of the `Worksheet` object, like `ThisWorkbook.Worksheets("OldName").Name = "NewName"`.
How can I loop through all worksheets in a workbook and perform an action on each?
+Use a `For Each` loop with the `Worksheets` collection, like `For Each ws In ThisWorkbook.Worksheets`.
Can I create a new worksheet using VBA and name it dynamically?
+Yes, you can create a new worksheet with `ThisWorkbook.Worksheets.Add` and then set its name using the `Name` property.
As you explore the world of Excel VBA, mastering the manipulation of worksheet names will become an essential skill. Whether you're automating routine tasks, creating complex applications, or simply organizing your workbooks more efficiently, understanding how to work with worksheet names in VBA will significantly enhance your productivity and capabilities. Remember to practice regularly and experiment with different scenarios to deepen your understanding of these concepts. With time and practice, you'll become proficient in leveraging worksheet names to make your Excel tasks more efficient and your VBA scripts more powerful.
If you have any questions or need further clarification on any of the topics covered, don't hesitate to reach out. Share your experiences or tips on working with worksheet names in Excel VBA in the comments below. Your insights could help others in their journey to master Excel VBA.