Intro
Master Exit Sub in VBA, a crucial control statement for error handling and loop termination, with related keywords like VBA syntax, subroutine, and debugging techniques.
The world of Visual Basic for Applications (VBA) is a powerful tool for automating tasks and creating interactive applications in Microsoft Office. One of the fundamental concepts in VBA programming is the use of the "Exit Sub" statement. In this article, we will delve into the importance of the "Exit Sub" statement, its syntax, and how it is used in VBA programming.
When working with VBA, it is essential to understand the flow of your code and how to control it. The "Exit Sub" statement is a crucial element in achieving this control. It allows you to exit a subroutine prematurely, skipping any remaining code within the subroutine. This can be particularly useful in a variety of situations, such as when an error occurs, when a specific condition is met, or when you need to redirect the flow of your program.
Understanding the Syntax
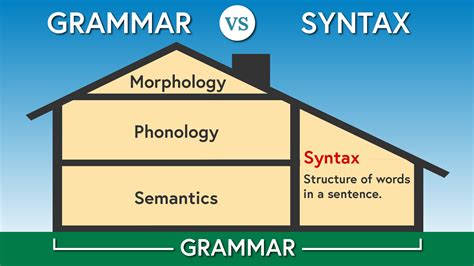
The syntax for the "Exit Sub" statement is straightforward: you simply type "Exit Sub" in your code where you want the subroutine to end. There are no arguments or parameters required. When VBA encounters an "Exit Sub" statement, it immediately exits the current subroutine, and program execution continues with the statement that called the subroutine.
Benefits of Using Exit Sub
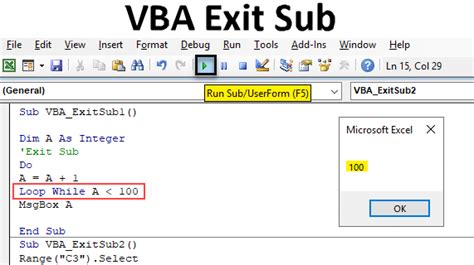
The use of "Exit Sub" in VBA offers several benefits, including improved code readability, reduced complexity, and enhanced error handling. By exiting a subroutine early, you can avoid executing unnecessary code, which can improve the performance of your application. Additionally, "Exit Sub" can be used to handle errors gracefully, allowing your program to recover from unexpected situations and continue running smoothly.
Example of Using Exit Sub
Here is a simple example of how "Exit Sub" might be used in a VBA subroutine: ```vba Sub ExampleSub() Dim userInput As String userInput = InputBox("Please enter your name")If userInput = "" Then
MsgBox "You must enter your name."
Exit Sub
End If
' If the user entered their name, the subroutine continues here
MsgBox "Hello, " & userInput
End Sub
In this example, if the user fails to enter their name, the subroutine displays an error message and then exits using the "Exit Sub" statement, preventing the rest of the subroutine from executing.
Best Practices for Using Exit Sub
While "Exit Sub" is a powerful tool, it should be used judiciously. Overuse or misuse of "Exit Sub" can lead to code that is difficult to understand and maintain. Here are some best practices to keep in mind:
- Use "Exit Sub" sparingly and only when necessary. It's generally better to use conditional statements to control the flow of your code.
- Always consider the impact of exiting a subroutine prematurely on the state of your application and its variables.
- Use comments to explain why "Exit Sub" is being used, especially in complex code.
Common Scenarios for Exit Sub
"Exit Sub" is commonly used in the following scenarios:
- Error handling: To exit a subroutine when an error occurs, allowing for graceful recovery or error reporting.
- Validation: To check if certain conditions are met before proceeding with the rest of the subroutine.
- Optional processing: To skip parts of a subroutine based on user input or other factors.
Alternatives to Exit Sub
While "Exit Sub" is useful, there are alternative ways to control the flow of your code. For example, you can use "If" statements to conditionally execute parts of your code, or you can use "Select Case" statements for more complex conditional logic. In some cases, restructuring your code to use functions instead of subroutines can also provide a cleaner way to exit early, by using the "Return" statement.
Conclusion and Next Steps
In conclusion, the "Exit Sub" statement is a valuable tool in VBA programming that allows for premature exit from a subroutine. By understanding its syntax, benefits, and best practices, you can write more efficient, readable, and maintainable code. Whether you're a beginner or an experienced VBA developer, mastering the use of "Exit Sub" can significantly enhance your programming skills.
VBA Exit Sub Image Gallery
What is the purpose of the Exit Sub statement in VBA?
+
The Exit Sub statement is used to exit a subroutine prematurely, skipping any remaining code within the subroutine.
How do I use the Exit Sub statement in my VBA code?
+
You can use the Exit Sub statement by typing "Exit Sub" in your code where you want the subroutine to end. There are no arguments or parameters required.
What are some best practices for using the Exit Sub statement in VBA?
+
Some best practices for using the Exit Sub statement include using it sparingly and only when necessary, considering the impact of exiting a subroutine prematurely on the state of your application and its variables, and using comments to explain why Exit Sub is being used.
We hope this comprehensive guide to the "Exit Sub" statement in VBA has been informative and helpful. Whether you're looking to improve your coding skills or simply need a refresher on VBA basics, understanding how to use "Exit Sub" effectively can make a significant difference in your programming abilities. Feel free to share your thoughts, ask questions, or provide feedback in the comments section below. Your engagement is valued, and we look forward to hearing from you.