Intro
Master File System Object VBA with expert tutorials, leveraging FSO for file management, scripting, and automation, using related keywords like Excel VBA, FileSystemObject, and VBA scripting.
The File System Object (FSO) is a powerful tool in Visual Basic for Applications (VBA) that allows developers to interact with the file system. It provides a simple and efficient way to perform various file-related operations, such as creating, deleting, and manipulating files and folders. In this article, we will delve into the world of FSO and explore its features, benefits, and applications.
The importance of FSO in VBA cannot be overstated. It enables developers to automate tasks that would otherwise require manual intervention, such as file management, data transfer, and system maintenance. With FSO, developers can write code that can create, delete, and manipulate files and folders, as well as read and write file contents. This makes it an essential tool for any VBA developer who needs to work with files and folders.
One of the key benefits of FSO is its ease of use. The object model is simple and intuitive, making it easy for developers to learn and use. Additionally, FSO provides a wide range of methods and properties that can be used to perform various file-related operations. For example, the CreateFolder
method can be used to create a new folder, while the DeleteFile
method can be used to delete a file. The FileExists
property can be used to check if a file exists, and the FolderExists
property can be used to check if a folder exists.
Introduction to File System Object
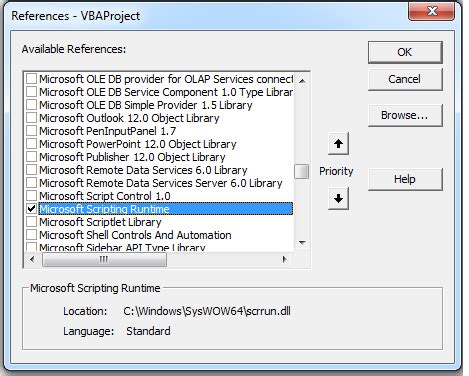
To use FSO in VBA, developers need to set a reference to the FSO library. This can be done by opening the Visual Basic Editor, clicking on "Tools" in the menu bar, and then selecting "References." In the References dialog box, developers can scroll down and check the box next to "Microsoft Scripting Runtime." This will enable the FSO library and allow developers to use its objects and methods.
Key Features of File System Object
The FSO library provides a range of objects and methods that can be used to interact with the file system. Some of the key features of FSO include:- Creating and deleting files and folders
- Reading and writing file contents
- Checking if a file or folder exists
- Getting the name and path of a file or folder
- Moving and copying files and folders
These features make FSO a powerful tool for automating file-related tasks in VBA.
Working with Files and Folders
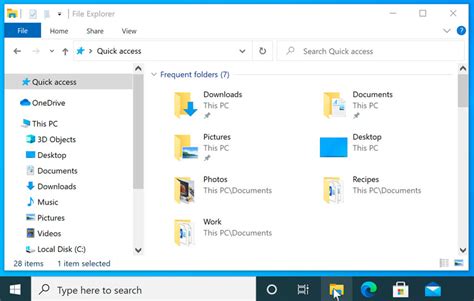
FSO provides several objects that can be used to work with files and folders. The File
object represents a file, while the Folder
object represents a folder. The Drive
object represents a drive, and the TextStream
object represents a text stream.
The File
object has several methods and properties that can be used to work with files. For example, the OpenAsTextStream
method can be used to open a file as a text stream, while the Delete
method can be used to delete a file. The Name
property can be used to get the name of a file, and the Path
property can be used to get the path of a file.
The Folder
object also has several methods and properties that can be used to work with folders. For example, the CreateFolder
method can be used to create a new folder, while the Delete
method can be used to delete a folder. The Name
property can be used to get the name of a folder, and the Path
property can be used to get the path of a folder.
Example Code
Here is an example of how to use FSO to create a new folder: ```vb Dim fso As FileSystemObject Dim folder As FolderSet fso = New FileSystemObject Set folder = fso.CreateFolder("C:\MyNewFolder")
MsgBox "Folder created successfully!"
This code creates a new folder called "MyNewFolder" in the root directory of the C drive.
Benefits of Using File System Object
There are several benefits to using FSO in VBA. Some of the key benefits include:
* **Ease of use**: FSO provides a simple and intuitive object model that makes it easy for developers to learn and use.
* **Flexibility**: FSO provides a wide range of methods and properties that can be used to perform various file-related operations.
* **Power**: FSO provides a powerful way to interact with the file system, allowing developers to automate complex tasks and workflows.
Overall, FSO is a powerful tool that can be used to automate file-related tasks in VBA. Its ease of use, flexibility, and power make it an essential tool for any VBA developer who needs to work with files and folders.
Best Practices for Using File System Object
Here are some best practices for using FSO in VBA:
* **Use the `FileSystemObject` variable**: Always use the `FileSystemObject` variable to access FSO objects and methods.
* **Use error handling**: Always use error handling to catch and handle errors that may occur when working with FSO.
* **Use the `Exists` method**: Always use the `Exists` method to check if a file or folder exists before attempting to access it.
By following these best practices, developers can ensure that their code is robust, reliable, and efficient.
Common Errors and Troubleshooting
Here are some common errors and troubleshooting tips for using FSO in VBA:
* **Error 76: Path not found**: This error occurs when the specified path does not exist. To troubleshoot, check the path and ensure that it is correct.
* **Error 70: Permission denied**: This error occurs when the user does not have permission to access the specified file or folder. To troubleshoot, check the permissions and ensure that the user has the necessary access rights.
By following these troubleshooting tips, developers can quickly and easily resolve common errors that may occur when using FSO in VBA.
Gallery of File System Object Examples
File System Object Image Gallery
Frequently Asked Questions
What is the File System Object?
+
The File System Object (FSO) is a powerful tool in Visual Basic for Applications (VBA) that allows developers to interact with the file system.
How do I use the File System Object in VBA?
+
To use FSO in VBA, developers need to set a reference to the FSO library. This can be done by opening the Visual Basic Editor, clicking on "Tools" in the menu bar, and then selecting "References."
What are the benefits of using the File System Object?
+
The benefits of using FSO include ease of use, flexibility, and power. FSO provides a simple and intuitive object model that makes it easy for developers to learn and use.
In conclusion, the File System Object is a powerful tool in VBA that allows developers to interact with the file system. Its ease of use, flexibility, and power make it an essential tool for any VBA developer who needs to work with files and folders. By following the best practices and troubleshooting tips outlined in this article, developers can ensure that their code is robust, reliable, and efficient. We hope this article has provided you with a comprehensive understanding of the File System Object and its applications in VBA. If you have any further questions or would like to share your experiences with FSO, please don't hesitate to comment below.