Intro
Learn about Global Variables in VBA, including declaration, scope, and usage, to enhance your Excel macros with modular, reusable, and efficient code, leveraging public variables and module-level variables.
The use of global variables in VBA (Visual Basic for Applications) is a topic of great importance for developers and users alike. Global variables are variables that are declared outside of any procedure, such as a subroutine or function, and are accessible from any part of the VBA code. In this article, we will delve into the world of global variables in VBA, exploring their benefits, drawbacks, and best practices for use.
To begin with, it's essential to understand why global variables are used in the first place. One of the primary reasons is to share data between different parts of the code. When a variable is declared as global, it can be accessed and modified by any procedure, making it a convenient way to pass data around. However, this convenience comes with a price, as we will discuss later.
Declaring Global Variables
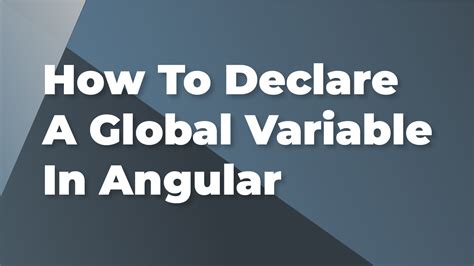
Declaring a global variable in VBA is relatively straightforward. You can declare a variable at the top of a module, outside of any procedure, using the Public
or Global
keyword. For example:
Public myGlobalVariable As String
Alternatively, you can use the Global
keyword to declare a global variable:
Global myGlobalVariable As String
Both of these declarations will make the variable accessible from any part of the code.
Benefits of Global Variables
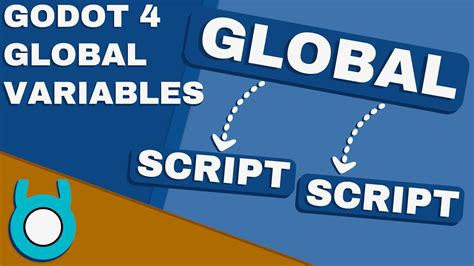
So, what are the benefits of using global variables in VBA? Here are a few:
- Convenience: Global variables make it easy to share data between different parts of the code.
- Simplified code: By using global variables, you can avoid passing variables as arguments to procedures, which can simplify your code.
- Easier debugging: With global variables, you can easily inspect the value of a variable from any part of the code.
Drawbacks of Global Variables
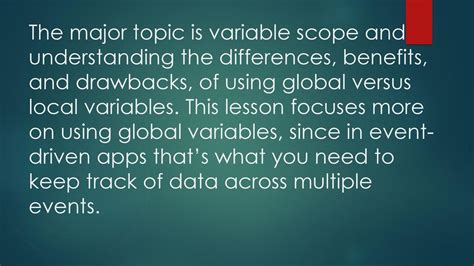
While global variables may seem like a convenient solution, they also have some significant drawbacks:
- Tight coupling: Global variables can create tight coupling between different parts of the code, making it harder to modify or maintain the code.
- Namespace pollution: Global variables can pollute the namespace, making it harder to find and use other variables and procedures.
- Debugging challenges: While global variables can make debugging easier in some cases, they can also make it harder to track down bugs, as the value of a global variable can be modified from anywhere in the code.
Best Practices for Using Global Variables
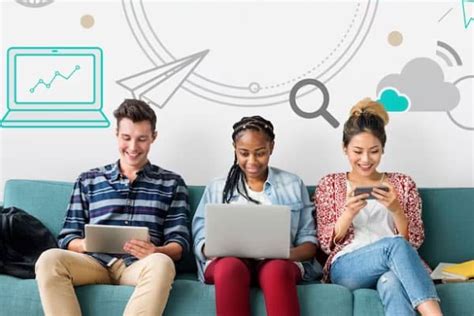
So, how can you use global variables effectively in VBA? Here are some best practices to keep in mind:
- Use them sparingly: Global variables should be used only when necessary, and only for variables that need to be shared across multiple procedures.
- Use meaningful names: Choose meaningful and descriptive names for your global variables, to make it clear what they represent.
- Document them: Document your global variables, so that other developers (or your future self) can understand what they do and how to use them.
Alternatives to Global Variables
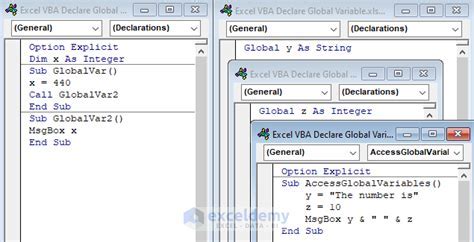
If global variables are not the best solution, what are the alternatives? Here are a few:
- Pass variables as arguments: Instead of using global variables, you can pass variables as arguments to procedures.
- Use modules: You can use modules to encapsulate related procedures and variables, making it easier to manage and maintain your code.
- Use classes: You can use classes to create objects that encapsulate data and behavior, making it easier to manage complex data structures.
Gallery of VBA Global Variables
VBA Global Variables Image Gallery
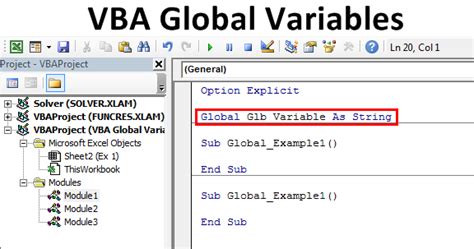
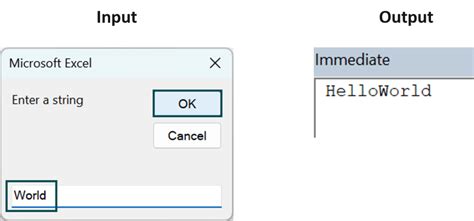
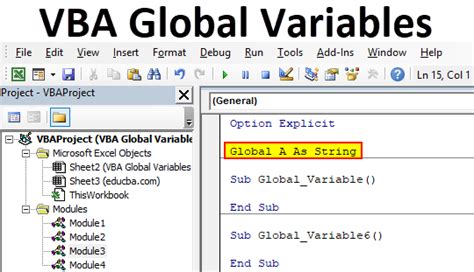
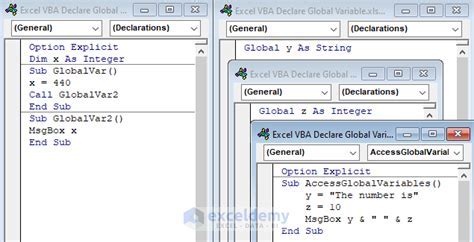
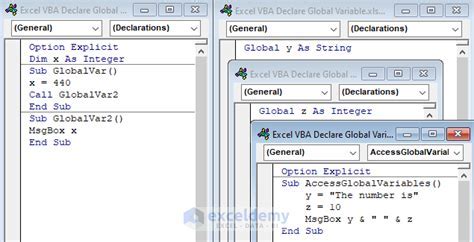
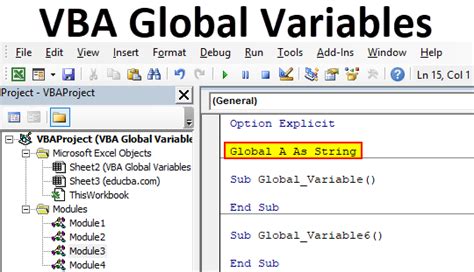

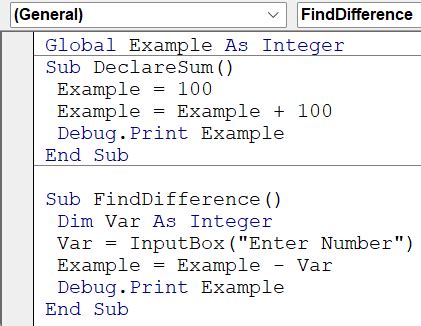
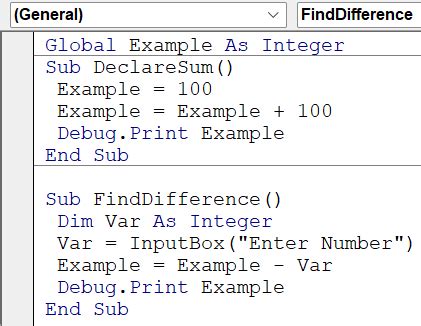
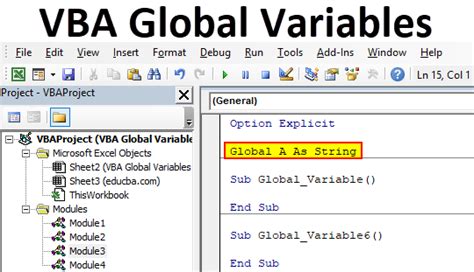
Frequently Asked Questions
What is a global variable in VBA?
+A global variable is a variable that is declared outside of any procedure and is accessible from any part of the VBA code.
How do I declare a global variable in VBA?
+You can declare a global variable using the `Public` or `Global` keyword, followed by the variable name and data type.
What are the benefits of using global variables in VBA?
+The benefits of using global variables include convenience, simplified code, and easier debugging.
What are the drawbacks of using global variables in VBA?
+The drawbacks of using global variables include tight coupling, namespace pollution, and debugging challenges.
How can I use global variables effectively in VBA?
+You can use global variables effectively by using them sparingly, choosing meaningful names, and documenting them.
In conclusion, global variables can be a powerful tool in VBA, but they should be used with caution. By understanding the benefits and drawbacks of global variables, and following best practices for their use, you can write more effective and maintainable code. We hope this article has provided you with a comprehensive understanding of global variables in VBA, and has inspired you to explore the world of VBA programming. If you have any questions or comments, please don't hesitate to share them with us.