Intro
Discover how to use Global Variables in VBA to enhance macro functionality, sharing data across modules with Public and Private declarations, and mastering scope, lifetime, and debugging techniques for efficient coding.
The world of Visual Basic for Applications (VBA) is a vast and powerful realm, allowing users to automate and enhance their Microsoft Office experiences. Within this realm, variables play a crucial role in storing and manipulating data. Among these variables, global variables hold a special place due to their scope and accessibility throughout an application. Understanding global variables in VBA is essential for any developer aiming to create robust, efficient, and well-structured code.
Global variables are defined outside any procedure or module, making them accessible from any part of the VBA project. This characteristic is both their greatest strength and most significant weakness. On one hand, global variables provide a convenient way to share data across different parts of an application. On the other hand, they can lead to tight coupling between different components of the code, making it harder to understand, test, and maintain.
Declaring Global Variables
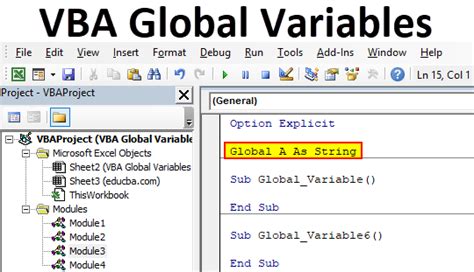
To declare a global variable in VBA, you use the Public
keyword outside any subroutine or function, typically at the top of a module. For example:
Public gUserName As String
This declaration makes gUserName
a global variable that can be accessed and modified from any module within the VBA project.
Scope and Lifetime

The scope of a global variable refers to where it can be accessed, which in this case is everywhere within the VBA project. The lifetime of a global variable, on the other hand, refers to how long it retains its value. In VBA, global variables retain their values for the duration of the session, meaning they are reset or lost when the application is closed.
Using Global Variables
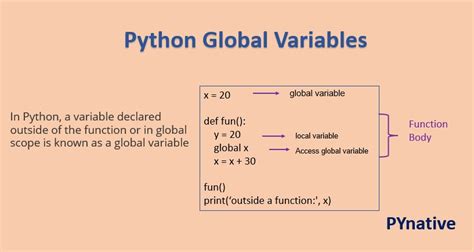
While global variables can be tempting to use due to their ease of access, it's essential to use them judiciously. Here are some best practices:
- Minimize Use: Try to limit the use of global variables to situations where it's absolutely necessary. Instead, consider passing variables as arguments to procedures.
- Clear Naming: Use clear and descriptive names for global variables to avoid confusion.
- Documentation: Document the purpose and use of each global variable.
Alternatives to Global Variables
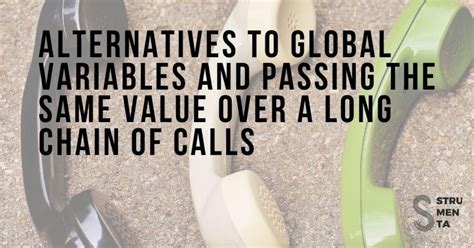
Given the potential drawbacks of global variables, it's worth considering alternatives:
- Module-Level Variables: These are declared within a module but outside any procedure, making them accessible to all procedures within that module.
- Procedure-Level Variables: Declared within a procedure, these variables are only accessible within that procedure.
- Object Properties: In object-oriented programming, properties of objects can serve as alternatives to global variables, encapsulating data within the object.
Best Practices for Global Variables
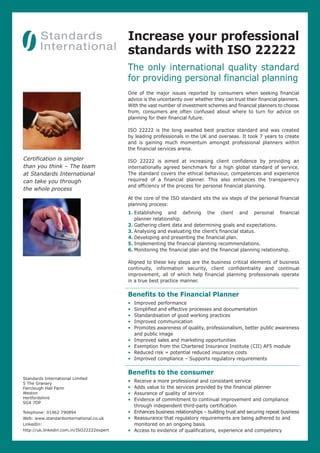
To ensure the effective and safe use of global variables:
- Keep Them to a Minimum: Only use global variables when necessary.
- Use Meaningful Names: Names should clearly indicate the variable's purpose.
- Avoid Magic Numbers: Instead of hard-coding values, define them as named constants.
- Comment Your Code: Clearly document the purpose and use of each global variable.
Common Pitfalls
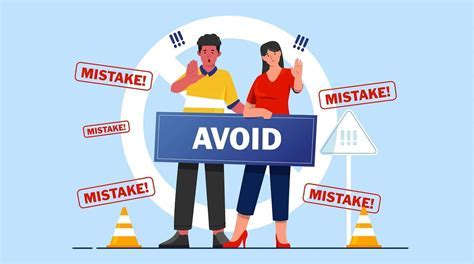
Be aware of the following common pitfalls:
- Tight Coupling: Global variables can make different parts of your code tightly coupled, making changes difficult.
- Namespace Pollution: Too many global variables can clutter the global namespace.
- Debugging Challenges: With data being modified from anywhere, debugging can become more complicated.
Gallery of Global Variables in VBA
Global Variables in VBA Image Gallery
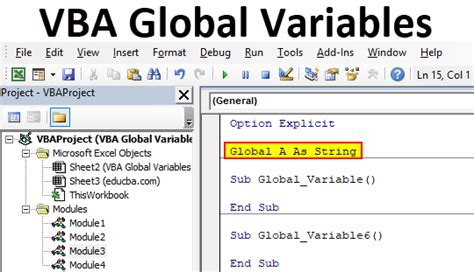

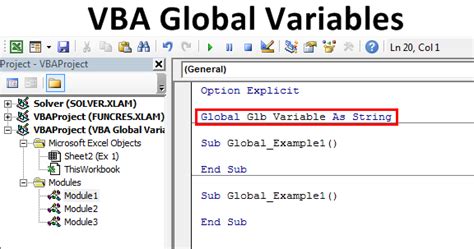
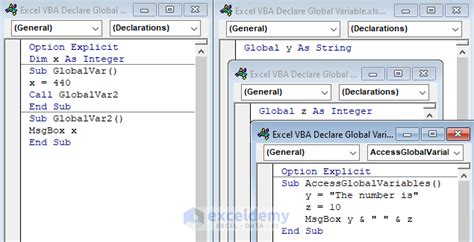
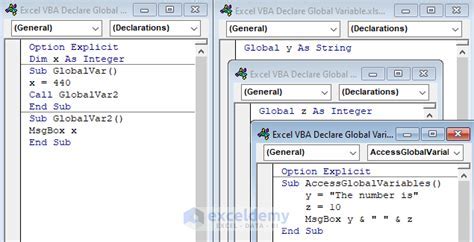
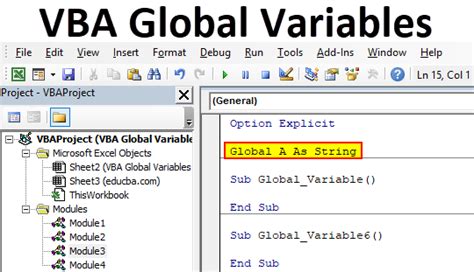
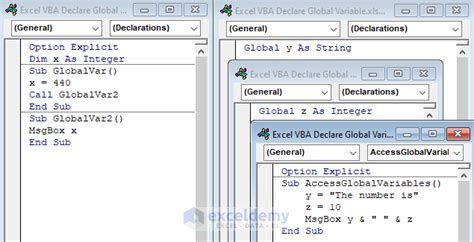
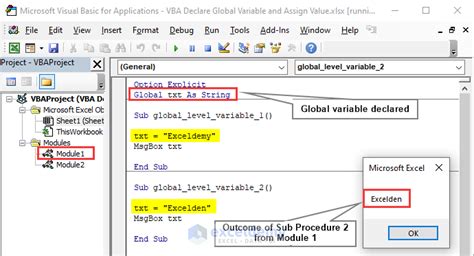
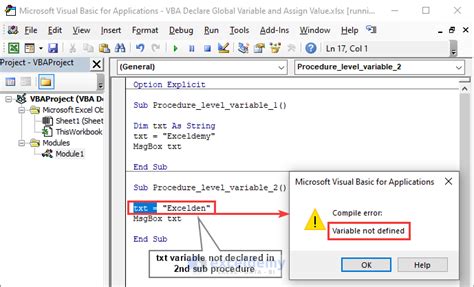
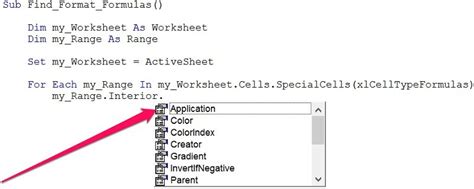
What are global variables in VBA?
+Global variables in VBA are variables that are declared outside any procedure or module, making them accessible from any part of the VBA project.
How do you declare a global variable in VBA?
+To declare a global variable, use the `Public` keyword outside any subroutine or function, typically at the top of a module.
What are the alternatives to using global variables in VBA?
+Alternatives include module-level variables, procedure-level variables, and object properties, which can help reduce the need for global variables and improve code organization.
In conclusion, while global variables in VBA offer a convenient way to share data across an application, their use should be carefully considered due to potential drawbacks such as tight coupling and debugging challenges. By understanding how to declare, use, and manage global variables effectively, and by being aware of their alternatives, developers can write more robust, maintainable, and efficient VBA code. Whether you're automating tasks in Excel, Word, or another Office application, mastering the use of global variables is a key skill for any VBA developer. Share your thoughts on global variables in VBA and how you've used them in your projects in the comments below.