Intro
The Goto function in VBA is a statement that transfers control to a specified line of code. This function is often used to skip over certain parts of a program or to repeat a section of code. However, it's generally considered poor programming practice to use Goto statements excessively, as they can make code difficult to read and debug.
In VBA, the Goto statement is used in conjunction with a label, which is a unique identifier that marks a specific line of code. The syntax for the Goto statement is Goto label
. For example, Goto Start
would transfer control to the line of code labeled Start
.
One of the main uses of the Goto function is in error handling. When an error occurs, the program can use a Goto statement to jump to a section of code that handles the error. This can be useful for preventing the program from crashing or displaying an error message to the user.
Another use of the Goto function is in loops. A Goto statement can be used to repeat a section of code, such as when reading a file or processing a list of items.
However, there are some drawbacks to using the Goto function. For one, it can make code difficult to read and understand, as the flow of the program is not always clear. Additionally, excessive use of Goto statements can lead to "spaghetti code," which is code that is tangled and hard to follow.
How to Use the Goto Function in VBA
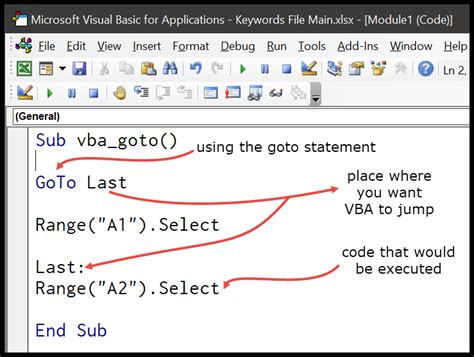
To use the Goto function in VBA, you need to create a label that marks the line of code where you want to transfer control. You can create a label by typing a unique identifier followed by a colon. For example, Start:
would create a label called Start
.
Once you have created a label, you can use the Goto statement to transfer control to that label. For example, Goto Start
would transfer control to the line of code labeled Start
.
Here is an example of how to use the Goto function in VBA:
Sub Example()
Goto Start
' This code will be skipped
MsgBox "This message will not be displayed"
Start:
MsgBox "This message will be displayed"
End Sub
In this example, the Goto statement transfers control to the line of code labeled Start
, skipping over the code in between.
Best Practices for Using the Goto Function
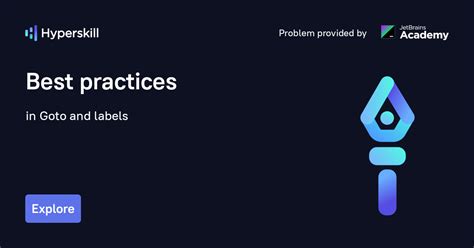
While the Goto function can be useful in certain situations, there are some best practices to keep in mind when using it:
- Use Goto statements sparingly. Excessive use of Goto statements can make code difficult to read and debug.
- Use meaningful labels. Choose labels that clearly indicate what the code is doing.
- Avoid using Goto statements to transfer control to a label that is not clearly defined.
- Use Goto statements only when necessary. In many cases, there are better ways to structure your code that don't involve using Goto statements.
Alternatives to the Goto Function
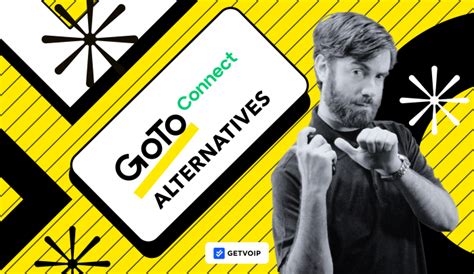
There are several alternatives to the Goto function that can be used in VBA, including:
- Loops: Loops can be used to repeat a section of code, eliminating the need for Goto statements.
- Conditional statements: Conditional statements, such as If-Then statements, can be used to control the flow of a program without using Goto statements.
- Subroutines: Subroutines can be used to break up a large program into smaller, more manageable pieces, reducing the need for Goto statements.
Here is an example of how to use a loop instead of a Goto statement:
Sub Example()
Dim i As Integer
For i = 1 To 10
' Code to be repeated
Next i
End Sub
In this example, the loop repeats the code 10 times, eliminating the need for a Goto statement.
Common Errors When Using the Goto Function
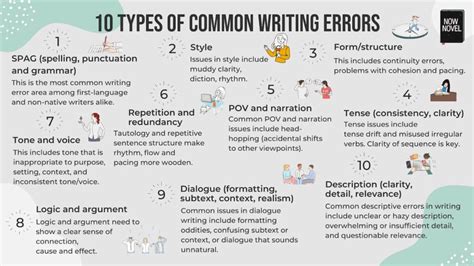
There are several common errors that can occur when using the Goto function, including:
- Undefined labels: If a label is not clearly defined, the Goto statement will not work as expected.
- Infinite loops: If a Goto statement is used to transfer control to a label that is inside a loop, it can create an infinite loop.
- Unintended consequences: Goto statements can have unintended consequences, such as skipping over important code or causing errors.
To avoid these errors, it's essential to use Goto statements carefully and only when necessary.
Conclusion and Next Steps
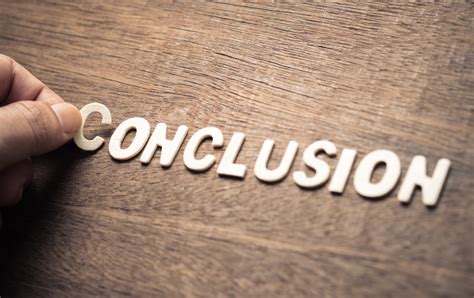
In conclusion, the Goto function is a powerful tool in VBA that can be used to transfer control to a specified line of code. However, it should be used sparingly and only when necessary. By following best practices and using alternatives to the Goto function, you can write more efficient and effective code.
If you're interested in learning more about VBA and the Goto function, there are several resources available, including online tutorials and coding communities. With practice and experience, you can become proficient in using the Goto function and other VBA tools to create powerful and efficient code.
Goto Function in VBA Image Gallery
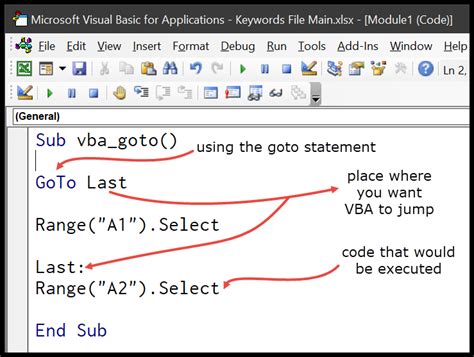
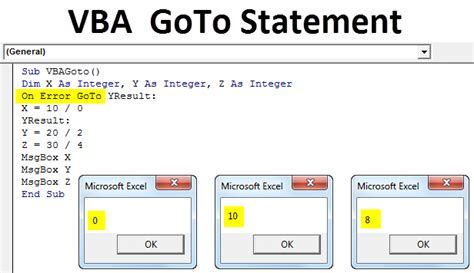
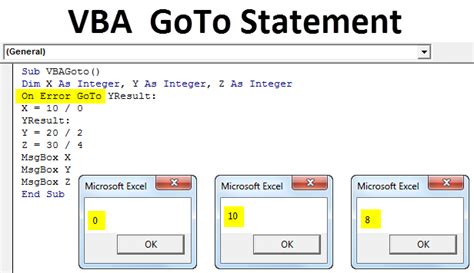
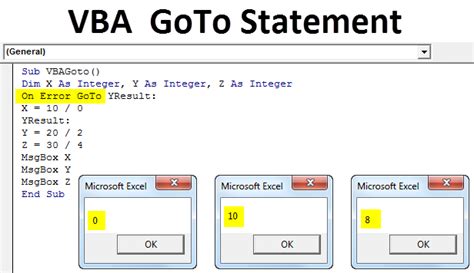
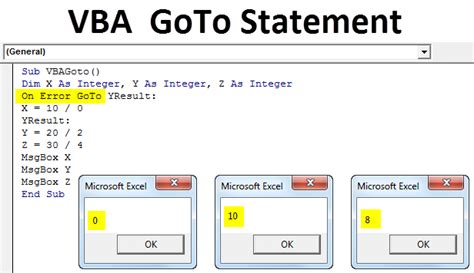
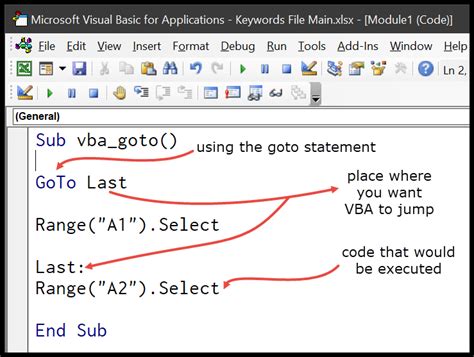
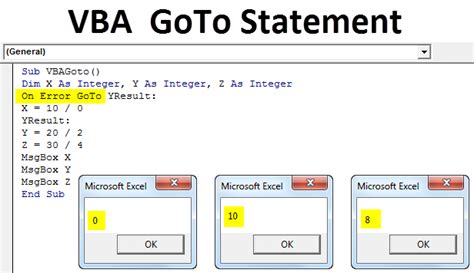
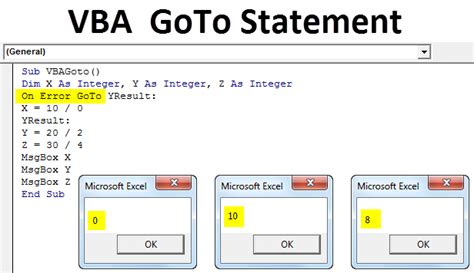
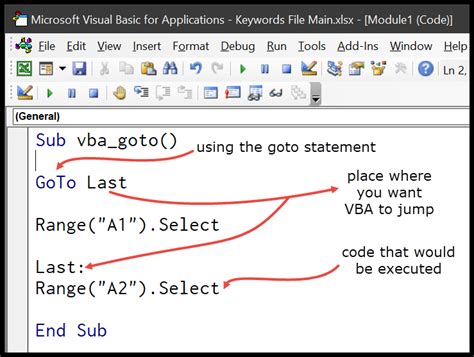
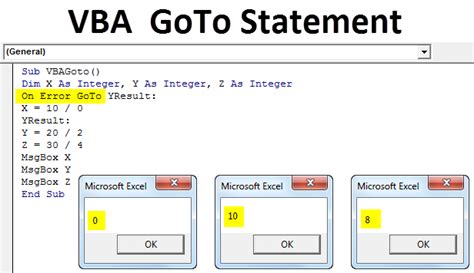
What is the Goto function in VBA?
+The Goto function in VBA is a statement that transfers control to a specified line of code.
How do I use the Goto function in VBA?
+To use the Goto function in VBA, you need to create a label that marks the line of code where you want to transfer control, and then use the Goto statement to transfer control to that label.
What are some alternatives to the Goto function in VBA?
+Some alternatives to the Goto function in VBA include loops, conditional statements, and subroutines.
What are some common errors when using the Goto function in VBA?
+Some common errors when using the Goto function in VBA include undefined labels, infinite loops, and unintended consequences.
How can I avoid using the Goto function in VBA?
+You can avoid using the Goto function in VBA by using loops, conditional statements, and subroutines instead.
We hope this article has provided you with a comprehensive understanding of the Goto function in VBA. If you have any further questions or would like to share your experiences with using the Goto function, please feel free to comment below. Additionally, if you found this article helpful, please share it with others who may benefit from it.