Intro
Master Excel VBA with 5 ways to insert rows, including looping, range offset, and worksheet change events, to automate tasks and boost productivity with Excel macros and VBA scripting.
Inserting rows in Excel using VBA can be a powerful tool for automating tasks and streamlining workflows. Understanding how to insert rows effectively is crucial for managing and manipulating data within spreadsheets. Here, we'll explore five different ways to insert rows in Excel using VBA, each with its own set of applications and benefits.
Excel VBA provides a flexible and efficient way to automate tasks, including the insertion of rows. This can be particularly useful for tasks that require repetitive actions, such as data entry, report generation, or spreadsheet maintenance. By leveraging VBA, users can create macros that can insert rows based on specific conditions, at specific locations, or in bulk, significantly enhancing productivity and reducing the risk of manual errors.
Understanding the Basics of Inserting Rows in Excel VBA
Before diving into the methods, it's essential to understand the basic syntax and principles of inserting rows in Excel VBA. The Rows
object in VBA represents the rows in a worksheet. To insert a row, you typically use the Insert
method, specifying the row number where you want the insertion to occur.
1. Inserting a Single Row
Inserting a single row is one of the most common operations in Excel VBA. This can be achieved using a straightforward piece of code that specifies the row number where the insertion should take place.
Sub InsertSingleRow()
Rows(5).Insert
End Sub
This code will insert a new row at row number 5, shifting all existing data and formatting down by one row.
2. Inserting Multiple Rows
Sometimes, you may need to insert more than one row at a time. Excel VBA allows you to do this by specifying a range of rows.
Sub InsertMultipleRows()
Rows("5:10").Insert
End Sub
This example inserts six new rows starting from row 5, effectively creating space for additional data entry or manipulation.
3. Inserting Rows Based on Conditions
Inserting rows based on specific conditions can be highly useful for dynamic data manipulation. For instance, you might want to insert a row whenever a certain condition is met in your data.
Sub InsertRowBasedOnCondition()
Dim i As Long
For i = 1 To 10
If Cells(i, 1).Value = "Insert" Then
Rows(i + 1).Insert
End If
Next i
End Sub
This code checks each cell in the first column of the first 10 rows. If a cell contains the word "Insert", it inserts a new row below that cell.
4. Inserting Rows at the Bottom of a Dataset
Often, you'll need to add new data to the bottom of an existing dataset. Excel VBA can automatically determine the last row of your data and insert new rows as needed.
Sub InsertRowAtBottom()
Dim lastRow As Long
lastRow = Cells.Find("*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
Rows(lastRow + 1).Insert
End Sub
This code finds the last row with data in the worksheet and inserts a new row immediately below it.
5. Inserting Rows with User Input
For more interactive applications, you might want to insert rows based on user input, such as asking the user where they want to insert the row or how many rows to insert.
Sub InsertRowWithUserInput()
Dim rowNumber As Variant
rowNumber = InputBox("Enter the row number where you want to insert a new row")
If IsNumeric(rowNumber) Then
Rows(rowNumber).Insert
Else
MsgBox "Please enter a valid row number"
End If
End Sub
This example prompts the user to enter a row number. If the input is a valid number, it inserts a new row at the specified position.
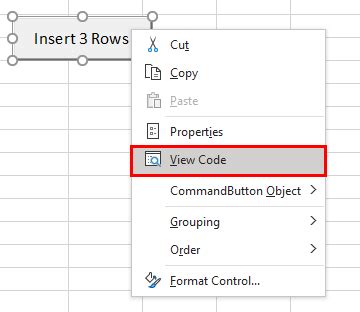
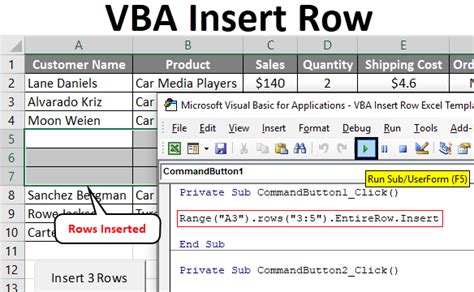
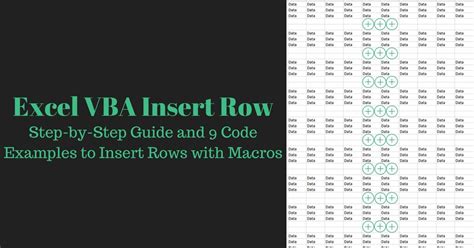
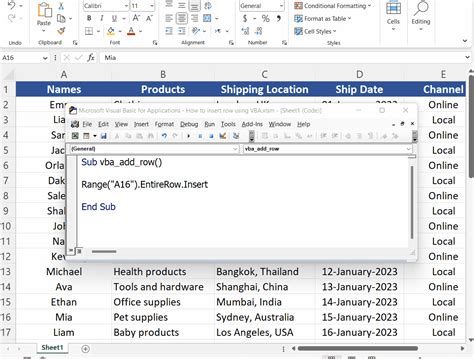
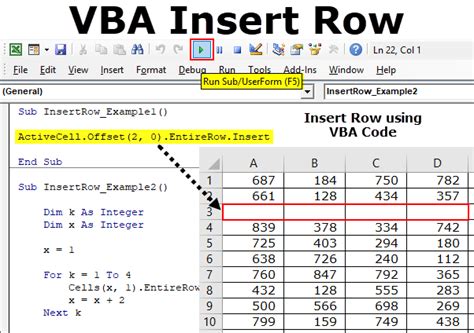
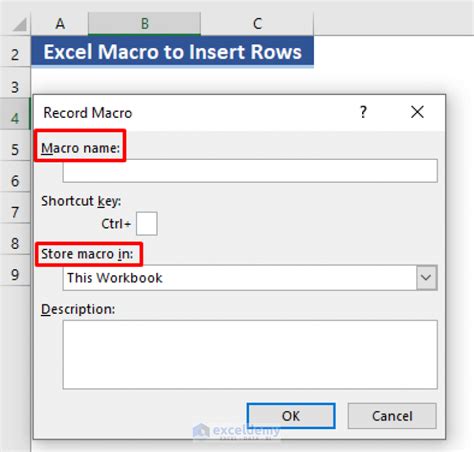
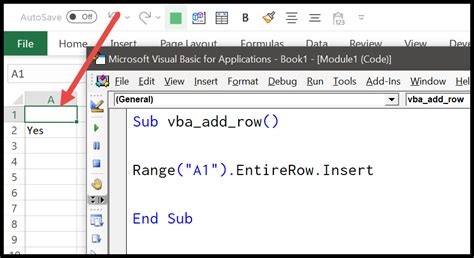
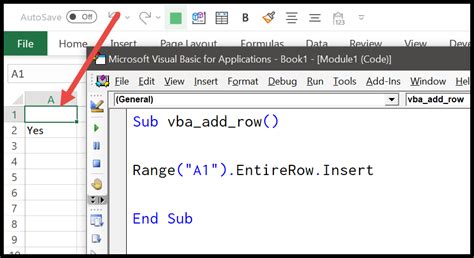
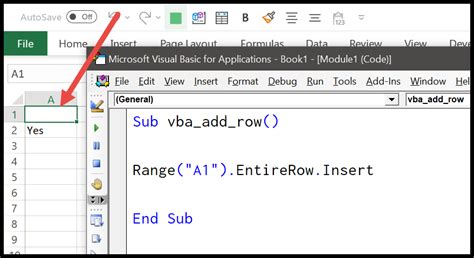
Gallery of Excel VBA Row Insertion
Excel VBA Row Insertion Gallery
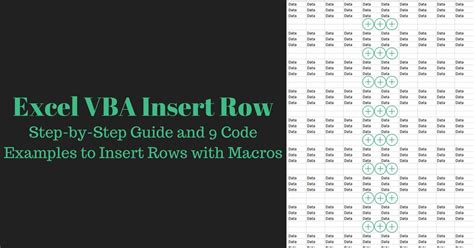
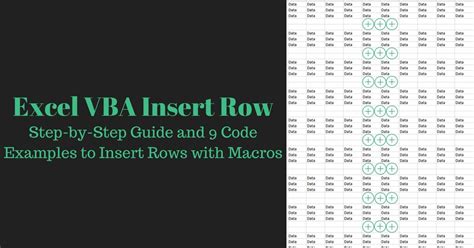
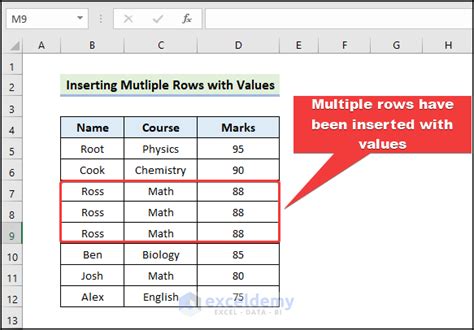
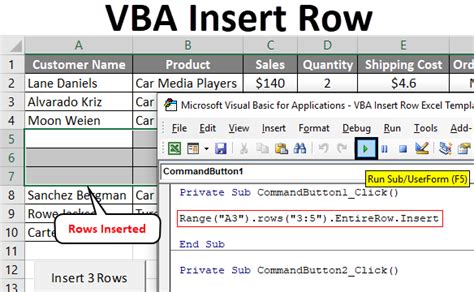

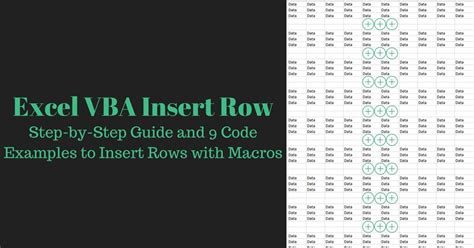
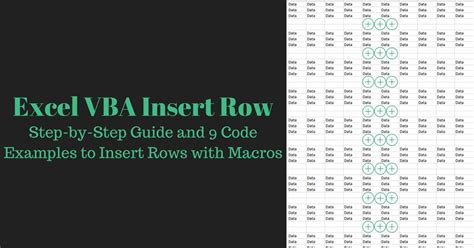
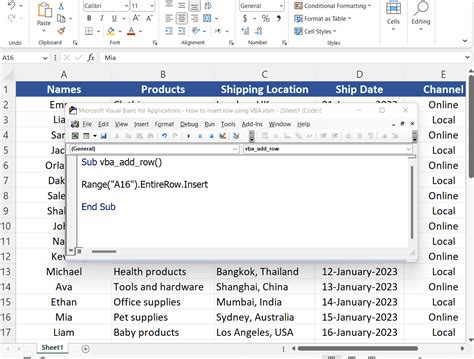
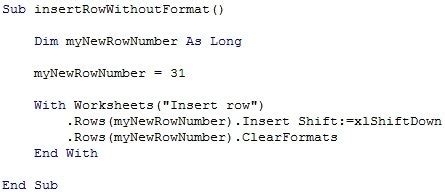
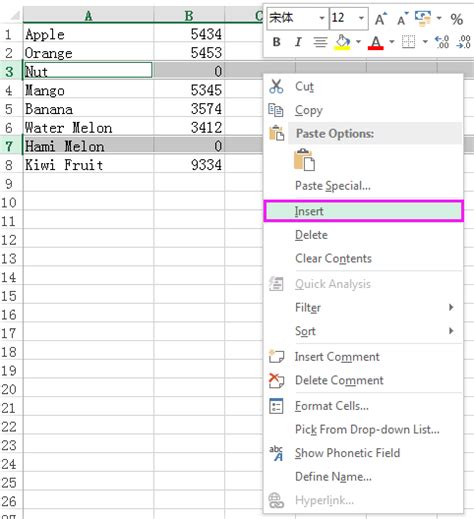
What is the basic syntax for inserting a row in Excel VBA?
+The basic syntax involves using the `Rows` object followed by the `Insert` method, specifying the row number where the insertion should occur, such as `Rows(5).Insert`.
How can I insert multiple rows at once using VBA?
+You can insert multiple rows by specifying a range of rows, such as `Rows("5:10").Insert`, which will insert six new rows starting from row 5.
Can I insert rows based on specific conditions in my data?
+Yes, you can use conditional statements (like `If` statements) to check for specific conditions in your data and insert rows accordingly.
To further enhance your understanding and application of Excel VBA for inserting rows, consider exploring more advanced topics such as looping through data, working with arrays, and integrating user forms for more interactive applications. By mastering these techniques, you can unlock the full potential of Excel VBA and significantly improve your productivity and data management capabilities. Feel free to comment below with any questions or share your experiences with using VBA for row insertion in Excel.