Intro
Generate random numbers in Excel with VBA, using macros and scripting to create custom random number generators, including uniform and non-uniform distributions, for simulations and modeling.
Excel VBA is a powerful tool that allows users to create custom functions and automate tasks within Excel. One of the many uses of Excel VBA is to generate random numbers, which can be useful in a variety of applications, such as simulations, modeling, and analysis. In this article, we will explore the different ways to generate random numbers using Excel VBA.
Random number generation is an important aspect of many fields, including finance, engineering, and science. It allows users to simulate real-world scenarios, test hypotheses, and make predictions about future outcomes. Excel VBA provides several ways to generate random numbers, including the use of built-in functions, such as the Rnd
function, and the creation of custom functions using VBA code.
The importance of random number generation cannot be overstated. It is a crucial component of many statistical and mathematical models, and is used in a wide range of applications, from financial modeling to scientific research. By using Excel VBA to generate random numbers, users can create more accurate and realistic models, and make better decisions based on their results.
In addition to its practical applications, random number generation is also an interesting topic from a theoretical perspective. The concept of randomness is complex and multifaceted, and has been the subject of much debate and research in the fields of mathematics and philosophy. By exploring the different ways to generate random numbers using Excel VBA, users can gain a deeper understanding of this concept and its many applications.
Introduction to Excel VBA Random Number Generation
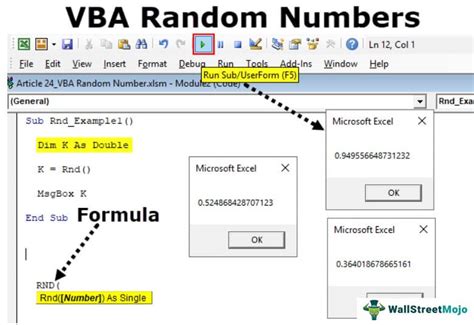
Excel VBA provides several ways to generate random numbers, including the use of built-in functions, such as the Rnd
function, and the creation of custom functions using VBA code. The Rnd
function is a simple and convenient way to generate random numbers, but it has some limitations, such as a limited range of values and a lack of control over the distribution of the numbers.
To generate random numbers using the Rnd
function, users can simply call the function and assign the result to a variable. For example: x = Rnd
. This will generate a random number between 0 and 1, which can then be used in calculations or assigned to a cell in the worksheet.
Using the Rnd Function
The `Rnd` function is a built-in function in Excel VBA that generates a random number between 0 and 1. To use the `Rnd` function, users can simply call the function and assign the result to a variable. For example: ```vb Sub GenerateRandomNumber() Dim x As Double x = Rnd Range("A1").Value = x End Sub ``` This code will generate a random number between 0 and 1 and assign it to cell A1 in the worksheet.Creating a Custom Random Number Generator
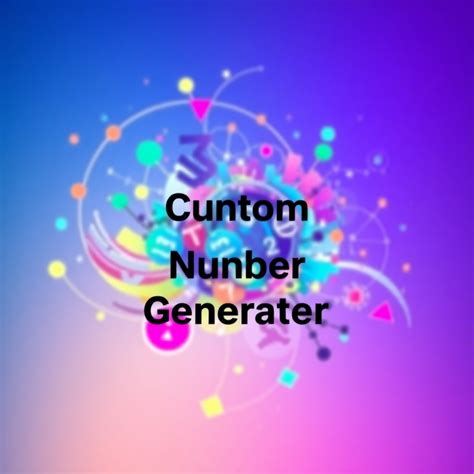
While the Rnd
function is a convenient way to generate random numbers, it has some limitations, such as a limited range of values and a lack of control over the distribution of the numbers. To overcome these limitations, users can create a custom random number generator using VBA code.
One way to create a custom random number generator is to use the Randomize
statement, which initializes the random number generator with a seed value. The Randomize
statement can be used in conjunction with the Rnd
function to generate a sequence of random numbers.
For example:
Sub GenerateRandomNumberSequence()
Dim x As Double
Randomize
For i = 1 To 10
x = Rnd
Range("A" & i).Value = x
Next i
End Sub
This code will generate a sequence of 10 random numbers and assign them to cells A1 through A10 in the worksheet.
Using a Loop to Generate Random Numbers
Another way to generate random numbers is to use a loop to iterate over a range of cells and assign a random number to each cell. For example: ```vb Sub GenerateRandomNumbers() Dim x As Double For Each cell In Range("A1:A10") x = Rnd cell.Value = x Next cell End Sub ``` This code will generate a random number for each cell in the range A1 through A10 and assign it to the corresponding cell.Advanced Random Number Generation Techniques
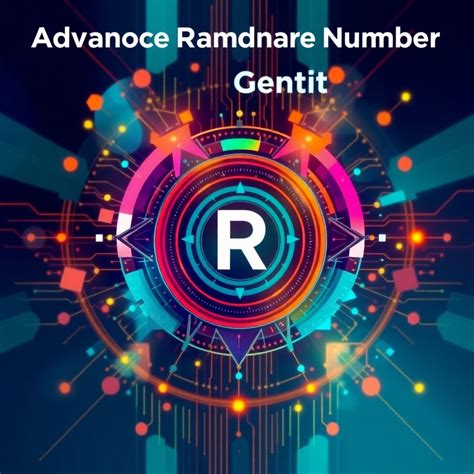
While the Rnd
function and custom random number generators are useful for generating random numbers, they have some limitations, such as a limited range of values and a lack of control over the distribution of the numbers. To overcome these limitations, users can use advanced random number generation techniques, such as the use of algorithms and statistical distributions.
One advanced technique is to use the Application.WorksheetFunction.Norm_Inv
function, which generates a random number from a normal distribution. For example:
Sub GenerateRandomNumberFromNormalDistribution()
Dim x As Double
x = Application.WorksheetFunction.Norm_Inv(Rnd, 0, 1)
Range("A1").Value = x
End Sub
This code will generate a random number from a normal distribution with a mean of 0 and a standard deviation of 1, and assign it to cell A1 in the worksheet.
Using Statistical Distributions to Generate Random Numbers
Another advanced technique is to use statistical distributions, such as the uniform distribution, the normal distribution, and the exponential distribution, to generate random numbers. For example: ```vb Sub GenerateRandomNumberFromUniformDistribution() Dim x As Double x = Application.WorksheetFunction.Uniform_Dist(Rnd, 0, 1) Range("A1").Value = x End Sub ``` This code will generate a random number from a uniform distribution between 0 and 1, and assign it to cell A1 in the worksheet.Random Number Generator Image Gallery
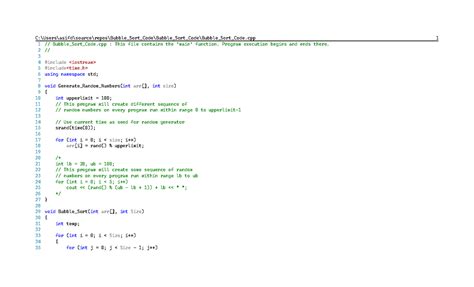
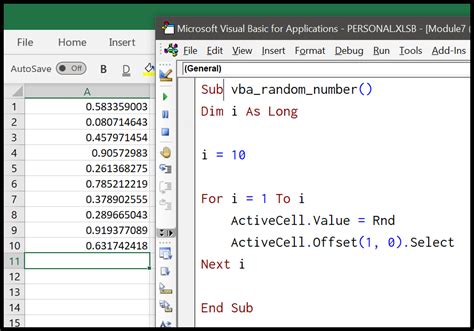
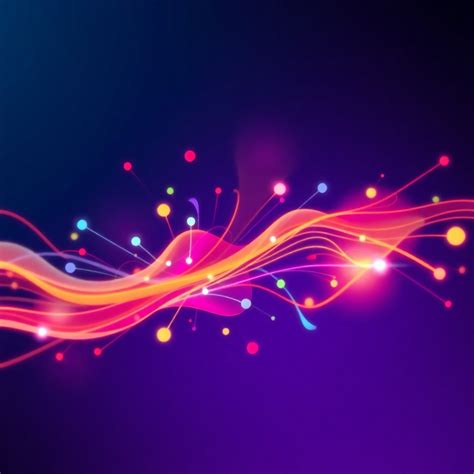
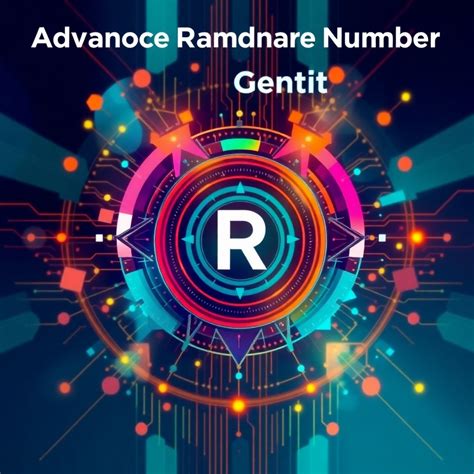
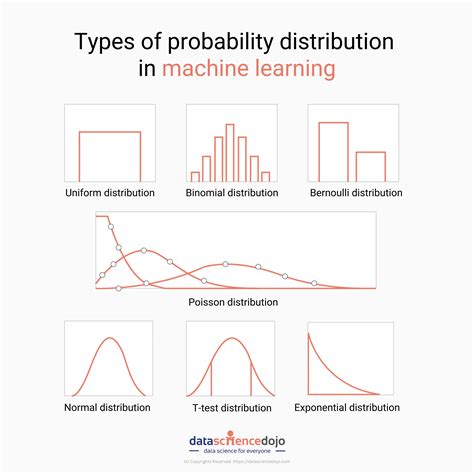
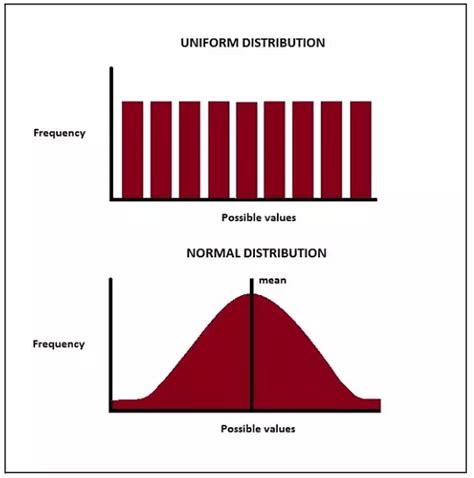
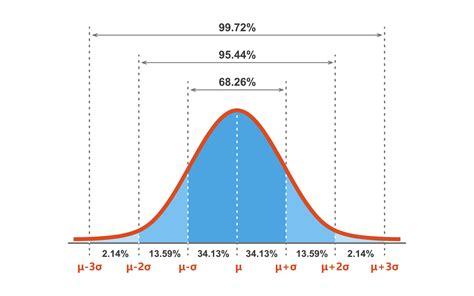
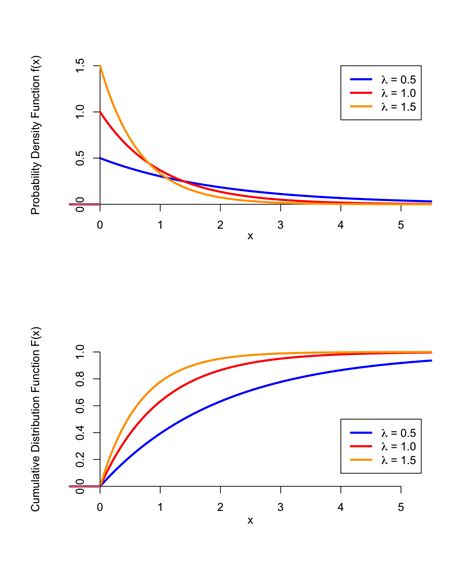
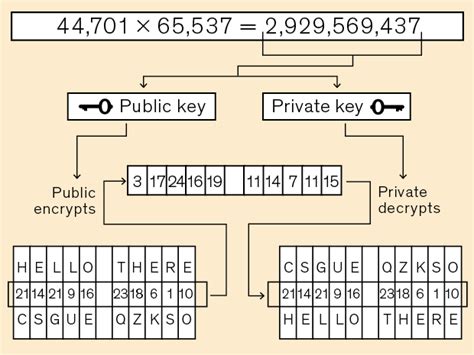
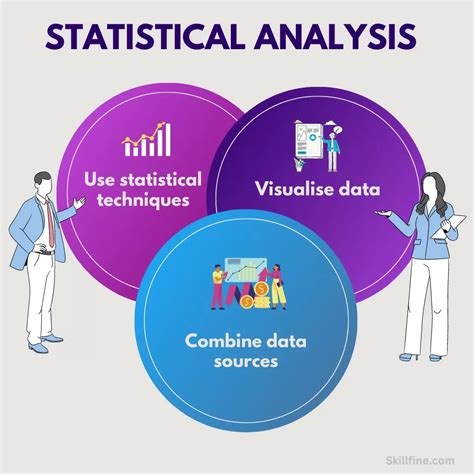
What is the purpose of random number generation in Excel VBA?
+The purpose of random number generation in Excel VBA is to simulate real-world scenarios, test hypotheses, and make predictions about future outcomes.
How do I generate a random number in Excel VBA?
+To generate a random number in Excel VBA, you can use the `Rnd` function or create a custom random number generator using VBA code.
What are some advanced techniques for generating random numbers in Excel VBA?
+Some advanced techniques for generating random numbers in Excel VBA include using statistical distributions, such as the uniform distribution, the normal distribution, and the exponential distribution, and using algorithms to generate random numbers.
How do I use the `Application.WorksheetFunction.Norm_Inv` function to generate a random number from a normal distribution?
+To use the `Application.WorksheetFunction.Norm_Inv` function to generate a random number from a normal distribution, you can call the function and pass in the `Rnd` function as an argument, along with the mean and standard deviation of the distribution.
What are some common applications of random number generation in Excel VBA?
+Some common applications of random number generation in Excel VBA include simulations, modeling, and analysis, as well as statistical analysis and data visualization.
We hope this article has provided you with a comprehensive overview of random number generation in Excel VBA. Whether you are a beginner or an experienced user, we encourage you to experiment with the different techniques and functions described in this article to see how they can be used to generate random numbers and simulate real-world scenarios. If you have any questions or need further assistance, please don't hesitate to comment below. Additionally, we invite you to share this article with others who may be interested in learning more about random number generation in Excel VBA. Thank you for reading!