Intro
Discover how to use Return Value in VBA effectively, leveraging functions, subroutines, and error handling to optimize code performance, debugging, and data analysis in Excel VBA programming.
When working with Visual Basic for Applications (VBA), understanding how to return values from functions and subroutines is crucial for effective programming. VBA is widely used in Microsoft Office applications such as Excel, Word, and Access for automating tasks, creating interactive forms, and developing custom tools. In this article, we'll delve into the world of VBA, focusing on how to return values from functions and subroutines, and explore best practices for using these programming constructs.
The importance of returning values in VBA cannot be overstated. It allows your code to communicate results back to the caller, enabling decision-making, data processing, and more complex program flows. Whether you're a beginner looking to learn the basics of VBA programming or an experienced developer seeking to refine your skills, understanding how to work with return values is essential.
VBA supports two primary types of procedures: Subroutines (Sub) and Functions (Function). Subroutines are used to perform actions and do not return values, whereas Functions are designed to compute and return a value. This distinction is fundamental to understanding how to structure your code effectively.
Subroutines in VBA
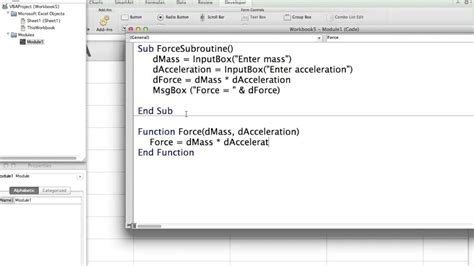
Subroutines are declared using the Sub
keyword followed by the name of the subroutine and optionally parameters in parentheses. They are useful for performing tasks that do not require returning a value, such as formatting a document, sending an email, or updating a database.
Sub SayHello()
MsgBox "Hello, World!"
End Sub
Functions in VBA
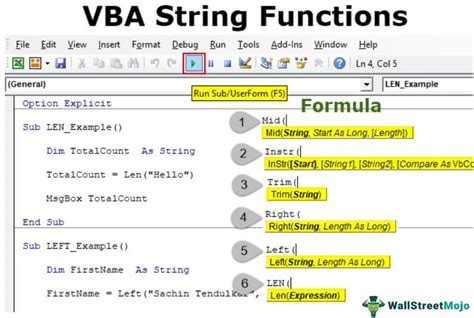
Functions, on the other hand, are declared using the Function
keyword and must include a return type. They are used to perform calculations or operations and return a result. The return value is assigned to the function name within the function body.
Function Add(x As Integer, y As Integer) As Integer
Add = x + y
End Function
Returning Values from Functions
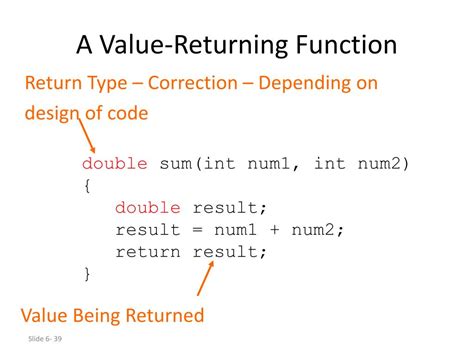
To return a value from a function, you assign the result to the function name. This value can then be used by the caller. For example, if you have a function that calculates the area of a rectangle, you would assign the calculated area to the function name.
Function CalculateArea(length As Double, width As Double) As Double
CalculateArea = length * width
End Function
Best Practices for Returning Values
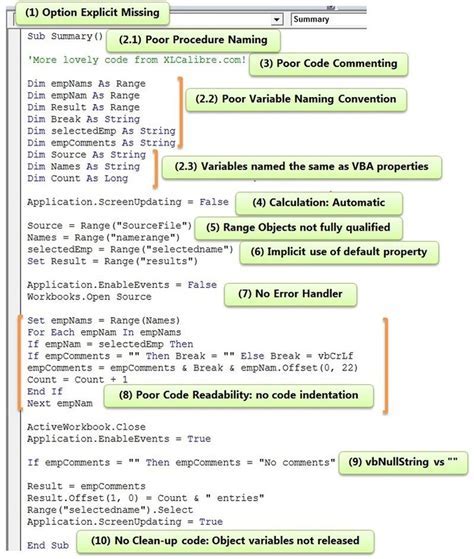
- Use Meaningful Names: Choose function and variable names that clearly indicate their purpose.
- Validate Inputs: Ensure that the inputs to your functions are valid to prevent errors.
- Handle Errors: Implement error handling to gracefully manage unexpected situations.
- Keep it Simple: Avoid complex functions; break them down into simpler, more manageable pieces.
Common Errors and Troubleshooting
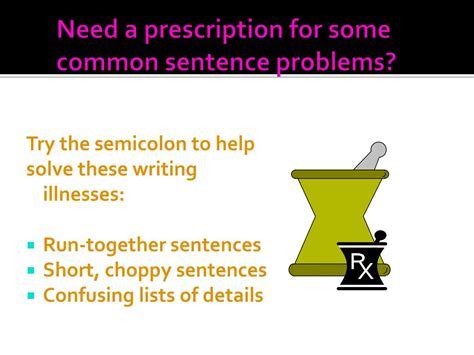
When working with return values in VBA, common issues include forgetting to assign a return value to the function name, using the wrong data type for the return value, or not handling errors properly. Troubleshooting involves checking the function's logic, verifying data types, and using VBA's built-in debugging tools.
Advanced Topics: Using Arrays and Objects as Return Values
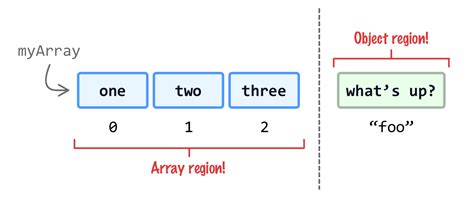
VBA also supports returning arrays and objects from functions, which can be incredibly powerful for complex data processing and manipulation.
Function GetNames() As Variant
Dim names(2) As String
names(0) = "John"
names(1) = "Mary"
names(2) = "David"
GetNames = names
End Function
Gallery of VBA Return Value Examples
VBA Return Value Examples
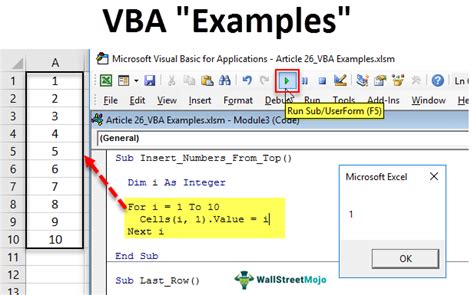
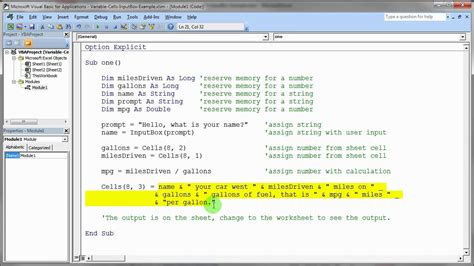

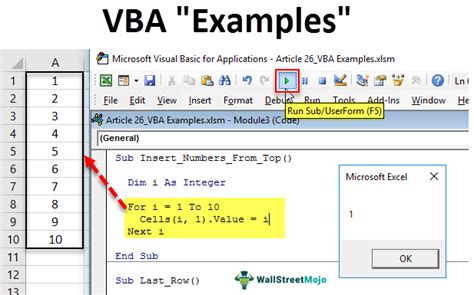
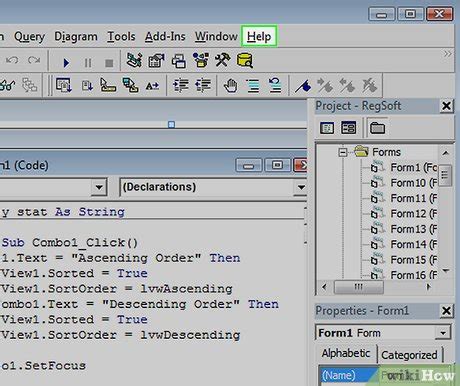
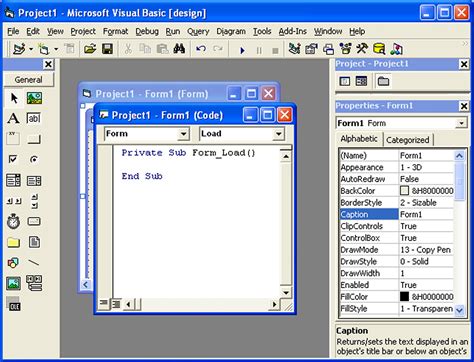
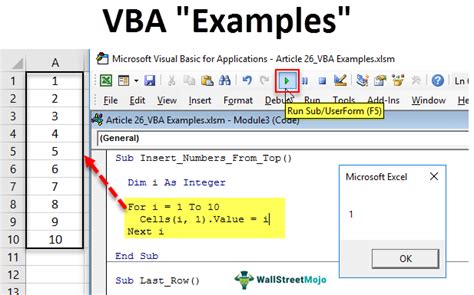
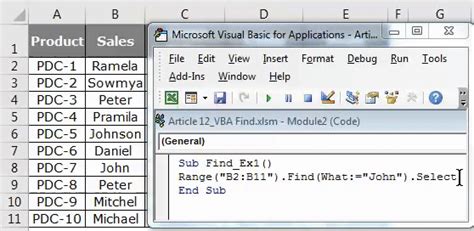
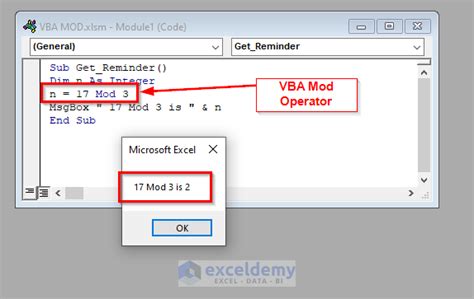
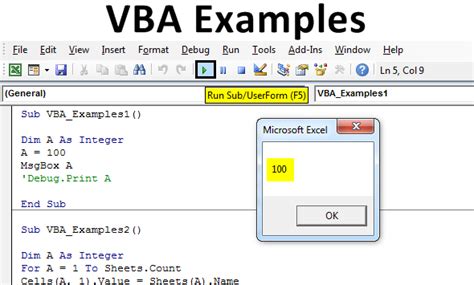
What is the difference between a Subroutine and a Function in VBA?
+A Subroutine performs actions and does not return a value, while a Function computes and returns a value.
How do you return a value from a Function in VBA?
+You assign the result to the function name within the function body.
Can Functions in VBA return arrays or objects?
+Yes, VBA functions can return arrays and objects, which is useful for complex data processing.
In conclusion, mastering the art of returning values in VBA is a skill that will greatly enhance your ability to create powerful, interactive, and dynamic applications within the Microsoft Office suite. By understanding the differences between Subroutines and Functions, learning how to effectively use return values, and applying best practices, you'll be well on your way to becoming a proficient VBA programmer. We invite you to share your experiences, ask questions, or provide feedback on this topic, and don't forget to explore more resources and tutorials to further your VBA programming journey.