Intro
Learn VBA to create new worksheets efficiently. Master Excel VBA programming with worksheet creation techniques, macros, and automation.
Visual Basic for Applications (VBA) is a powerful tool for automating tasks in Microsoft Excel. One common task that users need to perform is creating a new worksheet. In this article, we will explore how to create a new worksheet using VBA.
Creating a new worksheet can be useful in a variety of situations, such as when you need to add a new data set to an existing workbook or when you want to create a template for future use. VBA provides a simple and efficient way to create new worksheets, and we will cover the basics of how to do so.
Why Create a New Worksheet with VBA?
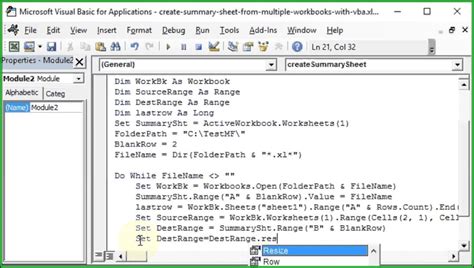
There are several reasons why you might want to create a new worksheet using VBA. For one, it can save you time and effort by automating the process of creating a new worksheet. Additionally, VBA can be used to create multiple worksheets at once, which can be useful if you need to create a large number of worksheets for a project.
Another benefit of using VBA to create new worksheets is that it allows you to customize the creation process. For example, you can use VBA to specify the name of the new worksheet, the location of the worksheet in the workbook, and even the formatting of the worksheet.
How to Create a New Worksheet with VBA
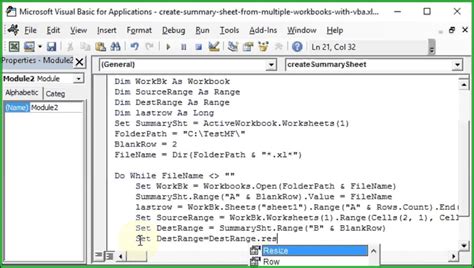
To create a new worksheet using VBA, you will need to use the Worksheets.Add
method. This method allows you to specify the location of the new worksheet in the workbook, as well as the type of worksheet to create.
Here is an example of how to use the Worksheets.Add
method to create a new worksheet:
Sub CreateNewWorksheet()
Worksheets.Add After:=Worksheets(Worksheets.Count)
End Sub
This code will create a new worksheet and add it to the end of the workbook.
Specifying the Location of the New Worksheet

You can specify the location of the new worksheet by using the After
or Before
argument of the Worksheets.Add
method. For example, to add a new worksheet after the first worksheet in the workbook, you can use the following code:
Sub CreateNewWorksheet()
Worksheets.Add After:=Worksheets(1)
End Sub
This code will create a new worksheet and add it after the first worksheet in the workbook.
Specifying the Name of the New Worksheet
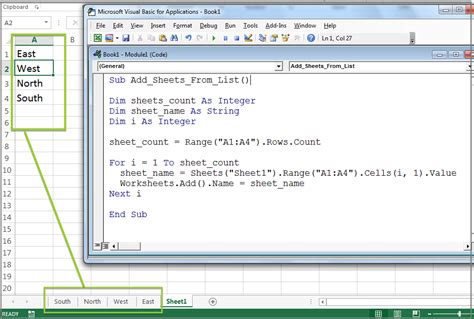
You can specify the name of the new worksheet by using the Name
property of the Worksheet
object. For example, to create a new worksheet and name it "My New Worksheet", you can use the following code:
Sub CreateNewWorksheet()
Dim newWorksheet As Worksheet
Set newWorksheet = Worksheets.Add(After:=Worksheets(Worksheets.Count))
newWorksheet.Name = "My New Worksheet"
End Sub
This code will create a new worksheet, add it to the end of the workbook, and name it "My New Worksheet".
Best Practices for Creating New Worksheets with VBA
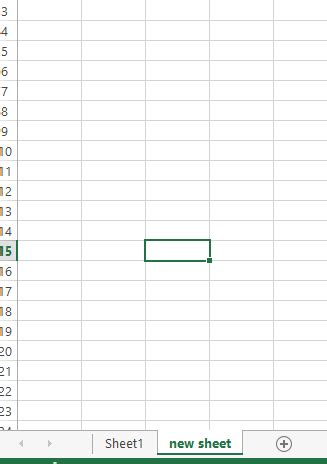
Here are some best practices to keep in mind when creating new worksheets with VBA:
- Always specify the location of the new worksheet using the
After
orBefore
argument of theWorksheets.Add
method. - Use the
Name
property of theWorksheet
object to specify the name of the new worksheet. - Avoid using the
ActiveSheet
property to refer to the new worksheet, as this can cause problems if the user has changed the active sheet. - Use the
Worksheets
collection to refer to the new worksheet, rather than using theActiveSheet
property.
Common Errors When Creating New Worksheets with VBA
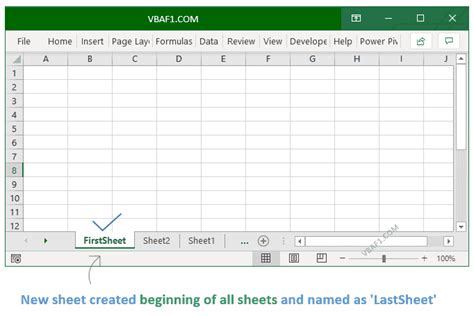
Here are some common errors that can occur when creating new worksheets with VBA:
- Forgetting to specify the location of the new worksheet using the
After
orBefore
argument of theWorksheets.Add
method. - Using the
ActiveSheet
property to refer to the new worksheet, rather than using theWorksheets
collection. - Not specifying the name of the new worksheet using the
Name
property of theWorksheet
object.
VBA Create New Worksheet Image Gallery
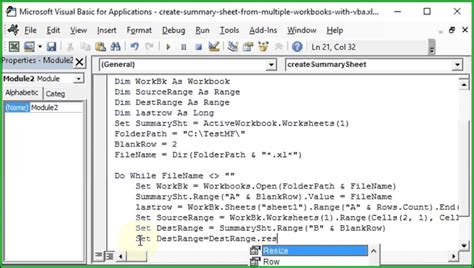
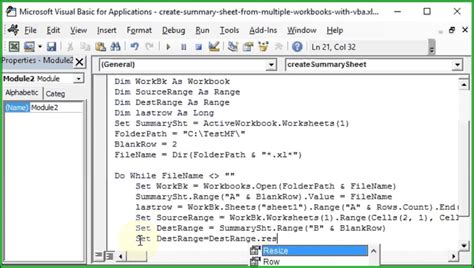
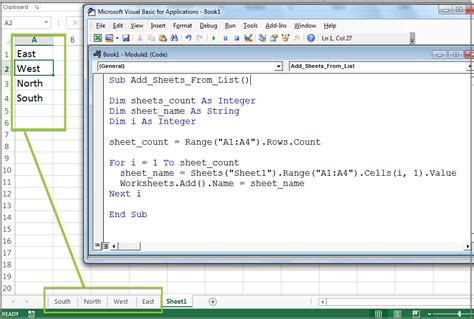
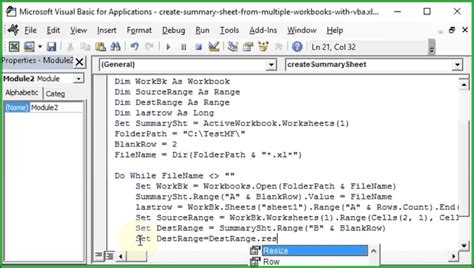
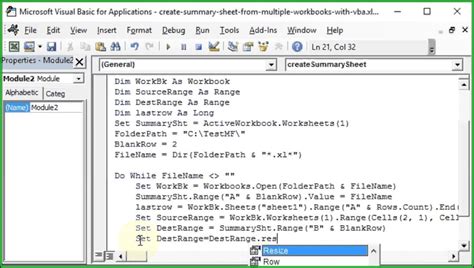
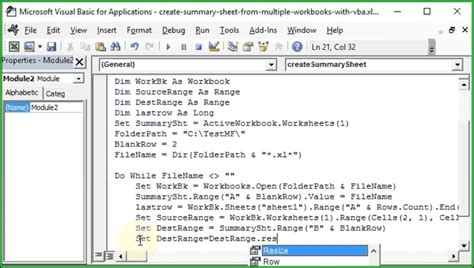
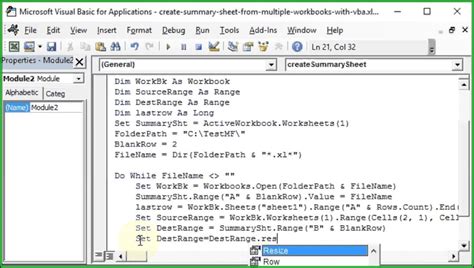
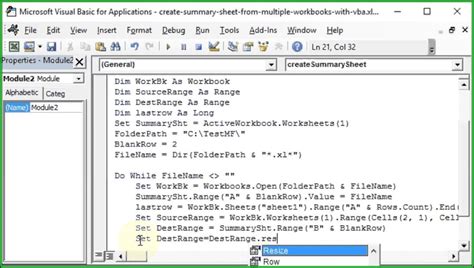
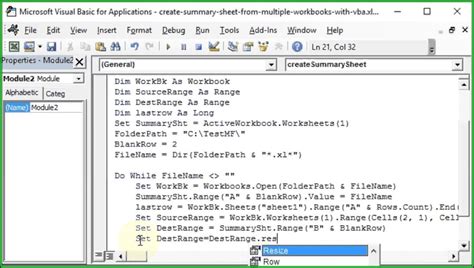
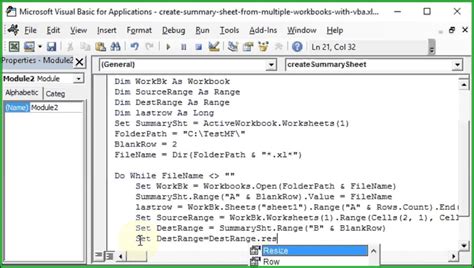
What is the purpose of creating a new worksheet with VBA?
+The purpose of creating a new worksheet with VBA is to automate the process of creating a new worksheet, customize the creation process, and save time and effort.
How do I specify the location of the new worksheet using VBA?
+You can specify the location of the new worksheet using the `After` or `Before` argument of the `Worksheets.Add` method.
What are some best practices for creating new worksheets with VBA?
+Some best practices for creating new worksheets with VBA include specifying the location of the new worksheet, using the `Name` property to specify the name of the new worksheet, and avoiding the use of the `ActiveSheet` property.
In conclusion, creating a new worksheet with VBA is a powerful tool that can save you time and effort. By following the best practices outlined in this article, you can ensure that your VBA code is efficient, effective, and easy to maintain. Whether you are a beginner or an experienced VBA programmer, this article has provided you with the knowledge and skills you need to create new worksheets with confidence. So why not give it a try? Start creating new worksheets with VBA today and see the difference it can make in your work. We invite you to share your thoughts, ask questions, and provide feedback on this article. Your input is valuable to us, and we look forward to hearing from you.