Intro
The world of Visual Basic for Applications (VBA) can be intimidating, but with the right guidance, it can also be incredibly empowering. One of the most basic yet essential tasks in VBA is creating a new sheet in an Excel workbook. This process can be automated, making it easier to manage and organize your worksheets. In this article, we will delve into the details of how to create a new sheet easily using VBA, exploring its importance, the steps involved, and providing examples to get you started.
Creating new sheets in Excel is a fundamental operation that can be performed manually by clicking on the "Insert" tab and then selecting "Sheet." However, when dealing with large workbooks or repetitive tasks, automating this process with VBA can save a significant amount of time. VBA allows you to create sheets with specific names, formats, and even content, all with just a few lines of code. This automation capability is what makes VBA so powerful for Excel users looking to streamline their workflow.
Before diving into the code, it's essential to understand the basics of VBA and how to access the Visual Basic Editor in Excel. The Visual Basic Editor can be opened by pressing "Alt + F11" or by navigating to the "Developer" tab and clicking on "Visual Basic." If the Developer tab is not visible, you can enable it through Excel's settings. Once in the Visual Basic Editor, you can insert a new module to start writing your VBA code.
Getting Started with VBA
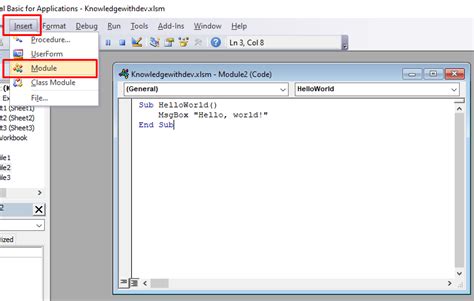
To create a new sheet using VBA, you will typically use the Worksheets.Add
method. This method allows you to add a new worksheet to the workbook. You can specify the position of the new sheet, the type of sheet (worksheet or chart sheet), and even the template to use for the new sheet. The basic syntax for adding a new worksheet is Worksheets.Add([Before], [After], [Count], [Type])
, where [Before]
and [After]
specify the relative position of the new sheet, [Count]
specifies the number of sheets to add, and [Type]
specifies the type of sheet.
Basic Syntax and Parameters
The parameters for the `Worksheets.Add` method give you flexibility in how you create new sheets. For example, you can insert a new sheet before or after an existing sheet, or you can add multiple sheets at once. Understanding these parameters is crucial for effectively using VBA to manage your worksheets.Practical Examples of Creating New Sheets
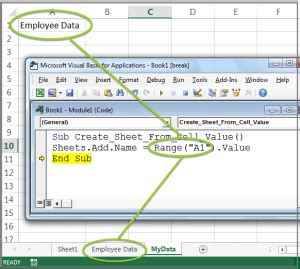
Let's consider a few examples to illustrate how to create new sheets with VBA.
-
Adding a Single New Sheet: To add a single new sheet to your workbook, you can use the following code:
Sub AddNewSheet() Worksheets.Add End Sub
This code will add a new sheet after the last existing sheet in your workbook.
-
Specifying the Position of the New Sheet: If you want to insert a new sheet before the first sheet, you can modify the code as follows:
Sub AddNewSheetBeforeFirst() Worksheets.Add Before:=Worksheets(1) End Sub
This will insert a new sheet before the first sheet in your workbook.
-
Adding Multiple New Sheets: You can also add multiple sheets at once by specifying the
[Count]
parameter:Sub AddMultipleNewSheets() Worksheets.Add Count:=5 End Sub
This code will add five new sheets after the last existing sheet in your workbook.
Common Errors and Troubleshooting
When working with VBA, it's not uncommon to encounter errors. These can range from syntax errors in your code to runtime errors that occur when your code is executed. For example, attempting to add a sheet with a name that already exists in the workbook will result in an error. Understanding how to troubleshoot these errors is an essential part of working with VBA.Advanced Topics in Creating New Sheets
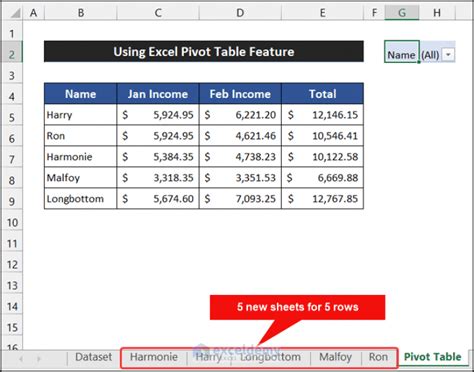
Beyond the basic creation of new sheets, VBA offers a wide range of advanced functionalities. You can rename sheets, change their colors, or even copy data from one sheet to another. These advanced operations can significantly enhance your workflow, especially when dealing with complex data analysis or report generation tasks.
For instance, renaming a newly created sheet can be achieved with the following code:
Sub RenameNewSheet()
Dim newSheet As Worksheet
Set newSheet = Worksheets.Add
newSheet.Name = "MyNewSheet"
End Sub
This code adds a new sheet and then renames it to "MyNewSheet."
Best Practices for VBA Coding
Following best practices when coding in VBA can make your code more readable, maintainable, and efficient. This includes using meaningful variable names, commenting your code, and structuring your macros in a logical manner. Adopting these practices will help you avoid common pitfalls and ensure that your VBA projects are successful.Gallery of VBA Examples
VBA Create New Sheet Easily Image Gallery
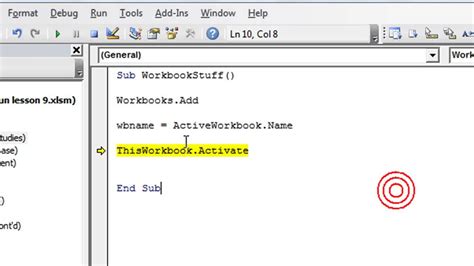
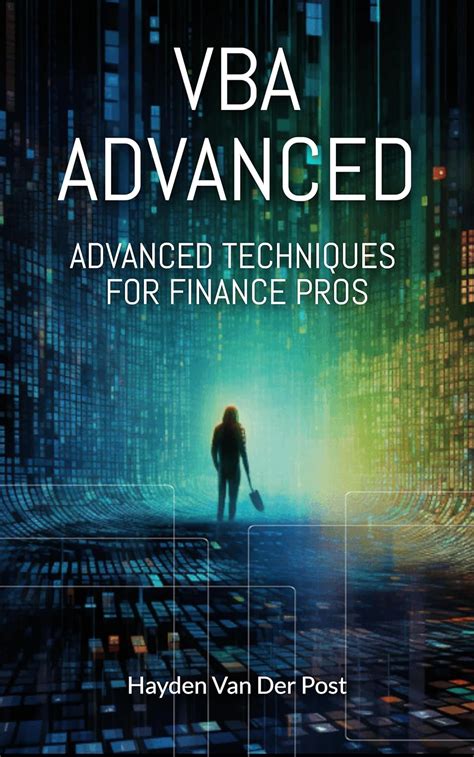
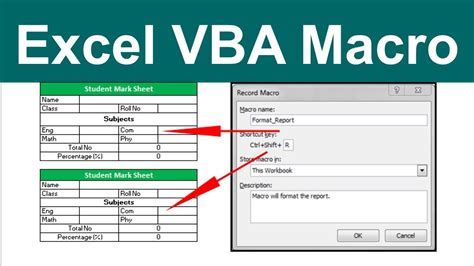
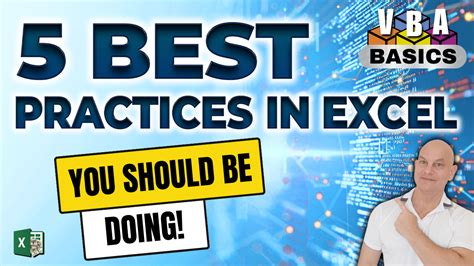
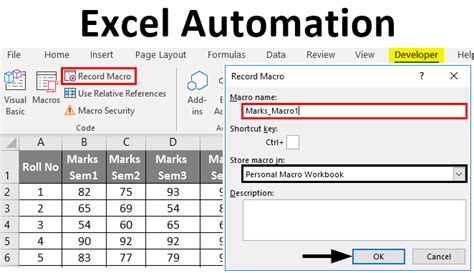
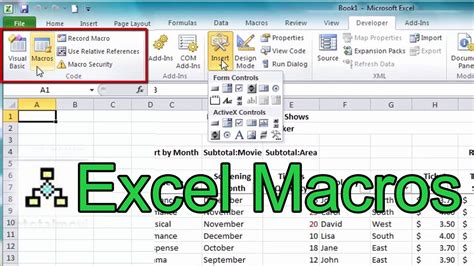
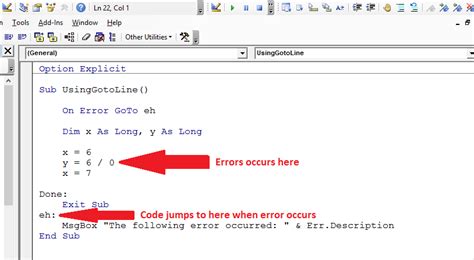
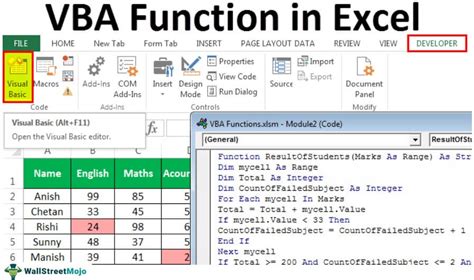
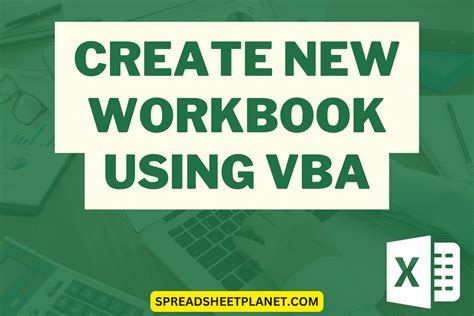
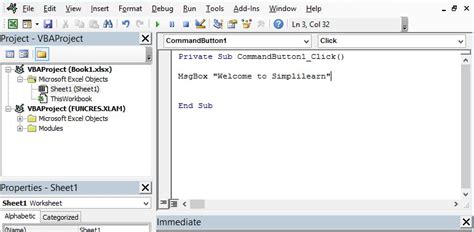
Frequently Asked Questions
What is VBA in Excel?
+VBA stands for Visual Basic for Applications. It's a programming language built into Excel that allows users to create and automate tasks, making it easier to manage and analyze data.
How do I open the Visual Basic Editor in Excel?
+You can open the Visual Basic Editor by pressing "Alt + F11" or by navigating to the "Developer" tab and clicking on "Visual Basic." If you don't see the Developer tab, you can enable it through Excel's settings.
What is the basic syntax for adding a new worksheet in VBA?
+The basic syntax is `Worksheets.Add([Before], [After], [Count], [Type])`. This method allows you to specify the position and type of the new sheet, as well as how many sheets to add.
How can I rename a newly created sheet in VBA?
+You can rename a sheet by setting the `Name` property of the worksheet object. For example, `newSheet.Name = "MyNewSheet"` will rename the newly created sheet to "MyNewSheet".
What are some best practices for coding in VBA?
+Best practices include using meaningful variable names, commenting your code, and structuring your macros in a logical and organized manner. This makes your code more readable, maintainable, and efficient.
In conclusion, creating new sheets in Excel using VBA is a powerful tool for automating tasks and enhancing productivity. By understanding the basics of VBA, the Worksheets.Add
method, and how to apply best practices in coding, you can unlock a wide range of possibilities for managing and analyzing data in Excel. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to develop complex applications, VBA offers the flexibility and functionality to meet your needs. We invite you to explore the world of VBA further, to share your experiences and tips with others, and to continue learning about the vast capabilities that VBA has to offer.