Intro
Unlock Excels full potential with VBA activation, enabling macros, and automating tasks with Visual Basic for Applications, enhancing spreadsheet functionality and efficiency.
Working with Excel sheets and Visual Basic for Applications (VBA) can significantly enhance your productivity and automate various tasks. VBA is a powerful tool that allows you to create, edit, and manage Excel sheets programmatically. In this article, we will delve into the world of Excel VBA, exploring how to activate an Excel sheet using VBA, along with other essential aspects of working with Excel sheets and VBA.
When you work with Excel, you often find yourself navigating through multiple sheets within a workbook. Activating a specific sheet is crucial for performing operations on it, such as data entry, formatting, or running macros. VBA provides a straightforward way to activate Excel sheets, which can be particularly useful in macros that need to process data across multiple sheets.
To start working with VBA in Excel, you first need to access the Visual Basic Editor. This can be done by pressing Alt + F11
or by navigating to the Developer tab (if available) and clicking on Visual Basic. If the Developer tab is not visible, you can add it by going to File > Options > Customize Ribbon and checking the Developer checkbox.
Once in the Visual Basic Editor, you can insert a new module by right-clicking on any of the objects for your workbook in the Project Explorer, selecting Insert, and then Module. This action creates a new module where you can write your VBA code.
Activating an Excel Sheet with VBA
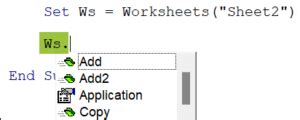
Activating a sheet in VBA is straightforward. You use the Activate
method on the Worksheet
object. Here's a basic example:
Sub ActivateSheet()
ThisWorkbook.Sheets("Sheet1").Activate
End Sub
In this example, replace "Sheet1"
with the name of the sheet you want to activate. This code will activate the specified sheet in the active workbook.
Working with Multiple Sheets
When working with multiple sheets, it's often necessary to loop through all sheets in a workbook or to activate a specific sheet based on certain conditions. Here's how you can loop through all sheets:Sub LoopThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Activate
' Perform actions on the active sheet
MsgBox ws.Name
Next ws
End Sub
This code loops through each sheet in the workbook, activates it, and then displays a message box with the name of the active sheet.
Best Practices for Working with Excel Sheets and VBA
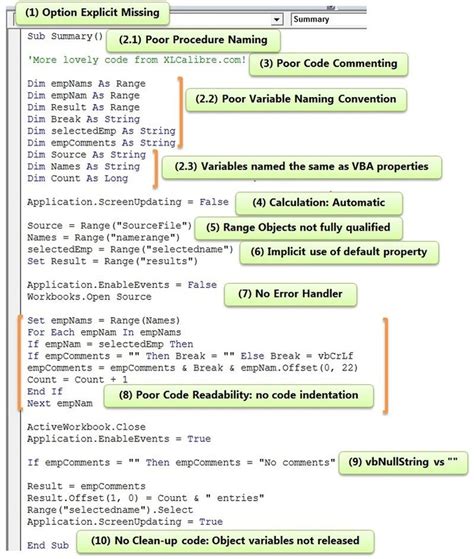
-
Use Meaningful Variable Names: When declaring variables, use names that describe what the variable represents. This improves the readability and maintainability of your code.
-
Comment Your Code: Comments help explain what your code is doing, which is especially useful for complex operations or when someone else needs to understand your code.
-
Error Handling: Implement error handling to manage potential errors that might occur during the execution of your macros. This can be done using
On Error
statements. -
Optimize Performance: When working with large datasets, optimize your code for performance. This can include avoiding unnecessary loops, using
Application.ScreenUpdating = False
to prevent screen updates during macro execution, and more.
Common VBA Errors and Solutions
-
Runtime Error 1004: This error often occurs when Excel is unable to perform an operation, such as when trying to activate a sheet that does not exist. Ensure that the sheet name is correct and the sheet exists in the workbook.
-
Compile Error: This type of error occurs when there's a syntax mistake in your VBA code. Check your code for any typos or missing elements.
Advanced VBA Techniques for Excel Sheets
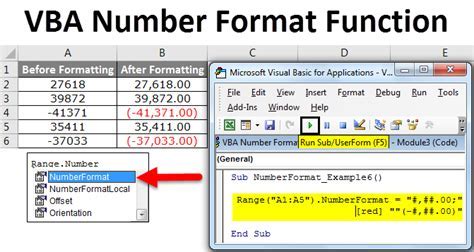
For more complex tasks, you might need to delve into advanced VBA techniques, such as working with arrays, using advanced looping methods, or interacting with other Office applications.
-
Working with Arrays: Arrays can significantly improve the performance of your VBA code by reducing the need to interact with the Excel worksheet directly. You can load data into an array, perform operations on the array, and then output the results back to the worksheet.
-
User Forms: For more interactive applications, consider using User Forms. These allow you to create custom interfaces for your macros, making them more user-friendly.
Learning More About VBA
-
Online Resources: There are numerous online resources, including tutorials, forums, and blogs, dedicated to learning VBA. Sites like MSDN, Excel-Easy, and Mr. Excel are invaluable for both beginners and advanced users.
-
Books: For a more structured learning approach, consider purchasing books on VBA programming. These can provide comprehensive guides and examples to help you master VBA.
Gallery of Excel VBA Examples
Excel VBA Image Gallery
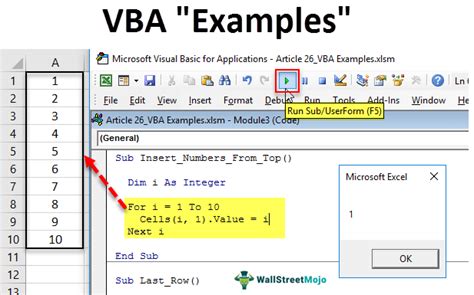
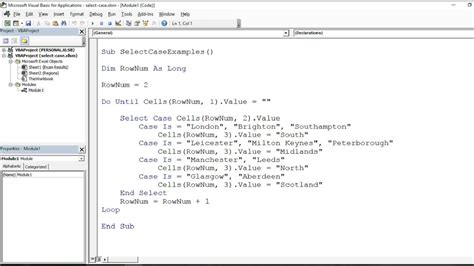
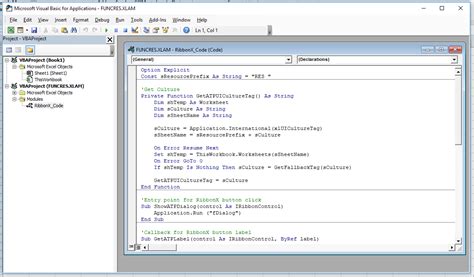
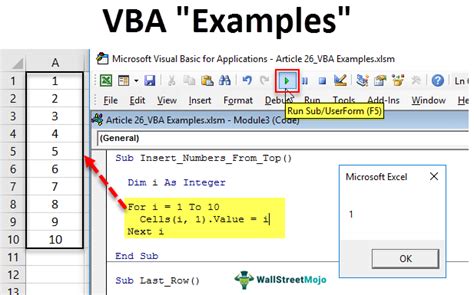
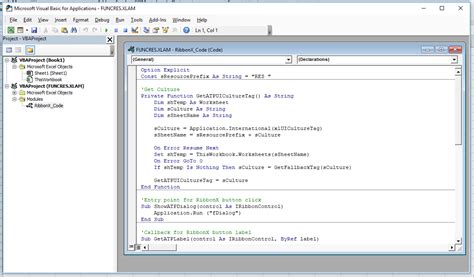
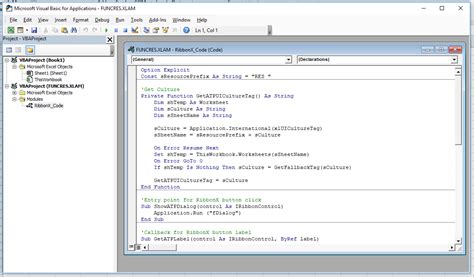
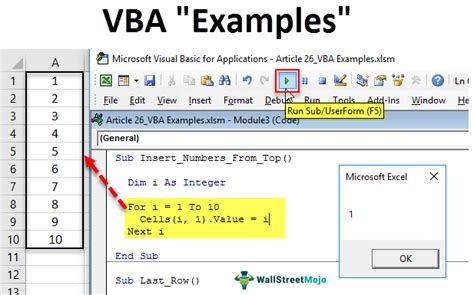
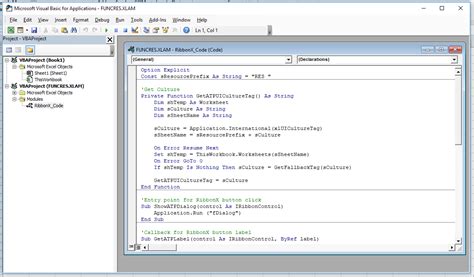
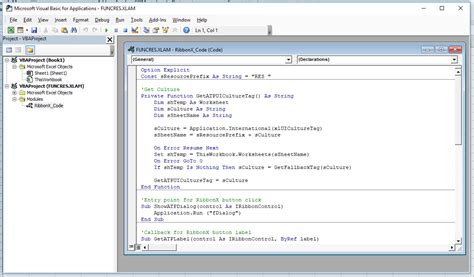
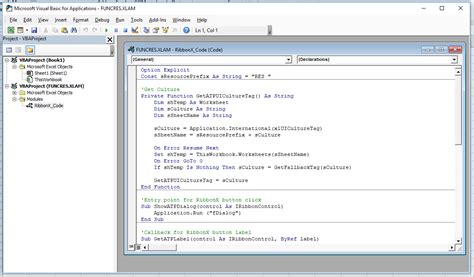
Frequently Asked Questions
What is VBA in Excel?
+VBA stands for Visual Basic for Applications. It's a programming language used for creating and automating tasks in Microsoft Excel and other Office applications.
How do I activate a sheet in Excel using VBA?
+You can activate a sheet by using the `Activate` method on the `Worksheet` object, such as `ThisWorkbook.Sheets("Sheet1").Activate`.
What are some best practices for writing VBA code?
+Best practices include using meaningful variable names, commenting your code, implementing error handling, and optimizing performance.
As you continue on your journey to master Excel VBA, remember that practice and experimentation are key. Start with simple macros and gradually move on to more complex projects. The ability to automate tasks and create interactive applications will not only save you time but also open up new possibilities for data analysis and presentation. Share your experiences, ask questions, and explore the vast community of VBA developers to further enhance your skills. Whether you're automating routine tasks or creating sophisticated applications, VBA is an indispensable tool for anyone looking to get the most out of Excel.