Intro
Learn to copy range to another sheet in VBA Excel using macros, worksheets, and workbooks, with Excel VBA coding techniques and range transfer methods.
The ability to copy a range of cells from one sheet to another is a fundamental skill in Excel VBA programming. This operation can be useful in a variety of situations, such as when you need to transfer data from a source sheet to a target sheet for further processing, analysis, or reporting. In this article, we'll delve into the details of how to accomplish this task using VBA.
To begin with, let's understand the basic syntax and structure of a VBA script that copies a range of cells from one worksheet to another. The core of this operation involves using the Range
object to specify the source and destination ranges, and then employing the Copy
method to transfer the data.
Basic Syntax
The basic syntax to copy a range from one sheet to another can be represented as follows:
Worksheets("SourceSheetName").Range("SourceRange").Copy Destination:=Worksheets("TargetSheetName").Range("TargetRange")
In this syntax:
SourceSheetName
is the name of the worksheet that contains the range you want to copy.SourceRange
specifies the range of cells you want to copy (e.g., "A1:B2").TargetSheetName
is the name of the worksheet where you want to paste the copied range.TargetRange
specifies the starting cell where the copied range will be pasted.
Example Usage
Let's say you have two worksheets named "Sheet1" and "Sheet2", and you want to copy the range "A1:B2" from "Sheet1" to "A3:B4" in "Sheet2". The VBA code would look like this:
Sub CopyRangeExample()
Worksheets("Sheet1").Range("A1:B2").Copy Destination:=Worksheets("Sheet2").Range("A3")
End Sub
Note that when specifying the destination, you only need to provide the top-left cell of the range where you want to paste the data. Excel will automatically adjust the paste area based on the size of the copied range.
Using Variables for Dynamic Ranges
In many cases, you might need to copy ranges dynamically based on certain conditions or user inputs. You can use variables to make your code more flexible. For example:
Sub CopyRangeDynamically()
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
Dim sourceRange As Range
Dim targetRange As Range
Set sourceSheet = Worksheets("Sheet1")
Set targetSheet = Worksheets("Sheet2")
' Define the source and target ranges dynamically
Set sourceRange = sourceSheet.Range("A1:B2")
Set targetRange = targetSheet.Range("A3")
sourceRange.Copy Destination:=targetRange
End Sub
Copying Without Using the Clipboard
Sometimes, using the Copy
method can be slow, especially with large datasets, because it involves the clipboard. An alternative approach is to use the Value
property to transfer data directly:
Sub CopyRangeWithoutClipboard()
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
Dim sourceRange As Range
Dim targetRange As Range
Set sourceSheet = Worksheets("Sheet1")
Set targetSheet = Worksheets("Sheet2")
Set sourceRange = sourceSheet.Range("A1:B2")
Set targetRange = targetSheet.Range("A3")
targetRange.Resize(sourceRange.Rows.Count, sourceRange.Columns.Count).Value = sourceRange.Value
End Sub
This method is generally faster and more efficient, especially for large ranges, since it bypasses the clipboard.
Handling Errors
When working with VBA, it's always a good practice to include error handling to ensure your code behaves predictably even when unexpected events occur. For example, you might want to check if the worksheets or ranges exist before attempting to copy:
Sub CopyRangeWithErrorHandling()
On Error GoTo ErrorHandler
' Your copy range code here
Worksheets("Sheet1").Range("A1:B2").Copy Destination:=Worksheets("Sheet2").Range("A3")
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
Conclusion and Next Steps
Copying ranges from one sheet to another is a basic yet powerful operation in Excel VBA. By mastering this skill, you can automate a wide range of tasks, from simple data transfers to complex data analysis and reporting workflows. Remember to always consider the size of your datasets and the performance implications of your code, opting for methods that bypass the clipboard when possible. As you continue to explore VBA, you'll find that understanding how to manipulate and analyze data programmatically opens up new possibilities for automating tasks and improving your productivity in Excel.
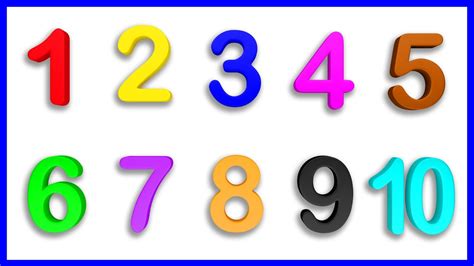
Advanced Copy Range Techniques
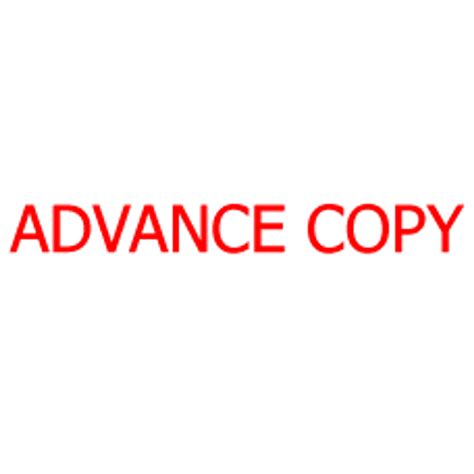
For more advanced scenarios, you might need to copy ranges based on specific conditions, such as copying only visible cells, or copying data from multiple worksheets into a single target sheet. These operations require a bit more sophistication in your VBA code but are entirely feasible with the right approach.
Copying Visible Cells Only
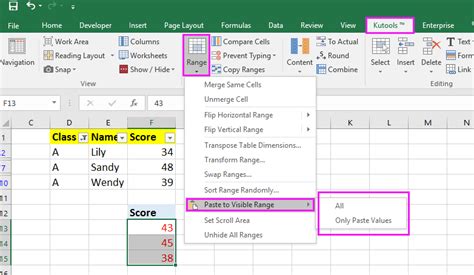
To copy only the visible cells in a range, you can use the SpecialCells
method with the xlCellTypeVisible
argument:
Sub CopyVisibleCells()
Worksheets("Sheet1").Range("A1:B2").SpecialCells(xlCellTypeVisible).Copy Destination:=Worksheets("Sheet2").Range("A3")
End Sub
Copying from Multiple Worksheets

Copying data from multiple worksheets into a single target sheet can be achieved by looping through each source worksheet and appending the data to the target range:
Sub CopyFromMultipleSheets()
Dim sourceSheet As Worksheet
Dim targetSheet As Worksheet
Dim lastRow As Long
Set targetSheet = Worksheets("TargetSheet")
lastRow = targetSheet.Cells(targetSheet.Rows.Count, "A").End(xlUp).Row + 1
For Each sourceSheet In ThisWorkbook.Worksheets
If sourceSheet.Name <> targetSheet.Name Then
sourceSheet.Range("A1:B2").Copy Destination:=targetSheet.Range("A" & lastRow)
lastRow = lastRow + sourceSheet.Range("A1:B2").Rows.Count
End If
Next sourceSheet
End Sub
Best Practices for Copying Ranges
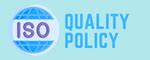
- Optimize Performance: For large datasets, consider using
Value
transfer instead of theCopy
method to improve performance. - Use Error Handling: Always include error handling to ensure your code can gracefully recover from unexpected errors.
- Specify Ranges Carefully: Be precise when defining source and target ranges to avoid overwriting unintended areas of your worksheets.
- Test Thoroughly: Before deploying your VBA scripts in production environments, test them extensively with various datasets and scenarios.
Copy Range Image Gallery
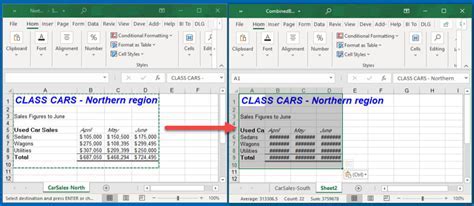
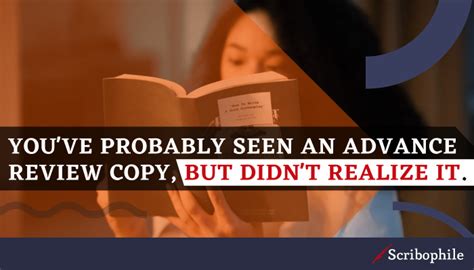
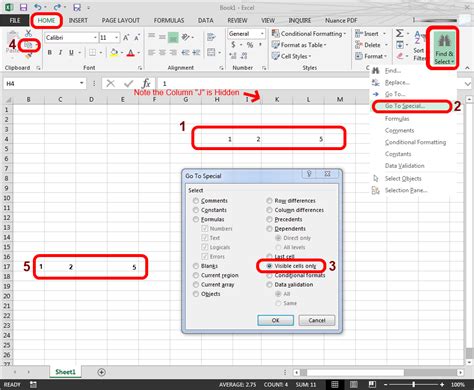
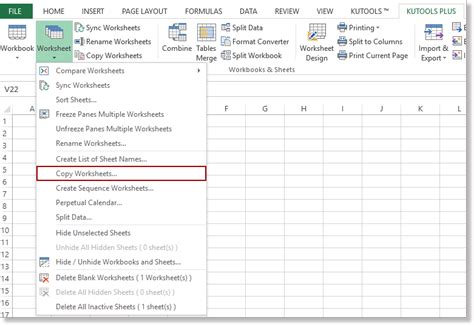
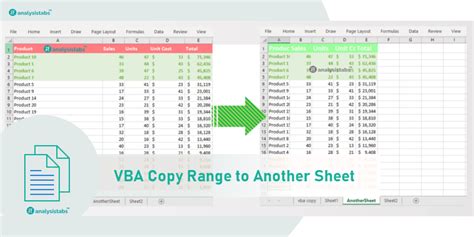
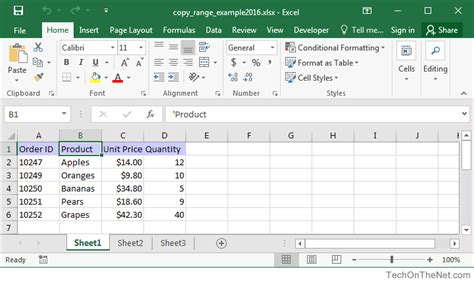
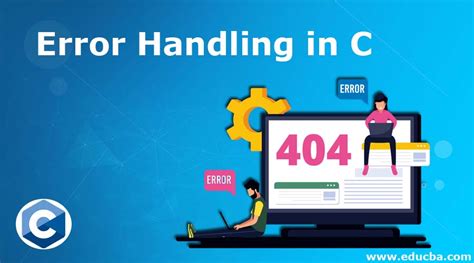
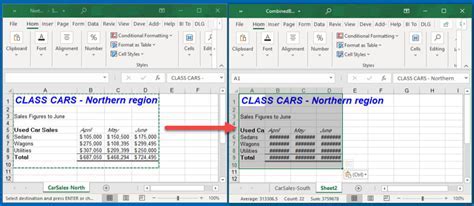
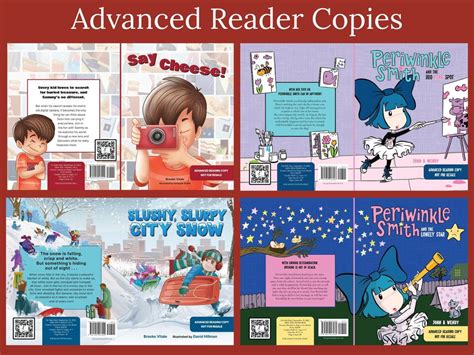
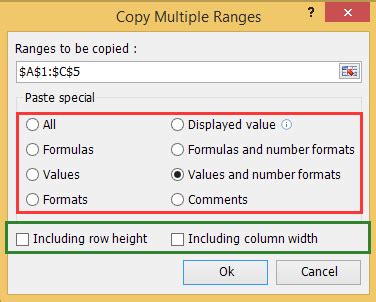
How do I copy a range from one sheet to another in Excel VBA?
+You can copy a range from one sheet to another using the `Range.Copy` method, specifying the source range, destination worksheet, and destination range. For example: `Worksheets("SourceSheet").Range("A1:B2").Copy Destination:=Worksheets("TargetSheet").Range("A3")`
What is the difference between using the `Copy` method and transferring values directly?
+The `Copy` method involves the clipboard and can be slower for large datasets. Transferring values directly using the `Value` property (e.g., `targetRange.Value = sourceRange.Value`) is generally faster and more efficient.
How can I copy only visible cells in a range?
+You can use the `SpecialCells` method with the `xlCellTypeVisible` argument to copy only visible cells. For example: `sourceRange.SpecialCells(xlCellTypeVisible).Copy Destination:=targetRange`
We hope this comprehensive guide has provided you with the knowledge and tools necessary to effectively copy ranges from one sheet to another in Excel VBA. Whether you're automating simple data transfers or complex data analysis tasks, mastering this skill will undoubtedly enhance your productivity and capabilities in Excel. Feel free to share your experiences, ask questions, or provide feedback in the comments section below.