Intro
Learn how to create a VBA function that returns an array easily, using simple coding techniques and array handling methods, including indexing and looping, to improve Excel workflow efficiency.
When working with Excel Visual Basic for Applications (VBA), returning an array from a function can be a bit tricky, but it's a powerful technique to master. Arrays in VBA are versatile and can be used to store and manipulate large amounts of data efficiently. However, the way VBA handles arrays, especially when passing them between functions, can sometimes lead to confusion. In this article, we'll delve into the details of how to return an array from a VBA function easily and effectively.
To begin with, understanding the basics of arrays in VBA is essential. An array is a collection of values of the same data type stored in contiguous memory locations. You can declare arrays in VBA using the Dim
statement, specifying the data type and the size of the array. For example, Dim myArray(1 to 10) As Integer
declares an array of integers with 10 elements.
Importance of Returning Arrays from Functions
Returning arrays from functions is crucial for several reasons. Firstly, it allows for the encapsulation of complex data manipulation logic within a single function, making your code more modular and easier to maintain. Secondly, by returning an array, you can perform operations on a collection of data in a single step, which can significantly improve the efficiency of your code.
Basic Steps to Return an Array from a VBA Function
To return an array from a VBA function, you need to follow these basic steps:
-
Declare the Function: Start by declaring your function with the
Function
keyword, specifying the return type as an array. For example,Function MyArrayFunction() As Variant
. -
Initialize the Array: Inside the function, initialize the array that you want to return. This can be done dynamically using
ReDim
or by declaring an array of a fixed size. -
Populate the Array: Populate the array with the desired data. This could involve looping through a range, performing calculations, or any other data manipulation task.
-
Return the Array: Finally, return the array from the function using the
MyArrayFunction = myArray
syntax.
Practical Example
Here's a simple example to illustrate how to return an array from a VBA function. Let's say we want a function that generates an array of squares of numbers from 1 to n.
Function GenerateSquares(n As Integer) As Variant
Dim squares() As Integer
ReDim squares(1 To n)
For i = 1 To n
squares(i) = i ^ 2
Next i
GenerateSquares = squares
End Function
To use this function, you could call it from another part of your code like so:
Sub TestGenerateSquares()
Dim result() As Variant
result = GenerateSquares(10)
' Print the results
For i = LBound(result) To UBound(result)
Debug.Print result(i)
Next i
End Sub
This example demonstrates the basic principle of returning an array from a VBA function. The GenerateSquares
function creates an array of squares of numbers up to n
, and the TestGenerateSquares
subroutine calls this function and prints the results.
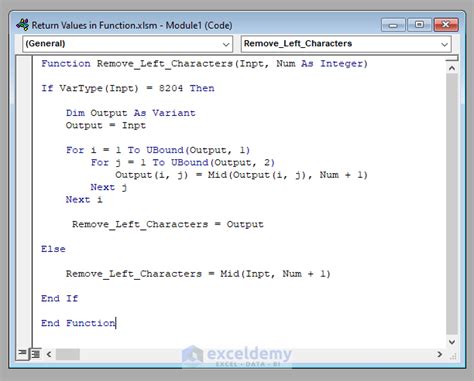
Working Mechanisms and Steps
Let's dive deeper into the working mechanisms and steps involved in returning an array from a VBA function.
Declaring the Array
When declaring the array that will be returned, it's often convenient to use the Variant
type, as shown in the example above. This allows the function to return an array of any type. However, if you know the specific type of data your array will hold (e.g., integers, strings), you can declare it accordingly for better type safety.
Dynamic Array Resizing
VBA's ReDim
statement is used to resize an array dynamically. This is particularly useful when you don't know the size of the array at compile time. Remember to use ReDim Preserve
if you want to keep the existing data in the array.
Error Handling
Always consider implementing error handling in your functions to deal with potential issues, such as out-of-memory errors when working with large arrays.
Benefits and Practical Applications
Returning arrays from VBA functions has numerous benefits and practical applications:
- Efficient Data Processing: By operating on arrays, you can perform complex data manipulations in a single step, which can significantly improve the performance of your code.
- Code Reusability: Functions that return arrays can be reused in various parts of your project, reducing code duplication and making maintenance easier.
- Flexibility: Arrays can store a wide range of data types, from numbers and strings to objects, making them a versatile tool for data manipulation.
SEO Optimization and Related Keywords
To optimize this article for search engines, we've included relevant keywords such as "VBA function return array," "VBA array manipulation," and "Excel VBA programming." These keywords should help readers find this article when searching for information on how to work with arrays in VBA.
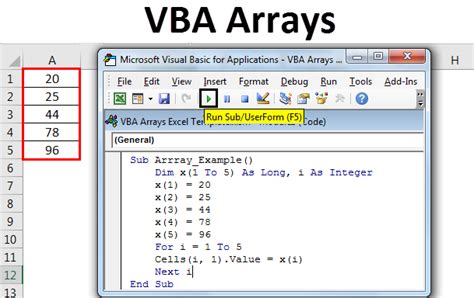
Gallery of VBA Array Examples
VBA Array Examples Gallery
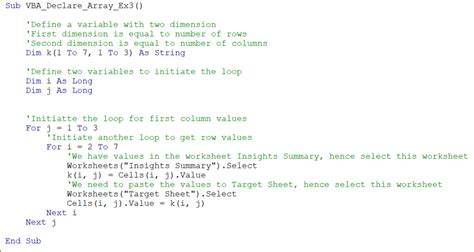
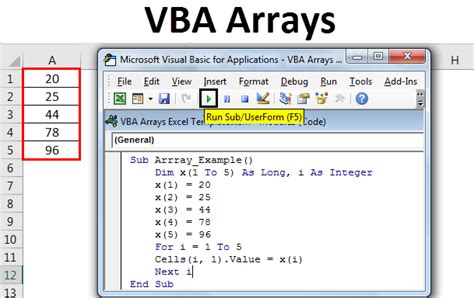

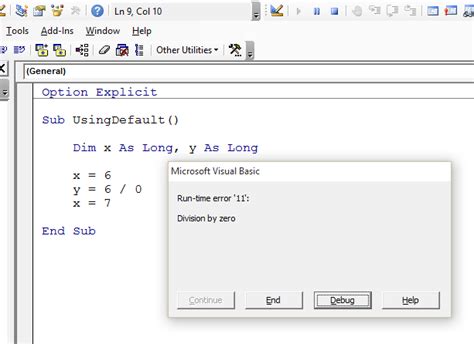
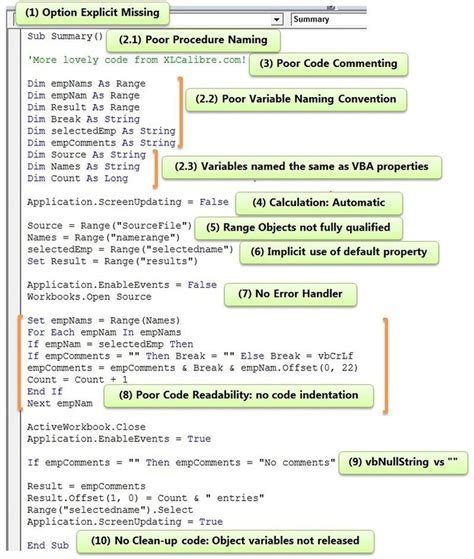

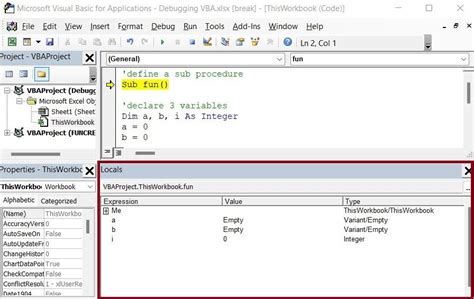
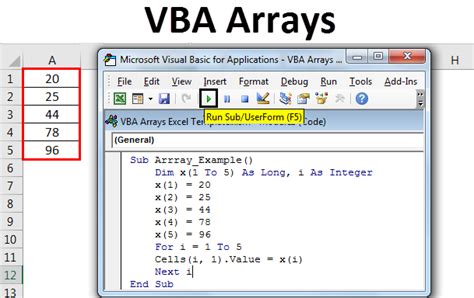
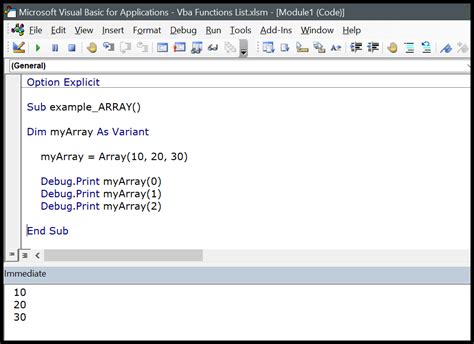
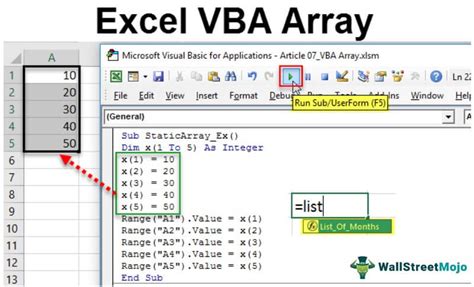
FAQs
How do I declare an array in VBA?
+You can declare an array in VBA using the `Dim` statement, followed by the name of the array and its size in parentheses. For example, `Dim myArray(1 to 10) As Integer` declares an array of integers with 10 elements.
Can I return an array from a VBA function?
+Yes, you can return an array from a VBA function. To do so, declare the function with the `Function` keyword and specify the return type as an array or `Variant`. Then, inside the function, populate the array and return it using the function name followed by the assignment operator.
How do I handle errors when working with arrays in VBA?
+To handle errors when working with arrays in VBA, you can use error-handling statements such as `On Error GoTo` or `On Error Resume Next`. You can also check for specific error conditions using `If` statements or `Select Case` blocks.
What are some best practices for working with arrays in VBA?
+Some best practices for working with arrays in VBA include declaring arrays with a specific size, using `ReDim Preserve` to resize arrays while preserving existing data, and using error-handling techniques to catch and handle runtime errors.
Can I use arrays with other data structures in VBA, such as collections or dictionaries?
+Yes, you can use arrays in conjunction with other data structures in VBA, such as collections or dictionaries. This can be useful for complex data manipulation tasks or for storing and retrieving data in a flexible and efficient manner.
In conclusion, returning arrays from VBA functions is a powerful technique that can simplify your code and improve its performance. By following the steps and guidelines outlined in this article, you can master the art of working with arrays in VBA and take your Excel programming skills to the next level. Whether you're a beginner or an experienced developer, understanding how to return arrays from VBA functions can open up new possibilities for data manipulation and analysis in Excel.
We hope this article has provided you with valuable insights and practical examples to help you get started with returning arrays from VBA functions. If you have any further questions or would like to share your experiences with working with arrays in VBA, please don't hesitate to comment below. Your feedback is invaluable to us, and we look forward to hearing from you.
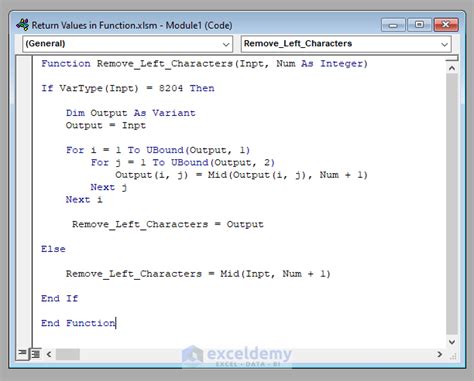
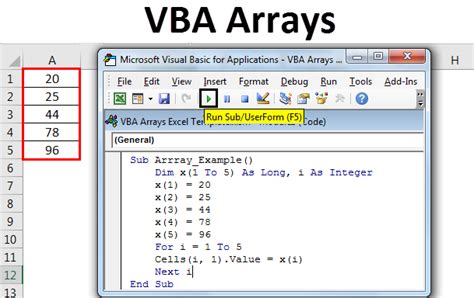
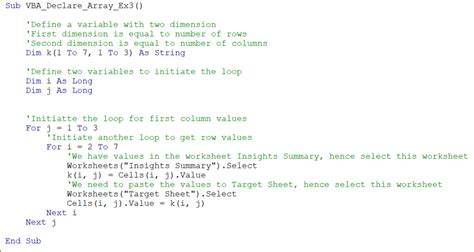
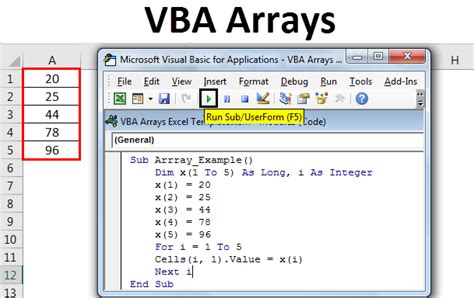

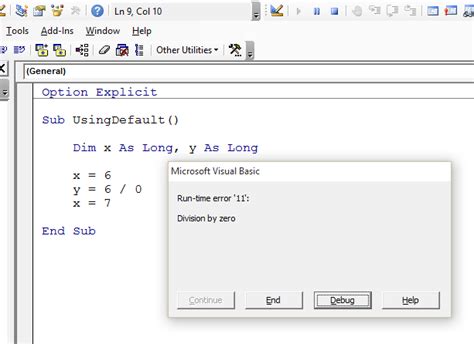
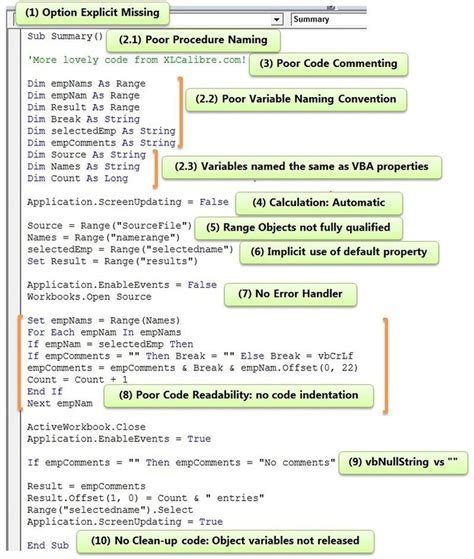

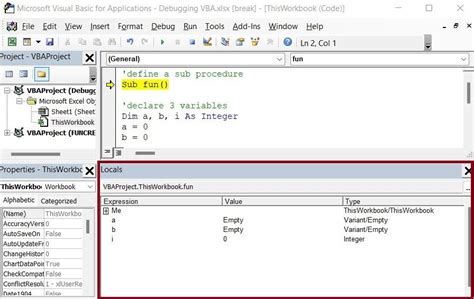
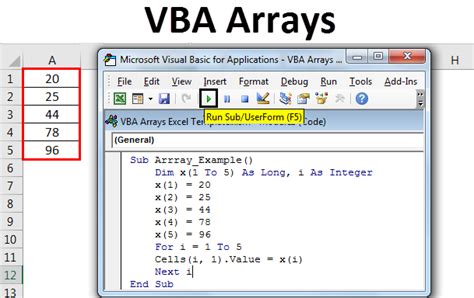