Intro
Master VBA return techniques with 5 expert tips, including error handling, function returns, and debugging, to optimize your Visual Basic for Applications code and improve productivity with efficient scripting and programming methods.
Visual Basic for Applications (VBA) is a powerful tool for automating tasks in Microsoft Office applications, such as Excel, Word, and Access. One of the key aspects of writing effective VBA code is understanding how to use the Return
statement to control the flow of your programs. In this article, we will delve into five VBA return tips that can help you improve your coding skills and make your VBA applications more efficient.
VBA is used by a wide range of professionals, from data analysts and accountants to software developers and IT professionals. Its versatility and ease of use make it an ideal tool for automating repetitive tasks, creating custom applications, and integrating with other Microsoft Office tools. However, like any programming language, VBA requires a good understanding of its syntax, structure, and best practices to write effective and efficient code.
The Return
statement in VBA is used to exit a function or subroutine and return control to the calling procedure. It can also be used to return a value from a function. Understanding how to use the Return
statement correctly is crucial for writing robust and reliable VBA code. In the following sections, we will explore five tips for using the Return
statement in VBA, along with examples and best practices to help you get the most out of your VBA applications.
Understanding the Basics of VBA Return
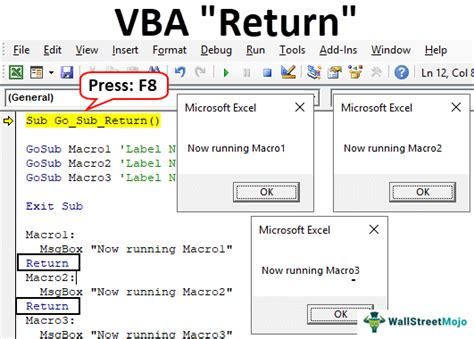
Example of VBA Return Basics
In this example, we have a simple function that calculates the sum of two numbers: ```vb Function AddNumbers(x As Integer, y As Integer) As Integer AddNumbers = x + y Return End Function ``` In this example, the `Return` statement is not strictly necessary, as the function will return automatically when it reaches the end. However, including the `Return` statement can make the code more readable and maintainable.Tip 1: Using Return to Exit a Subroutine
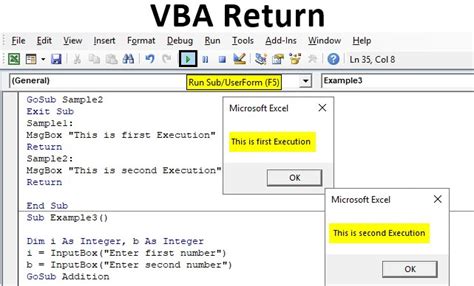
Example of Using Return to Exit a Subroutine
In this example, we have a subroutine that copies data from one worksheet to another: ```vb Sub CopyData() If WorksheetExists("Source") = False Then Return End If ' Copy data from Source worksheet to Destination worksheet End Sub ``` In this example, the `Return` statement is used to exit the subroutine if the "Source" worksheet does not exist.Tip 2: Using Return to Return a Value from a Function
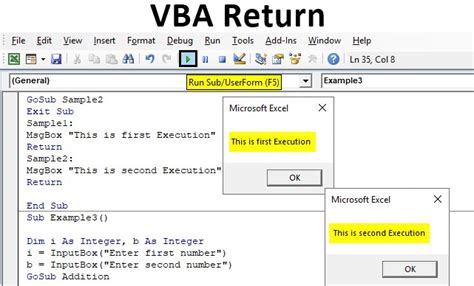
Example of Using Return to Return a Value from a Function
In this example, we have a function that calculates the area of a rectangle: ```vb Function CalculateArea(length As Integer, width As Integer) As Integer If length <= 0 Or width <= 0 Then CalculateArea = "Error: Invalid input" Return End If CalculateArea = length * width End Function ``` In this example, the `Return` statement is used to return an error message if the input values are invalid.Tip 3: Using Return to Handle Errors
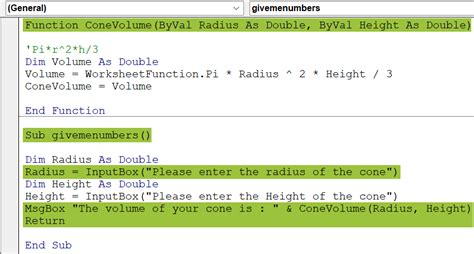
Example of Using Return to Handle Errors
In this example, we have a subroutine that opens a file and reads its contents: ```vb Sub OpenFile() On Error Resume Next Open "C:\example.txt" For Input As #1 If Err.Number <> 0 Then Return End If ' Read file contents End Sub ``` In this example, the `Return` statement is used to exit the subroutine if an error occurs when trying to open the file.Tip 4: Using Return to Improve Code Readability
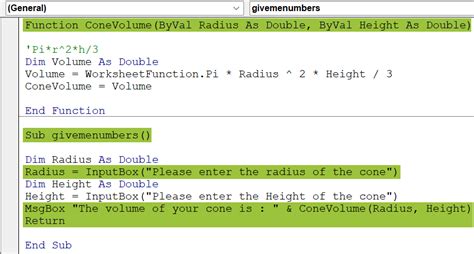
Example of Using Return to Improve Code Readability
In this example, we have a subroutine that performs a complex calculation: ```vb Sub CalculateResult() If inputValuesValid = False Then Return End If ' Perform calculation End Sub ``` In this example, the `Return` statement is used to exit the subroutine if the input values are invalid, making the code more readable and maintainable.Tip 5: Using Return to Reduce Code Duplication
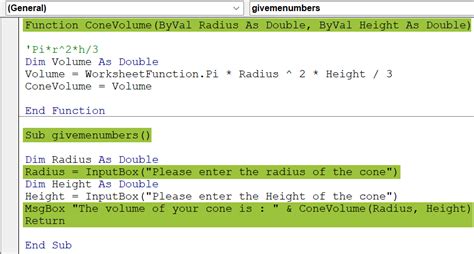
Example of Using Return to Reduce Code Duplication
In this example, we have a subroutine that performs a calculation and returns the result: ```vb Sub CalculateResult() If inputValuesValid = False Then Return End If ' Perform calculation result = CalculateResultHelper() Return End Sub ``` In this example, the `Return` statement is used to exit the subroutine and return the result, reducing the need for duplicated code.VBA Return Image Gallery
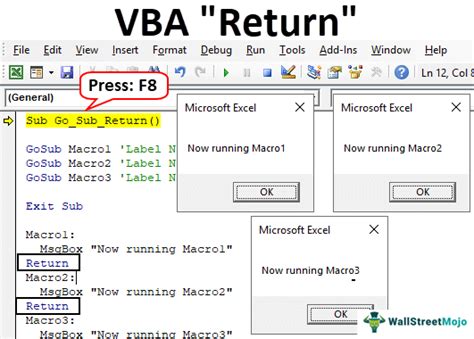
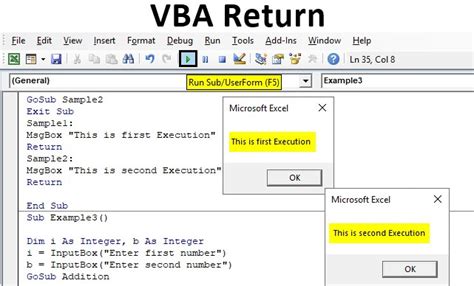
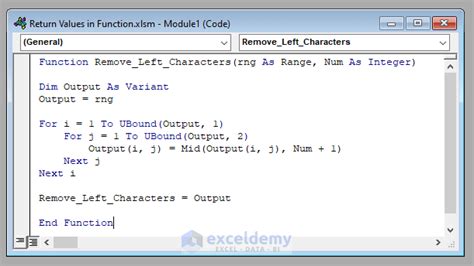
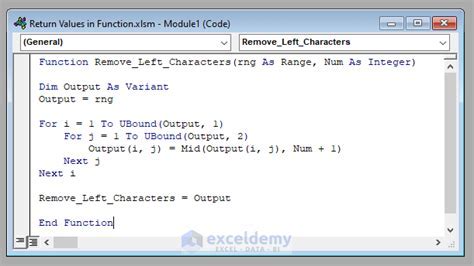
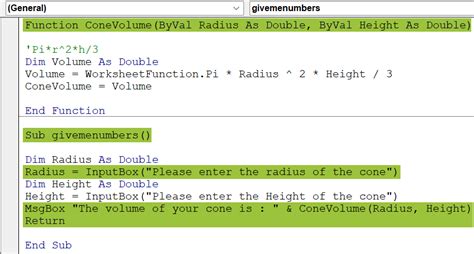
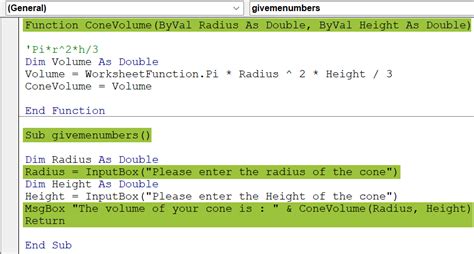
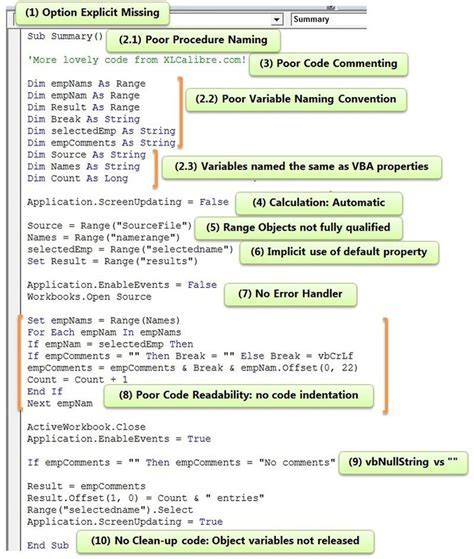
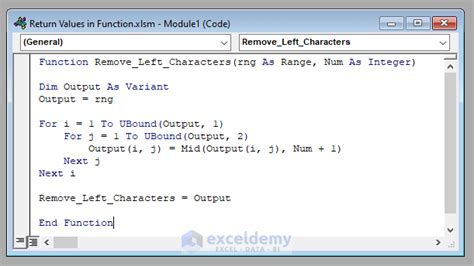
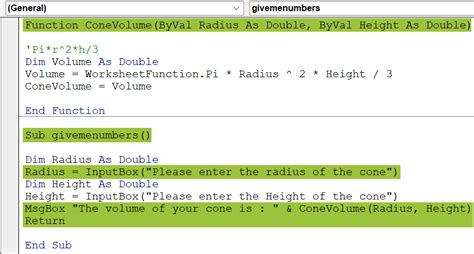
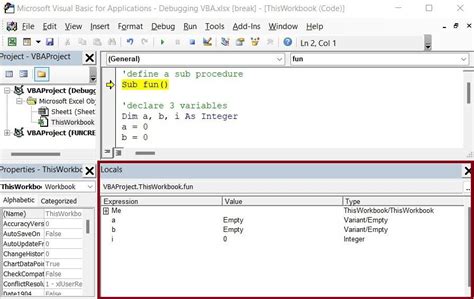
What is the purpose of the Return statement in VBA?
+The Return statement is used to exit a function or subroutine and return control to the calling procedure. It can also be used to return a value from a function.
How do I use the Return statement to handle errors in VBA?
+You can use the Return statement to exit a subroutine or function if an error occurs, for example, if a file cannot be opened or if a calculation fails.
Can I use the Return statement to improve code readability in VBA?
+Yes, you can use the Return statement to exit a subroutine or function and return control to the calling procedure, making your code more modular and easier to understand.
How do I use the Return statement to reduce code duplication in VBA?
+You can use the Return statement to exit a subroutine or function and return control to the calling procedure, reducing the need for duplicated code.
What are some best practices for using the Return statement in VBA?
+Some best practices for using the Return statement in VBA include using it to exit a subroutine or function and return control to the calling procedure, handling errors, improving code readability, and reducing code duplication.
In conclusion, the Return
statement is a powerful tool in VBA that can be used to control the flow of your programs, handle errors, improve code readability, and reduce code duplication. By following the five tips outlined in this article, you can write more efficient and effective VBA code. Whether you are a beginner or an experienced VBA programmer, understanding how to use the Return
statement correctly is essential for creating robust and reliable VBA applications. We hope this article has been helpful in providing you with the knowledge and skills you need to take your VBA programming to the next level. If you have any questions or comments, please don't hesitate to reach out. Share this article with your friends and colleagues who may be interested in learning more about VBA programming. Happy coding!