Intro
Boost productivity with VBA wait techniques, including delay, sleep, and pause functions, to optimize macros and automate tasks efficiently with VBA scripting and Excel automation.
The importance of timing in Visual Basic for Applications (VBA) cannot be overstated. VBA is a powerful tool used for creating and automating tasks in Microsoft Office applications, such as Excel, Word, and Outlook. One crucial aspect of VBA programming is the ability to control the flow of a program, including making it wait or pause for a specified period. This can be essential for ensuring that tasks are executed in the correct order, allowing for data to be updated, or simply giving the user time to review information before proceeding. Understanding how to make VBA wait is fundamental for any developer looking to create robust and user-friendly applications.
In many scenarios, a VBA script might need to pause to wait for user input, to allow another process to complete, or to introduce a delay for synchronization purposes. The ability to implement a wait function effectively can significantly enhance the functionality and usability of VBA applications. Moreover, mastering different wait techniques can help developers troubleshoot issues related to timing and synchronization, leading to more efficient and reliable code.
VBA offers several methods to achieve a wait or pause in a program. These methods vary in their implementation, advantages, and use cases, allowing developers to choose the most appropriate one based on their specific needs. From simple delays to more complex synchronization techniques, understanding the different ways to make VBA wait is crucial for creating sophisticated and interactive applications. Whether it's for educational purposes, professional development, or personal projects, learning about VBA wait functions can open up new possibilities for automation and customization within Microsoft Office.
Introduction to VBA Wait Functions
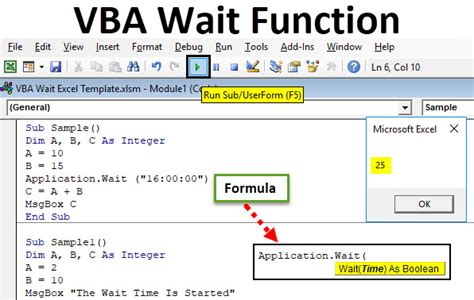
VBA wait functions are essential for controlling the execution flow of a program. They enable developers to introduce pauses or delays, ensuring that tasks are performed in a synchronized manner. The most common wait functions in VBA include the Sleep
function, Application.Wait
, Timer
function, using a loop to wait, and the DoEvents
function. Each of these methods has its unique characteristics and applications, making them suitable for different scenarios.
Understanding the Need for Wait Functions
The need for wait functions in VBA arises from the necessity to synchronize tasks, wait for user input, or allow sufficient time for other processes to complete. In a multitasking environment, where multiple applications and processes are running concurrently, timing becomes critical. Wait functions help in preventing conflicts, ensuring data integrity, and improving the overall user experience.1. Using the Sleep Function
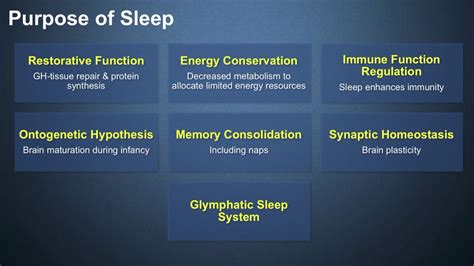
The Sleep
function is one of the most straightforward ways to introduce a delay in VBA. It pauses the execution of the current thread for a specified amount of time. The syntax for the Sleep
function is Sleep milliseconds
, where milliseconds
is the duration of the pause in milliseconds. For example, Sleep 1000
would pause the program for 1 second.
Sub WaitForSecond()
Sleep 1000
MsgBox "Waited for 1 second"
End Sub
Advantages and Limitations of Sleep
The `Sleep` function is simple to use and effective for introducing short delays. However, it can be less precise for longer delays due to the way Windows handles thread scheduling. Additionally, `Sleep` does not allow for other tasks to be performed during the wait period, which can be a limitation in some scenarios.2. Application.Wait Method
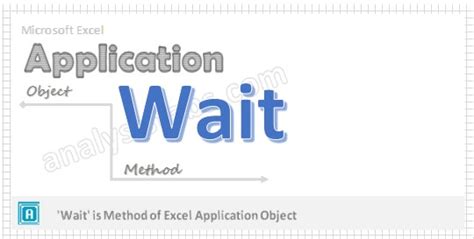
The Application.Wait
method is another way to pause a VBA program. It is specifically designed for Excel and allows the program to wait until a specified time. The syntax is Application.Wait Time
, where Time
is the time at which you want the program to continue. For example, Application.Wait (Now + TimeValue("0:00:05"))
would pause the program for 5 seconds.
Sub WaitFor5Seconds()
Application.Wait (Now + TimeValue("0:00:05"))
MsgBox "Waited for 5 seconds"
End Sub
Using Application.Wait for Synchronization
`Application.Wait` is particularly useful for synchronizing tasks with specific times or for waiting until a certain condition is met. It allows for other Excel tasks to be performed during the wait period, making it more flexible than the `Sleep` function in certain scenarios.3. Timer Function
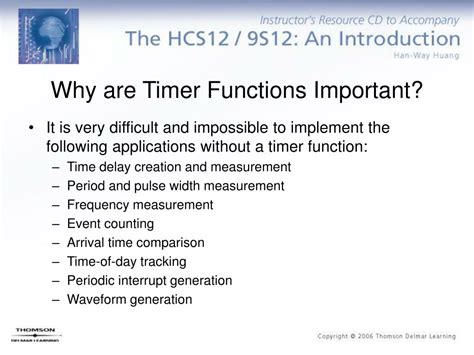
The Timer
function returns the number of seconds that have elapsed since midnight. It can be used to create a wait loop that pauses the program for a specified duration. The syntax involves recording the current time before the loop and then comparing it with the current time inside the loop until the desired delay has passed.
Sub WaitFor3SecondsUsingTimer()
Dim StartTime As Single
StartTime = Timer
Do While Timer < StartTime + 3
DoEvents
Loop
MsgBox "Waited for 3 seconds"
End Sub
Timer Function for Precise Delays
The `Timer` function provides a more precise way to introduce delays compared to `Sleep` and can be used in scenarios where exact timing is crucial. It also allows for other tasks to be processed during the wait period by incorporating `DoEvents`.4. Using a Loop to Wait
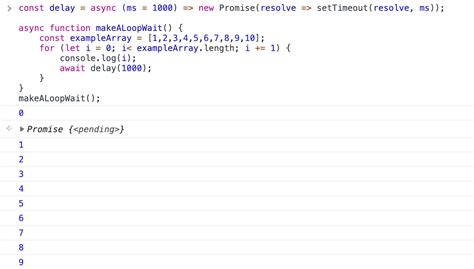
Another method to introduce a delay in VBA is by using a loop that runs for a specified number of iterations. This method is less accurate than using time-based waits but can be useful in certain situations.
Sub WaitForALittleWhile()
Dim i As Long
For i = 1 To 10000000
Next i
MsgBox "Waited for a little while"
End Sub
Loop Wait for Simple Delays
Using a loop to wait is a simple approach but lacks precision and can consume significant system resources, making it less desirable for most applications.5. DoEvents Function
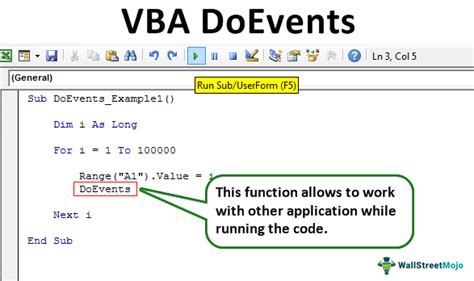
The DoEvents
function yields execution so that the operating system can process other events. It can be used within a loop to create a wait that allows the system to remain responsive.
Sub WaitFor2SecondsUsingDoEvents()
Dim StartTime As Single
StartTime = Timer
Do While Timer < StartTime + 2
DoEvents
Loop
MsgBox "Waited for 2 seconds"
End Sub
DoEvents for System Responsiveness
`DoEvents` is crucial for maintaining system responsiveness during waits. It ensures that the application does not freeze and allows users to interact with other parts of the program or even close it if necessary.VBA Wait Functions Image Gallery
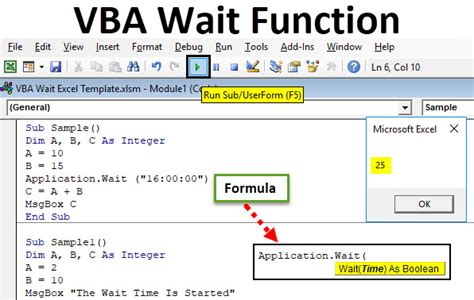
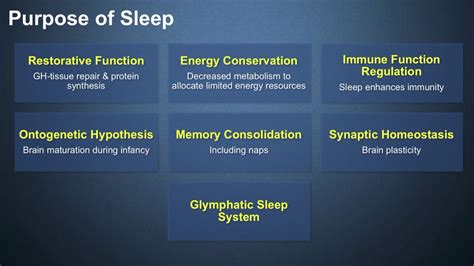
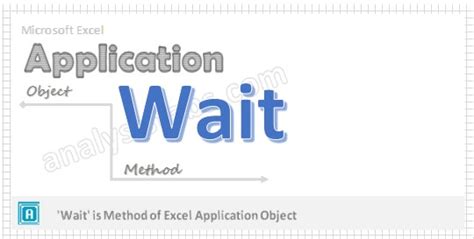
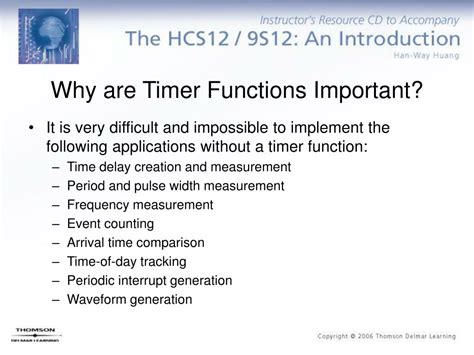
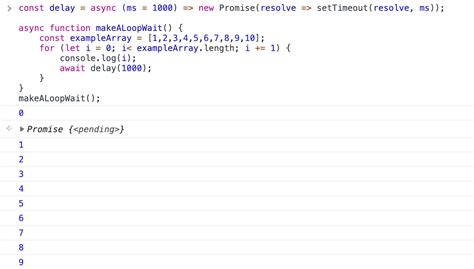
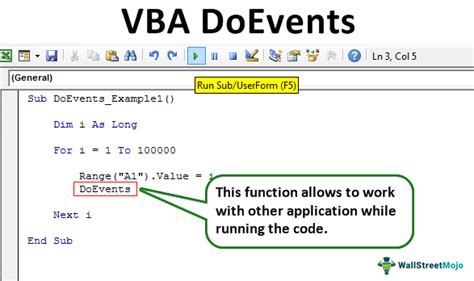
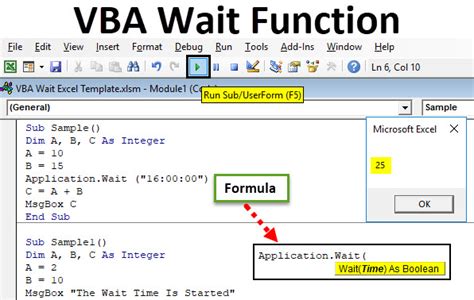
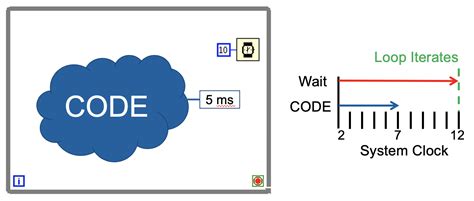
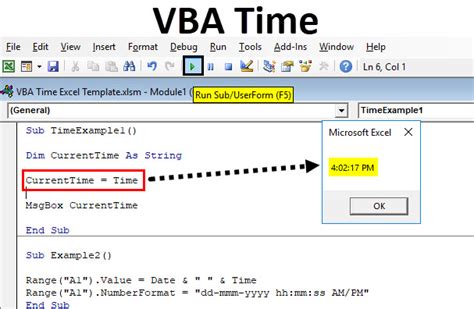
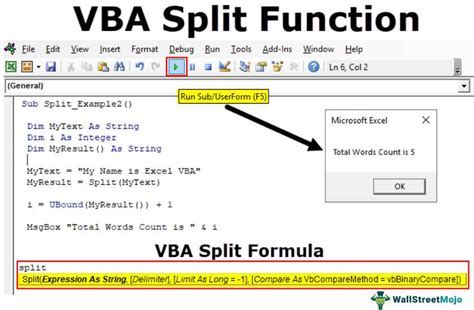
What is the purpose of wait functions in VBA?
+Wait functions in VBA are used to introduce delays or pauses in a program, allowing for synchronization, waiting for user input, or ensuring that tasks are executed in the correct order.
How do I choose the right wait function for my VBA application?
+The choice of wait function depends on the specific requirements of your application, including the need for precision, system responsiveness, and the type of delay required.
Can I use wait functions for synchronization in multi-threaded VBA applications?
+Yes, wait functions can be used for synchronization in multi-threaded VBA applications to ensure that tasks are performed in a coordinated manner.
How do I implement a precise delay in VBA?
+A precise delay in VBA can be implemented using the Timer function, which provides a more accurate way to introduce delays compared to other methods.
What are the advantages of using DoEvents in wait loops?
+Using DoEvents in wait loops allows the system to remain responsive, enabling users to interact with other parts of the application during the wait period.
In conclusion, mastering the different ways to make VBA wait is essential for any developer aiming to create efficient, user-friendly, and robust applications. Whether it's for educational purposes, professional development, or personal projects, understanding VBA wait functions can significantly enhance your programming skills and open up new possibilities for automation and customization within Microsoft Office. We invite you to share your experiences, ask questions, or provide feedback on using VBA wait functions in the comments below. Your input can help others learn from your experiences and contribute to a community of VBA enthusiasts.