Intro
Discover 5 efficient ways to sort data, lists, and files using algorithms, methods, and techniques, including quicksort, mergesort, and more, to improve organization and productivity with effective sorting strategies.
Sorting is a fundamental concept in various fields, including computer science, data analysis, and everyday life. It involves arranging items in a specific order, either ascending or descending, based on certain criteria. In this article, we will explore five ways to sort, their applications, and the benefits of using each method.
Sorting is essential in many aspects of life, from organizing files on a computer to arranging data in a spreadsheet. It helps to simplify complex information, making it easier to understand and analyze. With the increasing amount of data being generated every day, sorting has become a crucial tool for individuals and organizations to manage and make sense of their data.
The importance of sorting cannot be overstated. It saves time, reduces errors, and improves decision-making. By sorting data, individuals can identify patterns, trends, and correlations that may not be apparent when the data is in a random order. Moreover, sorting enables efficient retrieval of information, making it easier to locate specific items or data points.
Introduction to Sorting Methods
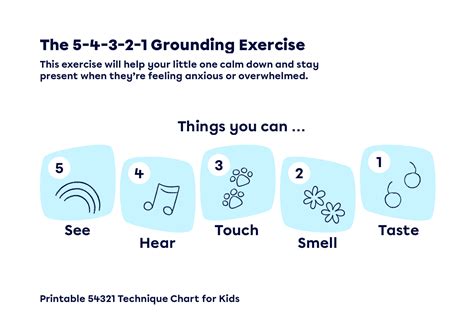
There are several sorting methods, each with its strengths and weaknesses. The choice of method depends on the type of data, the size of the dataset, and the desired outcome. In this article, we will discuss five common sorting methods: bubble sort, selection sort, insertion sort, merge sort, and quick sort.
Bubble Sort
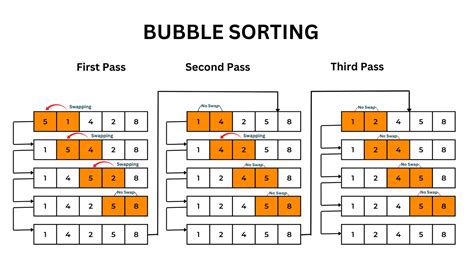
Bubble sort is a simple sorting algorithm that works by repeatedly iterating through a list of items, comparing adjacent elements and swapping them if they are in the wrong order. This process continues until no more swaps are needed, indicating that the list is sorted. Bubble sort is easy to implement and understand, making it a popular choice for educational purposes.
However, bubble sort has a time complexity of O(n^2), making it inefficient for large datasets. It is also not suitable for sorting lists with a large number of unique elements, as the number of comparisons required can be excessive.
Advantages and Disadvantages of Bubble Sort
The advantages of bubble sort include:
- Easy to implement and understand
- Simple to code
- Works well for small datasets
The disadvantages of bubble sort include:
- Inefficient for large datasets
- Not suitable for sorting lists with a large number of unique elements
- Has a high time complexity
Selection Sort
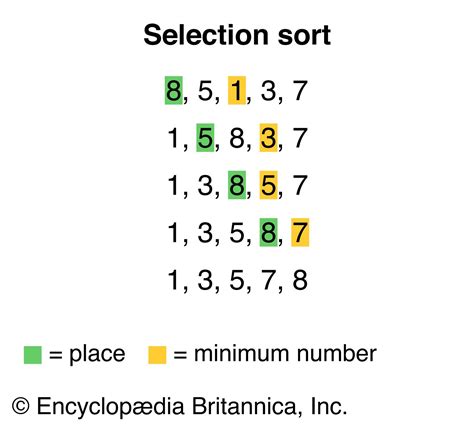
Selection sort is another simple sorting algorithm that works by selecting the smallest (or largest) element from the unsorted portion of the list and moving it to the beginning (or end) of the sorted portion. This process continues until the entire list is sorted.
Selection sort has a time complexity of O(n^2), making it less efficient than other sorting algorithms like quick sort or merge sort. However, it has the advantage of minimizing the number of swaps required, making it suitable for sorting lists where swapping elements is expensive.
Advantages and Disadvantages of Selection Sort
The advantages of selection sort include:
- Minimizes the number of swaps required
- Simple to implement and understand
- Works well for small datasets
The disadvantages of selection sort include:
- Inefficient for large datasets
- Has a high time complexity
- Not suitable for sorting lists with a large number of unique elements
Insertion Sort
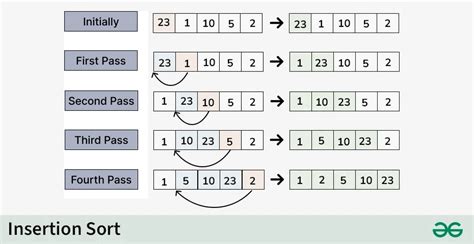
Insertion sort is a simple sorting algorithm that works by iterating through a list of items one at a time, inserting each item into its proper position in the sorted portion of the list. This process continues until the entire list is sorted.
Insertion sort has a time complexity of O(n^2), making it less efficient than other sorting algorithms like quick sort or merge sort. However, it has the advantage of being adaptive, meaning that it performs well on nearly sorted lists.
Advantages and Disadvantages of Insertion Sort
The advantages of insertion sort include:
- Adaptive, meaning it performs well on nearly sorted lists
- Simple to implement and understand
- Works well for small datasets
The disadvantages of insertion sort include:
- Inefficient for large datasets
- Has a high time complexity
- Not suitable for sorting lists with a large number of unique elements
Merge Sort
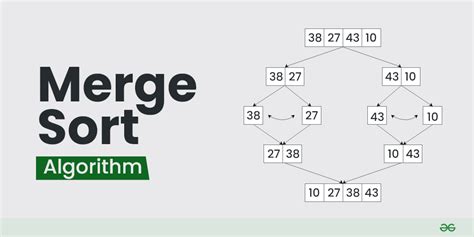
Merge sort is a divide-and-conquer sorting algorithm that works by dividing a list of items into smaller sublists, sorting each sublist, and then merging the sorted sublists back together. This process continues until the entire list is sorted.
Merge sort has a time complexity of O(n log n), making it more efficient than other sorting algorithms like bubble sort or selection sort. It is also stable, meaning that it preserves the order of equal elements.
Advantages and Disadvantages of Merge Sort
The advantages of merge sort include:
- Efficient, with a time complexity of O(n log n)
- Stable, meaning it preserves the order of equal elements
- Works well for large datasets
The disadvantages of merge sort include:
- Requires extra memory to store the temporary sublists
- Can be slow for small datasets
Quick Sort
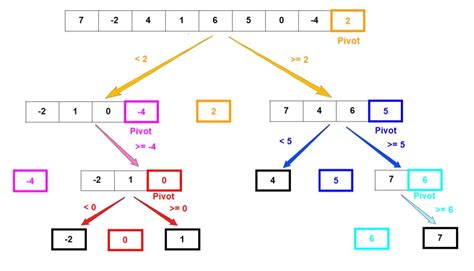
Quick sort is a divide-and-conquer sorting algorithm that works by selecting a pivot element, partitioning the list around the pivot, and then recursively sorting the sublists. This process continues until the entire list is sorted.
Quick sort has an average time complexity of O(n log n), making it one of the fastest sorting algorithms. However, it can have a worst-case time complexity of O(n^2) if the pivot is chosen poorly.
Advantages and Disadvantages of Quick Sort
The advantages of quick sort include:
- Fast, with an average time complexity of O(n log n)
- In-place, meaning it does not require extra memory
- Works well for large datasets
The disadvantages of quick sort include:
- Can have a worst-case time complexity of O(n^2) if the pivot is chosen poorly
- Not stable, meaning it does not preserve the order of equal elements
Sorting Image Gallery
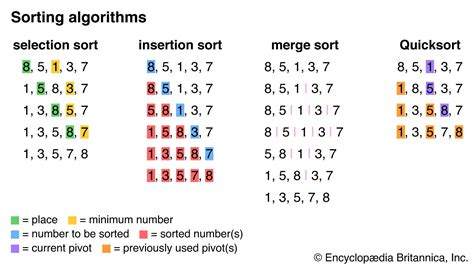
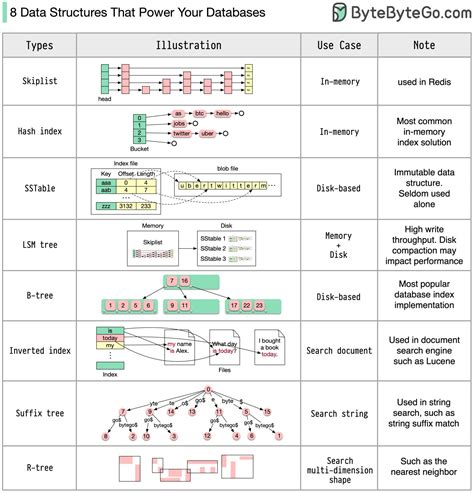
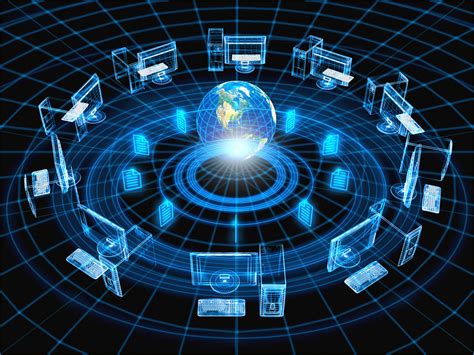
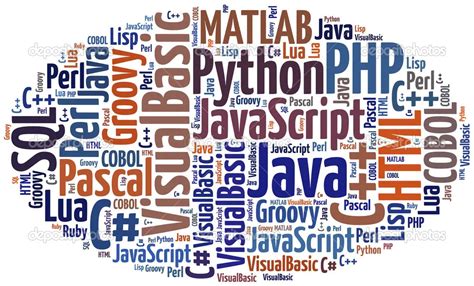
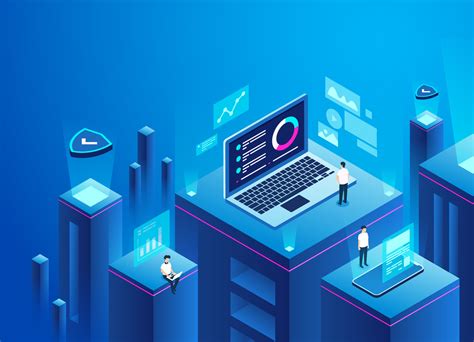
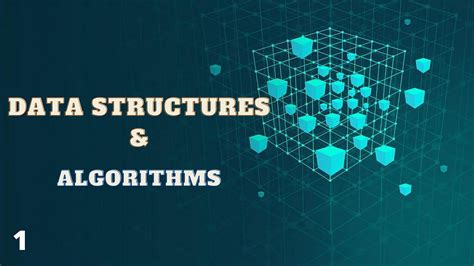
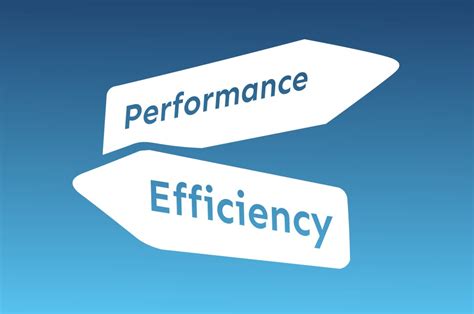
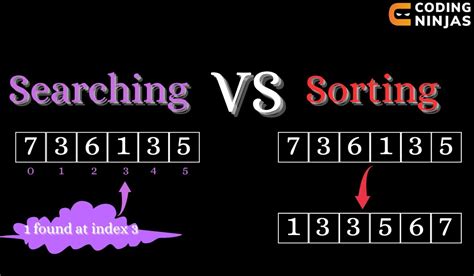
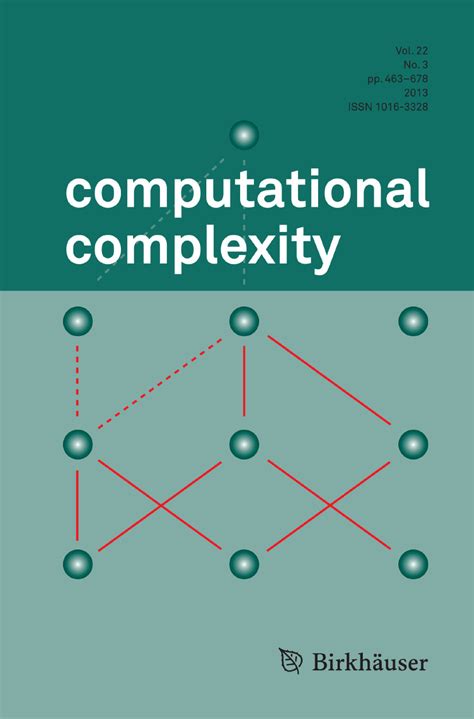
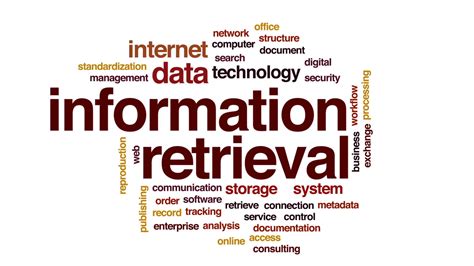
What is the difference between bubble sort and selection sort?
+Bubble sort and selection sort are both simple sorting algorithms, but they work in different ways. Bubble sort repeatedly iterates through a list, comparing adjacent elements and swapping them if they are in the wrong order. Selection sort, on the other hand, selects the smallest (or largest) element from the unsorted portion of the list and moves it to the beginning (or end) of the sorted portion.
What is the time complexity of merge sort?
+The time complexity of merge sort is O(n log n), making it one of the most efficient sorting algorithms.
What is the advantage of using quick sort?
+The advantage of using quick sort is that it has an average time complexity of O(n log n), making it one of the fastest sorting algorithms. Additionally, it is an in-place algorithm, meaning it does not require extra memory.
In conclusion, sorting is a crucial concept in various fields, and there are several sorting methods to choose from, each with its strengths and weaknesses. By understanding the different sorting methods and their applications, individuals can make informed decisions about which method to use in a given situation. Whether you are a student, a programmer, or a data analyst, sorting is an essential tool that can help you to simplify complex information, identify patterns and trends, and make better decisions. We hope this article has provided you with a comprehensive understanding of the different sorting methods and their applications. If you have any further questions or would like to share your experiences with sorting, please do not hesitate to comment below.