Intro
Learn to create a new Excel workbook using VBA, with step-by-step guides on workbook creation, Excel macros, and spreadsheet automation, optimizing workflow and data management with Visual Basic for Applications.
Creating a new Excel workbook using VBA (Visual Basic for Applications) is a straightforward process that can be accomplished with a few lines of code. This can be particularly useful for automating tasks, such as generating reports or templates, where you need to create new workbooks programmatically. Below is a step-by-step guide on how to create a new Excel workbook using VBA, along with explanations and examples to help you understand the process better.
Step 1: Opening the Visual Basic Editor
To start working with VBA in Excel, you first need to open the Visual Basic Editor. You can do this by pressing Alt
+ F11
or by navigating to the Developer tab in Excel and clicking on the Visual Basic button. If you don't see the Developer tab, you may need to add it to your ribbon by going to File > Options > Customize Ribbon and checking the box next to Developer.
Step 2: Creating a New Module
Once the Visual Basic Editor is open, you'll need to insert a new module to write your code. To do this:
- In the Visual Basic Editor, right-click on any of the objects for your workbook in the Project Explorer on the left side. If the Project Explorer is not visible, press
Ctrl
+R
or go to View > Project Explorer. - Choose
Insert
>Module
from the context menu. This action creates a new module.
Step 3: Writing the VBA Code to Create a New Workbook
Now, you can write the VBA code to create a new Excel workbook. Here is a simple example:
Sub CreateNewWorkbook()
' Declare a variable for the new workbook
Dim newWorkbook As Workbook
' Create a new workbook
Set newWorkbook = Workbooks.Add
' Save the new workbook with a specific name and path
newWorkbook.SaveAs Filename:="C:\YourPath\NewWorkbook.xlsx"
' Close the new workbook
newWorkbook.Close
End Sub
In this example:
Workbooks.Add
is used to create a new workbook.newWorkbook.SaveAs
is used to save the workbook with a specific name and path. Make sure to replace"C:\YourPath\NewWorkbook.xlsx"
with your desired path and filename.newWorkbook.Close
is used to close the new workbook after it has been saved.
Step 4: Running the VBA Code
To run your VBA code:
- Press
F5
while in the Visual Basic Editor with the module that contains your code active. - Alternatively, you can run the macro by going to the Developer tab in Excel, clicking on the Macros button, selecting the
CreateNewWorkbook
macro, and then clicking Run.
Tips and Variations
- Saving Without Closing: If you want to keep the new workbook open after saving, simply remove the
newWorkbook.Close
line. - Specifying the Workbook Template: You can create a new workbook based on a specific template by passing the template's path to the
Workbooks.Add
method, like so:Workbooks.Add Template:="C:\YourPath\YourTemplate.xltx"
. - Handling Errors: It's a good practice to include error handling in your code to deal with potential issues, such as the file already existing or lack of permissions to save the file.
Conclusion and Next Steps
Creating a new Excel workbook using VBA is a basic yet powerful skill that can significantly enhance your productivity and automate repetitive tasks. As you become more comfortable with VBA, you can explore more advanced topics, such as manipulating data within workbooks, interacting with other Office applications, and even creating custom user interfaces. Remember, practice is key, so experiment with different VBA scripts to deepen your understanding and find new ways to apply your skills.
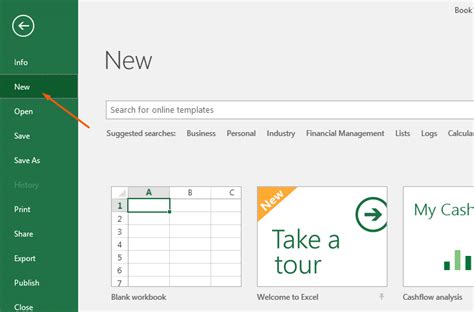
Advanced VBA Techniques for Workbook Creation
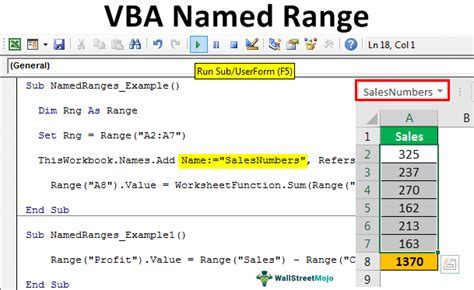
For those looking to delve deeper into VBA programming, understanding how to create and manipulate workbooks is fundamental. Advanced techniques include using loops to create multiple workbooks at once, applying specific formatting or data to new workbooks, and integrating VBA with other tools or applications to automate complex workflows.
Using Loops to Create Multiple Workbooks

Loops are a powerful feature in VBA that allow you to repeat a set of instructions. When creating workbooks, loops can be used to generate multiple workbooks with similar properties or to automate tasks across several workbooks.
Best Practices for VBA Coding
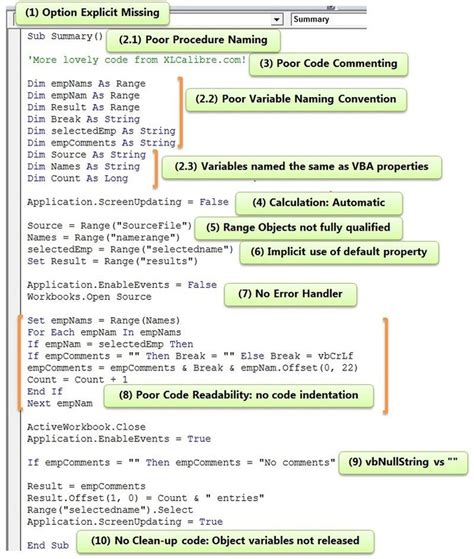
Following best practices when coding in VBA can significantly improve the readability, maintainability, and performance of your scripts. This includes using meaningful variable names, commenting your code, and structuring your macros in a logical and organized manner.
Debugging VBA Code
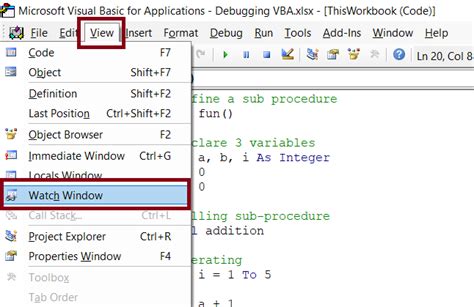
Debugging is an essential part of the coding process. VBA offers several tools to help you identify and fix errors, including the ability to step through your code line by line, examine variable values, and set breakpoints.
Gallery of VBA Workbook Creation
VBA Workbook Creation Gallery
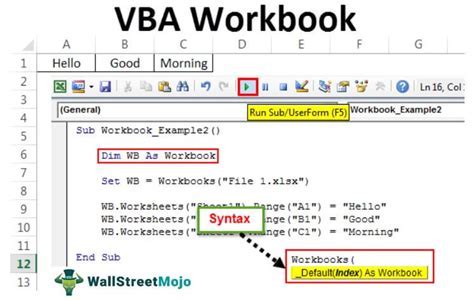
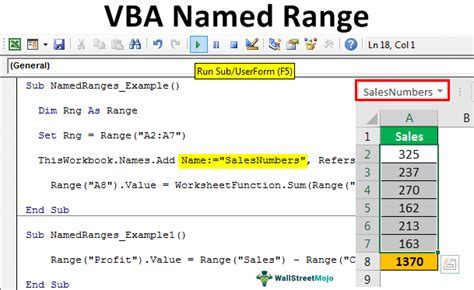

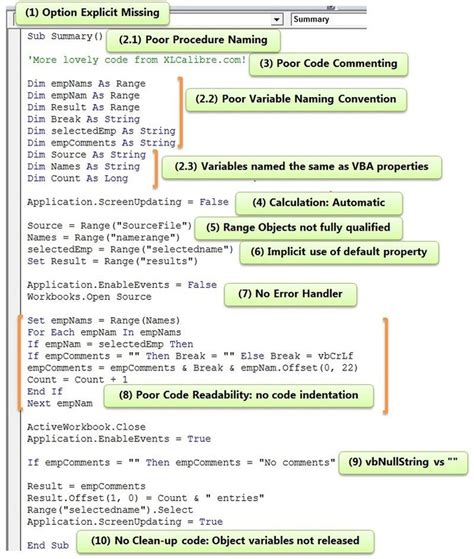
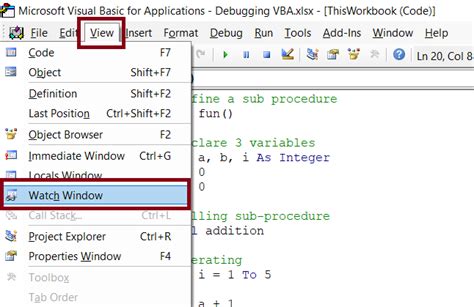
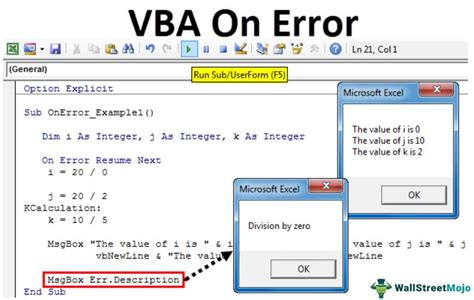
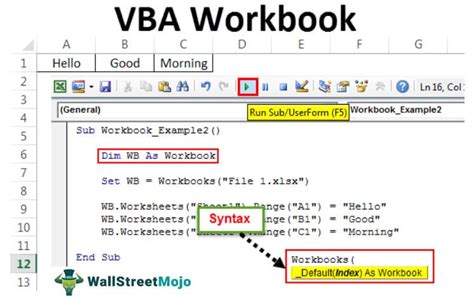
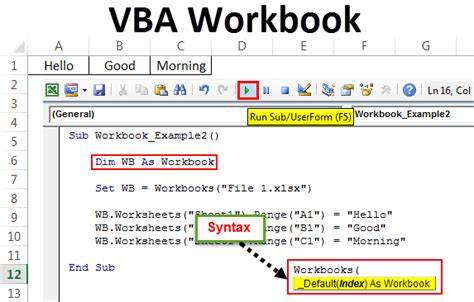
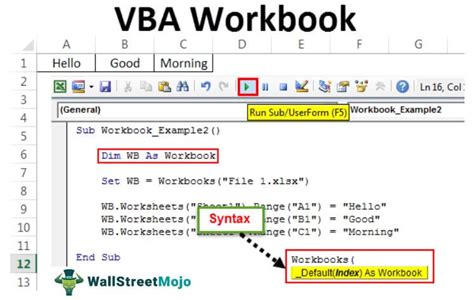
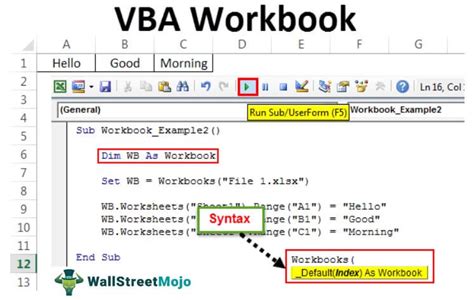
What is VBA used for in Excel?
+VBA (Visual Basic for Applications) is used in Excel for automating tasks, creating custom tools, and enhancing the functionality of worksheets and workbooks.
How do I create a new workbook in VBA?
+To create a new workbook in VBA, use the Workbooks.Add
method. This can be done by writing Set newWorkbook = Workbooks.Add
in your VBA code.
Can I save a new workbook with a specific name and path using VBA?
+Yes, you can save a new workbook with a specific name and path by using the SaveAs
method, such as newWorkbook.SaveAs Filename:="C:\YourPath\NewWorkbook.xlsx"
.
We hope this comprehensive guide to creating a new Excel workbook using VBA has been informative and helpful. Whether you're a beginner looking to automate simple tasks or an advanced user seeking to leverage VBA for complex projects, understanding how to create and manipulate workbooks is a crucial skill. Feel free to share your experiences, ask questions, or provide tips in the comments below.