Intro
Excel VBA is a powerful tool for automating tasks in Excel, and working with arrays is a common requirement in many VBA scripts. Adding an item to an array in VBA can be a bit tricky, but there are several ways to do it. In this article, we will explore the different methods to add an item to an array in Excel VBA.
To start with, let's consider the importance of arrays in VBA programming. Arrays are used to store collections of data, and they are a fundamental data structure in programming. In VBA, arrays can be used to store and manipulate data, perform calculations, and automate tasks. However, working with arrays can be challenging, especially when it comes to adding or removing items.
One of the common challenges when working with arrays in VBA is that they are fixed-size, meaning that once an array is declared, its size cannot be changed. This can make it difficult to add new items to an array, especially if the array is already full. However, there are several workarounds to this problem, and we will explore them in this article.
Understanding Arrays in VBA
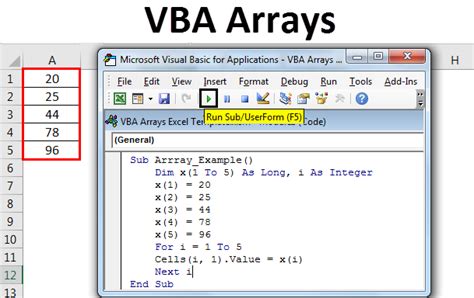
Before we dive into the methods for adding an item to an array, let's take a brief look at how arrays work in VBA. In VBA, an array is a collection of variables of the same data type stored in a single variable. Arrays can be declared using the Dim statement, and they can be initialized using the Array function.
For example, the following code declares an array of integers and initializes it with some values:
Dim myArray(3) As Integer
myArray(0) = 10
myArray(1) = 20
myArray(2) = 30
myArray(3) = 40
This code creates an array called myArray with four elements, each containing an integer value.
Method 1: Using the ReDim Statement
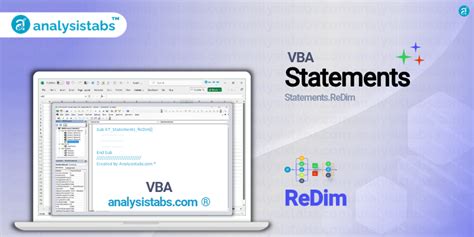
One way to add an item to an array in VBA is to use the ReDim statement. The ReDim statement is used to resize an array, and it can be used to add new elements to an existing array.
For example, the following code adds a new element to the myArray array:
ReDim Preserve myArray(4)
myArray(4) = 50
This code resizes the myArray array to have five elements, and then assigns the value 50 to the new element.
Note that the Preserve keyword is used to preserve the existing data in the array. If the Preserve keyword is not used, the existing data in the array will be lost.
Example Code
The following code demonstrates how to use the ReDim statement to add an item to an array: ```vb Sub AddItemToArray() Dim myArray(3) As Integer myArray(0) = 10 myArray(1) = 20 myArray(2) = 30 myArray(3) = 40ReDim Preserve myArray(4)
myArray(4) = 50
' Print the array
For i = 0 To 4
Debug.Print myArray(i)
Next i
End Sub
This code creates an array called myArray, adds a new element to it using the ReDim statement, and then prints the array to the debug console.
Method 2: Using a Collection
Another way to add an item to an array in VBA is to use a Collection object. A Collection is a built-in VBA object that can be used to store and manipulate collections of data.
For example, the following code creates a Collection and adds some items to it:
```vb
Dim myCollection As New Collection
myCollection.Add 10
myCollection.Add 20
myCollection.Add 30
myCollection.Add 40
This code creates a Collection called myCollection and adds four items to it.
To add a new item to the Collection, you can use the Add method:
myCollection.Add 50
This code adds a new item to the Collection with the value 50.
Example Code
The following code demonstrates how to use a Collection to add an item to an array: ```vb Sub AddItemToCollection() Dim myCollection As New Collection myCollection.Add 10 myCollection.Add 20 myCollection.Add 30 myCollection.Add 40myCollection.Add 50
' Print the collection
For i = 1 To myCollection.Count
Debug.Print myCollection(i)
Next i
End Sub
This code creates a Collection called myCollection, adds some items to it, and then prints the Collection to the debug console.
Method 3: Using a Dictionary
A third way to add an item to an array in VBA is to use a Dictionary object. A Dictionary is a built-in VBA object that can be used to store and manipulate collections of data.
For example, the following code creates a Dictionary and adds some items to it:
```vb
Dim myDictionary As Object
Set myDictionary = CreateObject("Scripting.Dictionary")
myDictionary.Add "Key1", 10
myDictionary.Add "Key2", 20
myDictionary.Add "Key3", 30
myDictionary.Add "Key4", 40
This code creates a Dictionary called myDictionary and adds four items to it.
To add a new item to the Dictionary, you can use the Add method:
myDictionary.Add "Key5", 50
This code adds a new item to the Dictionary with the key "Key5" and the value 50.
Example Code
The following code demonstrates how to use a Dictionary to add an item to an array: ```vb Sub AddItemToDictionary() Dim myDictionary As Object Set myDictionary = CreateObject("Scripting.Dictionary") myDictionary.Add "Key1", 10 myDictionary.Add "Key2", 20 myDictionary.Add "Key3", 30 myDictionary.Add "Key4", 40myDictionary.Add "Key5", 50
' Print the dictionary
For Each key In myDictionary.Keys
Debug.Print myDictionary(key)
Next key
End Sub
This code creates a Dictionary called myDictionary, adds some items to it, and then prints the Dictionary to the debug console.
Conclusion and Next Steps
In this article, we have explored three methods for adding an item to an array in Excel VBA: using the ReDim statement, using a Collection, and using a Dictionary. Each method has its own advantages and disadvantages, and the choice of method will depend on the specific requirements of your project.
We hope that this article has been helpful in providing you with the knowledge and skills you need to work with arrays in VBA. Whether you are a beginner or an experienced programmer, we hope that you have found this article informative and useful.
If you have any questions or need further help, please don't hesitate to ask. We are always here to help.
Excel VBA Array Image Gallery
What is an array in VBA?
+
An array in VBA is a collection of variables of the same data type stored in a single variable.
How do I declare an array in VBA?
+
You can declare an array in VBA using the Dim statement, for example: Dim myArray(3) As Integer.
How do I add an item to an array in VBA?
+
You can add an item to an array in VBA using the ReDim statement, a Collection, or a Dictionary.
What is the difference between a Collection and a Dictionary in VBA?
+
A Collection is a built-in VBA object that can be used to store and manipulate collections of data, while a Dictionary is a built-in VBA object that can be used to store and manipulate key-value pairs.
How do I use a Dictionary in VBA?
+
You can use a Dictionary in VBA by creating a new Dictionary object, adding key-value pairs to it, and then accessing the values using the keys.
We hope that you have found this article helpful in providing you with the knowledge and skills you need to work with arrays in VBA. If you have any questions or need further help, please don't hesitate to ask. We are always here to help. Please share this article with your friends and colleagues who may be interested in learning more about VBA programming.