Intro
Learn Excel VBA to count rows easily with macros, loops, and range objects, simplifying data analysis and automation tasks with efficient coding techniques.
When working with Excel, one of the most common tasks is counting the number of rows in a worksheet or a specific range. This can be particularly useful for managing data, automating tasks, and ensuring that your worksheets are up-to-date. Excel VBA provides a powerful way to achieve this through various methods, each with its own advantages and best use cases.
Counting rows is essential for a variety of applications, from simple data management to complex data analysis and automation tasks. Whether you're dealing with a small dataset or a large, complex spreadsheet, being able to accurately and efficiently count rows can save you a significant amount of time and reduce the likelihood of errors.
Excel VBA, or Visual Basic for Applications, is a programming language built into Excel that allows users to create and automate tasks. It's a versatile tool that can be used for everything from simple macros to complex applications. When it comes to counting rows, VBA offers several approaches, each tailored to different scenarios and requirements.
For those new to VBA, the concept of counting rows might seem daunting, but it's actually quite straightforward. With a few lines of code, you can automate the process of counting rows in your worksheets, making it easier to manage and analyze your data. This not only saves time but also helps in reducing manual errors that can occur when performing repetitive tasks.
The importance of accurately counting rows cannot be overstated. In data analysis, for instance, understanding the number of rows can help in identifying trends, managing datasets, and performing statistical analysis. In automation tasks, counting rows can be crucial for looping through data, applying formulas, or formatting cells based on specific conditions.
Given the significance of row counting in Excel VBA, it's essential to explore the various methods available. From using the Rows.Count
property to looping through cells and checking for blank rows, each method has its unique application and efficiency. By mastering these techniques, users can enhance their productivity and leverage the full potential of Excel for data management and analysis.
Methods for Counting Rows in Excel VBA
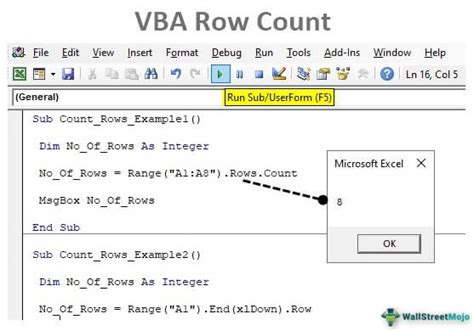
There are several methods to count rows in Excel VBA, each with its own set of advantages and ideal use cases. Understanding these methods and when to apply them is crucial for efficient data management and analysis.
1. Using the Rows.Count
Property
One of the most straightforward methods to count rows is by using the Rows.Count
property. This property returns the number of rows in the worksheet or the specified range. It's a simple and efficient way to get a row count, especially when dealing with the entire worksheet.
Sub CountRowsUsingRowsCount()
Dim rowCount As Long
rowCount = ActiveSheet.Rows.Count
MsgBox "Number of rows: " & rowCount
End Sub
2. Looping Through Cells
Another approach is to loop through cells in a column and count the number of cells that contain data. This method is useful when you need to count rows based on specific conditions, such as non-blank cells in a particular column.
Sub CountRowsByLooping()
Dim i As Long
Dim rowCount As Long
For i = 1 To ActiveSheet.Rows.Count
If ActiveSheet.Cells(i, 1).Value <> "" Then
rowCount = rowCount + 1
Else
Exit For
End If
Next i
MsgBox "Number of rows with data: " & rowCount
End Sub
3. Using the Range.Find
Method
The Range.Find
method can also be used to count rows by finding the last cell with data in a column. This method is particularly useful for quickly identifying the last row with data without having to loop through all rows.
Sub CountRowsUsingFind()
Dim lastRow As Long
lastRow = ActiveSheet.Cells.Find(What:="*", SearchOrder:=xlByRows, SearchDirection:=xlPrevious).Row
MsgBox "Last row with data: " & lastRow
End Sub
4. Using the SpecialCells
Method
For worksheets that contain formulas, using the SpecialCells
method to count rows with formulas can be very handy. This method allows you to count rows based on specific cell properties, such as containing formulas or constants.
Sub CountRowsWithFormulas()
Dim formulaRows As Range
Set formulaRows = ActiveSheet.Cells.SpecialCells(xlCellTypeFormulas)
MsgBox "Number of rows with formulas: " & formulaRows.Rows.Count
End Sub
Benefits of Using VBA for Row Counting
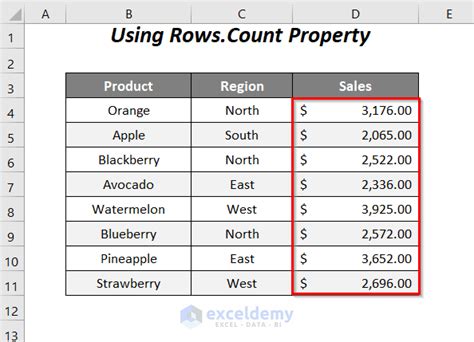
Using VBA for counting rows offers several benefits, including increased efficiency, reduced errors, and enhanced flexibility. By automating the row counting process, users can focus on more complex tasks and improve overall productivity.
- Efficiency: VBA scripts can run much faster than manual methods, especially for large datasets.
- Accuracy: Automated processes reduce the chance of human error, ensuring that row counts are accurate.
- Flexibility: VBA allows for complex logic and conditions to be applied to row counting, making it adaptable to a wide range of scenarios.
Common Applications of Row Counting in VBA
Row counting is a fundamental operation in Excel VBA, with applications in various fields, including data analysis, automation, and reporting. Some common applications include:- Data Analysis: Counting rows is crucial for understanding dataset sizes and applying appropriate analytical techniques.
- Automation: Row counts are often used in loops to perform repetitive tasks, such as data formatting or calculation.
- Reporting: Accurate row counts can be essential for generating reports, especially when summarizing large datasets.
Best Practices for Row Counting in VBA
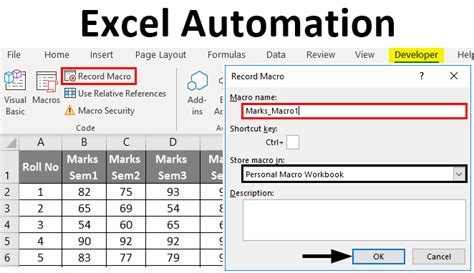
To ensure that your VBA scripts for row counting are efficient and reliable, follow these best practices:
- Use Efficient Methods: Choose the method that best fits your scenario, considering factors like dataset size and complexity.
- Test Thoroughly: Always test your scripts with different datasets to ensure they work as expected.
- Optimize for Performance: For large datasets, optimize your scripts to minimize execution time.
Tips for Troubleshooting Row Counting Issues
When issues arise with row counting scripts, troubleshooting can be straightforward if you follow a systematic approach:- Check Dataset: Ensure the dataset is correctly formatted and free of unexpected blank rows or columns.
- Review Code: Verify that the VBA code is correctly implemented, with no syntax errors or logical flaws.
- Test in Isolation: Test the row counting function independently of other code to isolate the issue.
Excel VBA Row Counting Gallery
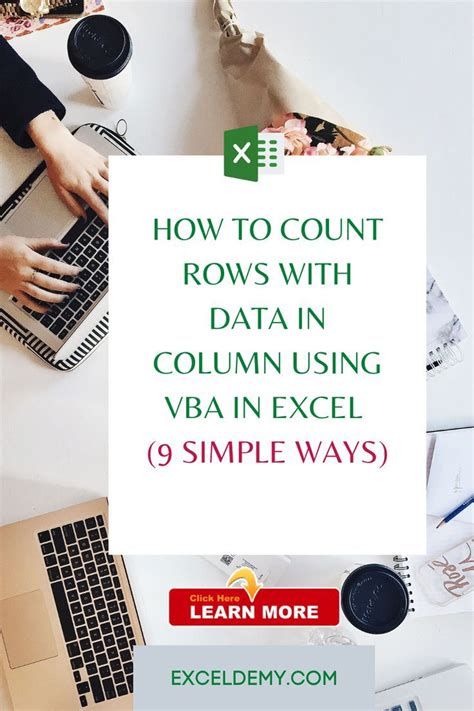
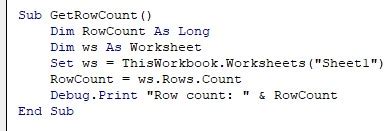
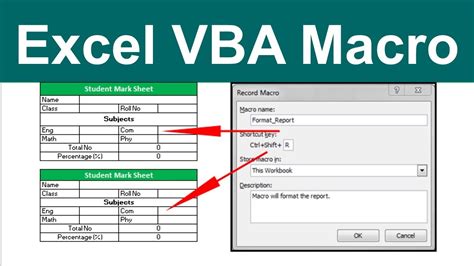
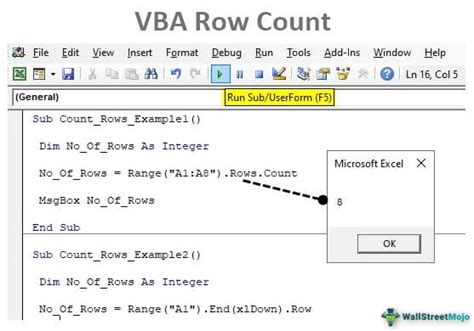
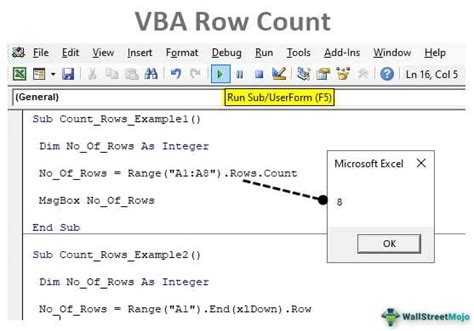
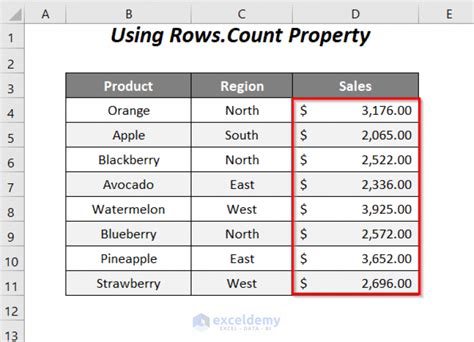
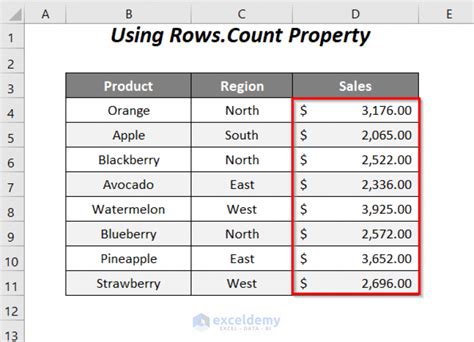
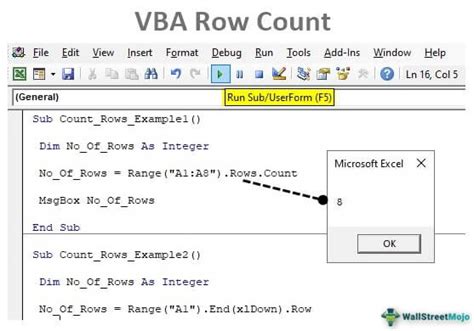
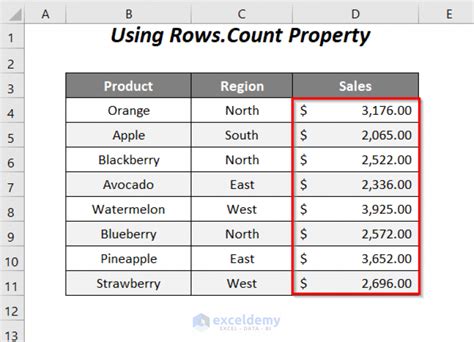
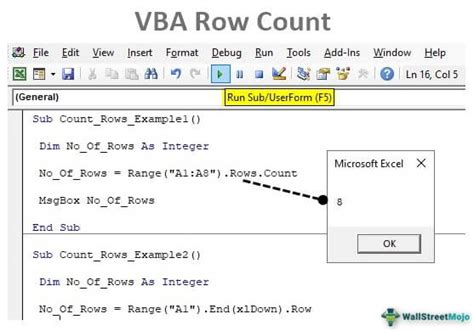
What is the most efficient way to count rows in Excel VBA?
+The most efficient way often involves using the `Rows.Count` property or the `Range.Find` method, depending on the specific requirements of your task.
How can I count rows with data in a specific column?
+You can use a loop to iterate through the cells in the column and increment a counter for each non-blank cell, or use the `Range.Find` method to find the last cell with data.
What are some common applications of row counting in Excel VBA?
+Row counting is commonly used in data analysis, automation tasks, and reporting, where understanding the number of rows is crucial for applying the right analytical techniques or generating accurate reports.
In conclusion, counting rows in Excel VBA is a versatile and powerful skill that can significantly enhance your data management and analysis capabilities. By mastering the various methods available and following best practices for efficiency and reliability, you can leverage the full potential of Excel VBA for your tasks. Whether you're dealing with simple datasets or complex spreadsheets, the ability to accurately and efficiently count rows can make a substantial difference in your productivity and the accuracy of your results. We invite you to share your experiences, ask questions, or explore more topics related to Excel VBA and row counting in the comments below.