Intro
The ability to find cells containing specific text is a powerful tool in Excel VBA, allowing for efficient data manipulation and analysis. This capability is crucial for automating tasks, especially in large datasets where manual searches would be time-consuming and prone to errors. Excel's VBA (Visual Basic for Applications) provides several methods to achieve this, including using the Find
method of the Range
object, looping through cells, and utilizing the Filter
function.
Finding cells with specific text can be applied in various scenarios, such as data cleaning, report generation, and data extraction for further analysis. The approach you choose may depend on the complexity of your task, the size of your dataset, and your familiarity with VBA.
Importance of Finding Cells with Specific Text
Being able to locate specific text within an Excel spreadsheet is vital for several reasons:
- Data Analysis: Identifying cells with particular text can help in filtering out relevant data for analysis.
- Automation: Automated tasks often require locating specific data points to perform actions such as formatting, copying, or moving data.
- Error Reduction: Manual searches can lead to human error, especially in large datasets. VBA scripts can perform these searches more accurately and quickly.
Using the Find
Method
The Find
method is one of the most straightforward ways to locate cells containing specific text in Excel VBA. Here's a basic example of how to use it:
Sub FindCell()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim foundCell As Range
Set foundCell = ws.Cells.Find(What:="SpecificText", LookIn:=xlValues)
If Not foundCell Is Nothing Then
MsgBox "Text found in cell " & foundCell.Address
Else
MsgBox "Text not found"
End If
End Sub
This script searches for the text "SpecificText" in the values of all cells in "Sheet1". If the text is found, it displays a message box with the address of the cell containing the text.
Looping Through Cells
Another approach is to loop through each cell in a range and check its value. This method provides more flexibility but can be slower for large datasets:
Sub LoopThroughCells()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim cell As Range
For Each cell In ws.UsedRange.Cells
If cell.Value = "SpecificText" Then
MsgBox "Text found in cell " & cell.Address
Exit For ' Stops the loop once the text is found
End If
Next cell
End Sub
Using AutoFilter
For scenarios where you need to work with all cells containing specific text, applying an AutoFilter
can be very effective:
Sub AutoFilterExample()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
ws.UsedRange.AutoFilter Field:=1, Criteria1:="SpecificText"
' Further code to handle the filtered data
End Sub
This example filters the first column of the used range to show only rows containing "SpecificText" in that column.
Practical Applications and Examples
- Data Cleaning: Use the
Find
method to locate and replace inconsistent data entries. - Report Generation: Loop through cells to gather specific data points for report generation.
- Automated Emailing: Use filtered data to send targeted emails based on cell contents.
Embedding Images for Illustration
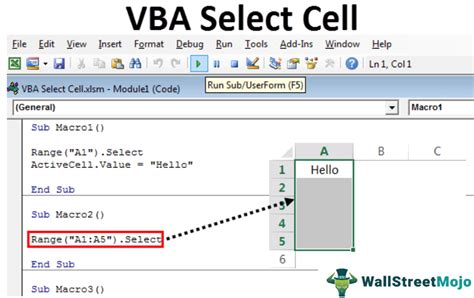
Gallery of Excel VBA Examples
Excel VBA Gallery
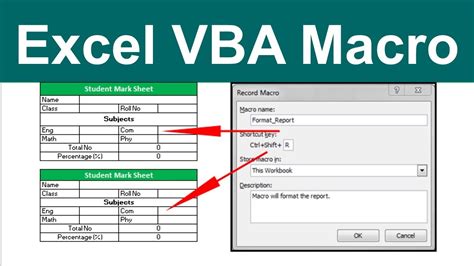
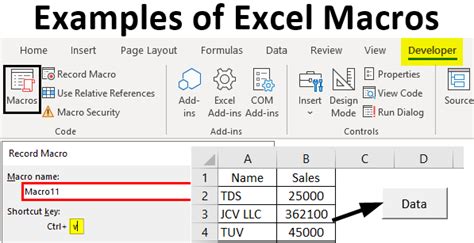
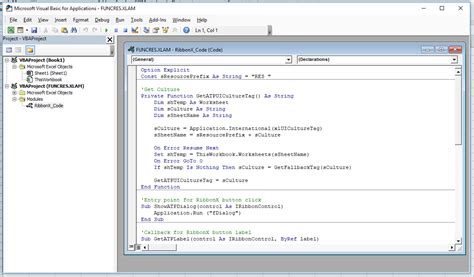
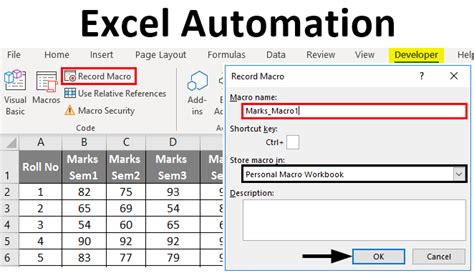
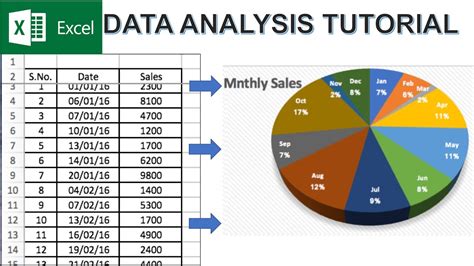
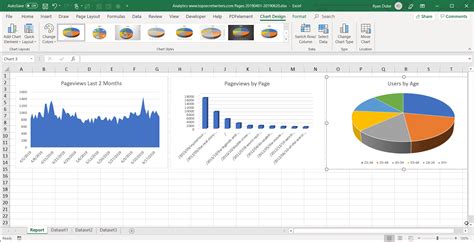
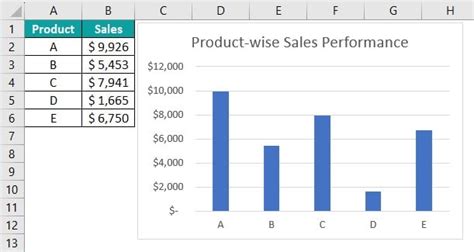
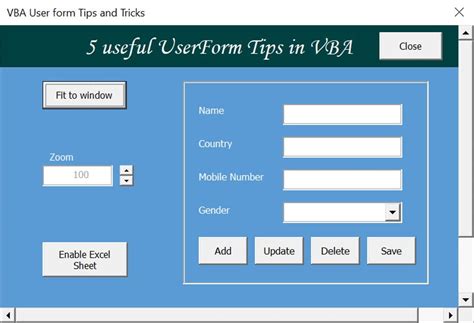
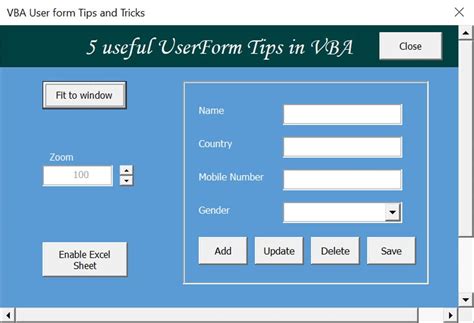
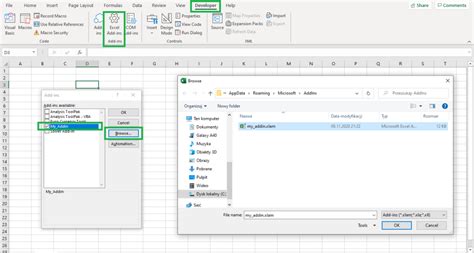
FAQs
What is Excel VBA used for?
+Excel VBA (Visual Basic for Applications) is used for creating and automating tasks in Excel, enhancing its functionality beyond basic spreadsheet operations.
How do I enable VBA in Excel?
+To enable VBA in Excel, go to the Developer tab. If the Developer tab is not visible, you can enable it through the Excel Options under the Customize Ribbon section.
What is the difference between a macro and a script in Excel VBA?
+A macro in Excel VBA is a recorded or written set of instructions that automates a task. A script, while often used interchangeably, typically refers to a more complex set of instructions or a program written in VBA.
Final Thoughts
Mastering the art of finding cells with specific text in Excel VBA is a foundational skill for any Excel power user or developer. Whether you're automating routine tasks, analyzing complex data sets, or simply looking to enhance your productivity, understanding how to efficiently locate and manipulate data based on text content is invaluable. As you delve deeper into the world of Excel VBA, you'll discover more advanced techniques and applications for this fundamental skill, further unlocking the potential of Excel as a powerful data analysis and automation tool.