Intro
Master VBA navigation with 5 ways to use Goto, including error handling, loop control, and subroutine management, optimizing code flow and efficiency in Excel macros.
The world of Visual Basic for Applications (VBA) is vast and complex, with numerous tools and techniques at a developer's disposal. One of the most fundamental and yet often misunderstood concepts in VBA is the Goto
statement. The Goto
statement is used to transfer control to a specified line of code, and it can be a powerful tool when used correctly. However, its use is often discouraged due to the potential for creating spaghetti code that is difficult to read and maintain. In this article, we will explore five ways to use Goto
in VBA, along with best practices for its use.
The importance of understanding how to use Goto
effectively cannot be overstated. While it is true that the use of Goto
can lead to code that is harder to understand and debug, there are situations where it is the most straightforward and efficient solution. For example, in error handling, Goto
can be used to direct the program to a specific section of code designed to handle and recover from errors. This makes it an essential tool for any VBA developer looking to create robust and reliable applications.
As we delve into the world of VBA and the Goto
statement, it's crucial to understand the basics of VBA programming. VBA is an event-driven programming language, meaning that code is executed in response to specific events, such as a button click or the opening of a document. The Goto
statement allows developers to control the flow of their program more directly, skipping over or repeating sections of code as needed. However, with great power comes great responsibility, and the use of Goto
requires careful planning and consideration to avoid the pitfalls of poorly structured code.
Introduction to Goto in VBA
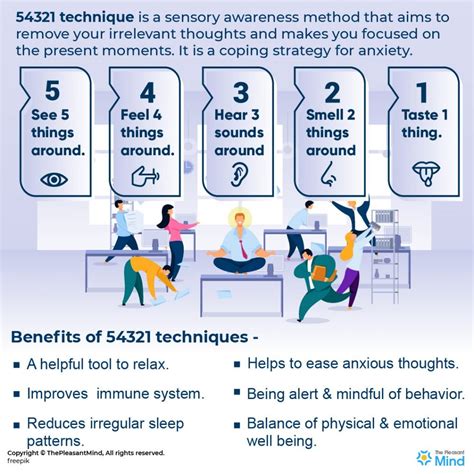
To understand how to use Goto
, one must first grasp the basic syntax and structure of VBA code. A VBA program consists of modules, which contain subroutines and functions. The Goto
statement is used within these subroutines and functions to jump to a labeled line of code. This labeled line is denoted by a colon (:) followed by the label name. For example, Goto ErrorHandler
would direct the program to the line labeled ErrorHandler:
.
Best Practices for Using Goto
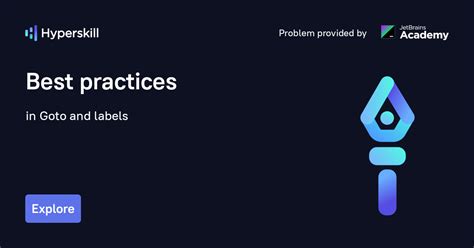
Despite the warnings against its use, there are best practices that can make Goto
a valuable tool in a VBA developer's toolkit. Firstly, Goto
should be used sparingly and only when necessary. Overuse can lead to code that is convoluted and difficult to follow. Secondly, clear and descriptive labels should be used to make it easy to understand where the Goto
statement is directing the program. Finally, Goto
should ideally be used to handle errors or exceptional conditions, rather than as a primary means of controlling program flow.
Error Handling with Goto
Error handling is one of the primary uses of `Goto` in VBA. By using `Goto` to direct the program to an error handling routine, developers can ensure that their applications are robust and can recover from unexpected errors. This is particularly important in applications that interact with external data sources or perform complex calculations, where errors are more likely to occur.5 Ways to Use Goto in VBA
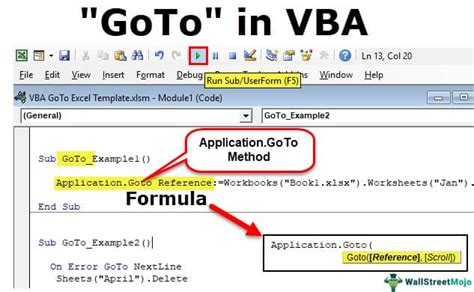
-
Error Handling: As mentioned,
Goto
is particularly useful in error handling scenarios. By usingOn Error Goto
followed by a label, developers can ensure that when an error occurs, the program jumps to a specific section of code designed to handle the error. -
Loop Control: While not the most conventional use,
Goto
can be used to control loops. For example,Goto
can be used to exit a loop prematurely or to restart a loop from the beginning. -
Conditional Execution:
Goto
can be used in conjunction with conditional statements (likeIf
) to execute different blocks of code based on certain conditions. -
Subroutine Control: In more complex programs,
Goto
can be used to control the flow between different subroutines or functions, although this use is generally discouraged in favor of more structured programming techniques. -
Restarting Code: Finally,
Goto
can be used to restart a section of code. This can be useful in scenarios where a process needs to be repeated, such as retrying a failed operation.
Example of Using Goto for Error Handling
An example of using `Goto` for error handling might look like this: ``` Sub DivideNumbers() On Error Goto ErrorHandler Dim a As Double Dim b As Double Dim result As Doublea = 10
b = 0
result = a / b
Exit Sub
ErrorHandler: MsgBox "Error: Division by zero is not allowed." End Sub
In this example, if an error occurs (in this case, division by zero), the program will jump to the `ErrorHandler` label and display a message box.
Alternatives to Goto
While `Goto` can be a useful tool, there are often alternatives that can achieve the same result without the potential drawbacks. For example, structured programming techniques such as `If` statements, `Select Case` statements, and loops can often replace the need for `Goto`. Additionally, in error handling scenarios, the use of `Try-Catch` blocks (in VBA, this is achieved with `On Error` statements) can provide a more elegant and readable solution.
Gallery of VBA Goto Examples
VBA Goto Image Gallery
Frequently Asked Questions
What is the primary use of Goto in VBA?
+
The primary use of Goto in VBA is for error handling, allowing the program to jump to a specific section of code when an error occurs.
Why is the use of Goto generally discouraged?
+
The use of Goto is discouraged because it can lead to spaghetti code that is difficult to read and maintain. However, when used sparingly and for specific purposes like error handling, it can be a valuable tool.
What are some alternatives to using Goto in VBA?
+
Alternatives to Goto include structured programming techniques such as If statements, Select Case statements, and loops, as well as the use of Try-Catch blocks for error handling.
In conclusion, the `Goto` statement in VBA is a powerful tool that, when used correctly, can enhance the functionality and robustness of VBA applications. By understanding the best practices for its use and being aware of the potential pitfalls, developers can leverage `Goto` to create more efficient and reliable code. Whether for error handling, loop control, or other specialized uses, `Goto` remains an important part of the VBA developer's toolkit. We invite you to share your thoughts and experiences with using `Goto` in VBA, and to explore the many resources available for learning more about this and other VBA topics.