Intro
Discover 5 ways VBA returns values, including functions, subroutines, and variables, to enhance Excel automation with Visual Basic for Applications, macros, and scripting.
The world of Visual Basic for Applications (VBA) is a powerful tool for automating tasks and creating interactive applications in Microsoft Office. One of the fundamental aspects of VBA is its ability to return values from various operations, functions, and methods. Understanding how VBA returns values is crucial for writing effective and efficient code. In this article, we will delve into the different ways VBA returns values, exploring the mechanisms, benefits, and practical applications of each method.
VBA is a versatile language that allows developers to create a wide range of applications, from simple macros to complex add-ins. At its core, VBA is designed to interact with the user, perform calculations, and manipulate data. The ability to return values is a critical component of this interaction, enabling developers to make informed decisions, update user interfaces, and store results for future reference. Whether you are a seasoned developer or just starting to explore the world of VBA, understanding how the language returns values is essential for unlocking its full potential.
The importance of VBA's value-returning mechanisms cannot be overstated. By mastering these techniques, developers can create more robust, flexible, and user-friendly applications. Moreover, the ability to return values enables developers to debug and test their code more effectively, identify errors, and optimize performance. As we explore the different ways VBA returns values, we will examine the benefits and challenges of each approach, providing practical examples and tips for implementation.
Returning Values from Functions
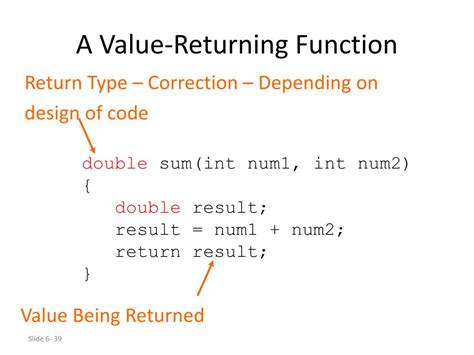
For example, consider a simple function that calculates the area of a rectangle:
Function CalculateArea(width As Double, height As Double) As Double
CalculateArea = width * height
End Function
In this example, the CalculateArea
function takes two arguments, width
and height
, and returns their product. The returned value can be assigned to a variable, like this:
Dim area As Double
area = CalculateArea(10, 20)
Alternatively, the returned value can be used directly in an expression:
Debug.Print CalculateArea(10, 20)
Returning Values from Properties
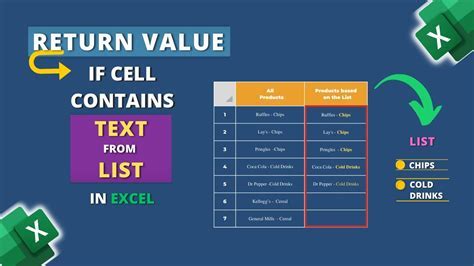
For example, consider a simple class that represents a person:
Class Person
Private mName As String
Public Property Get Name() As String
Name = mName
End Property
Public Property Let Name(value As String)
mName = value
End Property
End Class
In this example, the Person
class has a Name
property that returns a string value. The property can be accessed like this:
Dim person As New Person
person.Name = "John Doe"
Debug.Print person.Name
Returning Values from Methods
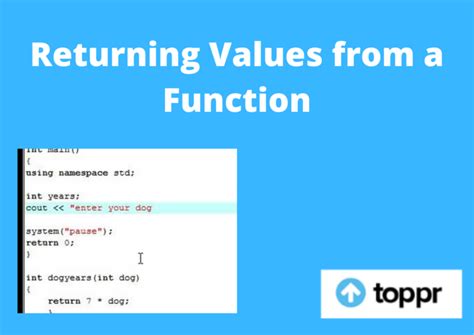
For example, consider a simple class that represents a calculator:
Class Calculator
Public Function Add(x As Double, y As Double) As Double
Add = x + y
End Function
Public Function Subtract(x As Double, y As Double) As Double
Subtract = x - y
End Function
End Class
In this example, the Calculator
class has two methods, Add
and Subtract
, that return double values. The methods can be called like this:
Dim calculator As New Calculator
Dim result As Double
result = calculator.Add(10, 20)
Debug.Print result
Returning Values from Events

For example, consider a simple class that represents a button:
Class Button
Public Event Click()
Public Sub RaiseClick()
RaiseEvent Click
End Sub
End Class
In this example, the Button
class has a Click
event that returns no value. The event can be raised like this:
Dim button As New Button
button.RaiseClick
To handle the event, you can use the WithEvents
keyword:
Dim WithEvents button As Button
Private Sub button_Click()
Debug.Print "Button clicked"
End Sub
Returning Values from Expressions

For example, consider a simple expression that calculates the area of a rectangle:
Dim area As Double
area = 10 * 20
In this example, the expression 10 * 20
returns a double value, which is assigned to the area
variable.
Gallery of VBA Return Values
VBA Return Values Image Gallery
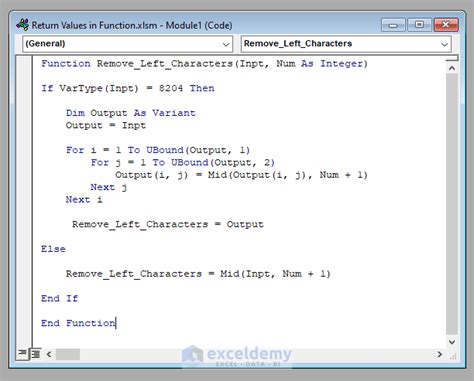
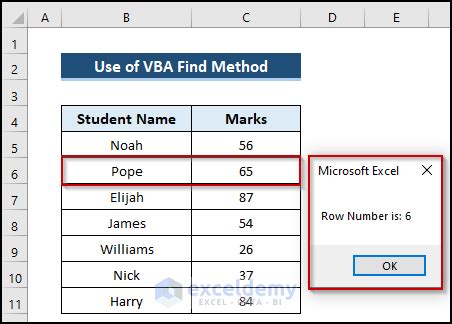
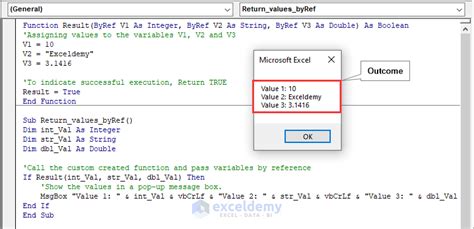
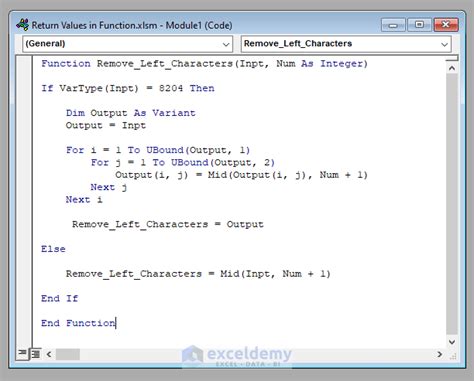
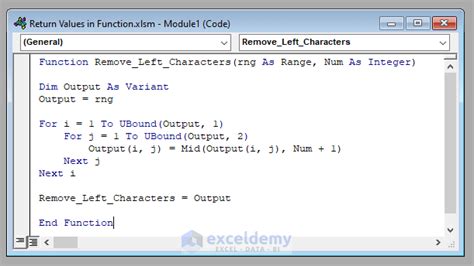

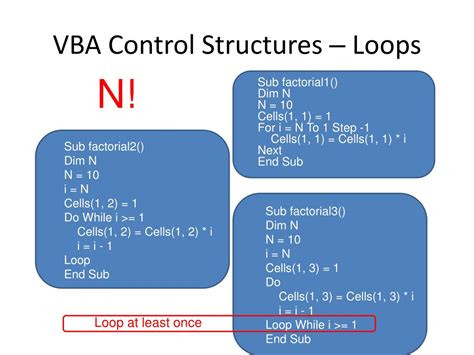
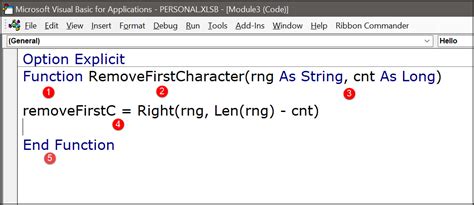
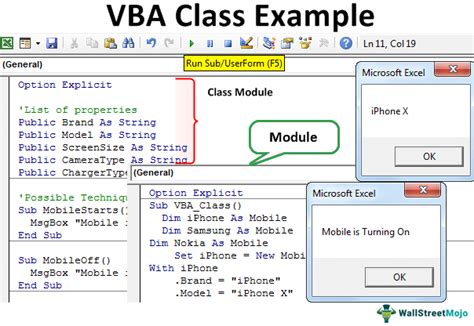
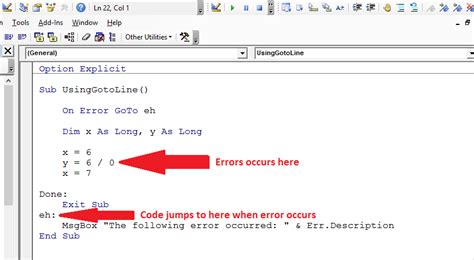
What are the different ways VBA returns values?
+VBA returns values through functions, properties, methods, events, and expressions.
How do I define a function in VBA?
+You can define a function in VBA using the `Function` keyword, followed by the function name and parameters.
What is the difference between a function and a method in VBA?
+A function is a block of code that performs a specific task and returns a value, while a method is a member of an object that performs an action and returns a value.
How do I handle events in VBA?
+You can handle events in VBA using the `WithEvents` keyword and defining an event handler procedure.
What are the benefits of using VBA to return values?
+The benefits of using VBA to return values include improved code readability, reduced errors, and increased flexibility.
In conclusion, VBA returns values through a variety of mechanisms, including functions, properties, methods, events, and expressions. Understanding these mechanisms is essential for writing effective and efficient VBA code. By mastering the different ways VBA returns values, developers can create more robust, flexible, and user-friendly applications. Whether you are a seasoned developer or just starting to explore the world of VBA, we hope this article has provided you with a deeper understanding of the language and its capabilities. If you have any questions or comments, please don't hesitate to reach out. Share this article with your colleagues and friends, and let's continue the conversation about the power and flexibility of VBA.