Intro
Master Excel VBA Round Up techniques with expert tips and tricks, including rounding numbers, formatting cells, and using VBA macros for efficient data management and calculation.
The importance of rounding numbers in Excel VBA cannot be overstated. Rounding is a crucial step in many calculations, as it helps to simplify complex numbers and make them more manageable. In Excel VBA, rounding can be achieved through various methods, each with its own strengths and weaknesses. In this article, we will delve into the world of Excel VBA rounding, exploring the different techniques and functions available to users.
Rounding numbers is a fundamental concept in mathematics, and it is essential in many real-world applications. For instance, in finance, rounding is used to calculate interest rates and investment returns. In science, rounding is used to simplify complex measurements and calculations. In Excel VBA, rounding is used to perform calculations, format numbers, and make decisions based on numerical values. With the help of Excel VBA, users can automate rounding tasks, making it easier to work with large datasets and complex calculations.
The need for rounding in Excel VBA arises from the fact that many calculations involve decimal numbers. Decimal numbers can be cumbersome to work with, especially when dealing with large datasets. Rounding helps to simplify these numbers, making it easier to perform calculations and make decisions. Furthermore, rounding is essential in many industries, such as finance, engineering, and science, where precision is critical. By using Excel VBA to round numbers, users can ensure accuracy and precision in their calculations, which is essential for making informed decisions.
Introduction to Excel VBA Rounding
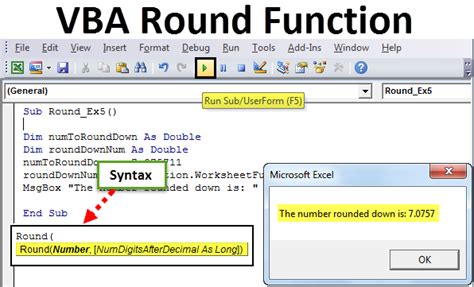
Excel VBA provides several functions for rounding numbers, including the Round, RoundUp, and RoundDown functions. These functions can be used to round numbers to a specified number of decimal places or to the nearest integer. The Round function is the most commonly used rounding function in Excel VBA, as it allows users to round numbers to a specified number of decimal places. The RoundUp and RoundDown functions, on the other hand, are used to round numbers up or down to the nearest integer.
Rounding Functions in Excel VBA
The Round function in Excel VBA is used to round a number to a specified number of decimal places. The syntax for the Round function is `Round(number, num_digits)`, where `number` is the number to be rounded and `num_digits` is the number of decimal places to round to. For example, `Round(12.345, 2)` would return `12.35`. The RoundUp and RoundDown functions, on the other hand, are used to round numbers up or down to the nearest integer. The syntax for these functions is `RoundUp(number)` and `RoundDown(number)`, respectively.Using the Round Function in Excel VBA
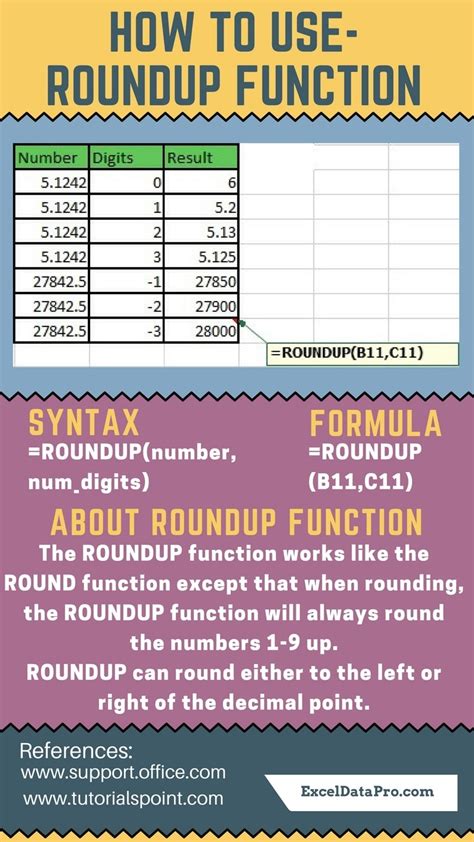
The Round function is a powerful tool in Excel VBA, as it allows users to round numbers to a specified number of decimal places. This function is commonly used in financial calculations, such as calculating interest rates and investment returns. For example, if you want to calculate the interest rate on a loan, you can use the Round function to round the interest rate to two decimal places. Here is an example of how to use the Round function in Excel VBA:
Sub CalculateInterestRate()
Dim interestRate As Double
interestRate = 0.123456
interestRate = Round(interestRate, 2)
MsgBox "The interest rate is: " & interestRate
End Sub
This code calculates the interest rate and rounds it to two decimal places using the Round function.
Rounding Numbers Up or Down
The RoundUp and RoundDown functions in Excel VBA are used to round numbers up or down to the nearest integer. These functions are commonly used in calculations where precision is critical, such as in engineering and science. For example, if you want to calculate the number of units to produce, you can use the RoundUp function to round up to the nearest whole number. Here is an example of how to use the RoundUp function in Excel VBA: ```vb Sub CalculateUnitsToProduce() Dim unitsToProduce As Double unitsToProduce = 12.5 unitsToProduce = RoundUp(unitsToProduce) MsgBox "The number of units to produce is: " & unitsToProduce End Sub ``` This code calculates the number of units to produce and rounds up to the nearest whole number using the RoundUp function.Best Practices for Rounding in Excel VBA
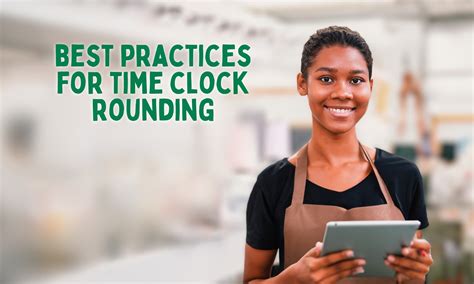
When using the Round function in Excel VBA, there are several best practices to keep in mind. First, it is essential to specify the correct number of decimal places to round to. If you do not specify the number of decimal places, the Round function will round to the nearest integer. Second, it is crucial to use the correct rounding function for your calculation. For example, if you want to round up to the nearest whole number, use the RoundUp function. Finally, it is essential to test your code thoroughly to ensure that the rounding is accurate and precise.
Common Mistakes to Avoid
When using the Round function in Excel VBA, there are several common mistakes to avoid. First, do not use the Round function to round numbers to a large number of decimal places. This can lead to precision errors and inaccurate results. Second, do not use the RoundUp or RoundDown functions to round numbers to a specified number of decimal places. These functions are designed to round numbers up or down to the nearest integer, not to a specified number of decimal places. Finally, do not forget to test your code thoroughly to ensure that the rounding is accurate and precise.Advanced Rounding Techniques in Excel VBA
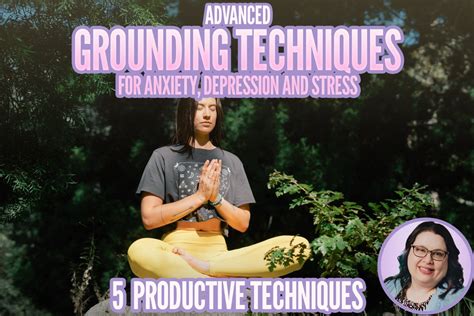
In addition to the basic rounding functions, Excel VBA provides several advanced rounding techniques. One of these techniques is the use of the Application.WorksheetFunction.Round
function. This function allows users to round numbers to a specified number of decimal places, using the same syntax as the Round function. Another advanced technique is the use of the Format
function, which allows users to format numbers to a specified number of decimal places.
Using the Application.WorksheetFunction.Round Function
The `Application.WorksheetFunction.Round` function is a powerful tool in Excel VBA, as it allows users to round numbers to a specified number of decimal places. This function is commonly used in financial calculations, such as calculating interest rates and investment returns. For example, if you want to calculate the interest rate on a loan, you can use the `Application.WorksheetFunction.Round` function to round the interest rate to two decimal places. Here is an example of how to use the `Application.WorksheetFunction.Round` function in Excel VBA: ```vb Sub CalculateInterestRate() Dim interestRate As Double interestRate = 0.123456 interestRate = Application.WorksheetFunction.Round(interestRate, 2) MsgBox "The interest rate is: " & interestRate End Sub ``` This code calculates the interest rate and rounds it to two decimal places using the `Application.WorksheetFunction.Round` function.Gallery of Excel VBA Rounding Examples
Excel VBA Rounding Image Gallery
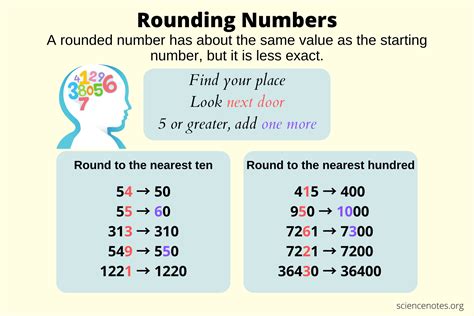
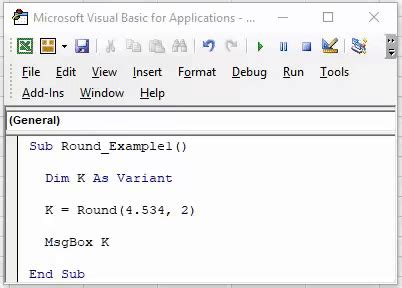
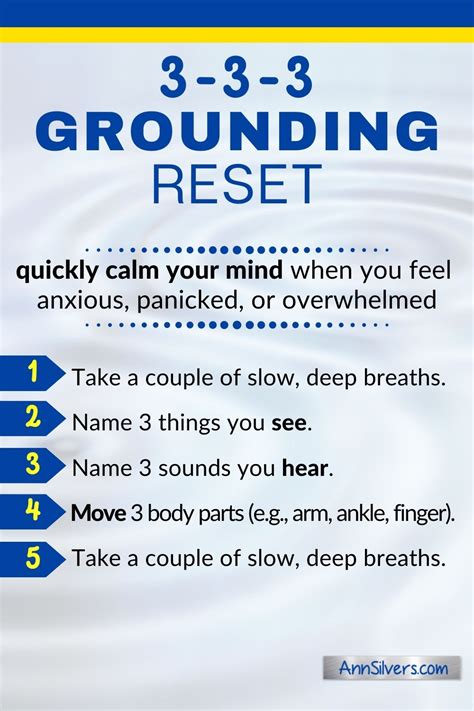
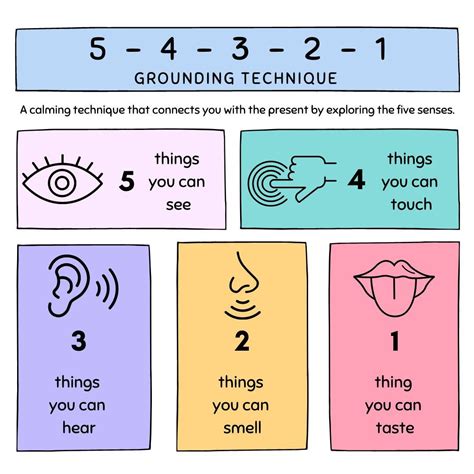
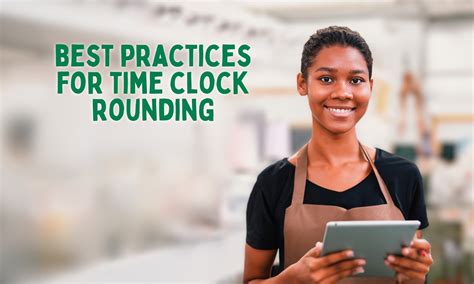
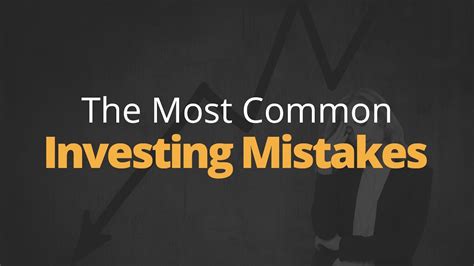
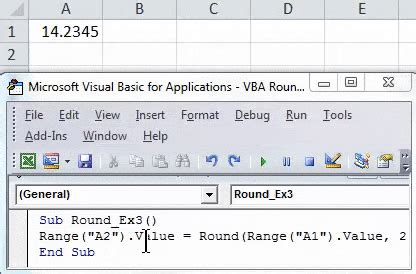
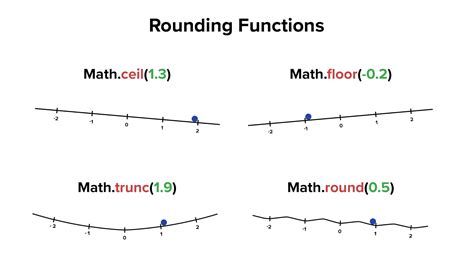
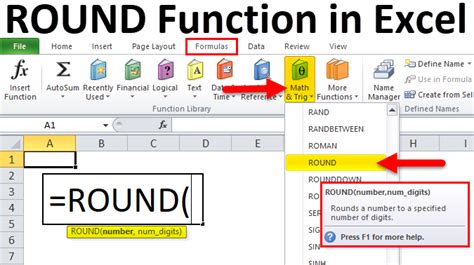
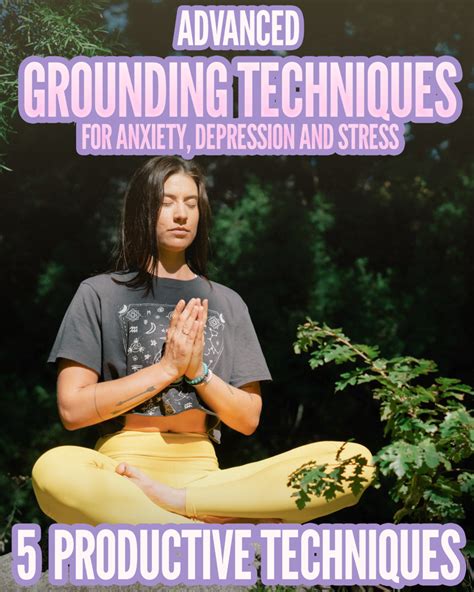
Frequently Asked Questions
What is the purpose of the Round function in Excel VBA?
+The Round function is used to round a number to a specified number of decimal places.
How do I use the RoundUp function in Excel VBA?
+The RoundUp function is used to round a number up to the nearest integer. The syntax for the RoundUp function is `RoundUp(number)`.
What is the difference between the Round and Application.WorksheetFunction.Round functions?
+The Round function and the Application.WorksheetFunction.Round function are both used to round numbers to a specified number of decimal places. However, the Application.WorksheetFunction.Round function is a more advanced function that allows users to round numbers using the same syntax as the Round function.
How do I avoid common mistakes when using the Round function in Excel VBA?
+To avoid common mistakes when using the Round function in Excel VBA, make sure to specify the correct number of decimal places to round to, use the correct rounding function for your calculation, and test your code thoroughly to ensure that the rounding is accurate and precise.
What are some best practices for rounding in Excel VBA?
+Some best practices for rounding in Excel VBA include specifying the correct number of decimal places to round to, using the correct rounding function for your calculation, and testing your code thoroughly to ensure that the rounding is accurate and precise.
In conclusion, rounding numbers is a crucial step in many calculations, and Excel VBA provides several functions and techniques for rounding numbers. By understanding how to use the Round function, RoundUp function, and Application.WorksheetFunction.Round function, users can perform calculations with precision and accuracy. Additionally, by following best practices and avoiding common mistakes, users can ensure that their code is efficient and effective. We hope this article has provided you with a comprehensive understanding of Excel VBA rounding and has helped you to improve your skills in using this powerful tool. If you have any questions or comments, please do not hesitate to reach out to us. Share this article with your friends and colleagues who may benefit from learning about Excel VBA rounding.