Intro
Discover how to find the last row in Excel using VBA, with tips on dynamic range, worksheet loops, and error handling for efficient data processing and automation.
Finding the last row in a dataset is a common task when working with Excel VBA. This is crucial for looping through data, copying ranges, or performing other operations that require knowing the extent of your data. The method you choose to find the last row can significantly impact the performance and reliability of your code, especially when dealing with large datasets or worksheets that have blank rows or columns.
Importance of Finding the Last Row
Before diving into the methods, it's essential to understand why finding the last row is important:
- Efficient Looping: When you need to perform an operation on each row in a dataset, knowing the last row allows you to loop through your data efficiently without unnecessarily checking empty rows.
- Data Integrity: Incorrectly assuming the last row can lead to data corruption or omission, especially when copying, moving, or deleting data.
- Performance: Checking every row in a worksheet to find data is inefficient. Excel worksheets can have over a million rows, so looping through all of them when your data only goes up to row 100, for example, is a waste of resources.
Methods to Find the Last Row
There are several methods to find the last row in Excel VBA, each with its own strengths and weaknesses.
1. Using Cells.Find
This method is robust and can find the last row with data, even if there are gaps in the data.
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlRows, SearchDirection:=xlPrevious, LookIn:=xlValues).Row
2. Using Cells.SpecialCells
This method is quick but can be unreliable if your worksheet has formatting in blank cells.
Dim lastRow As Long
lastRow = Cells.SpecialCells(xlCellTypeLastCell).Row
3. Using UsedRange.Rows.Count
This method considers the used range of the worksheet, which might not always accurately reflect the last row with data, especially if there are formatting or other non-data entries in cells.
Dim lastRow As Long
lastRow = UsedRange.Rows.Count
4. Looping Through Rows
A brute-force approach that checks each row for data. This is not efficient for large datasets.
Dim lastRow As Long
Dim i As Long
For i = Rows.Count To 1 Step -1
If Not IsEmpty(Cells(i, 1).Value) Then
lastRow = i
Exit For
End If
Next i
Choosing the Right Method
The best method depends on your specific needs:
- Robustness:
Cells.Find
is generally the most reliable as it directly searches for the last cell with data. - Speed: For very large datasets, a targeted loop or
UsedRange
might be faster, but beware of potential inaccuracies. - Specific Column: If you're only interested in the last row of a specific column, modify the loop method to check that column specifically.
Example Use Case
Suppose you want to copy data from column A to column B, starting from row 1 to the last row with data in column A.
Sub CopyData()
Dim lastRow As Long
lastRow = Cells.Find(What:="*", SearchOrder:=xlRows, SearchDirection:=xlPrevious, LookIn:=xlValues).Row
' Assuming data starts from row 1 and is continuous
Range("A1:A" & lastRow).Copy Destination:=Range("B1")
End Sub
Practical Considerations
- Worksheet Activation: Ensure the worksheet you're working with is active, or explicitly reference it in your code.
- Error Handling: Implement error handling in case
Find
doesn't locate any data (e.g., a completely blank worksheet). - Performance Optimization: For large datasets, consider optimizing your code by reducing the number of interactions with the worksheet, such as by working with arrays.
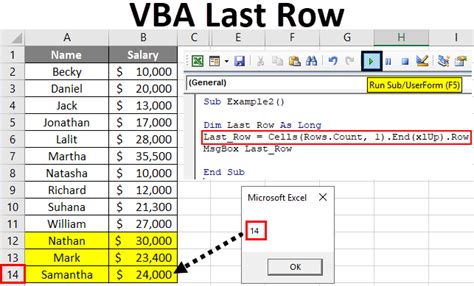
Gallery of Excel VBA Tips
Excel VBA Tips Image Gallery
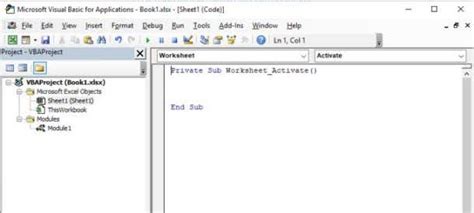
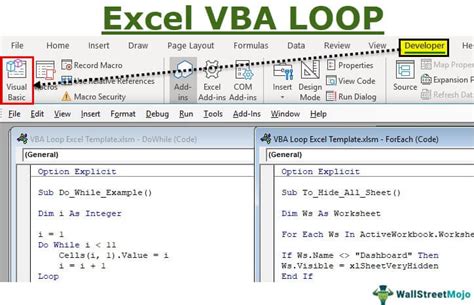
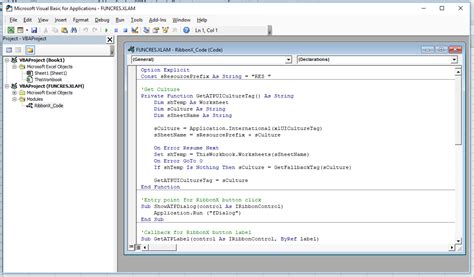
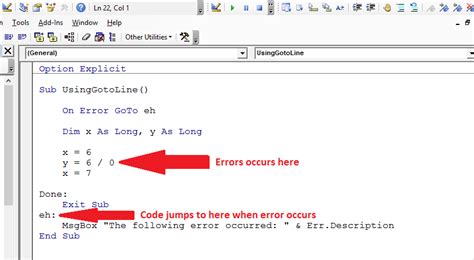
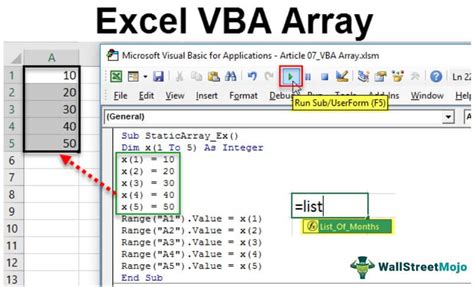
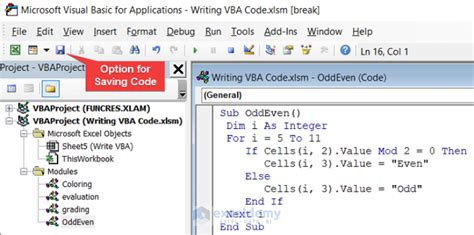

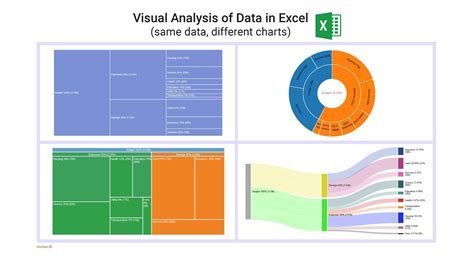
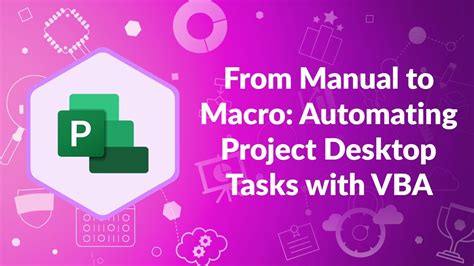
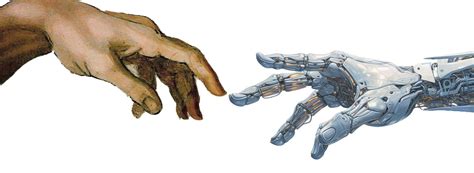
FAQs
What is the most reliable method to find the last row in Excel VBA?
+The most reliable method is using `Cells.Find` with the `SearchDirection` set to `xlPrevious` and `LookIn` set to `xlValues`. This method directly searches for the last cell with data.
How can I optimize my VBA code to run faster?
+Optimizing VBA code involves reducing interactions with the worksheet, using arrays for data manipulation, avoiding unnecessary loops, and applying other best practices such as disabling screen updating and calculations during execution.
What is the purpose of error handling in VBA?
+Error handling is crucial in VBA as it allows the code to gracefully manage and recover from errors, providing a better user experience and preventing data loss or corruption.
In conclusion, finding the last row in Excel VBA is a fundamental skill that can significantly impact the efficiency and reliability of your macros. By choosing the right method for your specific needs and optimizing your code, you can create powerful and robust VBA applications. Remember to always consider the context and potential pitfalls of each method to ensure your code works as intended across different datasets and scenarios. If you have any further questions or need more detailed guidance on implementing these methods, don't hesitate to ask. Sharing your experiences or tips on working with Excel VBA can also help others in the community.