Intro
Master VBA time formatting with 5 expert ways, including date and time functions, formatting codes, and worksheet manipulation, to efficiently manage time-based data in Excel.
Time management is crucial in various aspects of life, and when it comes to working with Microsoft Excel, being able to format time effectively can significantly enhance productivity. Visual Basic for Applications (VBA) is a powerful tool within Excel that allows users to automate tasks, including time formatting. Here, we'll delve into five ways to format time using VBA, exploring each method's benefits and applications.
The ability to manipulate and format time in Excel VBA opens up a wide range of possibilities, from creating automated reports that include time stamps to developing complex scheduling systems. Understanding how to work with time in VBA can help users create more efficient and user-friendly spreadsheets.
To begin working with time in VBA, it's essential to understand the basic time formatting options available. Excel stores times as decimal values, where midnight is 0, noon is 0.5, and so on. This understanding forms the basis of how we format and manipulate time in VBA.
Understanding Time in VBA
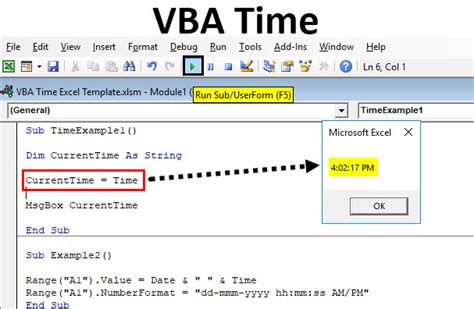
Before diving into the specific methods of formatting time, it's crucial to grasp how VBA interprets time. The Time
data type in VBA represents a time of day, stored internally as a fractional day. This means that when you work with times in VBA, you're essentially working with decimal numbers that represent portions of a 24-hour day.
Method 1: Basic Time Formatting
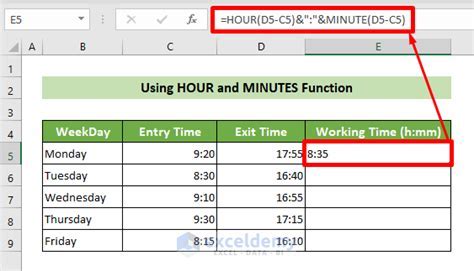
The most straightforward way to format time in VBA involves using the Format
function. This function allows you to specify how a time value should be displayed. For example, if you want to display the current time in a 12-hour format with AM/PM, you can use the Format
function with the "hh:mm:ss AM/PM" format string.
Sub DisplayCurrentTime()
Dim currentTime As Date
currentTime = Now()
MsgBox Format(currentTime, "hh:mm:ss AM/PM")
End Sub
This code snippet demonstrates how to display the current time in a message box, formatted as desired.
Method 2: Custom Time Formatting
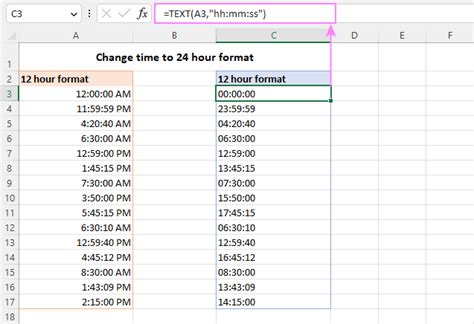
Beyond basic formatting, VBA allows for custom time formatting using specific format codes. These codes enable you to tailor the display of time to your exact needs. For instance, you might want to display time in a 24-hour format without seconds, which can be achieved using the "hh:mm" format string.
Sub DisplayCustomTime()
Dim customTime As Date
customTime = #12:30:00 PM#
MsgBox Format(customTime, "hh:mm")
End Sub
This example shows how to format a specific time, removing the seconds component.
Method 3: Time Calculations
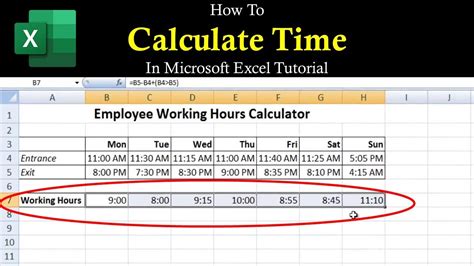
VBA also facilitates time calculations, such as determining the time difference between two dates or adding/subtracting time intervals. This is particularly useful in scheduling applications or when analyzing time-based data.
Sub CalculateTimeDifference()
Dim startTime As Date
Dim endTime As Date
Dim timeDifference As Long
startTime = #8:00:00 AM#
endTime = #5:00:00 PM#
timeDifference = DateDiff("n", startTime, endTime)
MsgBox "Time difference in minutes: " & timeDifference
End Sub
This code calculates the time difference in minutes between a start and end time.
Method 4: Time Validation
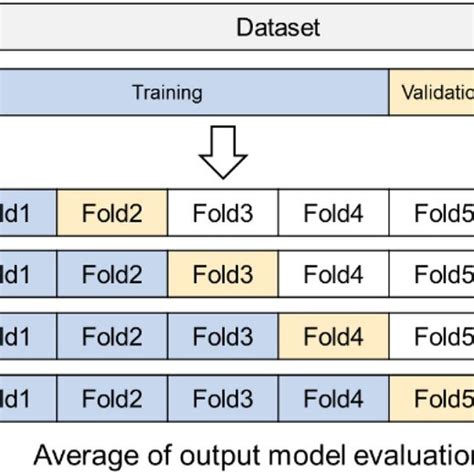
Validating user-input time is essential to ensure that the data entered into your application or spreadsheet is correct and usable. VBA provides mechanisms to validate time inputs, such as checking if a time falls within a specific range.
Sub ValidateTimeInput()
Dim userInput As String
Dim isValid As Boolean
userInput = InputBox("Enter time in hh:mm format")
If IsDate(userInput) Then
isValid = True
Else
isValid = False
End If
If isValid Then
MsgBox "Time is valid"
Else
MsgBox "Invalid time format"
End If
End Sub
This example demonstrates a basic approach to validating time inputs.
Method 5: Automated Time Stamping
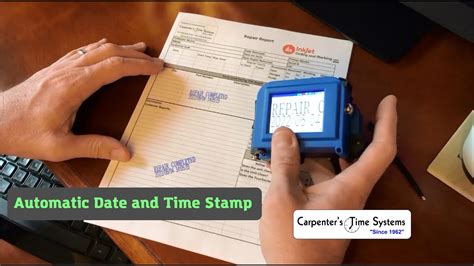
Finally, VBA can be used to automate the process of time stamping in Excel. This involves automatically recording the current time when a specific action occurs, such as when a worksheet is changed or when a button is clicked.
Sub AutoTimeStamp()
Dim currentTime As Date
currentTime = Now()
Range("A1").Value = Format(currentTime, "yyyy-mm-dd hh:mm:ss")
End Sub
This code snippet shows how to automatically insert the current date and time into a cell.
Time Formatting in VBA Image Gallery
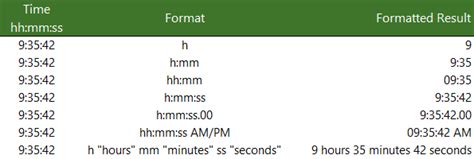
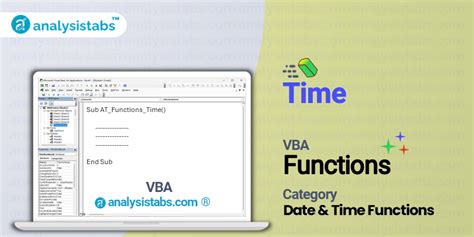
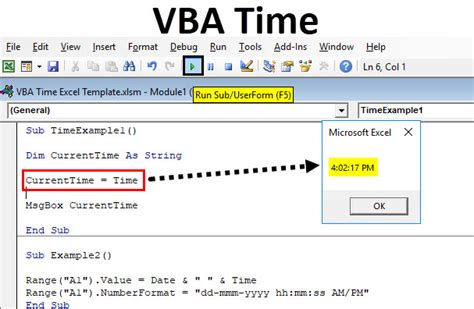
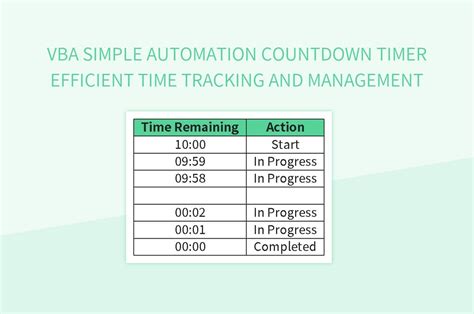
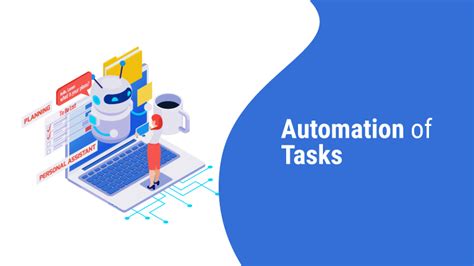
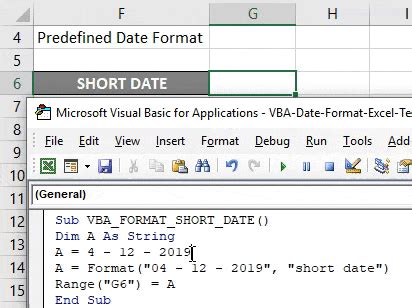
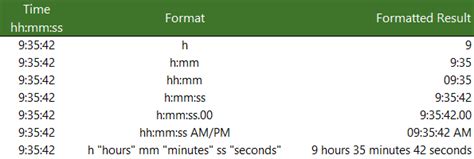
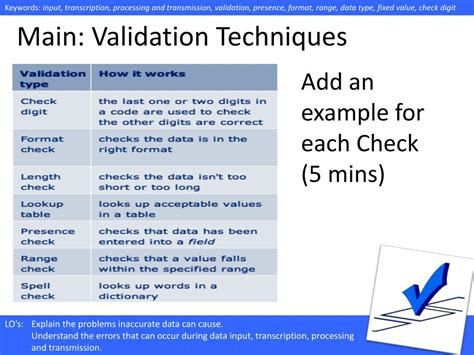
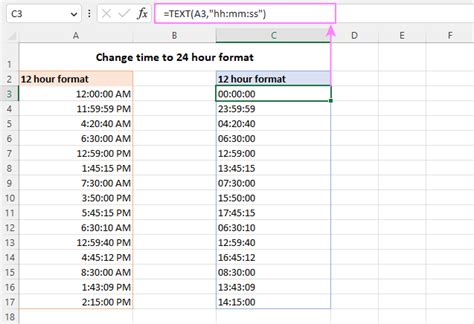
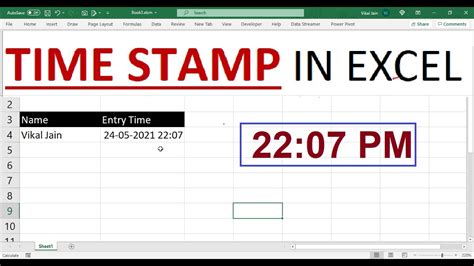
What is the purpose of formatting time in VBA?
+The primary purpose of formatting time in VBA is to display time values in a readable and understandable format, which can vary depending on the application or user preference.
How do I format time in a 24-hour format without seconds in VBA?
+To format time in a 24-hour format without seconds, you can use the "hh:mm" format string with the Format function in VBA.
Can I automate time stamping in Excel using VBA?
+Yes, VBA can be used to automate the process of time stamping in Excel, allowing you to automatically record the current time when a specific action occurs.
What are some common applications of time formatting in VBA?
+Common applications include creating automated reports, developing scheduling systems, and analyzing time-based data.
How do I validate user-input time in VBA?
+You can validate user-input time by checking if the input is a valid date using the IsDate function and ensuring it matches the required format.
In conclusion, mastering the art of formatting time in VBA can significantly enhance your productivity and the functionality of your Excel applications. Whether you're looking to automate reports, create complex schedules, or simply display time in a more user-friendly format, the methods outlined above provide a comprehensive foundation for working with time in VBA. We invite you to explore these methods further, share your experiences with time formatting in VBA, and discover how these techniques can be applied to real-world scenarios to streamline your workflow and improve your overall efficiency.